In MATLAB, you can convert a cell array to a vector using the `cell2mat` function, which combines the contents of the cell array into a single numeric array.
% Example of converting a cell array to a vector
cellArray = {1, 2, 3, 4, 5}; % Define a cell array
vector = cell2mat(cellArray); % Convert the cell array to a vector
Understanding MATLAB Data Types
Overview of MATLAB Data Types
Understanding different data types in MATLAB is crucial for effective programming and data manipulation. MATLAB supports various data types, including scalars, vectors, matrices, and cell arrays. A scalar is a single value, while a vector is a one-dimensional array of values. A matrix is a two-dimensional array. In contrast, cell arrays are special data types that can contain different types of data elements, such as numbers, strings, or other cell arrays.
What is a Cell Array in MATLAB?
Cell arrays are perhaps one of MATLAB's most powerful features. They allow for the storage of heterogeneous data types, meaning you can mix different types of data within the same array. This flexibility is particularly useful when your data sets do not conform to a single data type—for example, when dealing with a combination of numeric data and strings. Using cell arrays, you can index into complex structures easily and efficiently, making data management in MATLAB simpler.
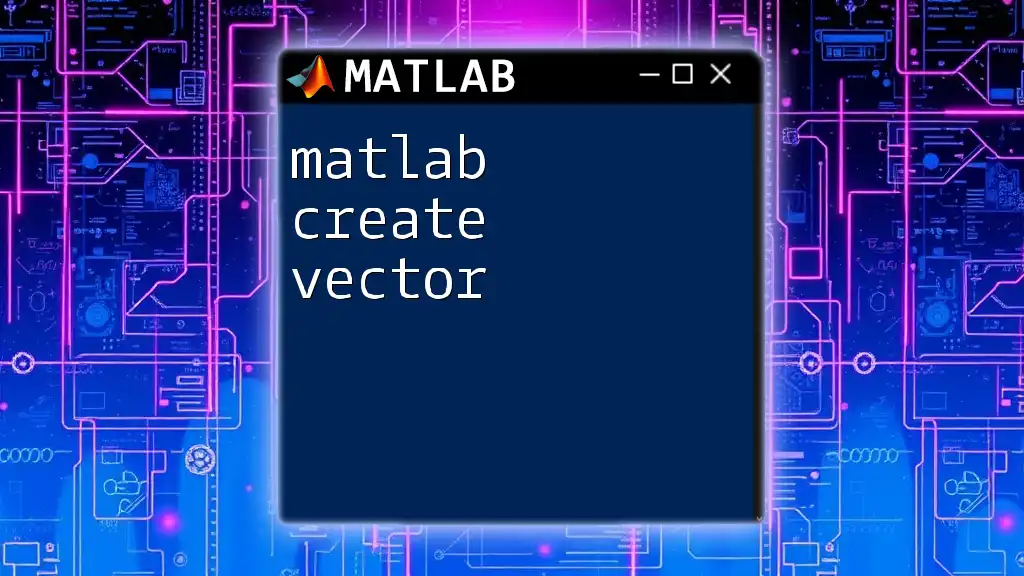
Converting Cell Arrays to Vectors
Why Convert Cell Arrays to Vectors?
In many scenarios, you may find the need to convert cell arrays to vectors. This can arise during data manipulation or mathematical operations, where working with vectors is usually more straightforward. Vectors are computationally more efficient for operations such as arithmetic, statistical calculations, and plotting. Therefore, knowing how to convert cell arrays to vectors effectively is essential for optimizing your MATLAB workflows.
Basic Conversion from Cell Array to Vector
The most straightforward method for converting a cell array to a vector in MATLAB is using the `cell2mat` function. This function seamlessly transforms a cell array of numeric data into a standard numeric array (vector).
How it works: The `cell2mat` function concatenates the contents of the cell array into one contiguous array. It is essential that all the elements in the cell are of a compatible data type.
cellArray = {1, 2, 3, 4}; % Example cell array
vector = cell2mat(cellArray); % Convert to a numeric vector
Expected Output: The output will be a numeric vector:
vector =
1 2 3 4
Handling Different Data Types in Cell Arrays
When dealing with cell arrays that contain strings or characters, you cannot typically use `cell2mat` directly because it is designed for numeric data. Instead, you'll need to convert the cell content to a different type using the `string` function for more straightforward handling.
cellArray = {'apple', 'banana', 'cherry'};
stringVector = string(cellArray); % Convert to a string array
In this example, `stringVector` will now be a string array containing:
"apple" "banana" "cherry"
Converting Nested Cell Arrays
Nested cell arrays introduce additional complexity because they contain other cell arrays as their elements. Flattening these nested structures into a single vector requires specific techniques.
