In MATLAB, you can create a matrix by specifying its elements in square brackets, using spaces or commas to separate elements in a row and semicolons to separate rows.
Here's a code snippet to illustrate how to create a 2x3 matrix:
A = [1 2 3; 4 5 6];
Understanding Matrices in MATLAB
What is a Matrix?
A matrix is a rectangular array of numbers, symbols, or expressions arranged in rows and columns. In essence, any matrix can be represented as a two-dimensional dataset. The size of the matrix is defined by its dimensions, which are described as the number of rows and columns it contains. For example, a matrix with 3 rows and 4 columns is described as a 3x4 matrix.
Why Use Matrices?
Matrices play a crucial role in numerical computing and are fundamental in various fields, such as data analysis, engineering, machine learning, and computer graphics. They allow the representation of complex data structures efficiently and provide a basis for sophisticated mathematical operations, including transformations, decompositions, and solving systems of equations.
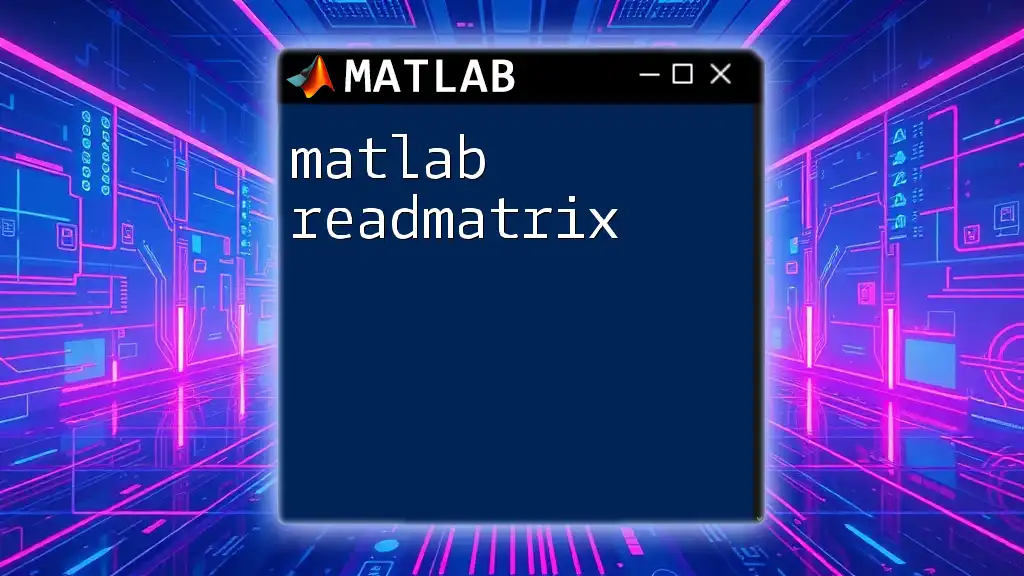
Creating Matrices in MATLAB
Basic Syntax for Matrix Creation
In MATLAB, creating a matrix is quite straightforward. You use square brackets to define the rows and columns. For instance, the following code creates a 2x2 matrix:
A = [1 2; 3 4];
In this example, the semicolon `;` indicates the end of a row. As a result, the first row contains the values `1` and `2`, while the second row contains `3` and `4`.
Creating Row and Column Vectors
Row Vectors
A row vector is a 1xN matrix, meaning it has one row and multiple columns. For example:
rowVector = [1 2 3 4];
This code creates a row vector with four elements.
Column Vectors
Conversely, a column vector is an Nx1 matrix with multiple rows and one column. You can create a column vector using semicolons to separate the rows:
colVector = [1; 2; 3; 4];
This defines a column vector with four rows.
