The `split` function in MATLAB allows you to divide a string into substrings based on a specified delimiter. Here's a simple code snippet demonstrating its use:
str = 'Hello,World,Matlab';
result = split(str, ',');
Understanding Strings in MATLAB
What is a String?
In programming, a string is a sequence of characters used to represent text. In MATLAB, there are two primary types of strings: character arrays and string arrays. Character arrays are enclosed in single quotes (e.g., `'Hello, World!'`), while string arrays are enclosed in double quotes (e.g., `"Hello, World!"`). This distinction is important when manipulating strings and determining which functions to utilize.
Creating Strings
Character Arrays:
You can create character arrays by using single quotes. For example:
charArray = 'Hello';
String Arrays:
For string arrays, use double quotes:
strArray = "Hello";
Both types of strings can hold the same information; however, each comes with specific functions and syntax in MATLAB.
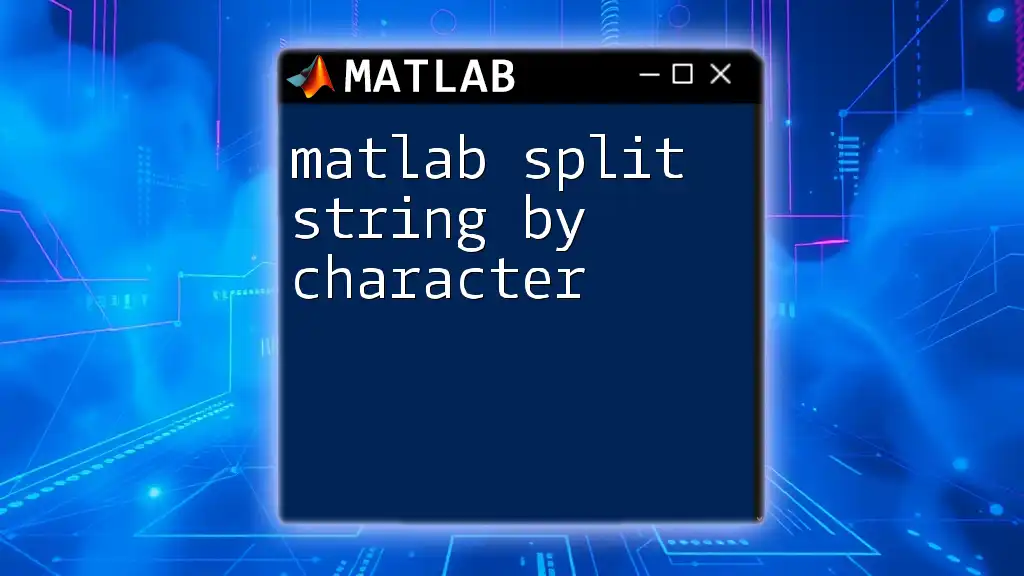
The Importance of String Splitting
Use Cases for Splitting Strings
Splitting strings in MATLAB is crucial for multiple reasons, such as:
- Data processing and cleaning: You may encounter strings that contain combined data that need to be separated for analysis.
- Parsing user input: When receiving input, it's often necessary to break it down into parts for further processing.
- Extracting relevant information from formatted text: For instance, if you have a readout from a sensor, you may need to extract specific measurements from a string.
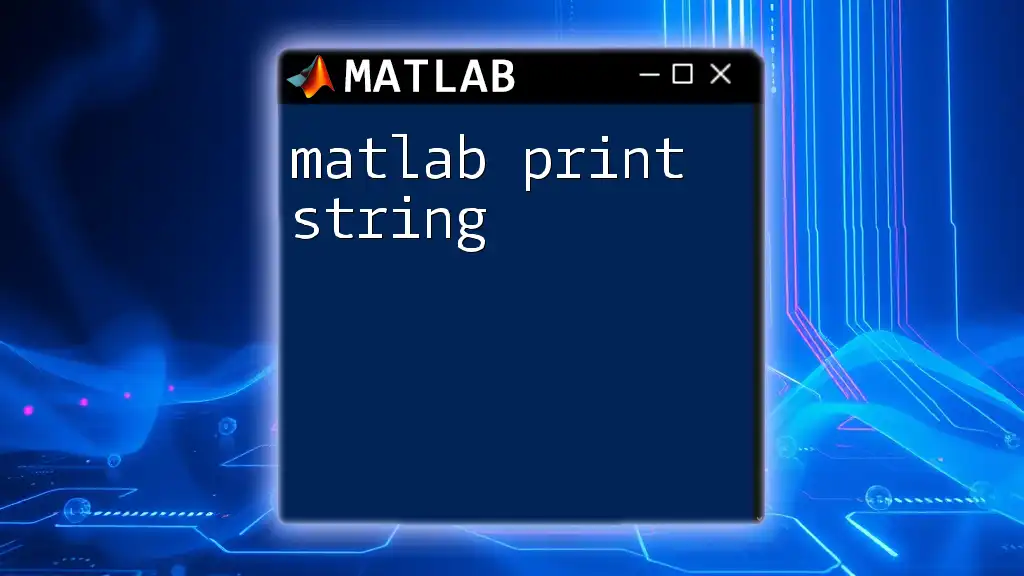
MATLAB Commands for Splitting Strings
The `strsplit` Function
The `strsplit` function is one of the principal methods in MATLAB for splitting strings. Its basic syntax is:
result = strsplit(string, delimiter)
Here, `string` is the input string you want to split, and `delimiter` is the character or string that indicates where to split the input string.
