The MATLAB determinant is a scalar value that provides insights into the properties of a square matrix, indicating whether the matrix is invertible (non-zero determinant) or singular (zero determinant).
Here's a simple code snippet to calculate the determinant of a matrix in MATLAB:
A = [1, 2; 3, 4];
det_A = det(A);
disp(det_A);
Understanding the Determinant
Definition of a Determinant
A determinant is a scalar value that can be computed from a square matrix and provides significant information about the matrix. In linear algebra, the determinant reflects the volume scaling factor of the linear transformation represented by the matrix. Determinants are not only crucial in solving systems of linear equations but also play an essential role in various applications such as physics, engineering, and computer graphics.
Properties of Determinants
Basic Properties
- Product of Eigenvalues: The determinant of a matrix is equal to the product of its eigenvalues, reflecting how the matrix scales the volume in its surrounding space.
- Multiplier Property: If you multiply a row of a matrix by a scalar, the determinant of the matrix is scaled by that same scalar.
- Effect of Row Operations: Swapping two rows of a matrix changes the sign of the determinant, while adding a multiple of one row to another does not affect the determinant.
Advanced Properties
- Block Matrices: The determinant behaves well with block matrices, and calculating it can often be simplified if the blocks are structured properly.
- Inverse Matrices: The determinant can indicate whether a matrix is invertible; a determinant of zero means that the matrix does not have an inverse.
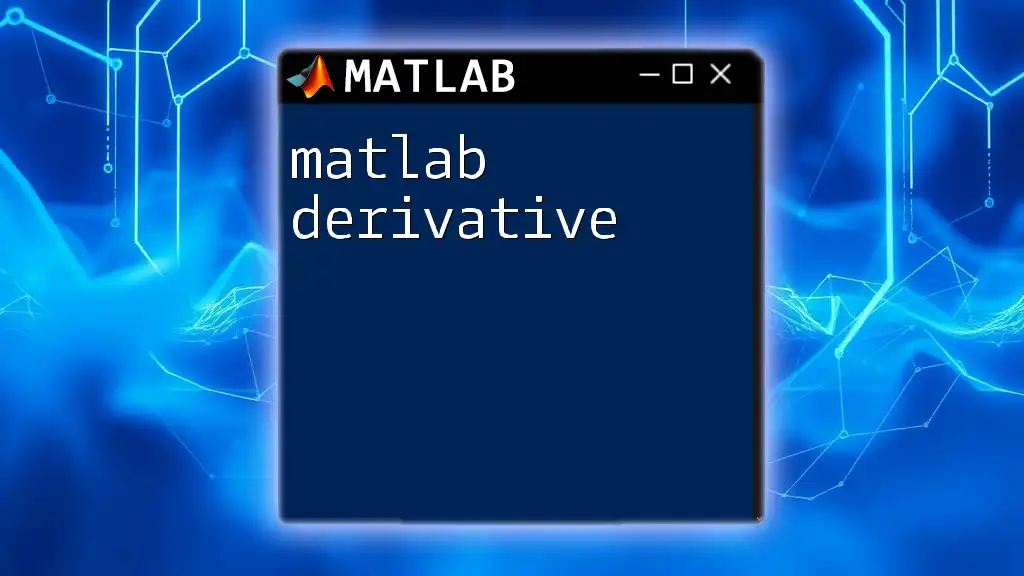
Creating Matrices in MATLAB
MATLAB Matrix Basics
In MATLAB, matrices are the fundamental data type, and understanding how to create them is crucial for working with the matlab determinant. You can create both 1D and 2D matrices using simple syntax.