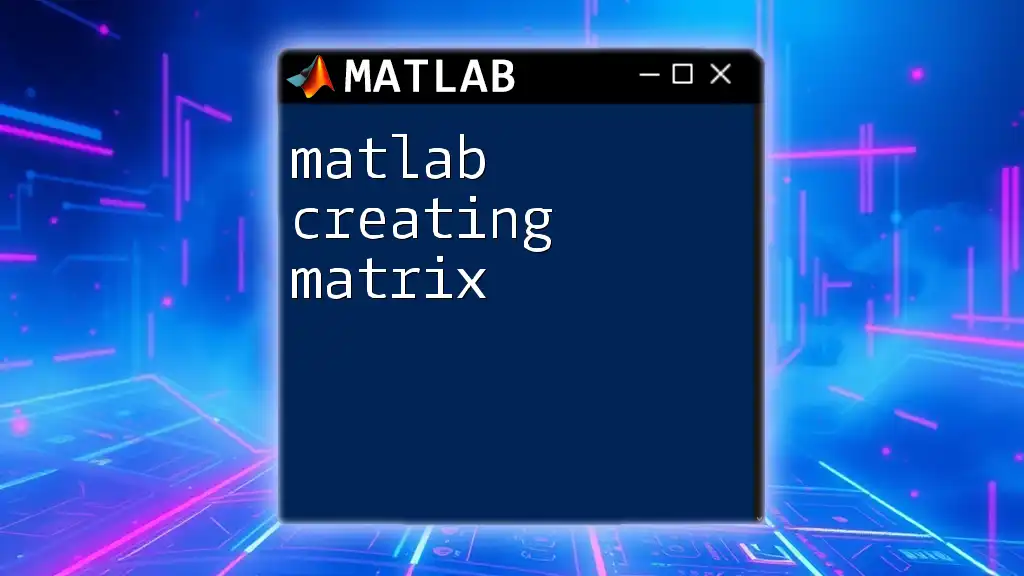
Specialized Matrix Creation Functions
Zeros, Ones, and Eye
Creating Zero Matrices
MATLAB provides a convenient function to create a matrix filled with zeros. The `zeros` function takes two arguments: the number of rows and the number of columns. For example:
zeroMatrix = zeros(3, 2); % Creates a 3x2 matrix of zeros
Creating One Matrices
Similarly, you can create a matrix filled with ones using the `ones` function:
oneMatrix = ones(2, 4); % Creates a 2x4 matrix of ones
Creating Identity Matrices
An identity matrix is a special square matrix with ones on the main diagonal and zeros elsewhere. You can create this using the `eye` function:
identityMatrix = eye(3); % Creates a 3x3 identity matrix
Other Useful Functions
Creating Random Matrices
MATLAB also features functions for generating matrices with random values. The `rand` function creates a matrix filled with uniformly distributed random numbers between 0 and 1:
randomMatrix = rand(3, 3); % Creates a 3x3 matrix with random values
You can use `randi` to create a matrix with random integers within a specified range:
randomIntMatrix = randi(10, 3, 3); % Creates a 3x3 matrix with random integers from 1 to 10
Creating Sparse Matrices