Example: Basic Usage of `strsplit`
To illustrate, consider the following example:
text = 'Learn MATLAB with ease';
result = strsplit(text);
disp(result);
In this case, MATLAB will split the string at the spaces, resulting in:
'Learn' 'MATLAB' 'with' 'ease'
This simple split provides each word as a separate element in a cell array.
Custom Delimiters with `strsplit`
You also have the ability to specify custom delimiters when using `strsplit`, allowing for more nuanced string manipulation.
Example: Using Custom Delimiters
Suppose you have a string that uses different characters to separate entries:
data = 'apple,banana;orange';
result = strsplit(data, {',', ';'});
disp(result);
In this example, the string is split at both commas and semicolons, resulting in the output:
'apple' 'banana' 'orange'
This flexibility makes `strsplit` a powerful tool when dealing with strings with varied delimiters.
Splitting Strings with Regular Expressions
Introduction to `regexp`
Regular expressions, or regex, offer a powerful way to specify complex search patterns. In situations where string delimiters are more intricate, `regexp` can be particularly useful.
Example: Using `regexp` to Split Strings
Let's say you want to split a string that combines both letters and numbers:
text = 'apple123banana456orange';
result = regexp(text, '\d+', 'split');
disp(result);
In this code, the regex pattern `\d+` matches sequences of digits, and `split` indicates that you want to split at those locations. The output for this string will be:
'apple' 'banana' 'orange'
This demonstrates how regex can be leveraged to achieve advanced string manipulation.
Other Useful Functions for String Manipulation
`split` Function (MATLAB R2016b and Later)
As of MATLAB R2016b, the `split` function was introduced as a more modern alternative to `strsplit`. Its syntax is similar:
result = split(string, delimiter)
Example of `split`
Here's how you can use `split`:
text = "apple,banana,orange";
result = split(text, ",");
disp(result);
Comparison: `strsplit` vs. `split`
Both functions have their merits:
- Pros of `strsplit`: More flexibility with delimiters and supports cell arrays naturally.
- Pros of `split`: More intuitive syntax for modern string arrays and generally easier to use for straightforward cases.
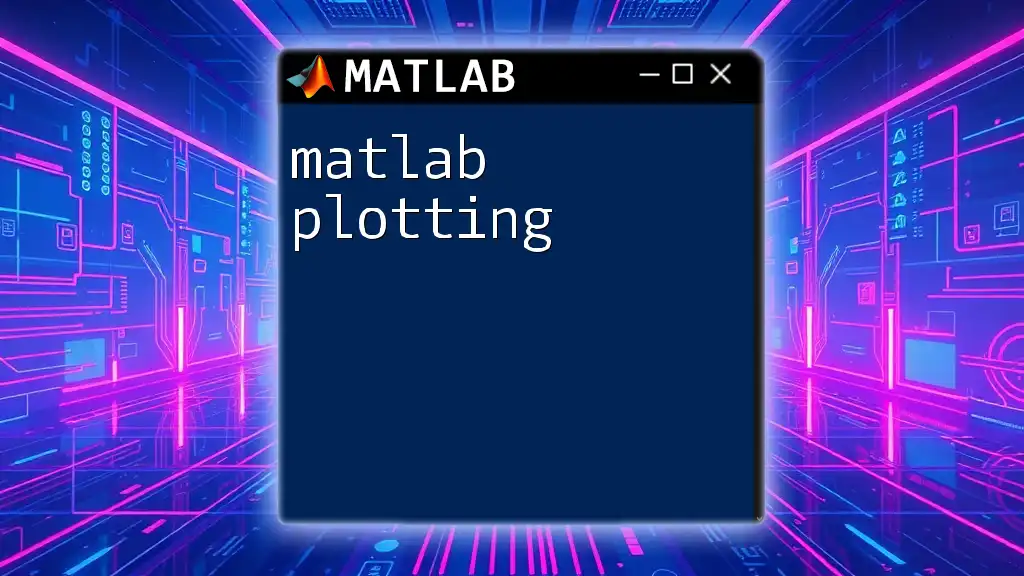
Practical Applications of String Splitting
Case Study: Processing CSV Data
Splitting strings is particularly relevant when managing CSV (Comma-Separated Values) data, a common format for storing tabular data. Inside MATLAB, you could represent a single row of a CSV file as a string and use `strsplit` or `split` to analyze its contents efficiently. For instance:
csvRow = 'John,Doe,25,Male';
fields = strsplit(csvRow, ',');
disp(fields);
This results in a cell array with each field as a separate element.
