In MATLAB, the exponential function can be utilized to calculate the mathematical constant \( e \) raised to the power of a specified number, using the `exp` function. Here's a code snippet demonstrating its usage:
% Calculate e^2 and store the result in a variable
result = exp(2);
disp(result); % Display the result
What is an Exponential Function?
Definition of Exponential Functions
Exponential functions are mathematical functions of the form \( f(x) = a \cdot b^{x} \), where \( a \) is a constant, \( b > 0 \), and \( b \) is the base of the exponential. The most common base used is Euler's number \( e \) (approximately 2.71828), leading to the specific function \( f(x) = e^{x} \).
Properties of Exponential Functions
Exponential functions exhibit unique characteristics, such as:
- Rapid Growth or Decay: Depending on the value of the exponent, exponential functions can grow extremely rapidly or decay swiftly. For instance, when the base \( b > 1 \), the function represents exponential growth; when \( 0 < b < 1 \), it represents exponential decay.
- Continuous and Smooth: These functions are continuous for all real numbers and have a smooth curve.
- Horizontal Asymptote: The x-axis (y = 0) is a horizontal asymptote for exponential decay.
These properties make exponential functions pivotal in various fields, including biology (population models), finance (compound interest), and physics (radioactive decay).
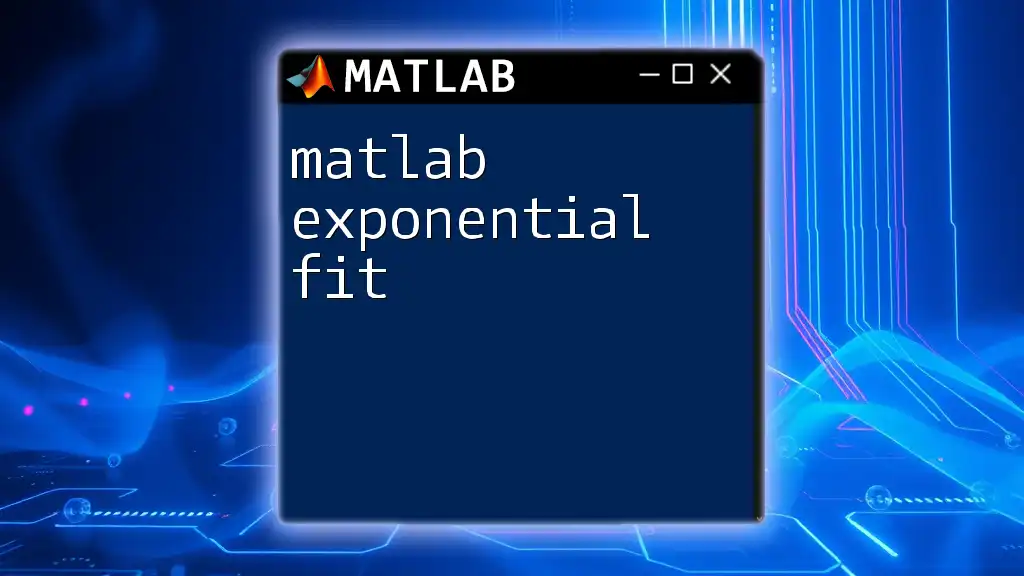
Working with Exponential in MATLAB
Basic Exponential Command
One of the simplest commands to compute the exponential of a number in MATLAB is the `exp()` function. The syntax is straightforward:
Y = exp(X);
Here, if you want to calculate the exponential of a number like 2, you can do so easily:
% Calculate the exponential of a number
x = 2;
y = exp(x); % y = e^2
disp(['The exponential of ' num2str(x) ' is ' num2str(y)]);
In this example, the output will display the value of \( e^2 \).
Generating Vectors with Exponential Values
Creating a Vector of Exponential Values
You can create a vector of exponential values over a specified range using the `linspace` function. This is especially useful for plotting functions or conducting further analysis. For example, if you want to generate exponential values from 0 to 5:
% Create a vector from 0 to 5 with 100 points
x = linspace(0, 5, 100);
y = exp(x); % Exponential values for each element in x
This code snippet results in a vector y that holds the exponential values \( e^{0}, e^{0.05}, ... , e^{5} \).
Plotting Exponential Functions
Visualizing exponential functions can provide insights into their behavior. In MATLAB, plotting is incredibly straightforward. After generating the vectors, you can plot them:
% Plotting the exponential curve
plot(x, y);
title('Exponential Function: y = e^x');
xlabel('x');
ylabel('y');
grid on;
This snippet will create a graph depicting how the exponential function behaves as x increases.
