Curve fitting in MATLAB involves using various techniques to construct a mathematical function that approximates a set of data points, enabling better data analysis and prediction.
Here's a simple code snippet demonstrating linear curve fitting using MATLAB:
% Sample data points
x = [1, 2, 3, 4, 5];
y = [2.2, 2.8, 3.6, 4.5, 5.1];
% Perform linear curve fitting
p = polyfit(x, y, 1);
% Generate fitted values
yfit = polyval(p, x);
% Plot original data and fitted curve
figure;
scatter(x, y, 'filled'); % Original data points
hold on;
plot(x, yfit, '-r'); % Fitted curve
title('Linear Curve Fitting');
xlabel('X-axis');
ylabel('Y-axis');
legend('Data Points', 'Fitted Line');
hold off;
Understanding the Basics of Curve Fitting
Types of Curve Fitting
Linear Curve Fitting involves creating a straight-line equation that best describes the relationship between two variables. This is often the simplest and most widely used type of fitting, especially when there is a clear linear trend in the data.
For example, if you're analyzing height and weight data, a linear fit might be appropriate if taller individuals tend to weigh more.
Non-linear Curve Fitting is applicable when the data follows a curved trend that cannot be described by a straight line. Non-linear models may include exponential, logarithmic, or polynomial functions. This is particularly useful in cases such as growth phenomena, chemical reactions, or population studies where the relationship is more complex.
Polynomial Curve Fitting can accommodate a variety of curves by using polynomial functions of higher degrees. This allows for more flexibility in modeling data that has fluctuations or local maxima and minima.
Key Concepts in Curve Fitting
Goodness of Fit is a measure of how well the chosen model describes the observed data. It's crucial for validating the effectiveness of a curve fitting approach. The most common metrics used to evaluate the goodness of fit include:
-
R-squared: This value ranges from 0 to 1 and indicates the proportion of variance in the dependent variable that can be predicted from the independent variable. A value closer to 1 suggests a better fit.
-
Root Mean Square Error (RMSE): This metric assesses the average magnitude of the errors between predicted and observed values, providing insight into the model's accuracy.
In fitting a curve, you must also be aware of Overfitting and Underfitting.
-
Overfitting occurs when the model learns noise in the data instead of the underlying distribution, which results in high accuracy on training data but poor generalization to new data.
-
Underfitting happens when the model is too simple to capture the underlying trend of the data, leading to poor performance on both training and testing datasets.
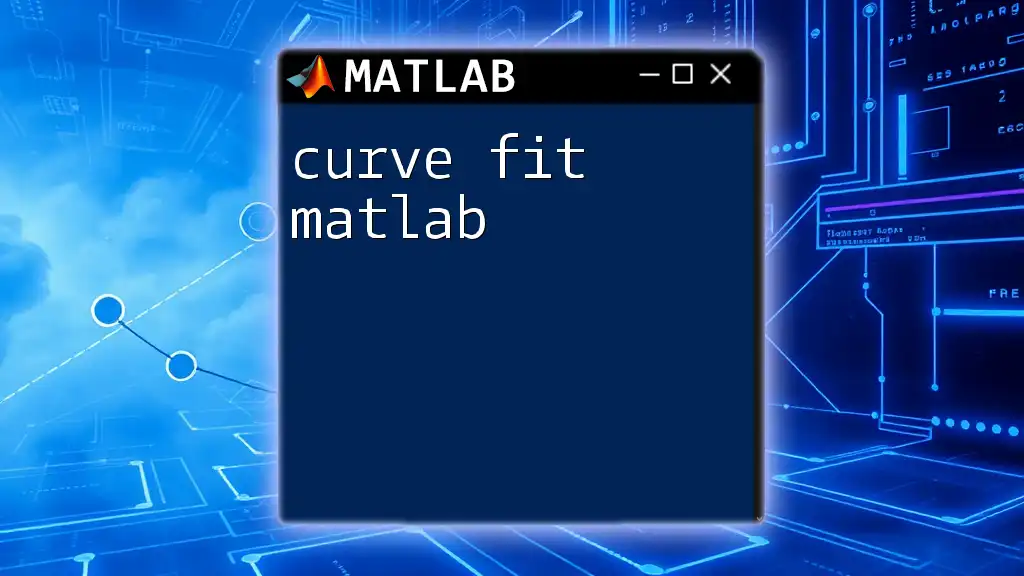
MATLAB Functions for Curve Fitting
Overview of MATLAB Curve Fitting Tools
MATLAB provides several features specifically designed for curve fitting. The Curve Fitting Toolbox is integral here, offering a user-friendly interface and powerful algorithms for quick analysis.
Key commands to remember include:
- polyfit: This function fits a polynomial to data.
- fit: This function supports fitting custom equations to data.
- lsqcurvefit: This function allows for the fitting of non-linear models by minimizing the sum of squares.
