In MATLAB, you can convert a double to a string using the `num2str` function, which allows for quick and easy formatting of numerical values into string representations.
Here's a code snippet demonstrating this:
doubleValue = 42.7;
stringValue = num2str(doubleValue);
Understanding MATLAB Data Types
What is a Double?
In MATLAB, a double is the default numeric data type used to represent decimal numbers. It is a floating-point representation that can accommodate a wide range of values due to its precision and flexibility.
- Characteristics of Double:
- A double is typically 64 bits in size, allowing for significant precision—up to approximately 15 to 17 decimal digits.
- It can represent values ranging from approximately -1.7976931348623157e+308 to 1.7976931348623157e+308.
- Any numerical operation performed in MATLAB without a specific type declaration will result in a double.
What is a String?
A string in MATLAB is essentially a sequence of characters. Starting from R2016a, MATLAB introduced a new string data type that simplifies the manipulation of text compared to traditional character arrays.
- String vs Character Array:
- MATLAB strings are created using double quotes, e.g., `"Hello, World!"`, while character arrays are created using single quotes, e.g., `'Hello, World!'`.
- Strings support array operations, allowing for easy concatenation and manipulation, while character arrays require more manual intervention.
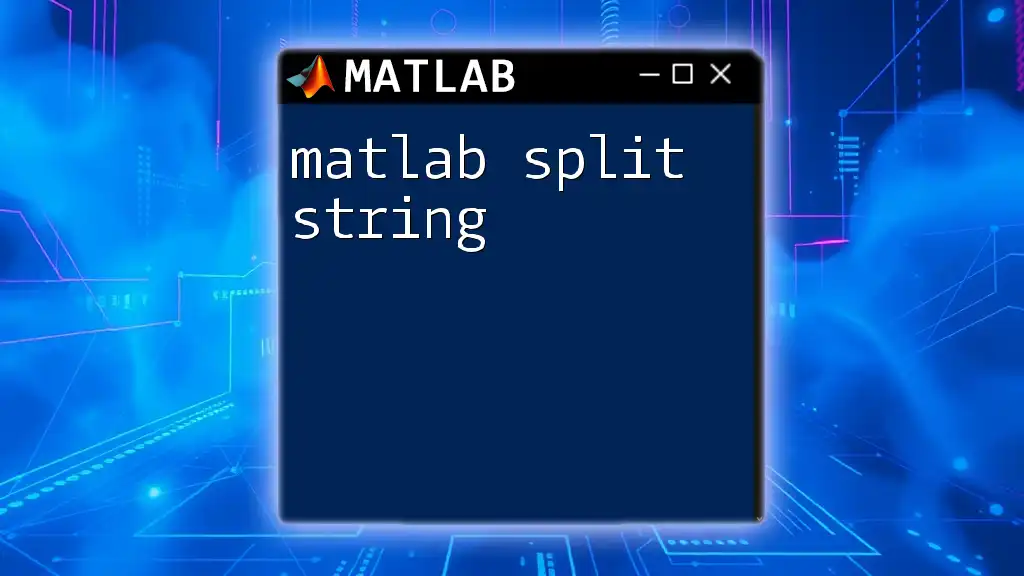
Why Convert Double to String?
Converting data types, particularly from double to string, is crucial in various applications.
Practical Applications
- Data Presentation: Strings are commonly used in user interfaces, reports, and plots to present numerical data in a comprehensible format. Converting doubles to strings enables formatted output that enhances readability.
- User Interaction: In interactive applications, messages displayed to the user often need strings. For instance, a prompt might need to display a numeric result alongside explanatory text.
