To reverse a vector in MATLAB, you can use the `flip` function or indexing to reorder the elements in the opposite direction.
Here’s a code snippet demonstrating both methods:
% Method 1: Using the flip function
original_vector = [1, 2, 3, 4, 5];
reversed_vector = flip(original_vector);
% Method 2: Using indexing
reversed_vector_indexing = original_vector(end:-1:1);
Understanding Vectors in MATLAB
What is a Vector?
In MATLAB, a vector is a one-dimensional array used for storing a sequence of numbers. Vectors can be categorized into two types: row vectors and column vectors. A row vector is defined as a single row with multiple columns, while a column vector consists of multiple rows with a single column.
Creating Vectors
Vectors can be created using arrays or MATLAB built-in functions.
To create a row vector, you can directly input the values within square brackets, separating each element with a comma or space:
rowVec = [1, 2, 3, 4, 5];
For a column vector, use a semicolon to separate each element:
colVec = [1; 2; 3; 4; 5];
Additionally, the `linspace` function allows for the generation of vectors where you specify the start value, end value, and the total number of points:
linspaceVec = linspace(1, 5, 5); % Creates a row vector: [1 2 3 4 5]
The colon operator is another convenient way to create vectors:
colonVec = 1:1:5; % Row vector using the colon operator: [1 2 3 4 5]
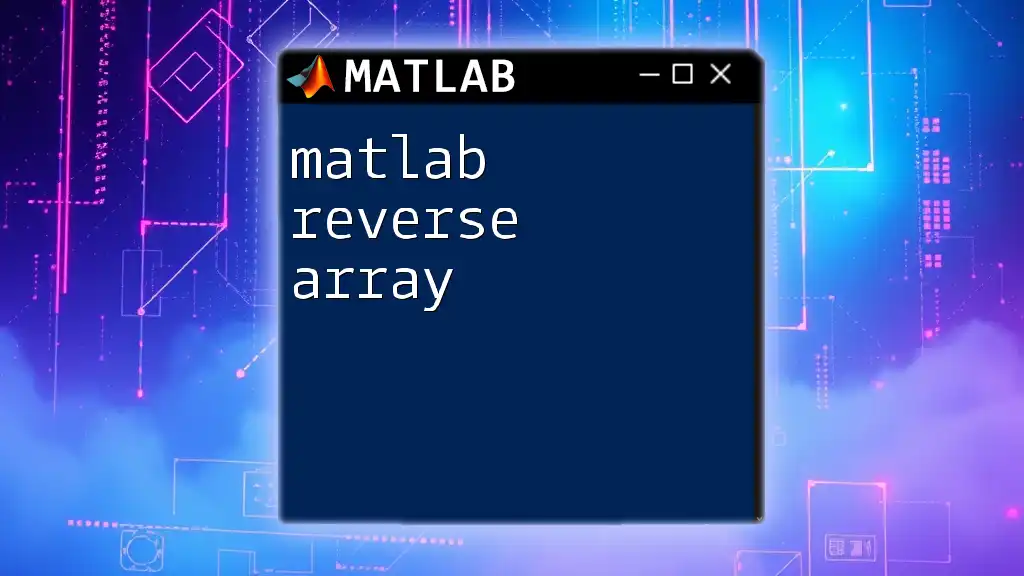
Reversing a Vector in MATLAB
Why Reverse a Vector?
Reversing a vector may be necessary in various real-world scenarios, including data preprocessing, analysis of time series data, or manipulating arrays in algorithms. Whether it’s flipping data for plotting or rearranging values for further computations, knowing how to reverse a vector is key.
Basic Command to Reverse a Vector
To reverse a vector in MATLAB, you can simply use the built-in function `flip`. This command can reverse any array, including matrices and multidimensional arrays.
Here’s how you can use it:
reversedVec = flip(originalVec);
Examples of Reversing Vectors
Reversing a Row Vector
For a practical example, consider the following code snippet that reverses a row vector:
originalRowVec = [1, 2, 3, 4, 5];
reversedRowVec = flip(originalRowVec);
% Output: reversedRowVec = [5, 4, 3, 2, 1]
This flips the order of the elements, allowing for easy data manipulation.
Reversing a Column Vector
Reversing a column vector follows the same principle. Here’s how you can achieve it:
originalColVec = [1; 2; 3; 4; 5];
reversedColVec = flip(originalColVec);
% Output: reversedColVec = [5; 4; 3; 2; 1]
The output organizes the elements in reverse, maintaining their structure.