Step-by-Step Implementation of Curve Fitting in MATLAB
Linear Curve Fitting Example
To illustrate linear curve fitting, let's generate a simple dataset:
x = 1:10;
y = 2*x + 3 + randn(1,10); % Simple linear relationship with noise
Here, `y` is generated with a linear relationship plus some random noise. Now, we can fit a linear model using the `polyfit` function:
p = polyfit(x, y, 1); % Fit a 1st degree polynomial (linear)
To visualize the fit, we can evaluate the polynomial and create a plot:
yfit = polyval(p, x); % Evaluate fitted polynomial
plot(x, y, 'o', x, yfit, '-');
legend('Data', 'Fitted Line');
title('Linear Curve Fitting');
This code will plot the original data points alongside the fitted line, providing visual evidence of the fit.
Non-Linear Curve Fitting Example
Next, let’s look at non-linear curve fitting using an exponential function. First, prepare your data:
x = [0 1 2 3 4 5];
y = [1 2.7 7.4 20.1 54.6 148.4]; % Exponential growth
We can fit an exponential model as follows:
f = fit(x', y', 'exp1'); % Exponential model
To visualize the fitted model against the data:
plot(f, x, y);
title('Non-Linear Curve Fitting');
This graphical representation will clarify how well the model describes the underlying trend in the data.
Polynomial Curve Fitting Example
Now let’s generate polynomial data and perform polynomial fitting:
x = linspace(-5, 5, 100);
y = 2*x.^3 - 15*x.^2 + 4 + randn(size(x)); % Cubic data with noise
To fit a polynomial to this data, we use:
p = polyfit(x, y, 3); % Fit a 3rd degree polynomial
Evaluating the fitted polynomial allows us to visualize how well it matches the original data:
yfit = polyval(p, x);
plot(x, y, 'o', x, yfit, '-');
legend('Data', 'Fitted Polynomial');
title('Polynomial Curve Fitting');
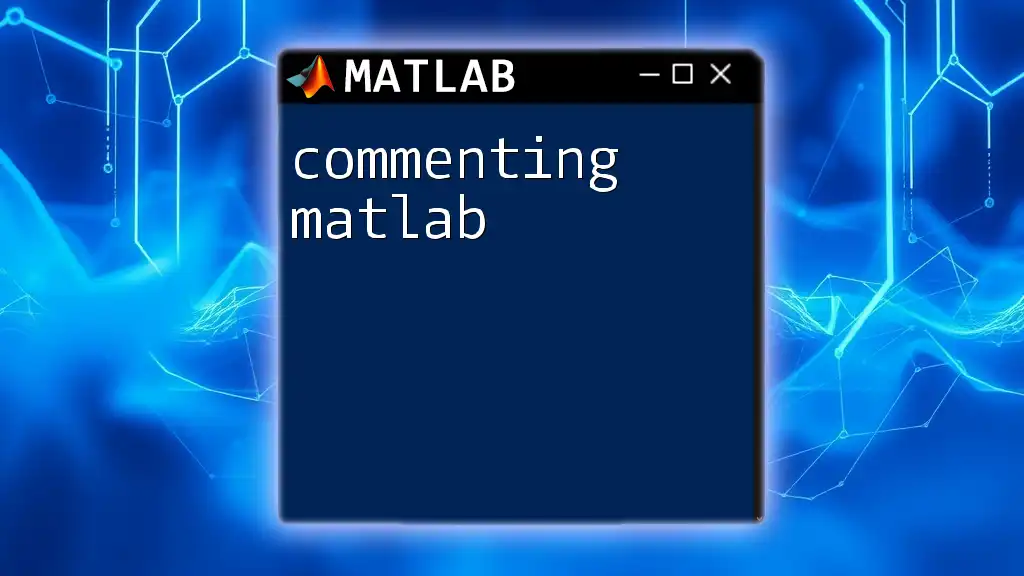
Evaluating the Fit
To evaluate the effectiveness of our fitting, analyzing the goodness of fit is essential.
Analyzing the Goodness of Fit
You can compute the R-squared value to assess how well your model fits your data:
SSR = sum((yfit - mean(y)).^2);
SST = sum((y - mean(y)).^2);
R2 = SSR / SST;
In addition to numerical assessment, visual inspection of residuals is a critical component of evaluation. Plotting residuals helps you identify patterns that may indicate issues with the fit:
residuals = y - yfit;
figure;
scatter(x, residuals);
title('Residuals');
By examining the distribution of residuals, you can gauge the quality of your fit and whether further adjustments are necessary.
