In MATLAB, you can rotate a matrix 90 degrees counterclockwise using the `rot90` function, as illustrated in the following code snippet:
A = [1 2 3; 4 5 6; 7 8 9]; % Original matrix
B = rot90(A); % Rotated matrix
disp(B);
Understanding Matrices in MATLAB
What is a Matrix?
A matrix is a rectangular array of numbers arranged in rows and columns. In MATLAB, matrices are fundamental data structures used for a wide range of applications, from mathematical computations to data analysis. They can be one-dimensional (vectors) or multi-dimensional (2D matrices, 3D matrices, etc.).
Types of Matrices
MATLAB supports various types of matrices, such as:
- Square Matrices: Matrices that have the same number of rows and columns (e.g., a 3x3 matrix).
- Rectangular Matrices: Matrices with different numbers of rows and columns (e.g., a 2x3 matrix).
Creating Matrices in MATLAB
To work with matrices effectively, you need to know how to create them. You can create matrices using square brackets:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
You can also use built-in functions to create matrices:
- `zeros()`: Creates a matrix filled with zeros.
- `ones()`: Creates a matrix filled with ones.
- `eye()`: Generates an identity matrix.
For example, to create a 3x3 identity matrix:
I = eye(3);
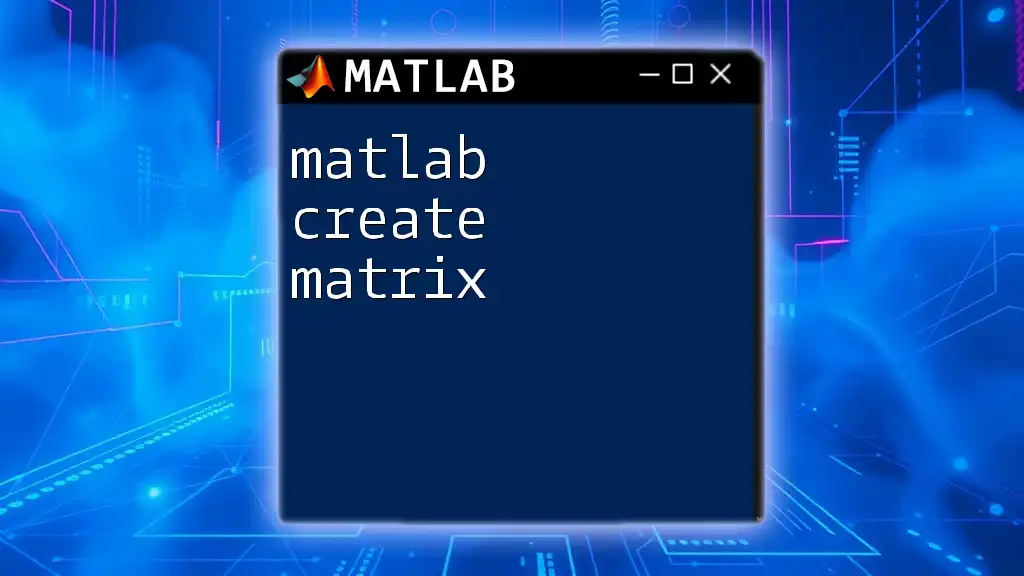
Rotating a Matrix
Importance of Matrix Rotation
Matrix rotation is essential in many fields, including computer graphics, robotics, and engineering simulations. For instance, in image processing, rotating an image allows for various transformations and adjustments, such as aligning images, creating panoramic views, or simulating camera movements.
Basic Concepts of Matrix Rotation
When discussing rotation in a 2D plane, it is crucial to differentiate between degrees and radians. A complete rotation of 360 degrees is equivalent to 2π radians. This understanding is important when transforming matrices in MATLAB, as many functions may require angles in either form.
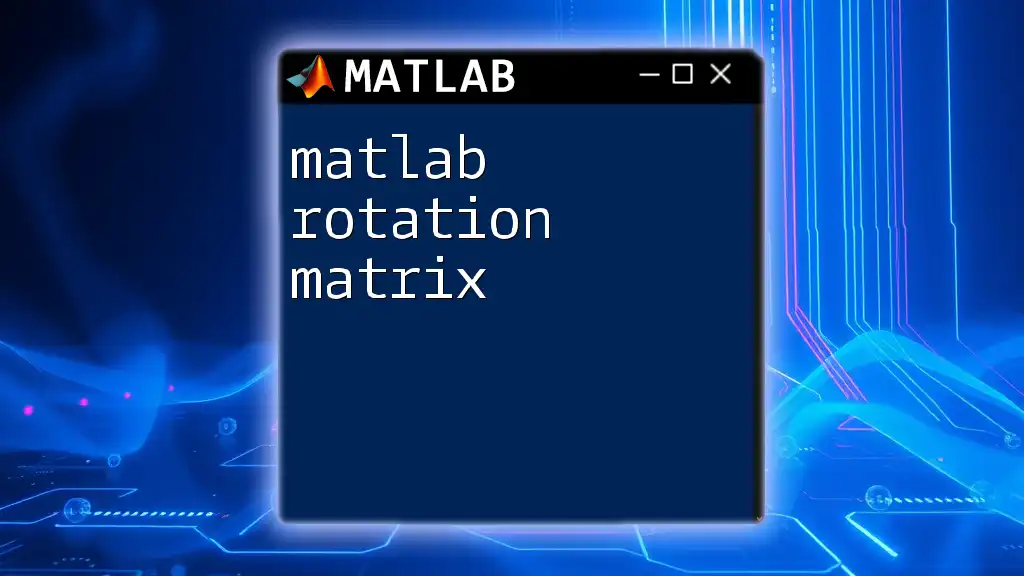
How to Rotate a Matrix in MATLAB
Using the `rot90` Function
One of the simplest methods to rotate a matrix in MATLAB is by using the built-in function `rot90`. This function rotates the matrix 90 degrees counter-clockwise by default.
