The `clear` command in MATLAB removes variables from the workspace, freeing up system memory and ensuring that scripts run with fresh variables.
clear myVariable; % Removes 'myVariable' from the workspace
Understanding the `clear` Command
The `clear` command in MATLAB serves a critical purpose: it helps manage memory by removing specified variables or all variables from the workspace. By effectively utilizing `clear`, you can ensure that your MATLAB environment is organized and free from unnecessary variables that may cause confusion or consume valuable memory resources.
The basic syntax of the `clear` command is straightforward:
clear
When executed, this command removes all variables from the current workspace, allowing you to start fresh without any clutter.
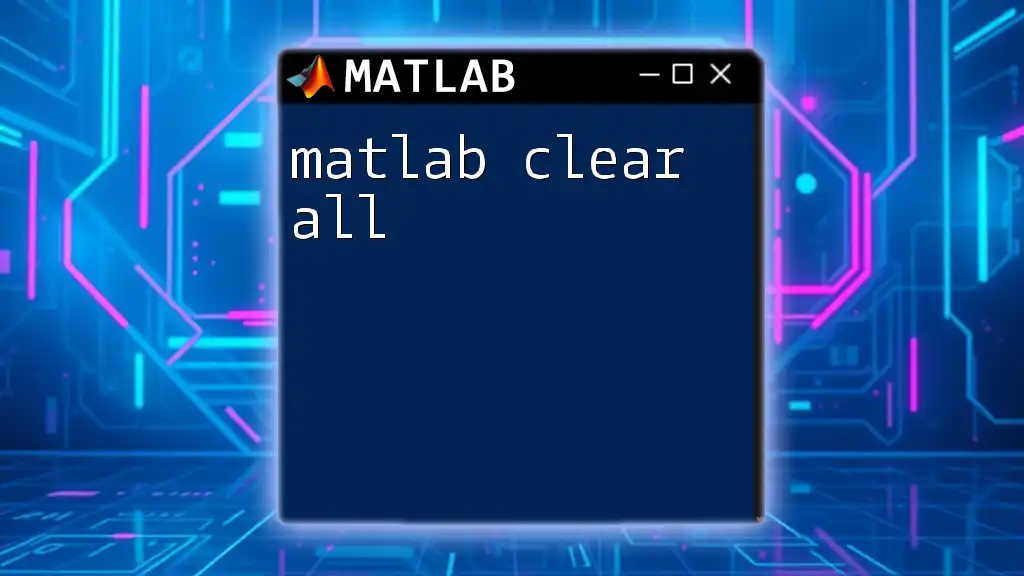
How to Use the `clear` Command
Clearing Variables
One of the most fundamental uses of `clear` is to remove all variables currently stored in the workspace. This is especially useful when you're running multiple scripts or functions and want a clean slate. Consider the following example:
a = 10;
b = 20;
clear; % This will remove 'a' and 'b' from the workspace
Once you execute `clear`, attempting to access `a` or `b` will result in an error, as they no longer exist in the workspace.
Clearing Specific Variables
Sometimes, you may not want to erase everything but only specific variables. In such cases, you can specify which variables to clear. The syntax is as follows:
clear variableName1 variableName2
For example:
a = 10;
b = 20;
clear a; % This will remove 'a' but keep 'b'
After running this code, only the variable `b` will remain available in the workspace, while `a` will be cleared.
Clearing Functions and Global Variables
Beyond variables, `clear` can also be used to manage functions and global variables. To clear all functions from memory, you can use:
clear functions
Clearing global variables is similarly straightforward:
clear global
Here’s an example:
global gVar;
gVar = 5;
clear global; % This removes 'gVar' from the global scope
After executing this command, `gVar` will no longer be accessible in your workspace.