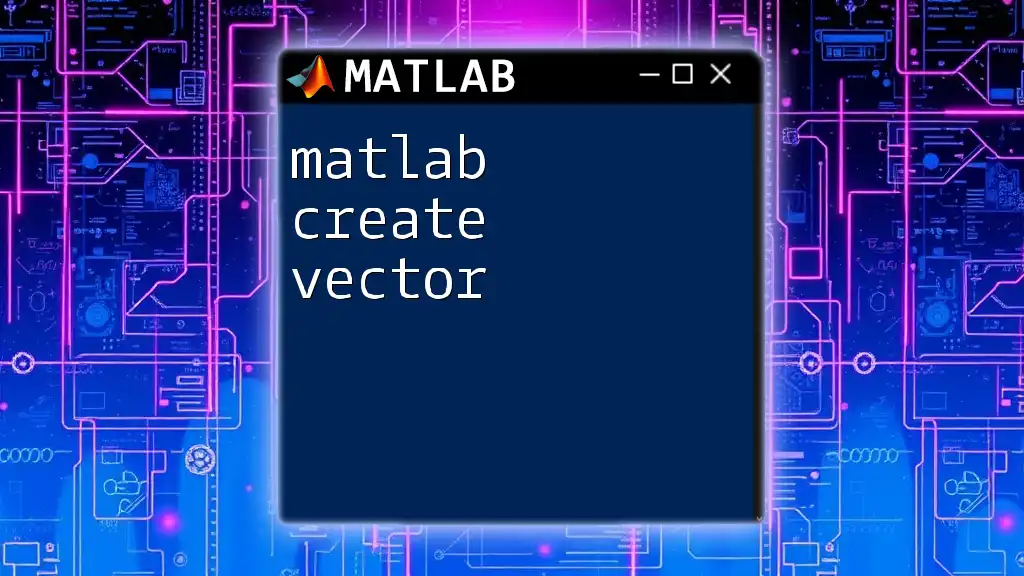
Alternative Methods to Reverse Vectors
Using Indexing
Another straightforward method to reverse a vector is by using indexing. This technique exploits MATLAB’s capability of handling ranges and can be particularly useful in programmatic scenarios:
reversedVecUsingIndexing = originalVec(end:-1:1);
This command constructs a new vector by accessing elements of `originalVec` from the last to the first.
Using Loop (for educational purposes)
While less efficient, implementing a reversing mechanism using a loop can provide insights into how vectors are structured. Here’s a simple example:
n = length(originalVec);
reversedLoopVec = zeros(1, n);
for i = 1:n
reversedLoopVec(i) = originalVec(n-i+1);
end
This code manually assigns elements in reverse order to a new vector initialized to zeros.
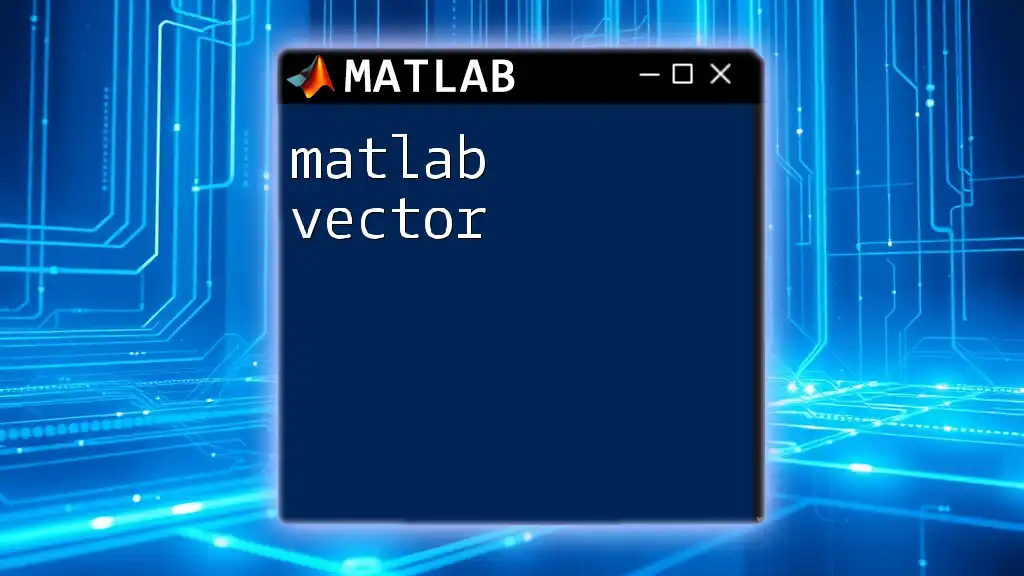
Common Mistakes to Avoid
Confusing Row and Column Vectors
One common mistake when reversing vectors involves treating row and column vectors interchangeably. Always ensure that the orientation of your vector matches your intended operations. If you have a row vector and use functions designed for column vectors (or vice-versa), you may encounter unexpected results.
