In MATLAB, the `extractSubstring` function allows you to retrieve a portion of a string based on specified starting and ending indices.
str = 'Hello, World!';
substring = extractSubstring(str, 1, 5); % Returns 'Hello'
Overview of Substrings in MATLAB
Understanding how to manipulate strings is crucial in programming. In MATLAB, strings are ubiquitous, and operations involving substrings are particularly common. A substring is simply a sequence of characters extracted from another string. These are valuable in various applications, from data parsing to text analysis.
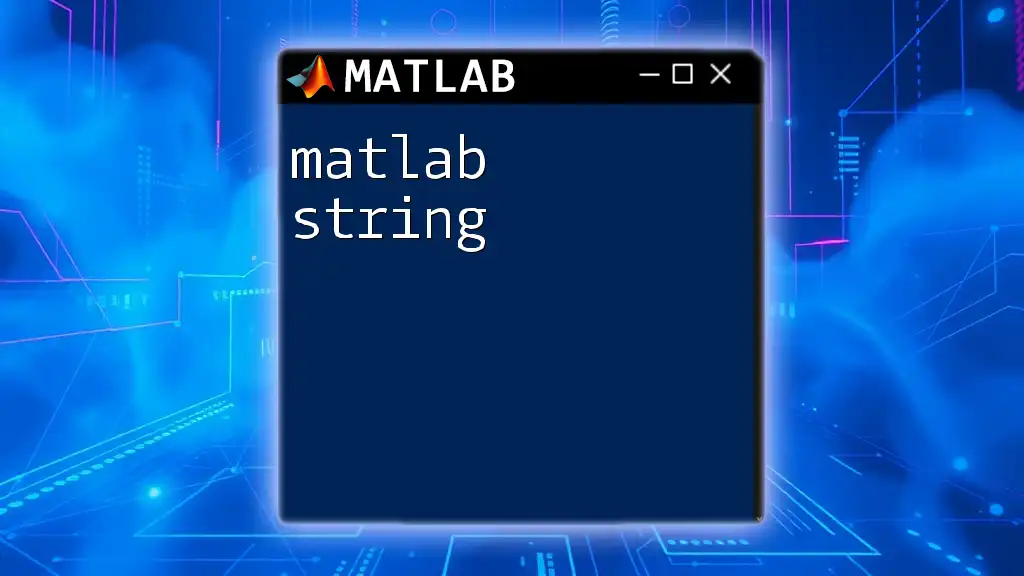
Basic Substring Concepts in MATLAB
Types of Strings in MATLAB
In MATLAB, there are two main types of strings:
- Character Arrays: Traditional string representation, surrounded by single quotes (`'`). For example:
charArray = 'MATLAB is great!';
- String Arrays: Introduced in R2016b, these are more versatile and are denoted with double quotes (`"`). For instance:
strArray = "Learn MATLAB programming.";
It is essential to understand the differences between these types because their methods and functionalities can vary.
Accessing Characters in a String
In MATLAB, strings are indexed, allowing access to individual characters. Indexing begins at 1. For instance, consider how to access specific characters from a string:
str = 'MATLAB';
firstChar = str(1); % This will return 'M'
This capability allows for various operations, including extracting substrings based on character positioning.
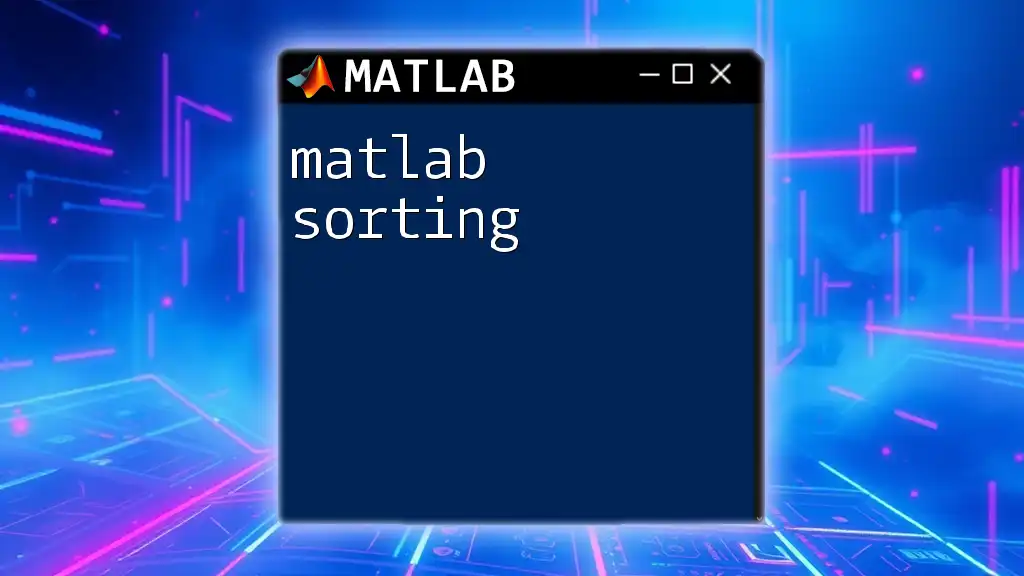
Using the `extract` Function
Introduction to `extract`
The `extract` function is a powerful tool used to retrieve substrings from a given string. The syntax is straightforward, and this function allows you to pull specific patterns directly from a string.