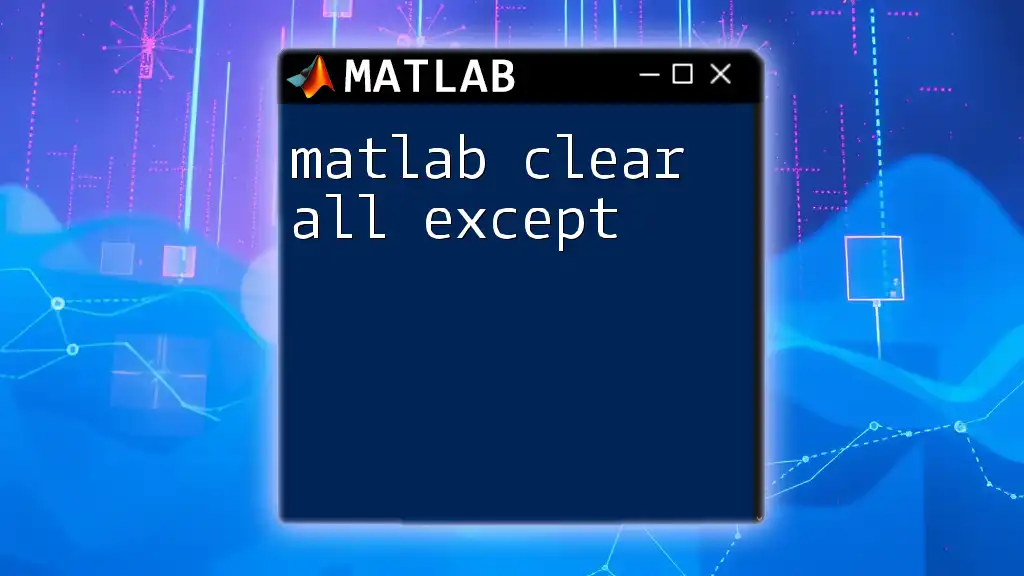
Key Variants of the `clear` Command
Using `clear all`
The command `clear all` is a more comprehensive approach. When executed, it removes all variables, global variables, and even MEX-files from memory. This command can be particularly useful if you're working in a large program and need to ensure no remnants from previous executions are left.
The syntax is:
clear all
For instance:
clear all; % This removes all variables, functions, and MEX files
However, it’s essential to use this command judiciously, as it can significantly impact performance by forcing MATLAB to reload functions and variables when they are next referenced.
Using `clearvars`
For users seeking more control over their workspace, `clearvars` provides granular command options. The syntax allows you to specify which variables to retain in the workspace:
clearvars -except variableName
Here's an example:
a = 10; b = 20; c = 30;
clearvars -except c; % Keeps 'c' while removing 'a' and 'b'
This command is particularly beneficial when you want to keep certain variables while clearing out the others, maintaining essential data without losing context.
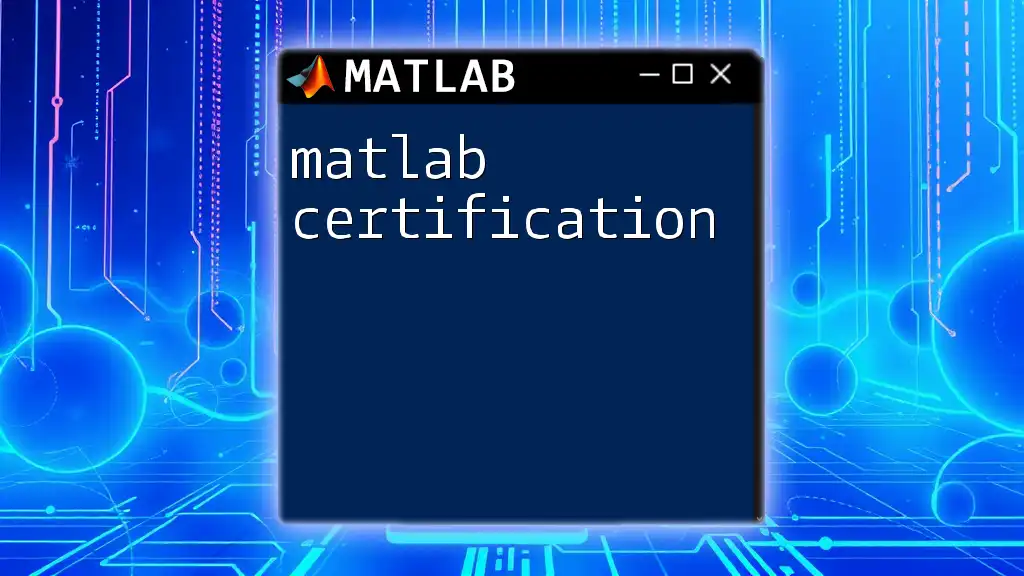
Important Considerations When Using `clear`
Impacts on Performance
While `clear` is a powerful tool for managing memory, excessive use can lead to performance issues. Each time `clear` is executed, MATLAB has to reload the variables or functions that you subsequently reference, which could slow down your workflow. Thus, it’s advisable to strategically decide when to use the `clear` command.
Use in Scripts vs. Functions
Another consideration is the behavior of `clear` within scripts compared to functions. In scripts, `clear` affects the global workspace, while in functions, it affects only that function's local workspace. Understanding the scope of each command helps in avoiding unintended deletions, especially in collaborative projects.
Noteworthy Limitations
When using `clear` in the context of object-oriented programming, it’s important to note that it doesn't clear objects from memory; rather, it deletes the references to them. This limitation means that you still need to manage object lifecycles to ensure effective memory management.