A sparse matrix is a matrix in which most of the elements are zero. Using MATLAB's sparse functionality can enhance performance when working with large datasets:
sparseMatrix = sparse(3, 3); % Creates a 3x3 sparse matrix
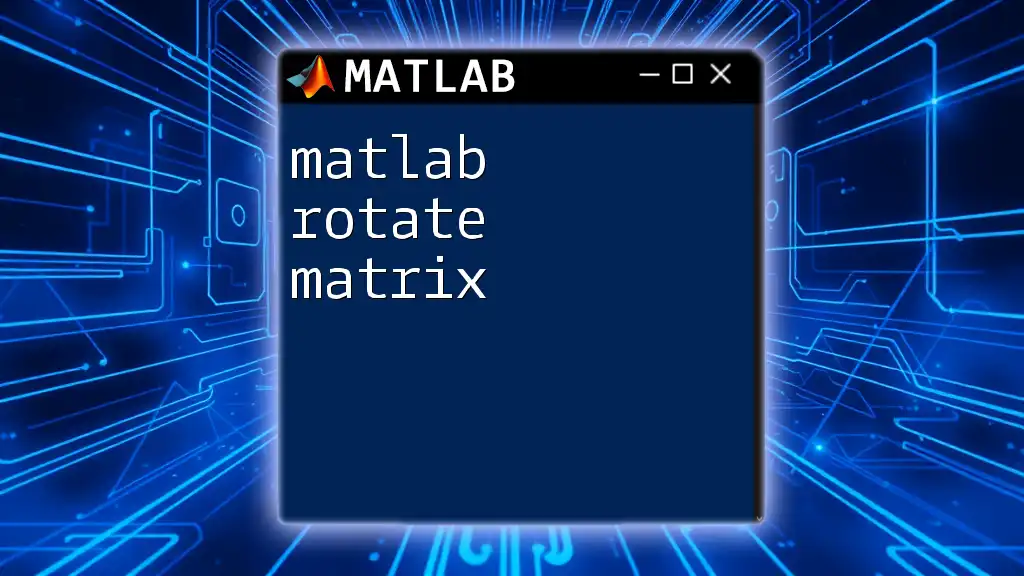
Constructing Matrices with Ranges and Patterns
Using the Colon Operator
MATLAB allows quick creation of vectors using the colon operator. You can generate a sequence of numbers with a specified increment. For example:
vectorFrom1To10 = 1:10; % Creates a vector with values from 1 to 10
You can also specify a different step size:
vectorWithStep = 1:2:10; % Creates a vector with values 1, 3, 5, 7, 9
Creating Matrices with `reshape`
The `reshape` function is useful if you want to change the dimensions of an existing matrix or vector. For instance, the following code reshapes a vector into a 3x4 matrix:
matrixReshaped = reshape(1:12, 3, 4); % Reshapes into 3 rows and 4 columns
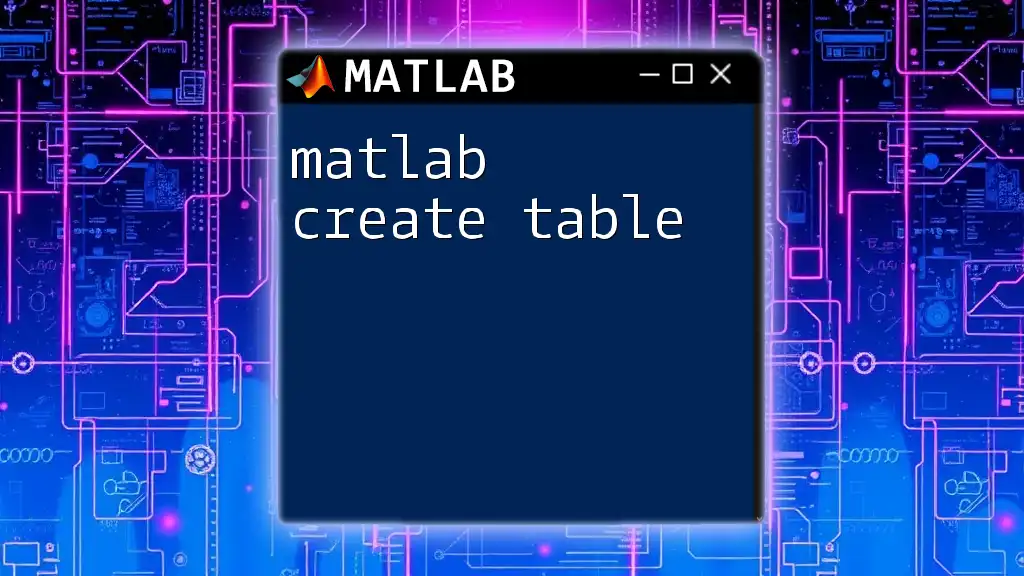
Concatenation of Matrices
Horizontal and Vertical Concatenation
Horizontal Concatenation
You can combine matrices horizontally by using a comma `,` to separate them within square brackets. For example:
A = [1 2; 3 4];
B = [5 6];
C = [A; B]; % Concatenates A and B vertically
This results in a new matrix with increased rows.