Example: Extracting Information from a Log File
Suppose you have log entries that contain timestamps and messages. One could utilize `strsplit` to isolate information:
logEntry = '2023-10-01 10:00:00 Error: Disk space low';
parts = strsplit(logEntry, ' ');
disp(parts);
This would help break down the log entry into manageable parts, allowing for further analysis.
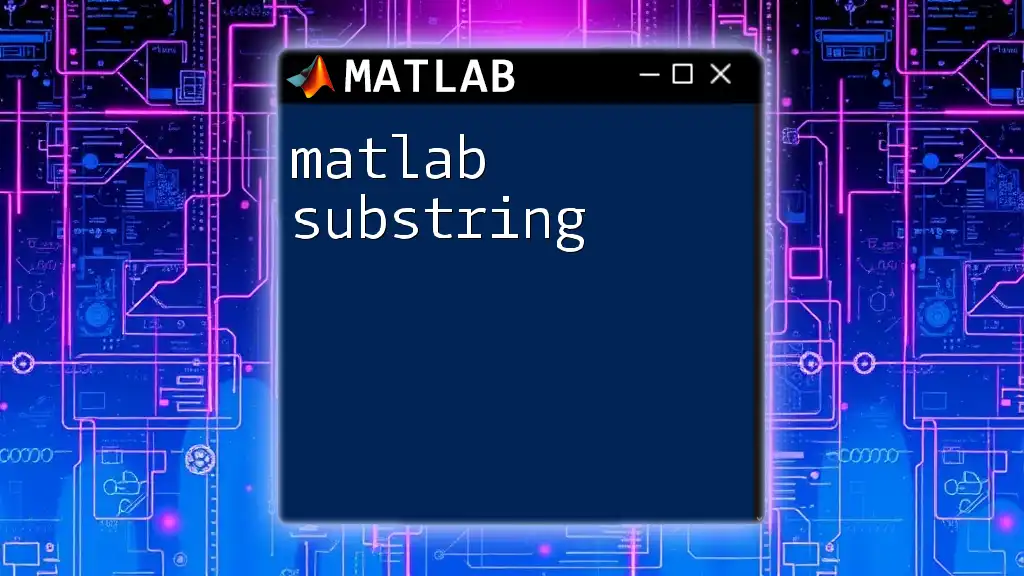
Common Errors and Troubleshooting Tips
Common Mistakes When Splitting Strings
When working with string splitting, programmers often encounter typical pitfalls, such as:
- Forgetting to specify the correct delimiter, leading to unexpected results.
- Mismatches between character arrays and string arrays, causing compatibility issues with functions that have specific type requirements.
Debugging Tips
To mitigate these issues, consider employing commands like `disp` and `fprintf` to gain insight into variables and outputs. Moreover, always check the dimensions of your output to ensure that the split operation performed as intended.
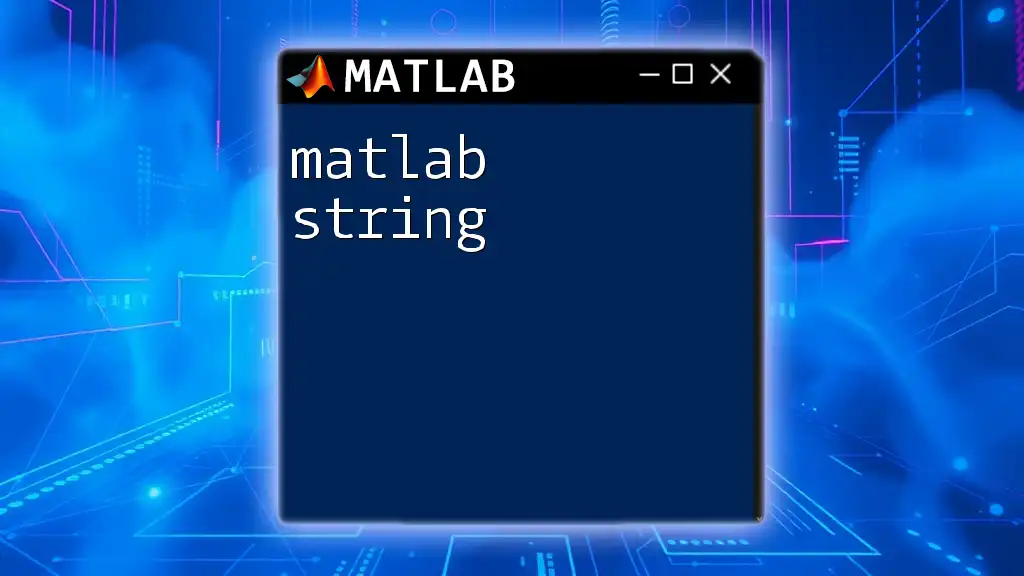
Conclusion
In summary, understanding how to utilize the `strsplit`, `split`, and `regexp` functions effectively can enhance your proficiency in manipulating strings in MATLAB. Practicing these techniques can lead to more efficient coding and data processing outcomes. Explore additional resources and documentation to deepen your grasp of string manipulation as you continue your MATLAB journey.