To create a 2x2 matrix, you can use the following code snippet:
A = [1, 2; 3, 4]; % 2x2 Matrix
Special Matrix Types
Understanding special kinds of matrices can significantly simplify the process of calculating determinants. Here are a few types you might want to familiarize yourself with:
- Identity Matrix: This square matrix has 1s on the diagonal and 0s elsewhere. You can create a 3x3 identity matrix in MATLAB like this:
I = eye(3); % 3x3 Identity Matrix
- Zero Matrix: A matrix where all elements are zero. Creating a 3x3 zero matrix is simple:
Z = zeros(3); % 3x3 Zero Matrix
- Symmetric Matrices: A matrix that is equal to its transpose. An example of creating a symmetric matrix is:
S = [1, 2; 2, 1]; % Symmetric Matrix
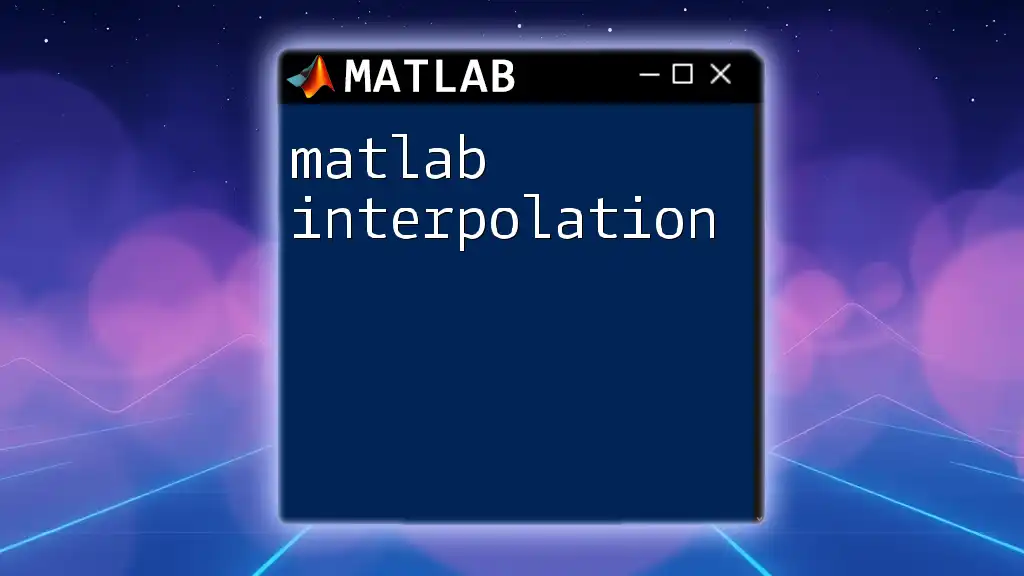
Calculating the Determinant in MATLAB
Using the `det` Function
The built-in `det` function in MATLAB is the primary method for calculating the determinant of a matrix. The syntax is straightforward and typically takes a matrix as input:
detA = det(A); % Calculate determinant of matrix A
The output, `detA`, provides the scalar value of the determinant, which you can then use for various analyses or checks in your calculations.
Manual Determinant Calculation
While the `det` function is convenient, understanding the underlying process can deepen your comprehension of determinants.
2x2 Matrix Example
For a 2x2 matrix, the determinant can be calculated using a simple formula. For instance:
A = [1, 2; 3, 4];
detA = (1*4) - (2*3); % Manual calculation
In this case, the determinant is calculated as \(1 \times 4 - 2 \times 3 = -2\).
3x3 Matrix Example
The determinant of a 3x3 matrix can be calculated using a more elaborate formula that includes minors and cofactors. Take the matrix:
B = [1, 2, 3; 0, 4, 5; 1, 0, 6];
detB = B(1, 1)*det(B(2:3, 2:3)) - B(1, 2)*det(B(2:3, [1,3])) + B(1, 3)*det(B(2:3, 1:2));
Here, `det(B(2:3, 2:3))` calculates the determinant of the submatrix formed by removing the first row and first column, and so forth for the other terms.