Vertical Concatenation
For vertical concatenation, you can use a semicolon within the brackets:
D = [7; 8];
E = [A, D]; % Concatenates A with D horizontally
This expands your matrix by adding new columns.
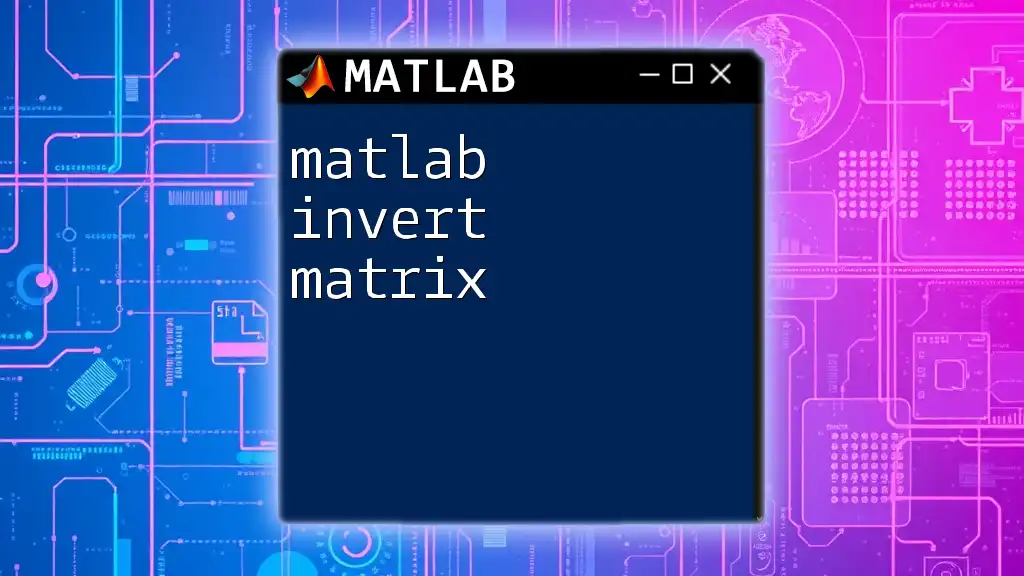
Practical Applications and Examples
Practical Example: Data Representation
Creating matrices to represent datasets is a frequent task in MATLAB. For example, if we want to create a matrix to reflect student scores in various subjects, you could do the following:
studentScores = [85, 90, 78; 92, 88, 84];
This matrix contains the scores of two students in three different subjects.
Solving a Linear Equation
Matrices are invaluable when solving linear equations. Consider the system:
2x + 3y = 8
x + 2y = 5
You can represent the coefficients and constants as follows:
A = [2 3; 1 2];
B = [8; 5];
X = A \ B; % Solving for X (the variables x and y)
This uses MATLAB's backslash operator to find the values of `x` and `y` that solve the equations.
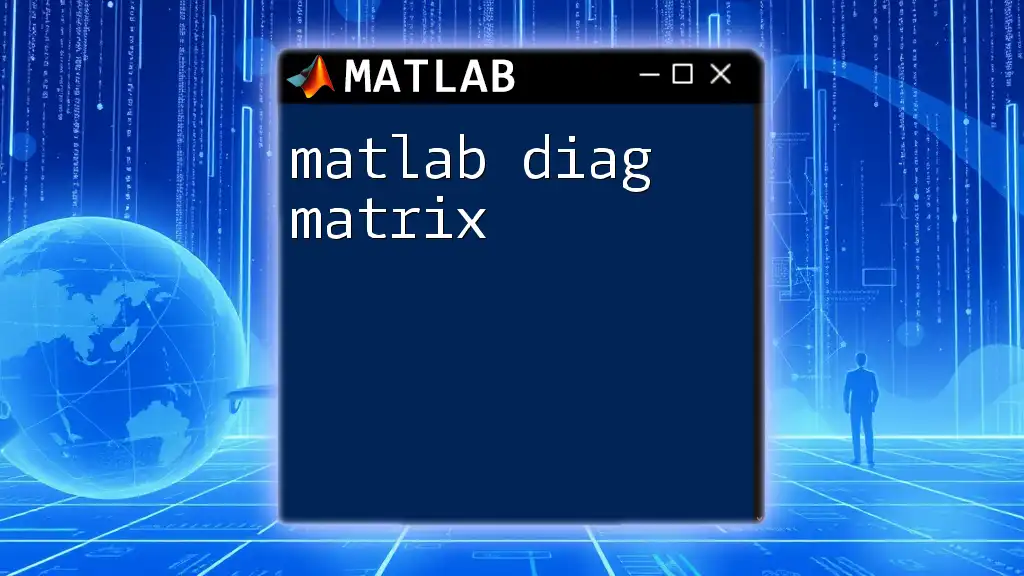
Tips and Best Practices
Matrix Naming Conventions
When creating matrices, it’s essential to use clear and descriptive names. This not only aids in code readability but also helps reduce errors. Names should reflect the purpose of the matrix, like `studentScores` or `coeffMatrix`.