Examples of Using `extract`
For example, if you want to extract the word "MATLAB" from the phrase "Learn MATLAB programming efficiently!", you can do so using:
str = "Learn MATLAB programming efficiently!";
substring = extract(str, "MATLAB"); % This will return "MATLAB"
This approach can prove useful in various applications, such as isolating keywords in a larger body of text.
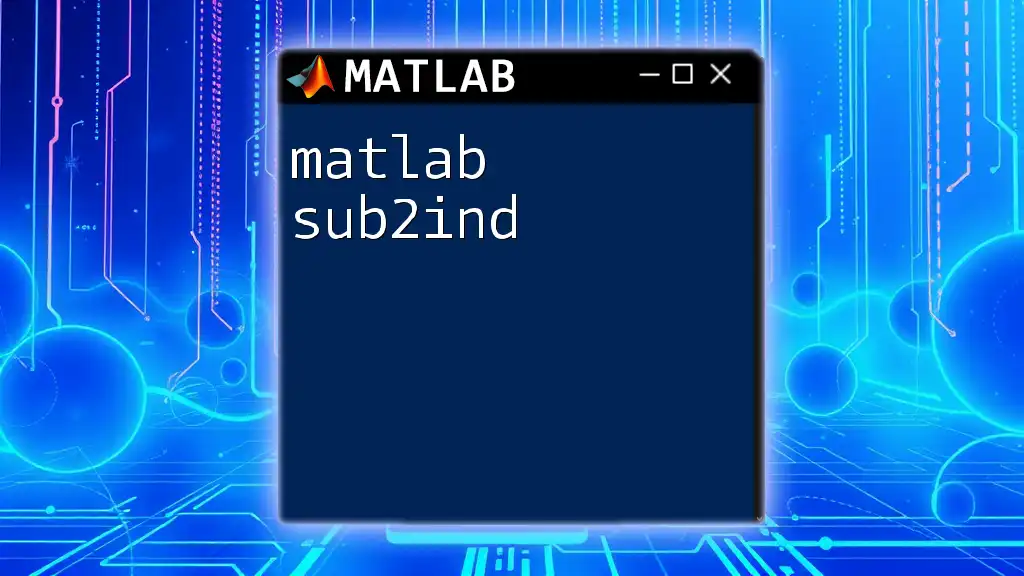
The `strfind` Function
Understanding `strfind`
The `strfind` function is essential for locating substrings within a string. It returns the starting index of the first occurrence of the substring.
Examples of Using `strfind`
To find the starting position of "MATLAB" in the string "Learn MATLAB programming", you can use:
str = 'Learn MATLAB programming';
index = strfind(str, 'MATLAB'); % This returns index 7
You can also locate substrings to perform further operations based on their position, allowing for powerful string manipulation.