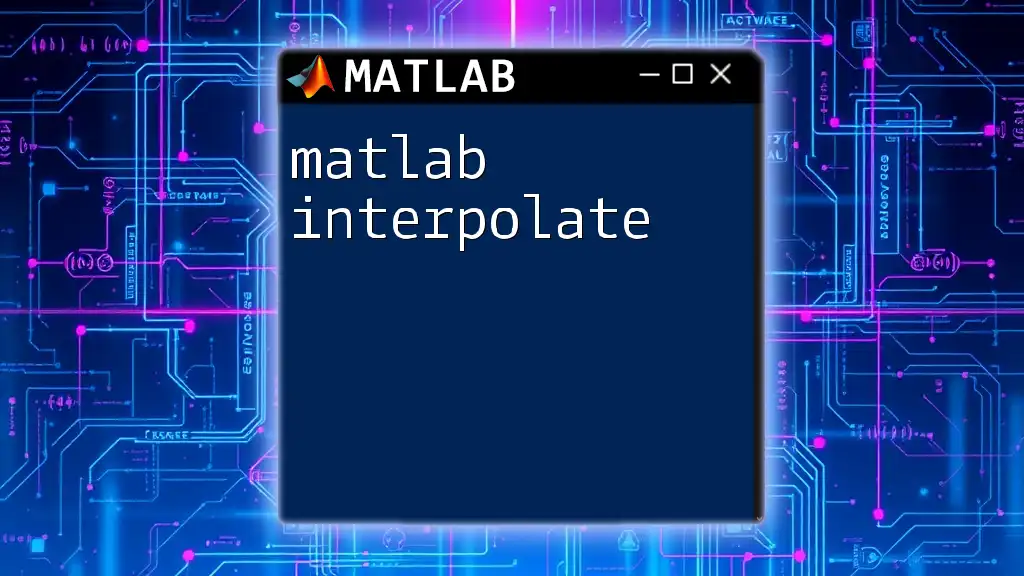
Understanding the Output of Determinants
Interpreting Determinant Values
The values of determinants provide important information about the properties of the matrix:
- A determinant value of 0 indicates that the matrix is singular, meaning it does not have an inverse and represents dependent vectors.
- A positive determinant indicates that the linear transformation preserves orientation and scales the volume positively.
- A negative determinant signifies that the transformation reverses orientation while also scaling the volumes.
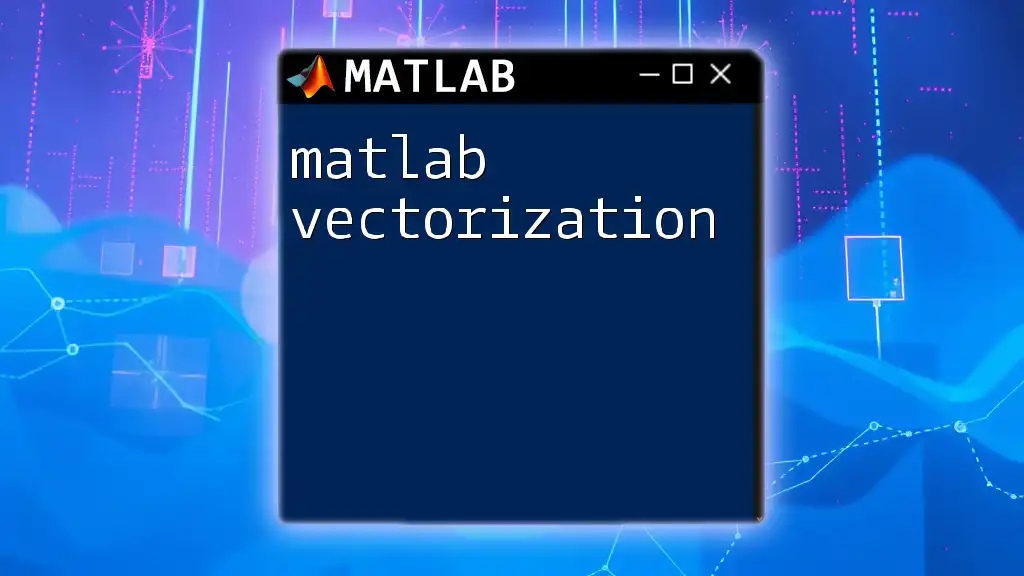
Common Issues and Troubleshooting
Errors while Calculating Determinants
Calculating determinants in MATLAB can sometimes lead to errors. Common issues include:
- Non-Square Matrices: Attempting to calculate the determinant of a non-square matrix results in an error. Always ensure your matrix is square.