Using Incorrect Function Syntax
Syntax errors can arise if you mistakenly use a function with misspelled names or incorrect parentheses. Always double-check your commands to maintain accuracy.
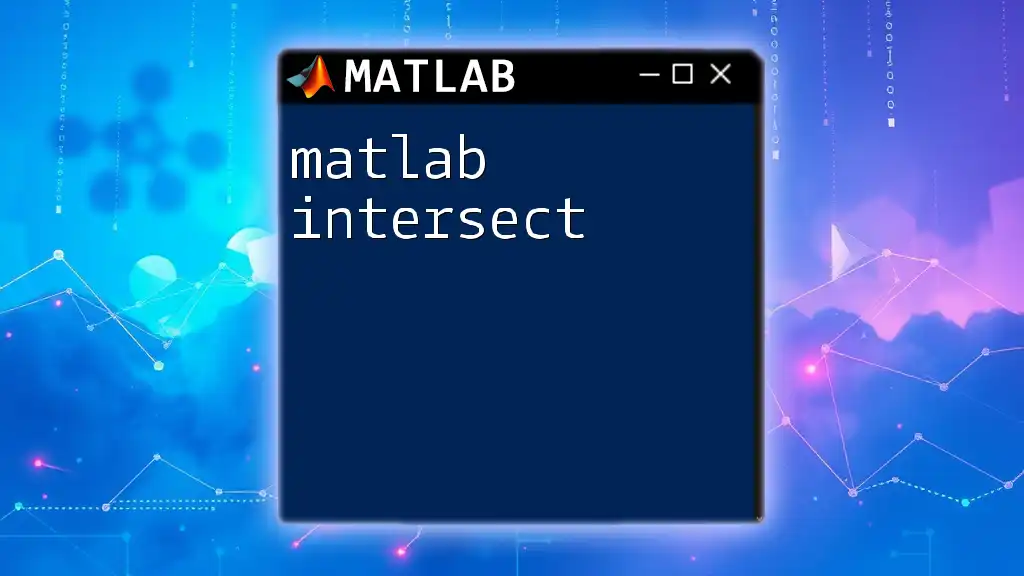
Practical Applications of Reversing a Vector
Data Processing
Reversing vectors is frequently utilized in data processing. For instance, when analyzing time series data, where the most recent observations are often stored at the end of a vector, reversing the vector gives you direct access to earlier values. This can be crucial in forecasting or visualizing trends over time.
Signal Processing
In signal processing applications, reversing a vector can play a significant role in manipulating signals, analyzing frequencies, and smoothing data for better clarity in results. For instance, in audio signal processing, reversing a sound waveform can create unique effects or assist in playback reversals.
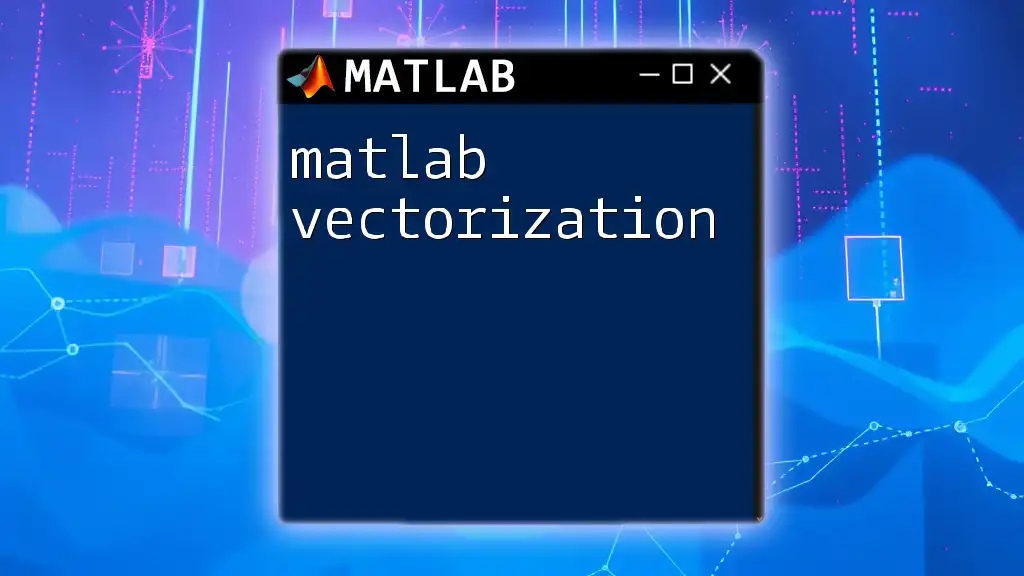
Conclusion
Mastering vector manipulation, including how to matlab reverse vector, is essential for efficient data analysis and programming in MATLAB. By utilizing the techniques outlined above, you can greatly enhance your capabilities in handling data and performing complex computations. So, dive into your MATLAB projects and practice reversing vectors—you'll find it an invaluable skill in your toolkit.