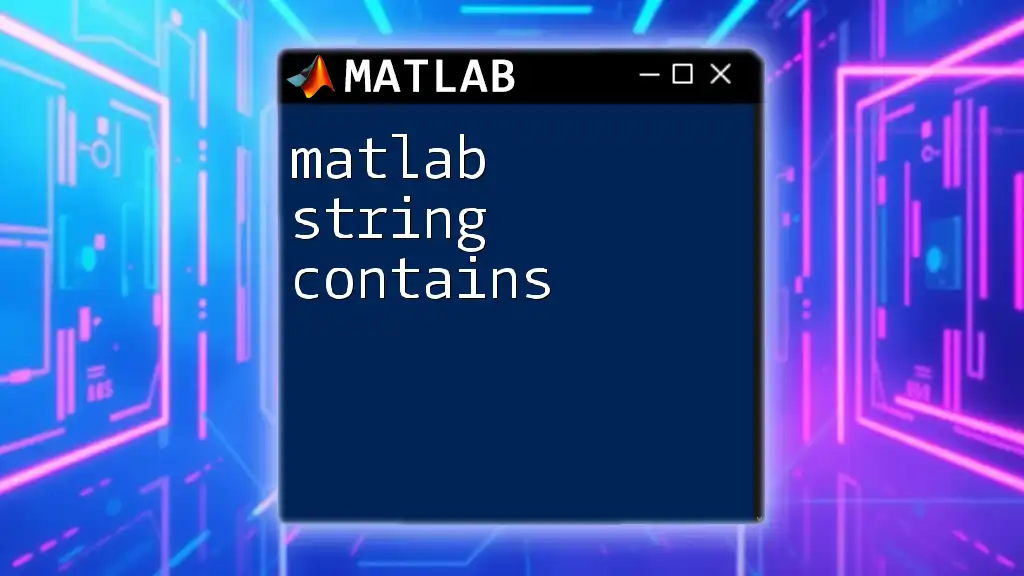
The `substr` Function
Overview of `substr` Functionality
While MATLAB does not possess a built-in `substr` function, it allows you to mimic this behavior through slicing. This method allows extraction of portions of a string based on specified index values.
Implementing a Custom `substr` Function
You can create a simple custom function to handle substring extraction:
function subStr = substr(str, startIdx, endIdx)
subStr = str(startIdx:endIdx); % Mimics substring behavior
end
This function enables you to specify any start and end index, thereby extracting the desired substring.
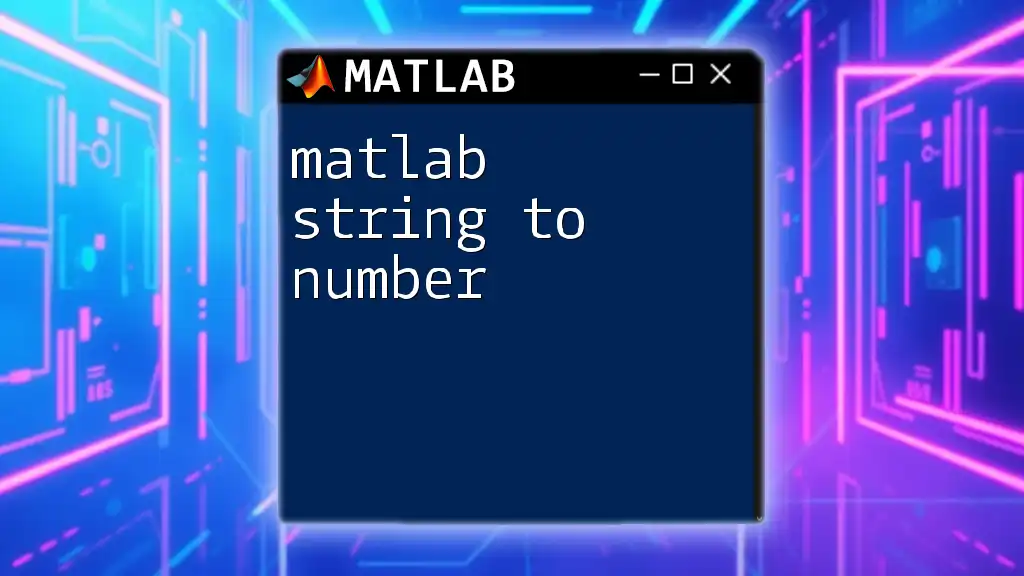
Using the `extractBetween` Function
What is `extractBetween`?
The `extractBetween` function enables you to extract substrings that lie between two specified delimiters. This method is especially useful when parsing structured strings.
Code Example for `extractBetween`
For example, if you want to extract a substring between the words "Learn" and "powerful" in the string "Learn MATLAB, a powerful programming tool!", you can use:
str = "Learn MATLAB, a powerful programming tool!";
result = extractBetween(str, "Learn ", ","); % This extracts "MATLAB"
This approach simplifies working with complex strings containing multiple segments or keywords.