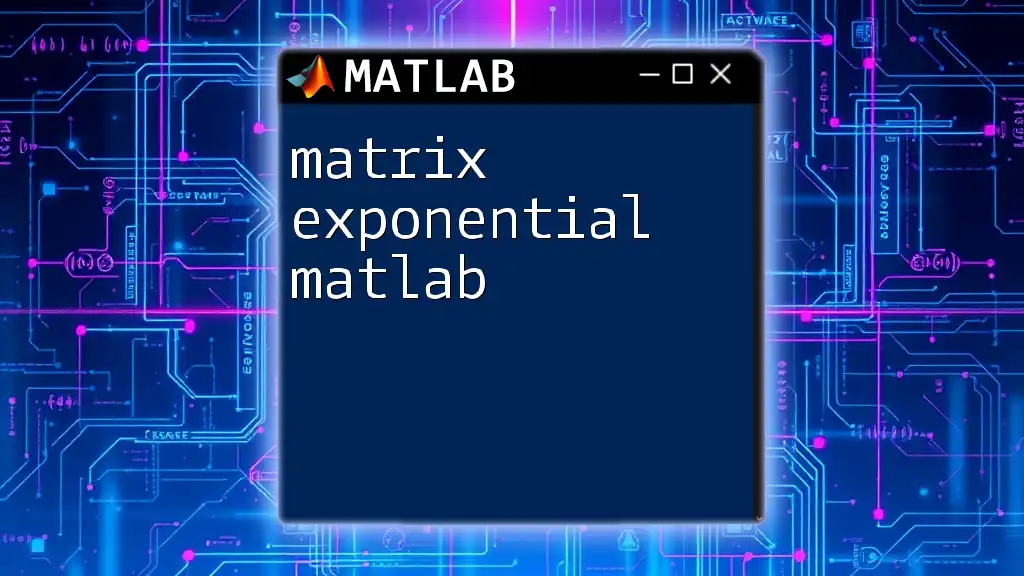
Exponential Growth and Decay
Introduction to Growth and Decay Models
Exponential functions effectively model growth and decay processes encountered in the real world. For example, population growth often follows an exponential trend, while radioactive decay is another process well modeled by exponential functions.
Exponential Growth Example: Population Growth
The general formula for modeling population growth is given by:
\[ P(t) = P_0 e^{rt} \]
where \( P_0 \) is the initial population, \( r \) is the growth rate, and \( t \) is time. The corresponding MATLAB code snippet for simulating and plotting exponential population growth looks like this:
% Parameters for exponential growth
P0 = 1000; % Initial population
r = 0.02; % Growth rate
t = linspace(0, 100, 100); % Time from 0 to 100 years
P = P0 * exp(r * t);
% Plotting Population Growth
plot(t, P);
title('Exponential Population Growth');
xlabel('Time (years)');
ylabel('Population');
grid on;
Exponential Decay Example: Radioactive Decay
Similarly, radioactive decay can be represented with the formula:
\[ N(t) = N_0 e^{-kt} \]
In this case, \( N_0 \) is the initial quantity, and \( k \) is the decay constant. Here’s a MATLAB example demonstrating radioactive decay:
% Parameters for exponential decay
N0 = 500; % Initial quantity
k = 0.1; % Decay constant
t = linspace(0, 50, 100); % Time from 0 to 50 years
N = N0 * exp(-k * t);
% Plotting Radioactive Decay
plot(t, N);
title('Exponential Radioactive Decay');
xlabel('Time (years)');
ylabel('Remaining Quantity');
grid on;
This code visualizes how the quantity dwindles over time, showcasing the decay process.