Syntax and Usages of `rot90`
The basic syntax of `rot90` is as follows:
B = rot90(A);
You can also specify the number of times you want to rotate it. For instance:
B = rot90(A, 2); % Rotates A by 180 degrees
B = rot90(A, -1); % Rotates A by 90 degrees clockwise
Example of Using `rot90`
Consider the following matrix:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
B = rot90(A);
The resulting matrix B will be:
B = [3, 6, 9;
2, 5, 8;
1, 4, 7];
Explanation: The matrix has been rotated 90 degrees counter-clockwise, demonstrating how the `rot90` function alters the arrangement of elements.
Using Custom Rotation Functions
Rotating by Arbitrary Angles
For cases where you need rotation by an arbitrary angle (not just multiples of 90), you'll need to create a custom function. Rotation in such cases usually involves the application of a rotation matrix, derived from trigonometric functions.
Custom Function to Rotate Matrices
Here’s how you can write a custom function in MATLAB to rotate a matrix by a specified angle:
function B = rotateMatrix(A, angle)
angle = angle * (pi / 180); % Convert degrees to radians
% Create rotation matrix
R = [cos(angle) -sin(angle); sin(angle) cos(angle)];
% Apply rotation and rounding for integer matrices (if necessary)
B = round(R * A);
end
Explanation of the Code: The function takes a matrix A and an angle in degrees, converts the angle to radians, constructs a rotation matrix R, and applies it to A. The resulting matrix B is rounded to maintain integer values if the original matrix consists of integers.
Image Rotation in MATLAB
In the context of image processing, MATLAB provides the `imrotate` function, specifically designed for rotating images. This function handles interpolation and can be crucial when you wish to preserve image quality.
Syntax and Example of `imrotate`
To rotate an image, you can use:
J = imrotate(I, 45); % Rotate image by 45 degrees
This will rotate the image I by 45 degrees, producing a new image J. The function manages pixel interpolation, which is essential in image rotation to avoid distortion.
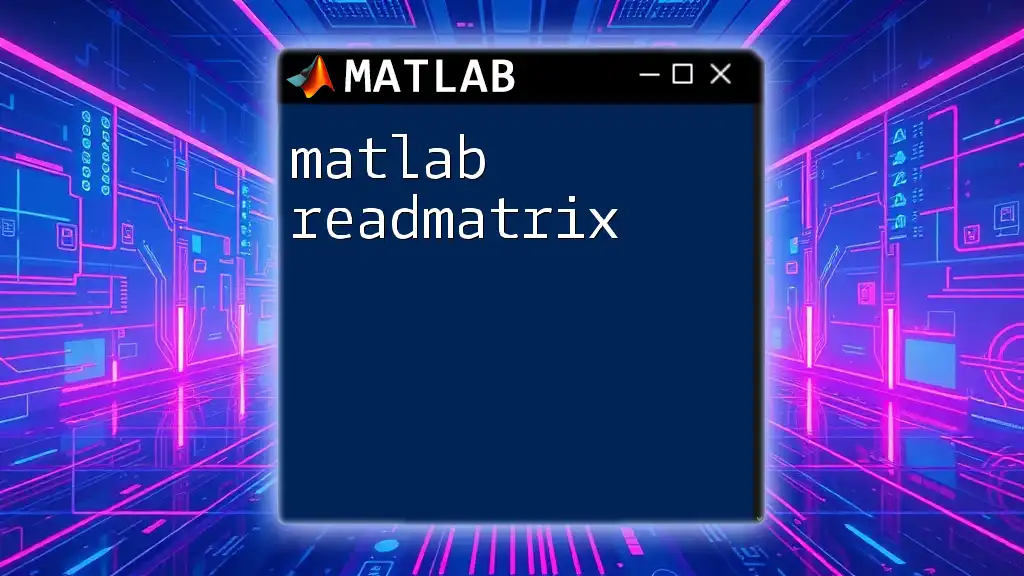
Practical Examples
Rotating Square and Non-Square Matrices
In practice, it’s crucial to understand how rotating matrices of different shapes behaves. Here's a quick comparison:
Square Matrix Example:
A = [1, 2; 3, 4];
B = rot90(A);
Result:
B = [2, 4;
1, 3];
Non-Square Matrix Example:
C = [1, 2, 3; 4, 5, 6];
D = rot90(C);
Result:
D = [3, 6;
2, 5;
1, 4];
Applications in Data Visualization
Rotating a matrix is not only applicable in image processing but also enhances data visualization. For example, in plotting data, you might want to rotate your labels or markers to improve readability or achieve a particular aesthetic effect.