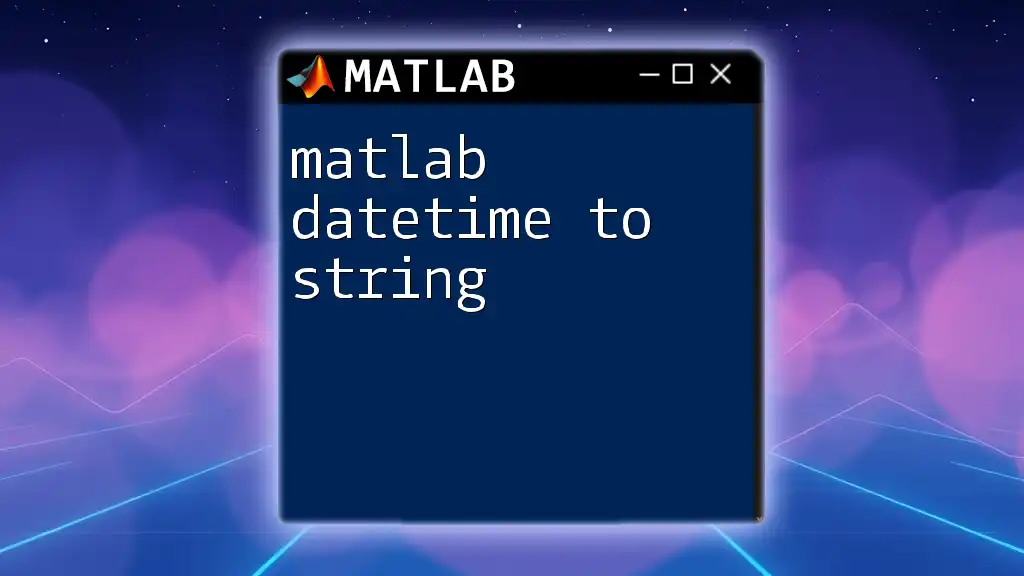
Methods to Convert Double to String
Using the `num2str` Function
Explanation
The `num2str` function is designed specifically for converting numeric values to their string representation. This function is especially useful when you want to display numbers in a specific format.
Syntax and Parameters
The basic syntax is as follows:
str = num2str(X)
Here, `X` is the numeric value or matrix of values you wish to convert. Additionally, `num2str` accepts a format specifier to control how the numeric value is converted to a string, such as specifying the number of decimal places.
Examples
-
Basic Example:
x = 123.456; str = num2str(x); disp(str); % Output: '123.456'
Here, `num2str` converts the double `x` to a string without any formatting, producing a straightforward representation.
-
Formatted Example:
x = 123.456; str = num2str(x, '%.2f'); disp(str); % Output: '123.46'
In this example, the format specifier `'%.2f'` rounds the number to two decimal places, returning a neatly formatted string.
Using the `sprintf` Function
Explanation
The `sprintf` function provides more advanced formatting capabilities than `num2str`. This is particularly useful when generating complex strings involving multiple variables.
Syntax and Parameters
The basic syntax is:
str = sprintf(formatSpec, A)
`formatSpec` describes how to format the output string, while `A` can be a single number or an array of numbers.
Examples
-
Basic Example:
x = 123.456; str = sprintf('Value: %.2f', x); disp(str); % Output: 'Value: 123.46'
This approach allows embedding the numeric value within a string, perfect for generating user-friendly outputs.
-
Multiple Values Example:
x = [12.34, 56.78]; str = sprintf('First: %.2f, Second: %.2f', x); disp(str); % Output: 'First: 12.34, Second: 56.78'
By using `sprintf`, we can dynamically include values into a formatted string representation.
Using the `string` Function
Explanation
The `string` function converts numerical arrays directly into string arrays. This approach is straightforward and works with both individual numbers and arrays.
Syntax and Parameters
The syntax for using the `string` function is:
str = string(A)
Where `A` can be any numeric value or array.
Examples
-
Basic Example:
x = 123.456; str = string(x); disp(str); % Output: "123.456"
This case showcases a simple conversion where numerical values become string representations.
-
Array Example:
x = [12.34, 56.78]; str = string(x); disp(str); % Output: "12.34" "56.78"
This capability highlights how the `string` function can accommodate arrays gracefully.