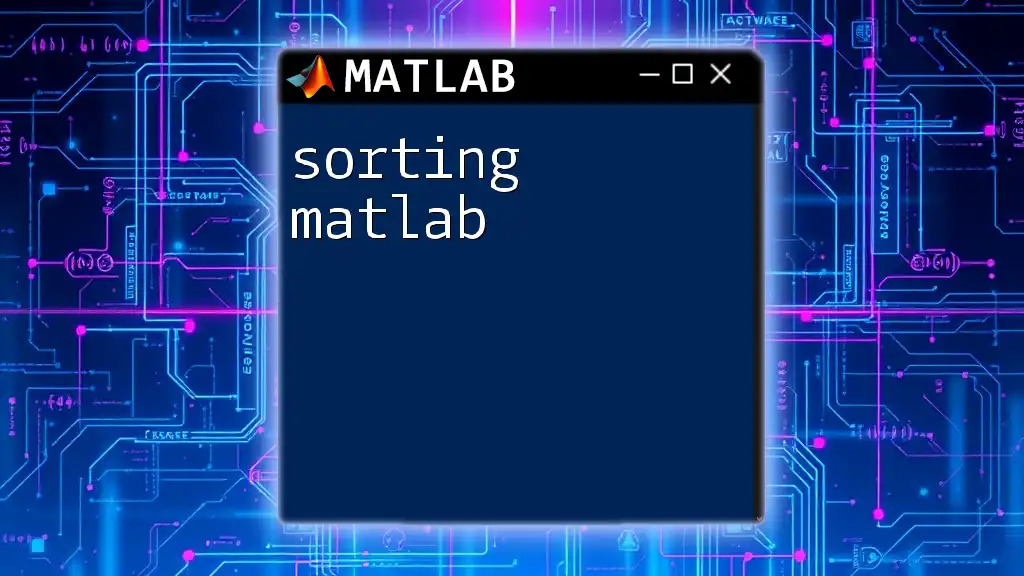
Advanced Topics in Curve Fitting
Custom Fitting Models
For scenarios where standard models do not suffice, creating custom fitting functions can be beneficial. For example, when using the `lsqcurvefit`, you can define a custom model function tailored to your specific data characteristics.
Here’s a simple illustration of a custom model function in MATLAB:
% Example Custom Model
customModel = @(b, x) b(1) * exp(b(2) * x);
Utilizing `lsqcurvefit` to fit this model to your data allows for a high level of optimization and customization.
Strategies for Improving Fit
Regularization Techniques, such as Lasso and Ridge regression, help moderate overfitting by adding a penalty for higher coefficients. These techniques can enhance model robustness, especially in high-dimensional datasets.
Data Transformation Techniques are also crucial. Scaling or normalizing data can greatly affect model performance, allowing for a clearer pattern to emerge from the noise.
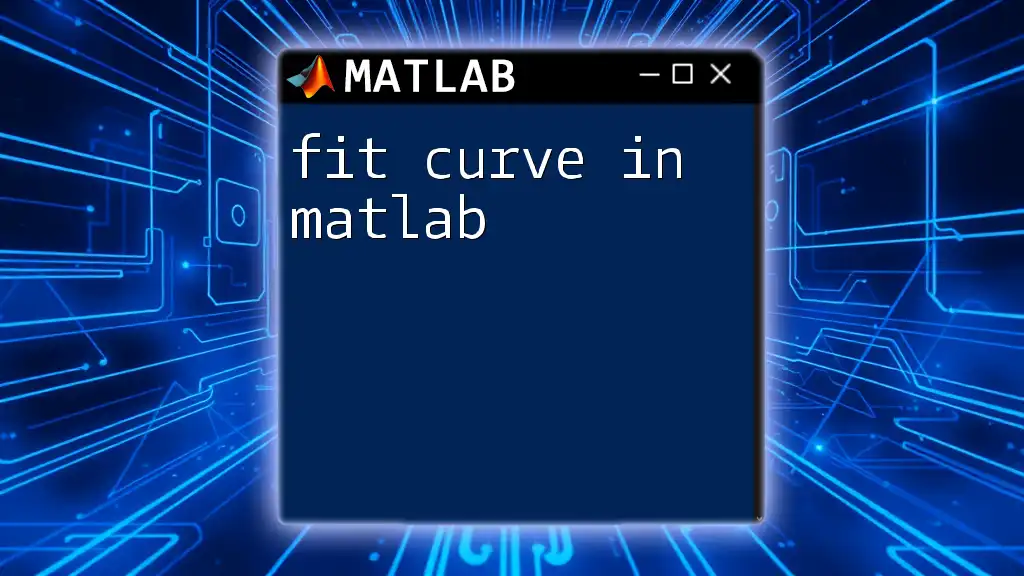
Conclusion
To summarize, understanding curve fitting in MATLAB involves mastering various fitting techniques, comprehending key concepts and metrics that ensure a quality fit, and leveraging MATLAB's robust toolkit for implementation. As you journey through curve fitting, practicing the provided examples will be instrumental in deepening your understanding. These foundations will serve you well as you tackle more complex datasets and fitting challenges.
Next Steps: Seek additional resources and curves to analyze, and join communities focused on MATLAB curve fitting to enhance your learning experience. Embrace the practice, and your skills will surely develop!