Understanding complexity: A nested cell array might look something like this:
nestedCell = {{1, 2}, {3, 4}};
To convert it into a vector, use the following syntax:
flatVector = [nestedCell{:}]; % Flatten and convert to vector
This command extracts all the elements from the nested cell array, resulting in the vector:
flatVector =
1 2 3 4
However, you must ensure that all elements are compatible types for successful conversion.
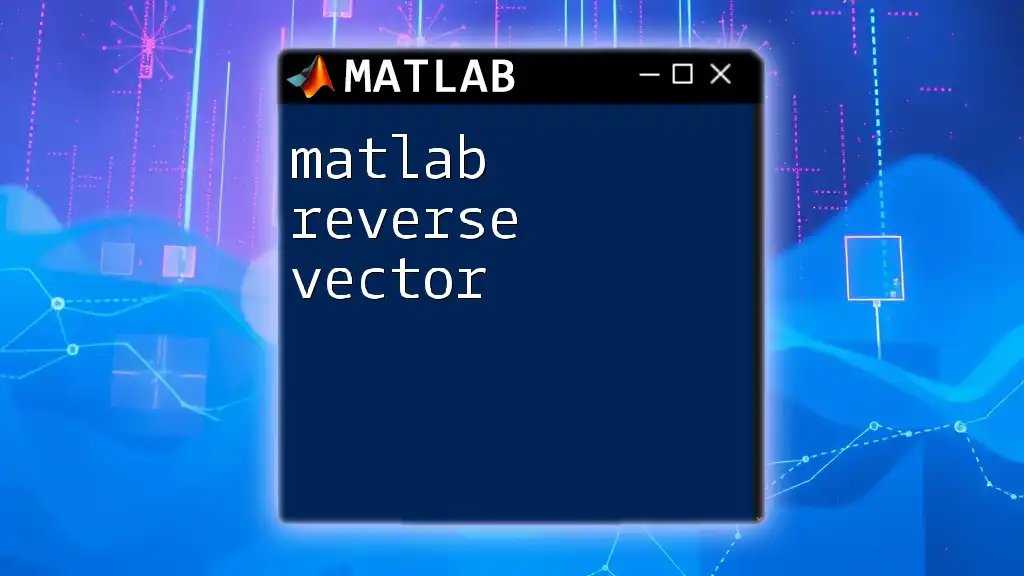
Practical Examples
Example 1: Numeric Cell Array to Vector
Let’s take a step-by-step approach to converting a numeric cell array into a vector. Consider the following example:
numCellArray = {1, 2, 3, 4.5};
numVector = cell2mat(numCellArray);
The resultant `numVector` will be:
numVector =
1 2 3 4.5
This output illustrates how simple it is to transform a numeric cell array into a vector, enabling further mathematical processing.
Example 2: Logical Cell Array to Vector
Logical data can also be effectively converted from a cell array to a vector. Here’s how you can do that:
logicalCell = {true, false, true};
logicalVector = cell2mat(logicalCell);
The converted vector will be:
logicalVector =
1 0 1
This output demonstrates that `true` is represented as `1` and `false` as `0`, showing how logical values can be numerically interpreted in MATLAB.
Example 3: Cell Array Containing Mixed Data Types
One of the common challenges you may face is when dealing with a cell array that contains mixed data types. Attempting to convert such a structure directly using `cell2mat` will result in an error:
mixedCell = {1, 'text', 3.5};
% Attempting to convert to vector directly will cause an error
To handle this scenario, you may need to iterate through the cell array and extract or convert the elements based on their types, ensuring uniformity before conversion.
numericValues = [];
for i = 1:length(mixedCell)
if isnumeric(mixedCell{i})
numericValues(end+1) = mixedCell{i}; % Store only numeric values.