Avoiding Common Pitfalls
Common mistakes when creating matrices include mismatched dimensions and incorrect syntax. Always double-check your matrices’ sizes and ensure you are using proper operators. For instance, trying to concatenate matrices of different dimensions will result in an error.
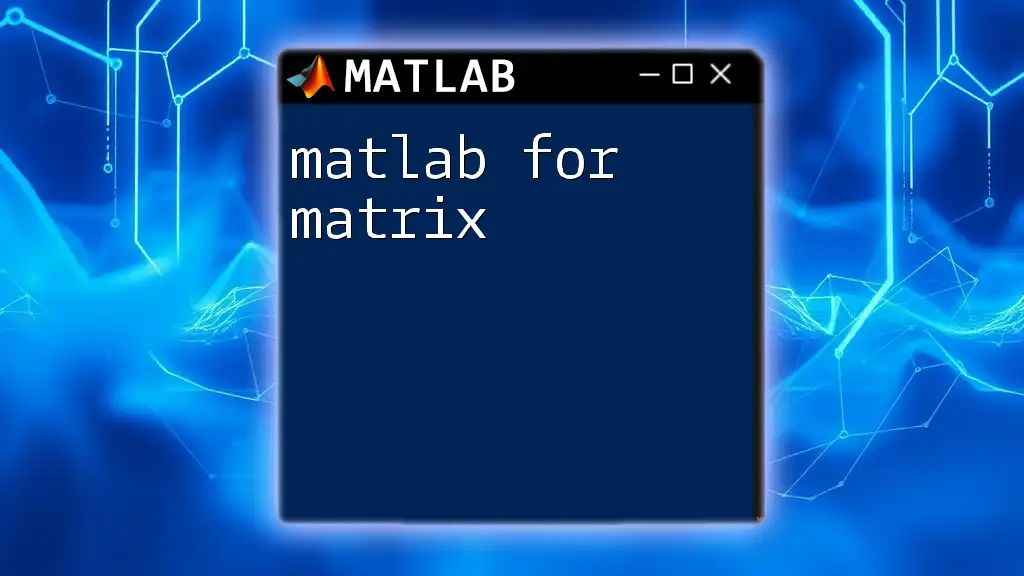
Conclusion
In this comprehensive guide on how to matlab create matrix, you have learned about the essential concepts of matrices, their creation, and their applications in MATLAB. From basic matrix creation to advanced functions like reshaping and concatenation, you've acquired the tools to manipulate matrices effectively.
As you continue your journey with MATLAB, don’t hesitate to experiment and apply these techniques in real-world scenarios. Whether you are analyzing data or solving complex equations, mastering matrix operations will enhance your proficiency in numerical computing.