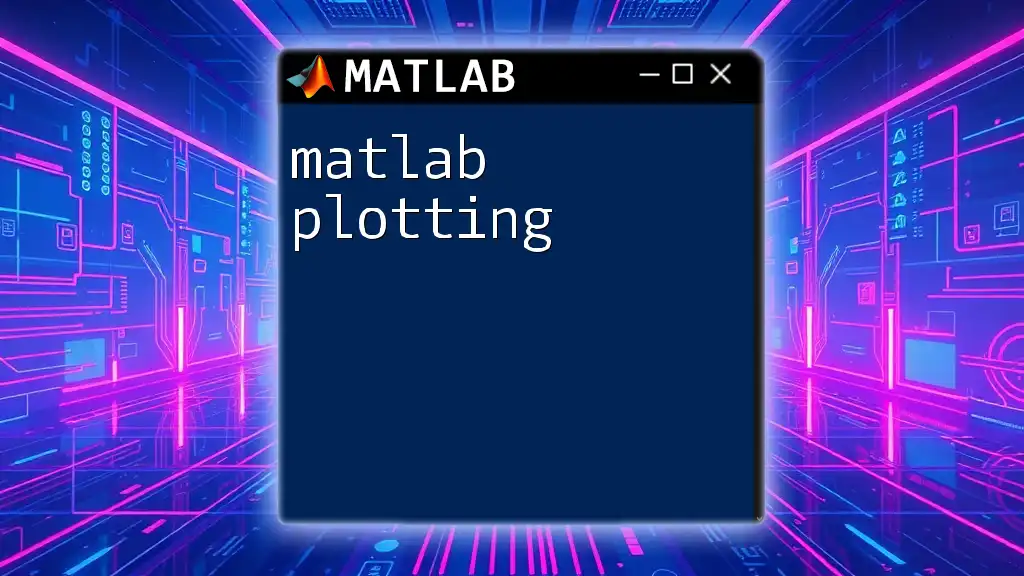
Practical Applications of Substring Functions
Real-World Examples
The ability to manipulate substrings has many real-world applications. For instance, when processing text data from files, you might need to extract specific fields or keywords repeatedly. Additionally, in text analysis and natural language processing, substring extraction can help isolate terms, phrases, or even patterns relevant to your study.
Best Practices for Substring Operations
To ensure efficiency when working with substrings, consider the following best practices:
- Optimize Performance: Be mindful of running substring functions within loops. Optimize your code by minimizing repetitive operations.
- Combine Operations: Often, you can chain substring functions together to achieve complex operations more succinctly.
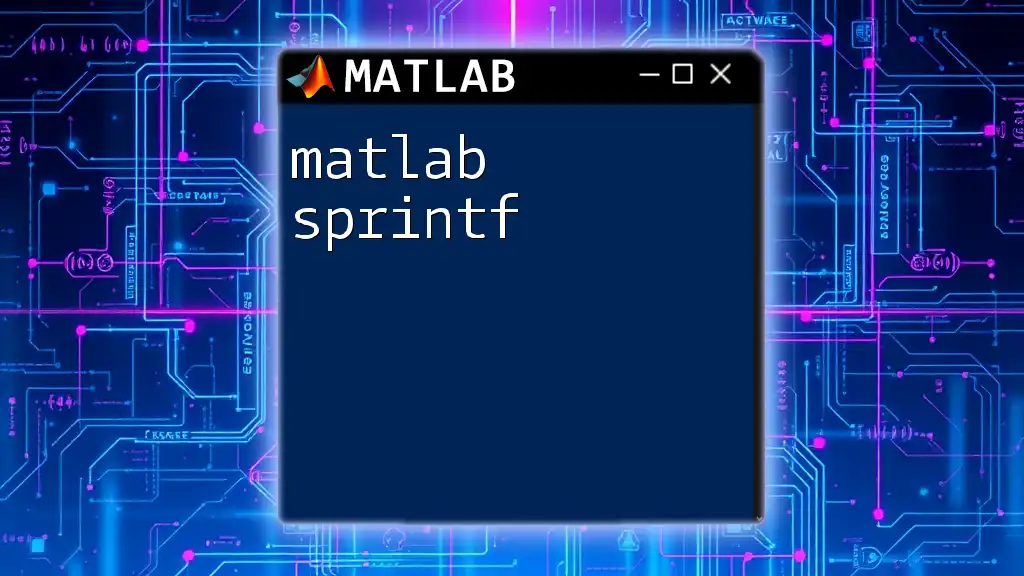
Troubleshooting Common Issues
Common Errors in Substring Operations
One prevalent error occurs when trying to access indices that exceed the string's length. For example, if you attempt to access an index of 15 in a string of length 10, MATLAB will throw an error.
Debugging Tips
Utilize MATLAB's built-in debugging tools, such as breakpoints and variable inspection, to trace issues. Consider the following example:
% Incorrect index example
str = 'Hello MATLAB!';
ind = 15; % This index is out of bounds
if ind > length(str)
error('Index exceeds string length');
end
By employing debugging strategies, you can efficiently identify and rectify issues in your substring operations.