end
end
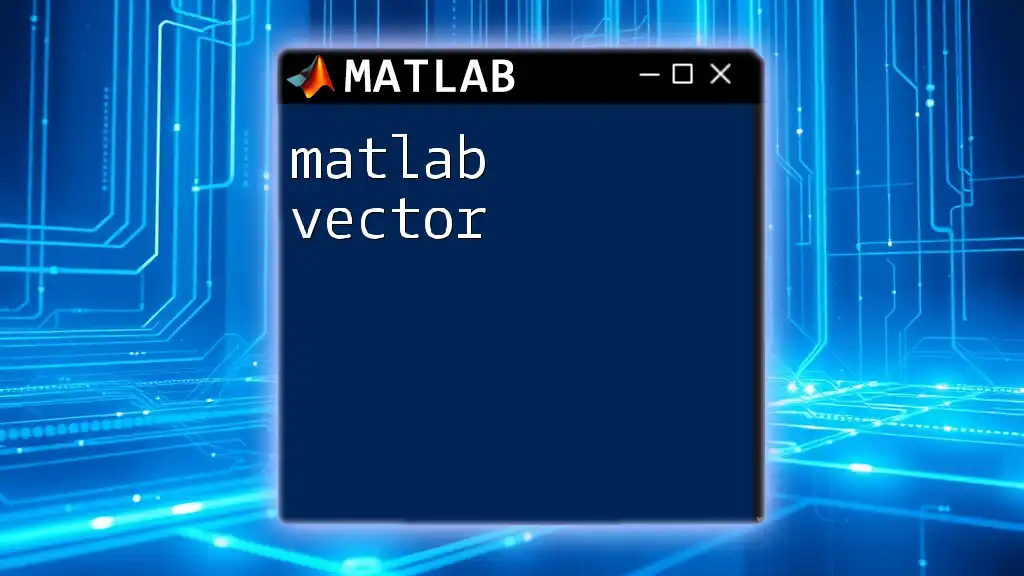
Tips and Best Practices
Best Practices for Cell to Vector Conversion
When converting from cell arrays to vectors, it’s crucial to ensure that all elements within the cell array are of a uniform data type. This uniformity makes the conversion process smoother and avoids common errors that arise during the transformation. Whenever possible, plan your data structures in advance to facilitate easy conversions.
Common Mistakes and How to Avoid Them
A frequent misunderstanding among newcomers is attempting to convert incompatible data types, leading to runtime errors. Always check your cell array data types before performing conversions. Using functions like `class()` can help you verify the type of each cell element, ensuring a compatible conversion.
cellArray = {1, 'banana', true};
for i = 1:length(cellArray)
disp(class(cellArray{i})); % Display the type of each element
end
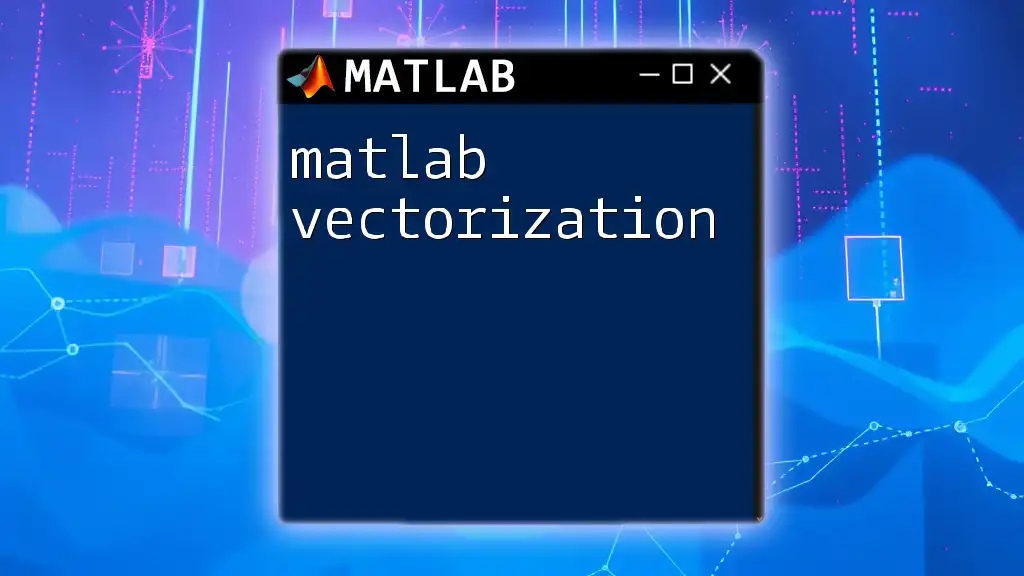
Conclusion
In summary, mastering the conversion of MATLAB cell to vector is essential for effective data manipulation in your MATLAB programming. By understanding the functions available and the characteristics of different data types, you can optimize your workflows and enhance your MATLAB programming proficiency. Experiment with the examples provided and consider expanding your knowledge through further resources or workshops to deepen your understanding of this versatile tool.