- Numeric Precision: For large matrices, the determinant can approach zero due to numerical precision issues.
Performance Considerations
Calculating determinants for exceptionally large matrices can be computationally intensive. To improve performance:
- Focus on optimizing the matrix structure.
- Consider using other methods (like examining the matrix properties) to determine invertibility rather than calculating the determinant directly.
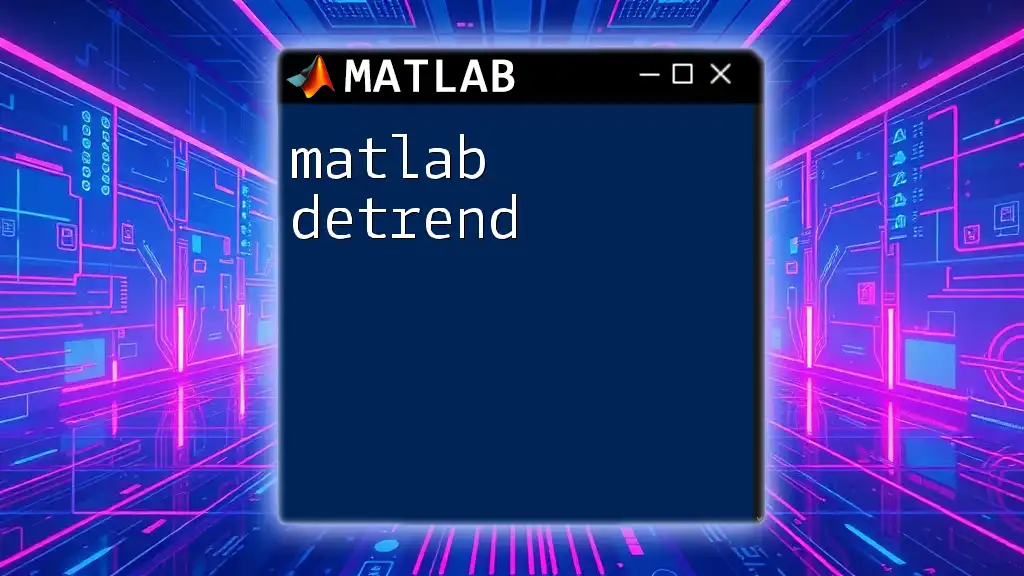
Practical Applications of Determinants
In Systems of Linear Equations
Determinants are integral in determining the solvability of systems of linear equations. A non-zero determinant indicates a unique solution, while a zero determinant suggests that the system may have either no solution or infinitely many. You could use the following code snippet to check this in MATLAB:
C = [2, -3, 1; 4, 2, -1; -2, 1, 1];
if det(C) ~= 0
% System has a unique solution
end
In Eigenvalue Problems
Determinants play a crucial role when finding eigenvalues. The eigenvalues can be understood as the roots of the characteristic polynomial, which is defined using determinants. When calculating eigenvalues, you often solve the equation \( \text{det}(A - \lambda I) = 0 \).