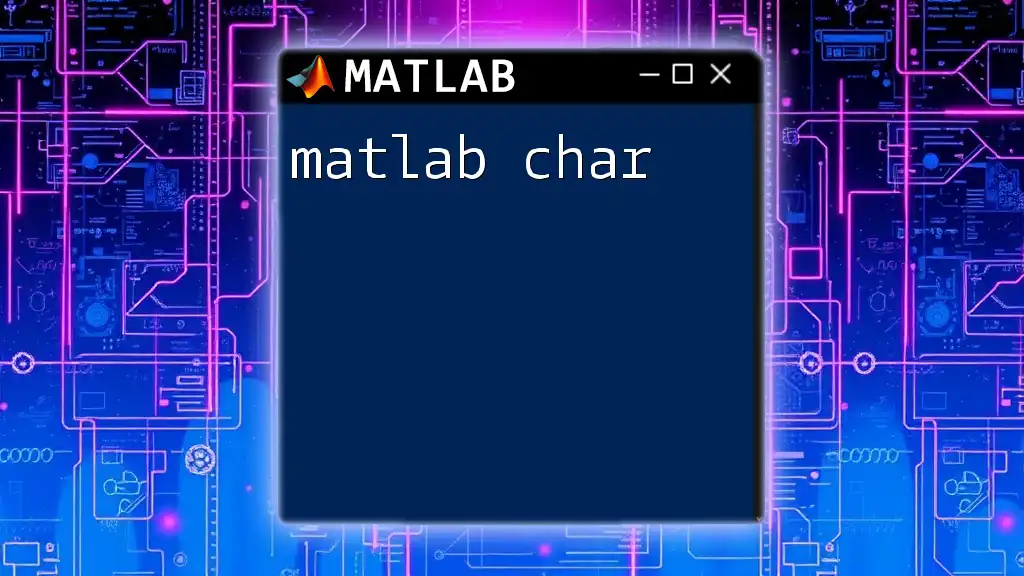
Best Practices for Memory Management with `clear`
When to Use `clear`
Use `clear` strategically when:
- You are starting a new analysis and want to ensure no previous variables interfere.
- Your workspace has become cluttered with temporary variables that are no longer needed.
Alternatives to `clear`
Rather than relying heavily on `clear`, consider employing local variables within functions or scripts. Limiting the scope of variables naturally promotes a cleaner workspace without the need for constant clearing, thereby enhancing performance.
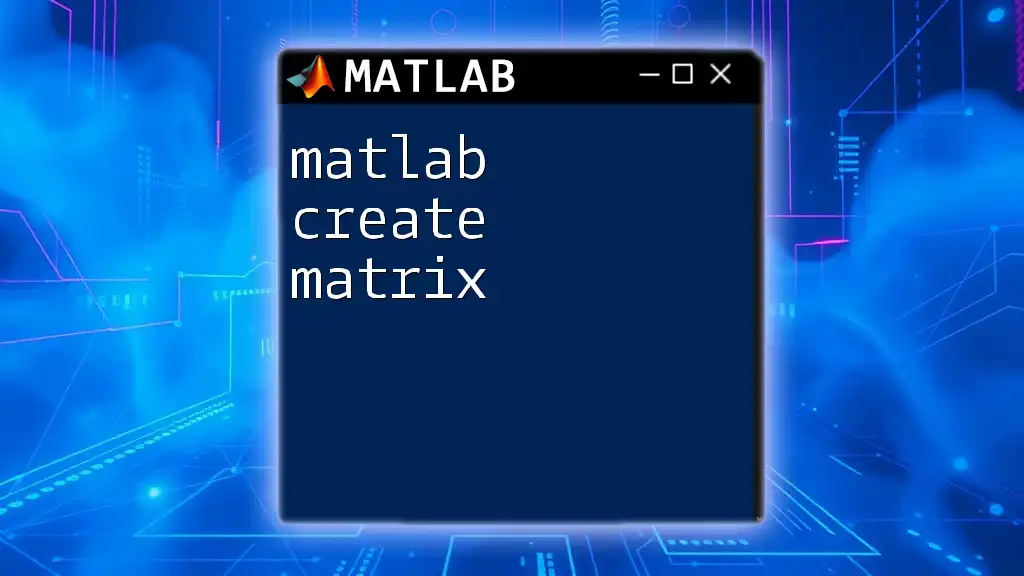
Common Mistakes and Troubleshooting Tips
Misunderstanding Scope
One common mistake users make is misjudging how `clear` interacts with variable scope. Users may think they’re clearing variables from a script when, in reality, they’re only affecting the function's scope. Always review the intended scope before using `clear`.