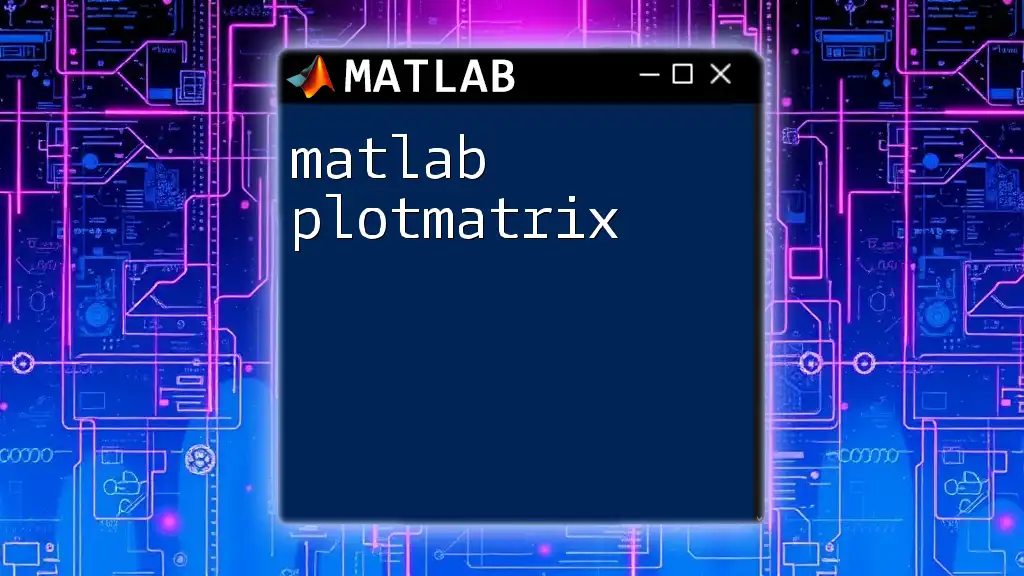
Common Errors and Troubleshooting
Misunderstandings and Pitfalls
When rotating matrices, there are common errors to be mindful of:
- Dimension Mismatch: Ensure the angle specified aligns with appropriate matrix dimensions.
- Angle Conversion Errors: Ensure angles are in the correct unit (degrees vs. radians) to avoid unexpected outcomes.
Tips for Troubleshooting
- Debugging: If you experience unexpected results, use `disp()` or `fprintf()` to print intermediate matrices and check transformations.
- Visual Inspection: Always visualize results, especially with images for clarity on the outcomes.
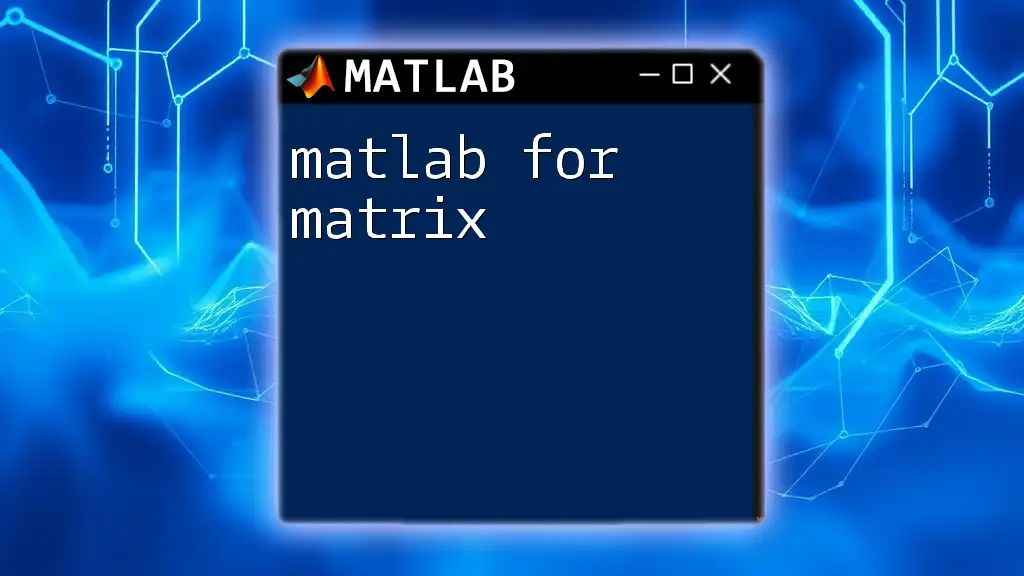
Conclusion
Mastering the ability to matlab rotate matrix effectively empowers you to manipulate and visualize data with precision in various applications. Practice the examples provided, experiment with your matrices, and create custom solutions to fully utilize MATLAB’s powerful features in matrix manipulation.