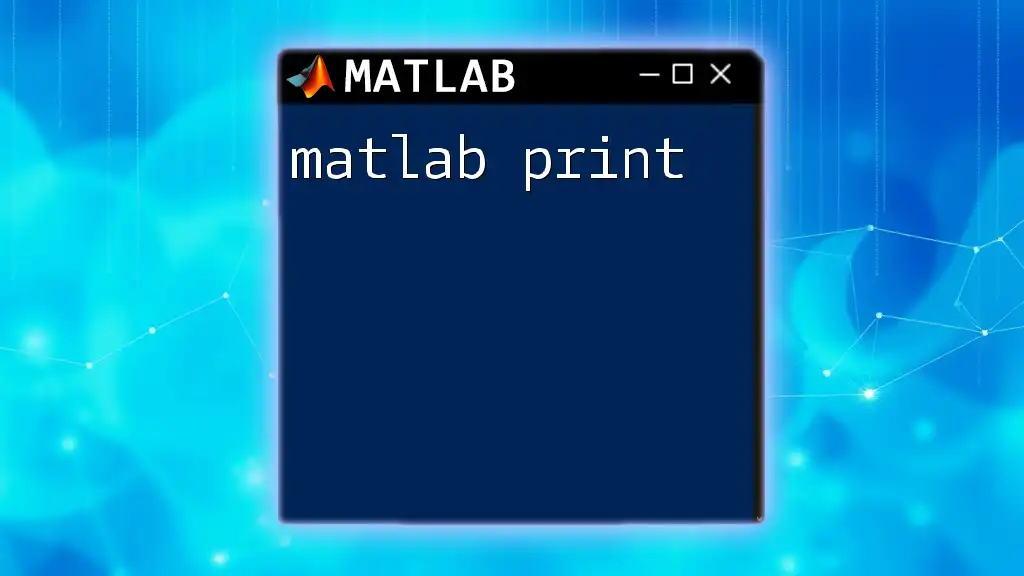
Conclusion
The matlab determinant is a fundamental concept in linear algebra with diverse applications across various fields. Mastering its calculation and properties can enhance your mathematical toolkit and improve your analytical skills. By practicing with MATLAB commands and understanding the concepts behind them, you can leverage the power of determinants in solving complex problems efficiently.
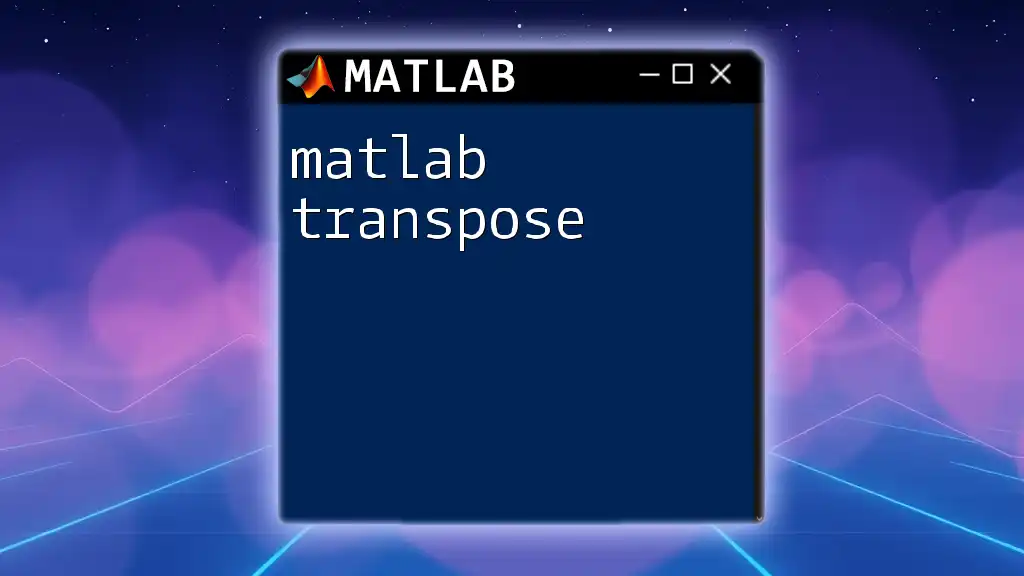
Additional Resources
Recommended Reading
For further exploration of determinants and their applications, consider diving into dedicated textbooks on linear algebra or accessing MATLAB’s official documentation.
Practice Problems
To solidify your understanding, tackle a series of practice problems involving the calculation of determinants in MATLAB.