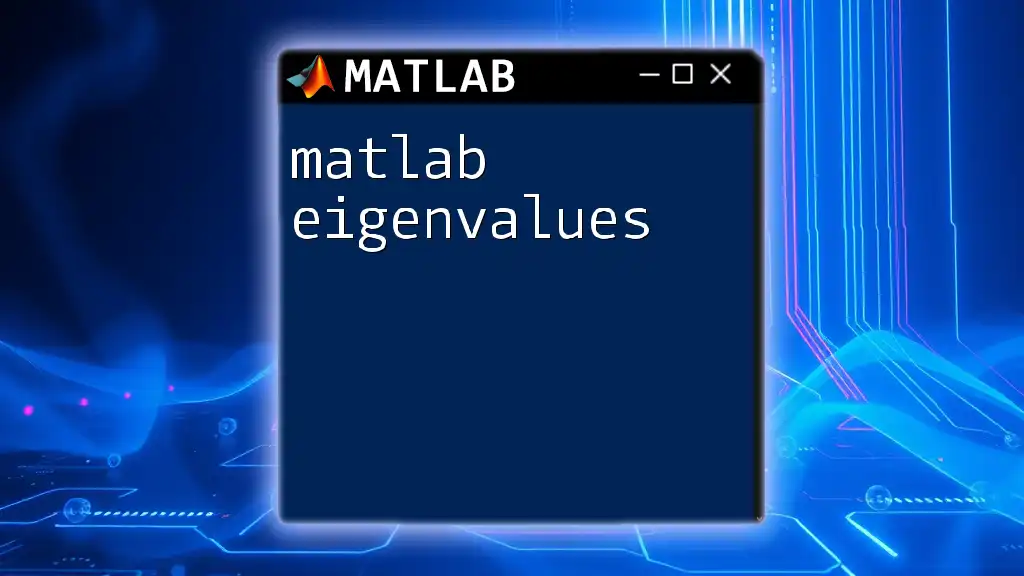
Advanced Exponential Functions in MATLAB
Complex Exponential Functions
An exciting area in mathematics is the use of complex numbers. Euler's formula states:
\[ e^{ix} = \cos(x) + i\sin(x) \]
This allows us to explore complex exponential functions in MATLAB. Here’s how you can visualize a complex exponential function:
% Using complex numbers in exponential
x = linspace(0, 2*pi, 100);
y = exp(1i * x); % Calculate e^(ix)
% Plotting the complex exponential
plot(real(y), imag(y));
title('Complex Exponential in the Complex Plane');
xlabel('Real Part');
ylabel('Imaginary Part');
grid on;
The result illustrates how complex exponential functions manifest in the complex plane.
Exponential Matrix Functions
In addition to scalar exponentials, MATLAB enables you to compute the exponential of matrices using the `expm()` function, which can be applied to a square matrix. For example:
% Matrix exponential
A = [0 1; -1 0]; % Example matrix
expA = expm(A); % Matrix exponential
disp('Matrix Exponential:');
disp(expA);
This code calculates the matrix exponential of matrix A, applying the concept of exponential functions to multi-dimensional data.
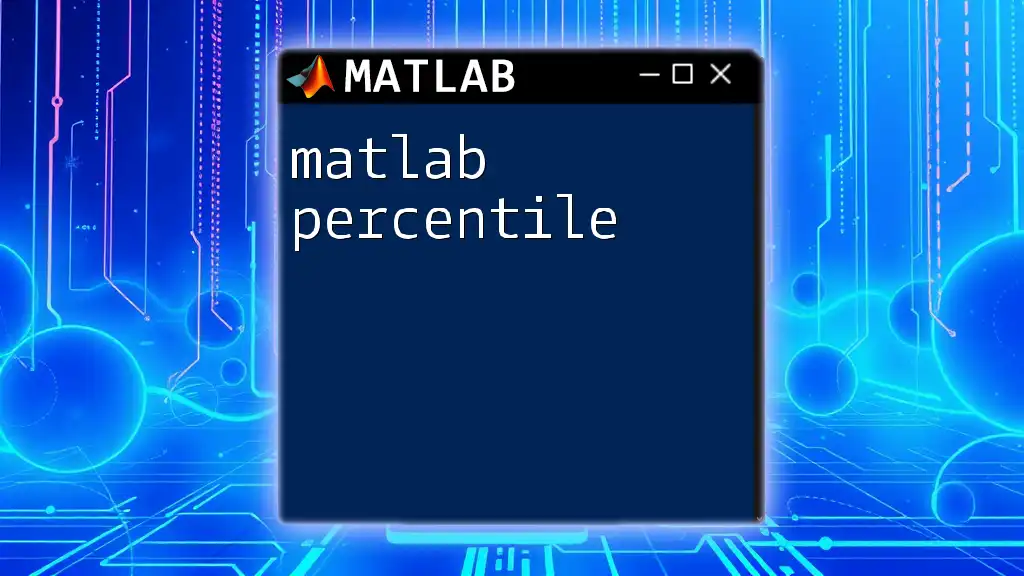
Conclusion
Understanding MATLAB Exponential functions opens up numerous possibilities in both theoretical and applied mathematics. By leveraging MATLAB's powerful built-in functions for computational tasks, you can effectively explore exponential growth and decay phenomena, both scalar and matrix-based.