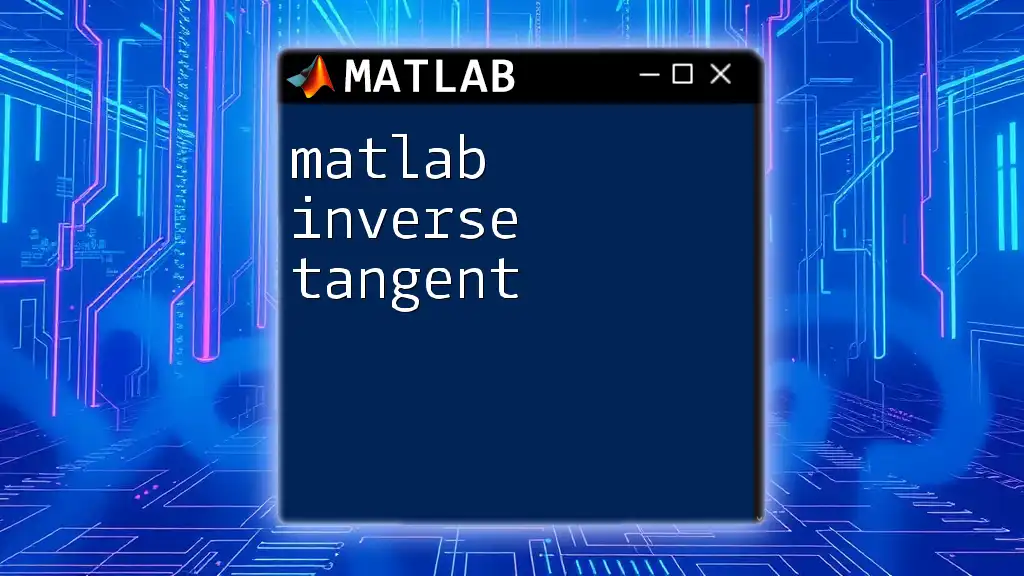
Additional Resources
To further your understanding of MATLAB and vector manipulation, consider referring to the official MATLAB documentation, which offers comprehensive resources on array and vector operations. Additionally, enrolling in recommended online courses or reading books dedicated to MATLAB can solidify your learning experience.
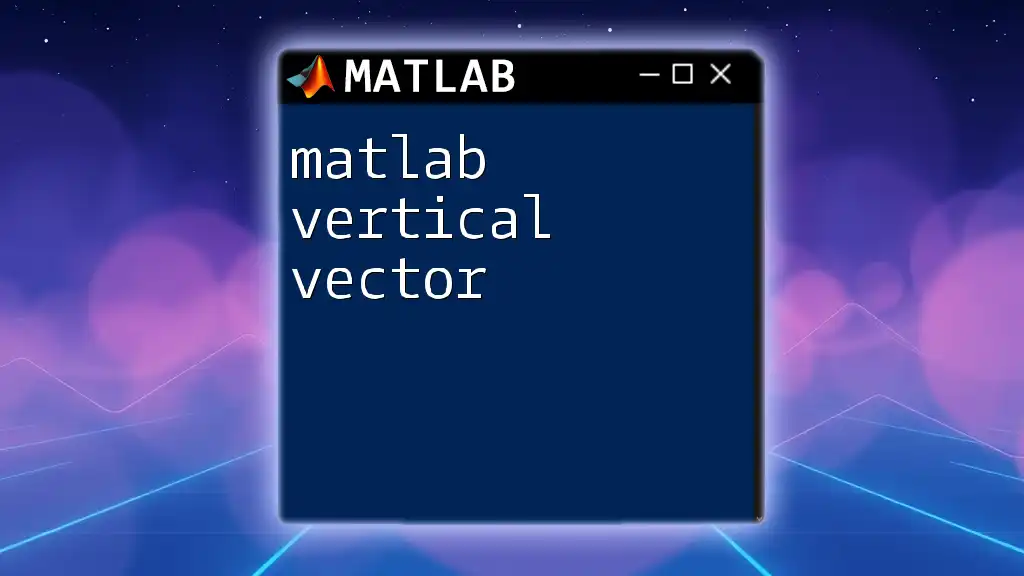
Call to Action
We invite you to join our MATLAB community where you gain access to further tutorials, insights, and tips on how to effectively use MATLAB commands. Don’t miss out on the opportunity to expand your skills and enhance your projects!