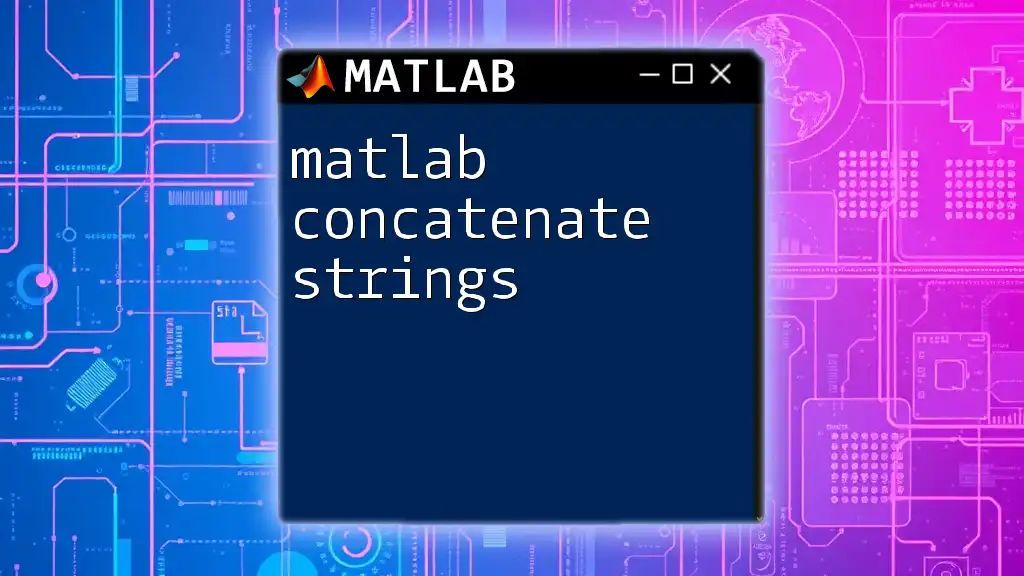
Common Pitfalls and Their Solutions
Handling Precision Issues
When converting doubles to strings, one common pitfall is the loss of precision. Sometimes, a double value might not appear as expected when converted, especially with many decimal places.
Solutions:
- Be cautious about the number of decimal places specified in the conversion functions, especially when high precision is required.
- Take advantage of format specifiers to maintain the desired level of accuracy.
Edge Cases
Special cases like negative values, zero, and special floating-point values (like Inf or NaN) can produce unexpected results when converting.
- Negative Values and Zero: Ensure that conversion functions handle negative numbers properly.
- Inf and NaN Values: Functions like `num2str` and `sprintf` manage these types by default, converting them to recognizable strings such as `'Inf'` or `'NaN'`.
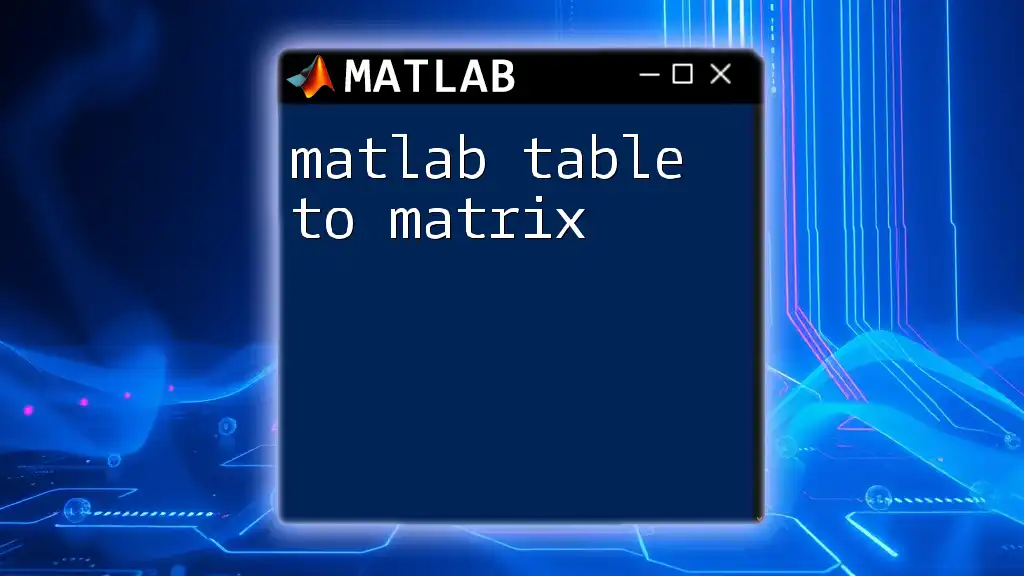
Best Practices for Double to String Conversion
When to Use Each Method
- Use `num2str`: For straightforward conversions without complex formatting.
- Use `sprintf`: When combining multiple variables into a single formatted string or requiring precise control over the output format.
- Use `string`: For ease of use with newer MATLAB versions when working with arrays, as it simplifies the conversion process significantly.
Performance Considerations
In performance-critical applications, consider the size of data. While `num2str`, `sprintf`, and the `string` function are generally efficient, using them on larger datasets can impact performance. If working on sizable matrices, try batch processing wherever possible to minimize overhead.