Experimenting with the code snippets provided will deepen your grasp of how exponential functions operate within MATLAB and inspire further investigation into various applications.
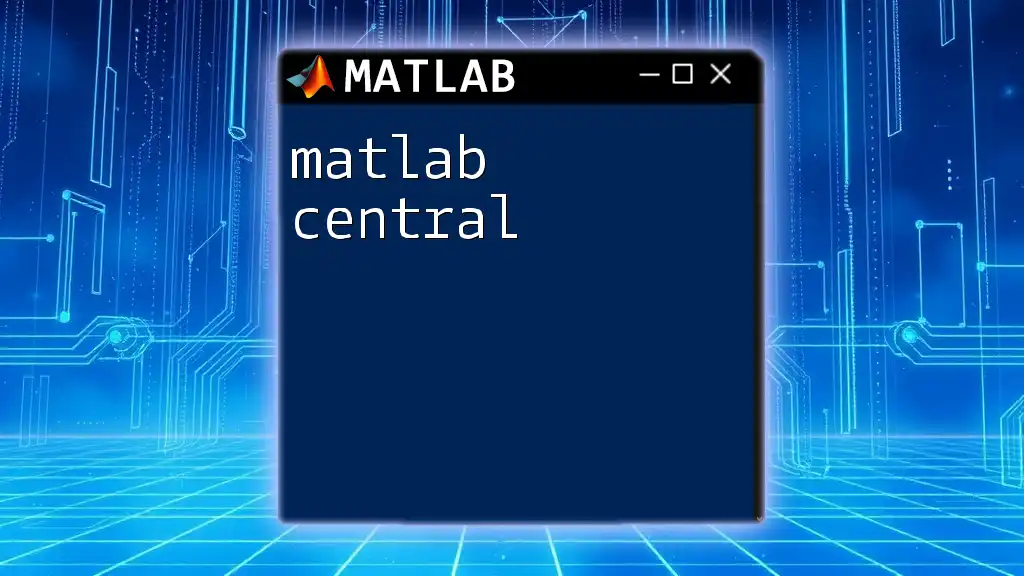
Additional Resources
For further insight, consult MATLAB’s official documentation, which is an invaluable resource for learning more about exponential functions and their applications. Books and articles focusing on advanced mathematics might also enhance your understanding of these concepts. Additionally, consider engaging in workshops or online classes to gain hands-on experience using MATLAB.
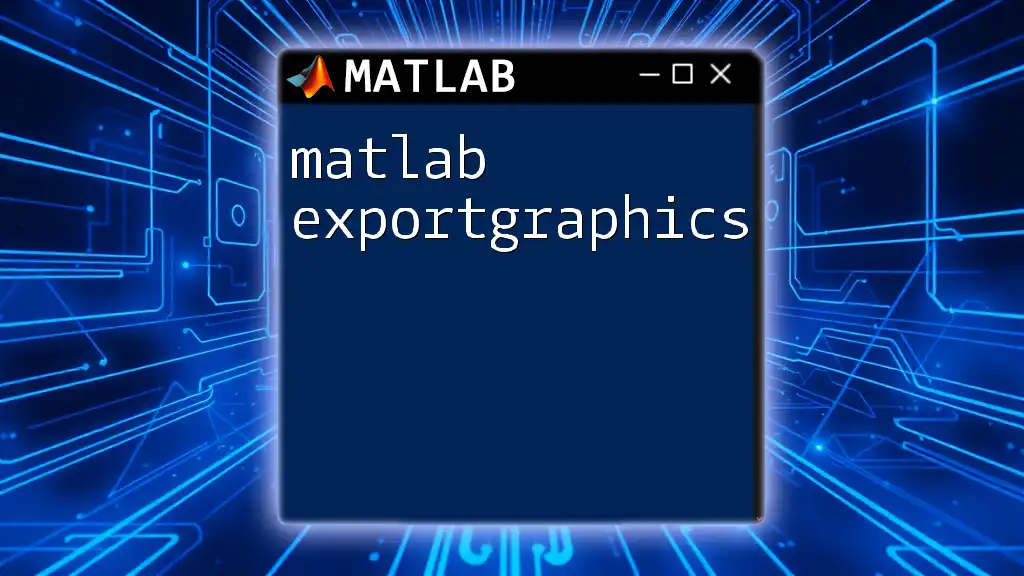
Call to Action
If you find this article helpful and want to dive deeper into concise and practical MATLAB tutorials, consider subscribing to our updates. Join our community forums to share your experiences and tips in mastering MATLAB!