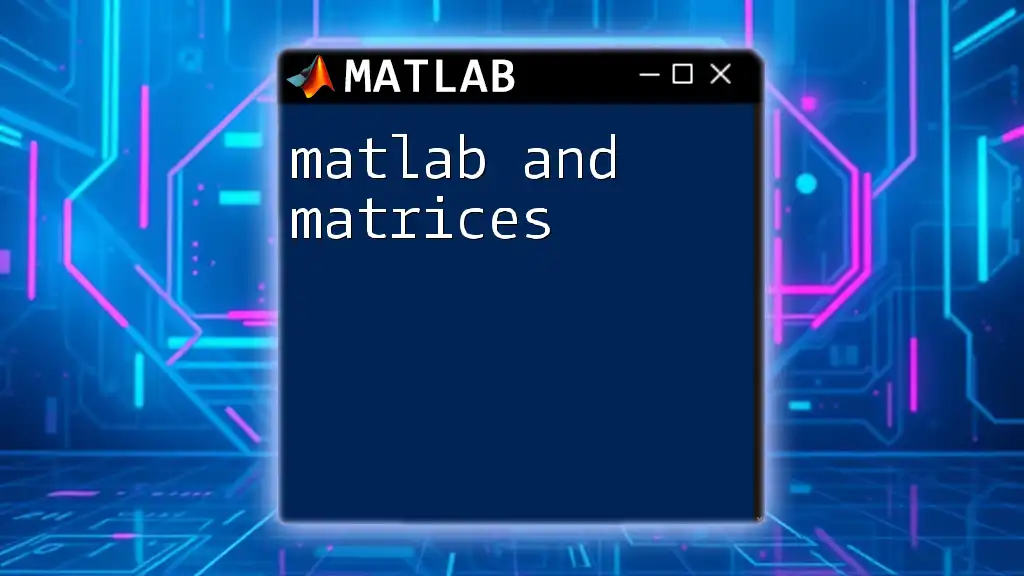
Additional Resources
For further education, consider exploring MATLAB's official documentation, online tutorials, and forums where you can engage with a community of MATLAB enthusiasts. With practice and experimentation, you'll be creating matrices like a pro in no time!
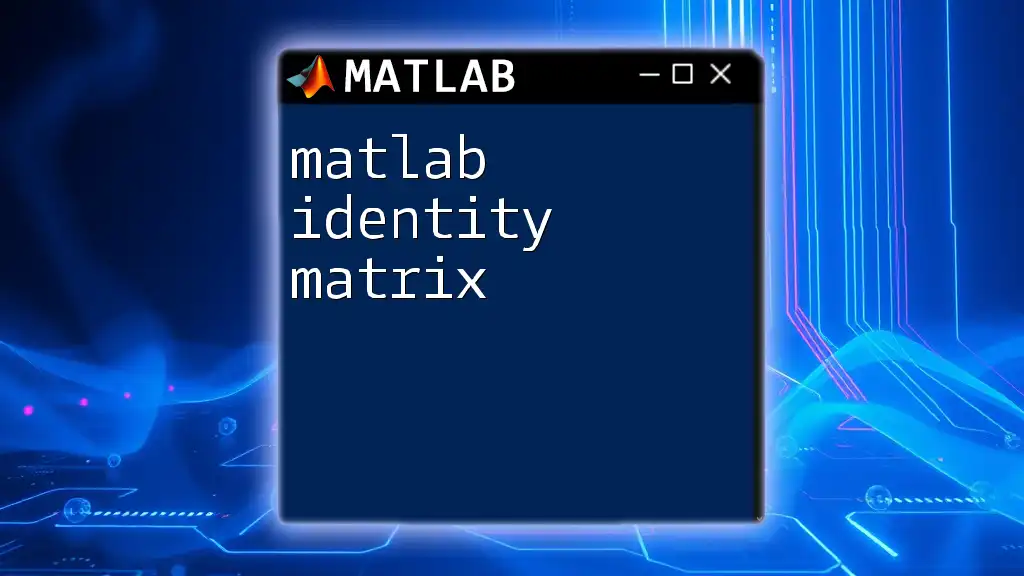
Call to Action
Take your skills to the next level by signing up for our services, designed to help you master MATLAB commands quickly and efficiently! Join our community of learners and unlock the full potential of MATLAB in your projects.