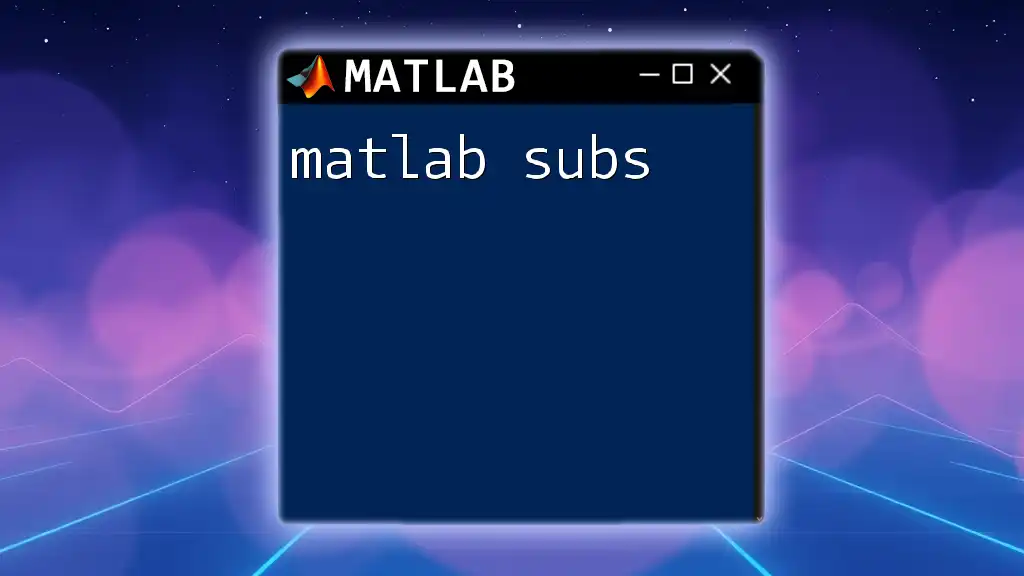
Conclusion
In summary, the concept of MATLAB substring operations is foundational for anyone working with strings in MATLAB. From accessing characters to extracting meaningful portions, these methods are invaluable. Embrace continued learning and practice using the code snippets provided here, and don't hesitate to explore MATLAB’s official documentation as you advance in your string manipulation skills.
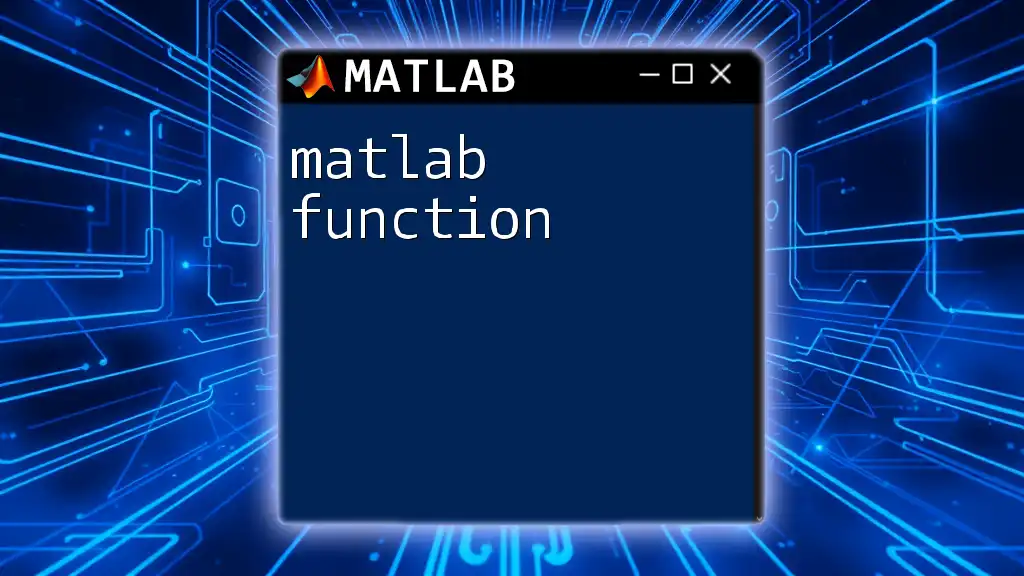
Additional Resources
To further your knowledge of MATLAB, consider engaging with the following:
- Books and Tutorials: Seek out well-reviewed resources that cover string operations and MATLAB programming comprehensively.
- Online Communities: Join forums or user groups where MATLAB enthusiasts share insights and techniques, enhancing your learning experience.