Using `clear` Too Frequently
Overuse of `clear` can lead to unnecessary performance issues. Instead, aim for structured coding practices, including better variable naming conventions, to reduce confusion without needing an ongoing cleanup.
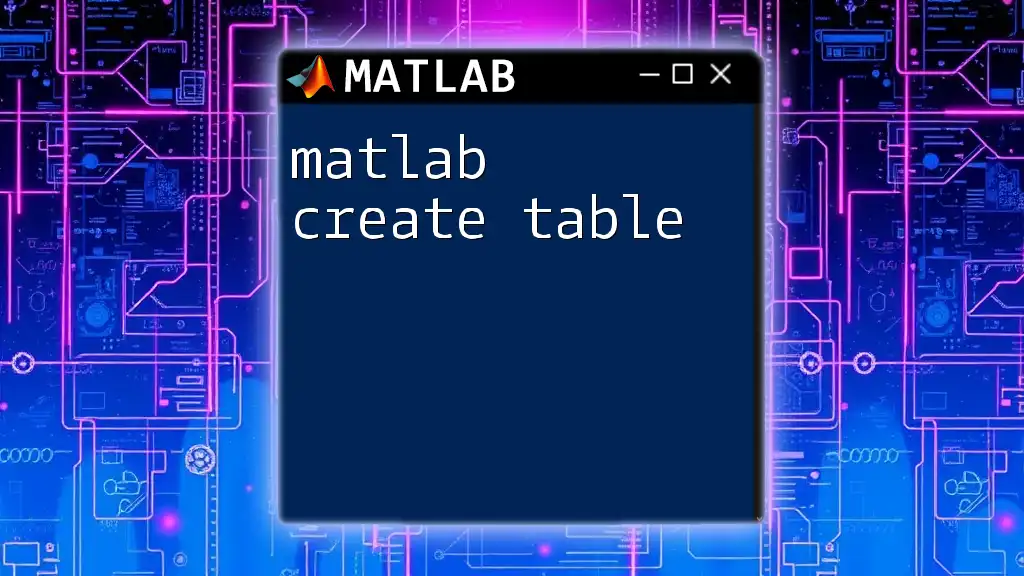
Conclusion
The MATLAB clear command is indispensable for managing your workspace efficiently. By understanding how and when to use `clear`, you can streamline your MATLAB operations and improve overall programming performance. Practice using the command with various examples, as hands-on experience will enhance your proficiency in MATLAB memory management.
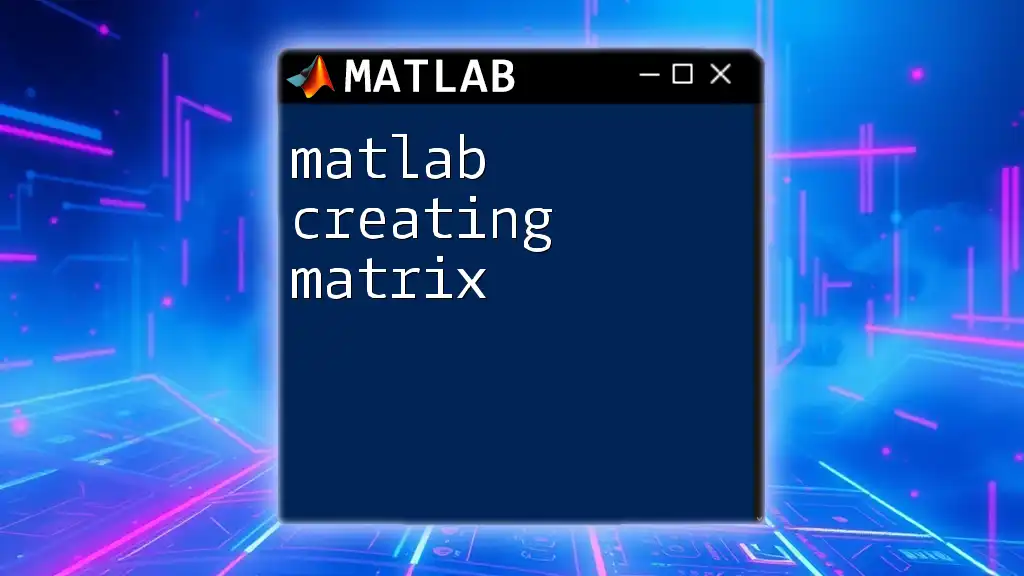
Resources for Further Learning
To further your knowledge, refer to the official MATLAB documentation for an in-depth understanding of the `clear` command and explore related commands. Engaging in dedicated exercises will deepen your practical understanding and increase your efficiency as you work with MATLAB.