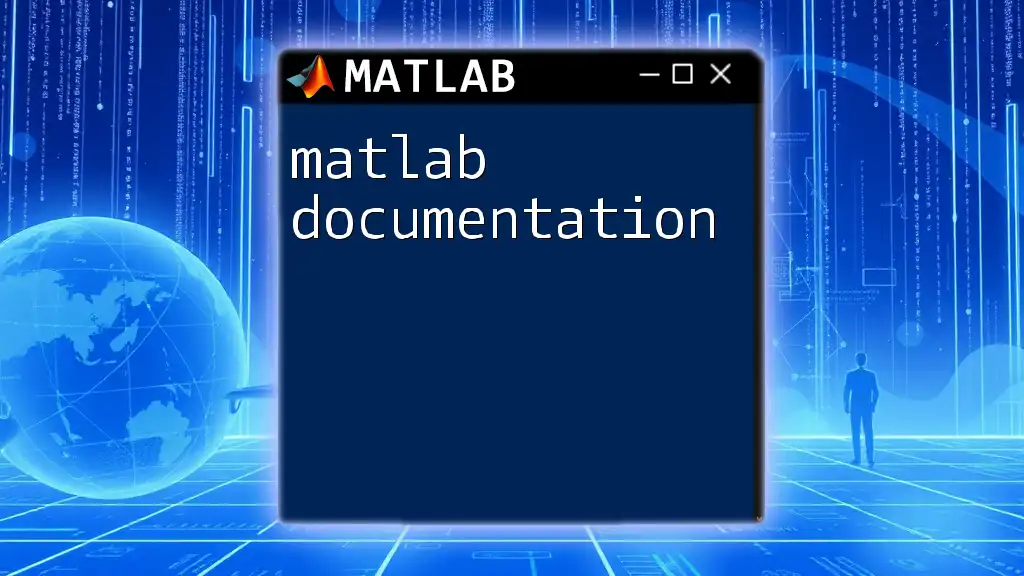
Conclusion
In conclusion, converting MATLAB doubles to strings is an essential skill for displaying, reporting, and interacting with numeric data. Understanding the differences between functions like `num2str`, `sprintf`, and `string`, as well as knowing when to apply each, empowers you to choose the right method for your specific needs. Regular practice with these conversion techniques will enhance your MATLAB proficiency, enabling you to create more user-friendly applications and analyses.
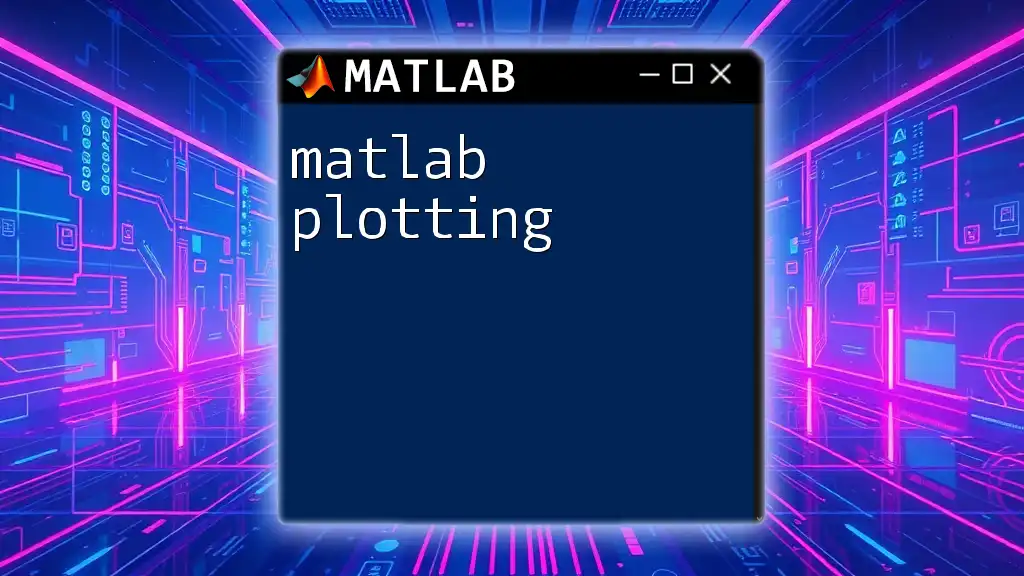
Additional Resources
For further exploration, consider diving into the official MATLAB documentation and a variety of online tutorials and courses that focus on mastering MATLAB functionalities. This knowledge will further enrich your ability to manipulate and present data effectively in your projects.