In MATLAB, a list can be represented as a cell array that allows you to store elements of different types, enabling flexibility in data management.
myList = {1, 'text', [3, 4, 5], true}; % Creating a cell array (list) with mixed data types
Understanding Lists in MATLAB
What is a List?
A list in the context of MATLAB is a flexible way to store a collection of items, which can vary in type and size. Lists are particularly useful for organizing and manipulating data. In MATLAB, lists often refer to three main structures: cell arrays, struct arrays, and tables. These structures provide the advantage of handling heterogeneous data, making them essential for many computational tasks.
Types of Lists in MATLAB
Cell Arrays
Cell arrays are one of the most flexible and dynamic data types in MATLAB. They allow for the storage of different types of data within a single array. This means one cell can contain a number, while another might contain a string or an array.
Key features of cell arrays include:
- Heterogeneous data types: You can mix different types of data.
- Flexible dimensions: They can be multidimensional.
An example of creating a cell array is as follows:
myCellArray = {1, 'text', [1, 2, 3]; 'another', 2.5, true};
In this example, the first element is a number, the second is a string, and the third is an array.
Struct Arrays
Struct arrays consist of a set of structures, each containing multiple fields. This is particularly useful when you have related data entries. Each structure can hold different data types, making it easier to manage complex datasets.
To create and access data in struct arrays, you can use the following code snippets:
student(1).name = 'Alice'; student(1).age = 21;
student(2).name = 'Bob'; student(2).age = 22;
In this example, we create a struct array called `student`, where each entry contains `name` and `age` fields for different students.
Tables
Tables are another highly advantageous format in MATLAB for organizing data. Tables are particularly suited for data analysis as they allow for easy manipulation of rows and columns. Unlike traditional arrays, tables also support variables of different types.
Creating a table is straightforward:
T = table({'Alice'; 'Bob'}, [21; 22], 'VariableNames', {'Name', 'Age'});
This creates a table `T` with two columns labeled `Name` and `Age`, making it intuitive for users familiar with databases and spreadsheet software.
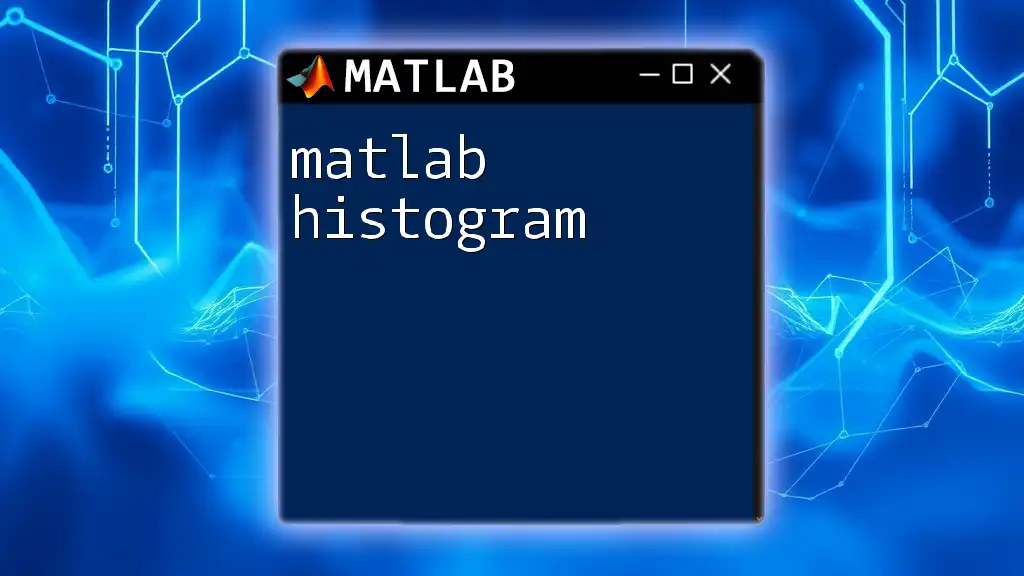
Creating and Modifying Lists
Creating Lists in MATLAB
Creating lists in MATLAB is simple due to its intuitive syntax. For cell arrays, you use curly braces `{}`; for struct arrays, you define the structure fields; and for tables, you use the `table` function. Always consider the type of data and its organization when you create lists. Proper planning can save you from complications later on.
Modifying Existing Lists
Once lists are created, MATLAB provides several built-in functions to modify them. You can append, remove, or change elements effectively.
For instance, modifying a cell array can be done as follows:
myCellArray{1, 2} = 'modified text'; % Modify an existing entry
For a struct array:
student(1).age = 22; % Changing age of the first student
And for tables:
T.Age(2) = 23; % Modifying the age of Bob
By understanding these methods, you can efficiently manage your data structures throughout your MATLAB projects.
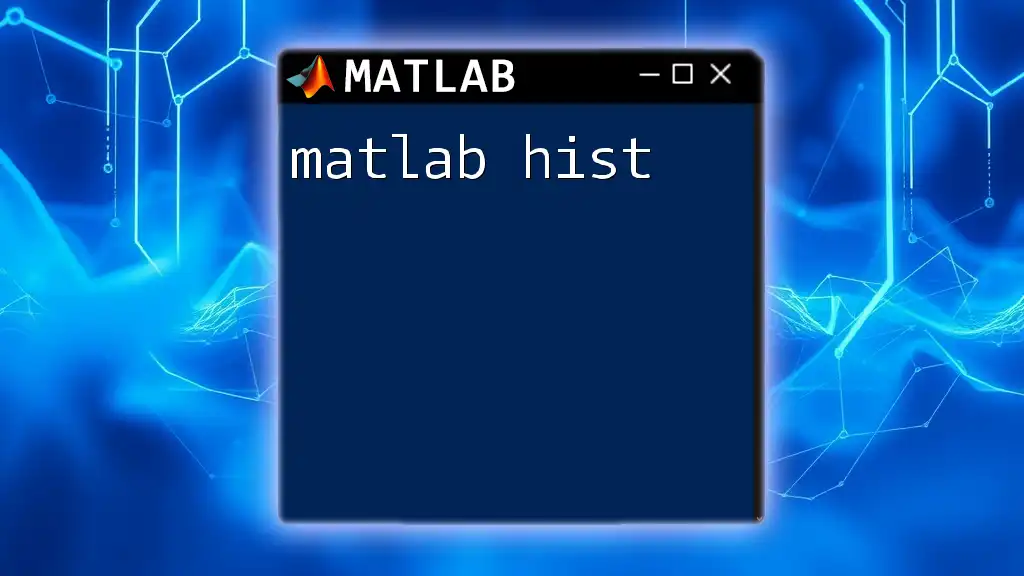
Accessing List Elements
Indexing Techniques
MATLAB allows for various indexing techniques to access list elements. You can use linear indexing for accessing elements in arrays or logical indexing for conditional access to elements.
Here are examples for each type of list:
-
Cell Array Element Access:
myCellArray{1} % Access first element
-
Struct Array Access:
student(1).name % Access name of the first student
-
Table Data Access:
T.Name{2} % Access name in table
Best Practices for Accessing Elements
While indexing is powerful, being aware of potential pitfalls is crucial. For instance, ensure that you do not exceed the dimensions of your list to prevent runtime errors. Understand the size and type of your lists before attempting complex indexing operations.
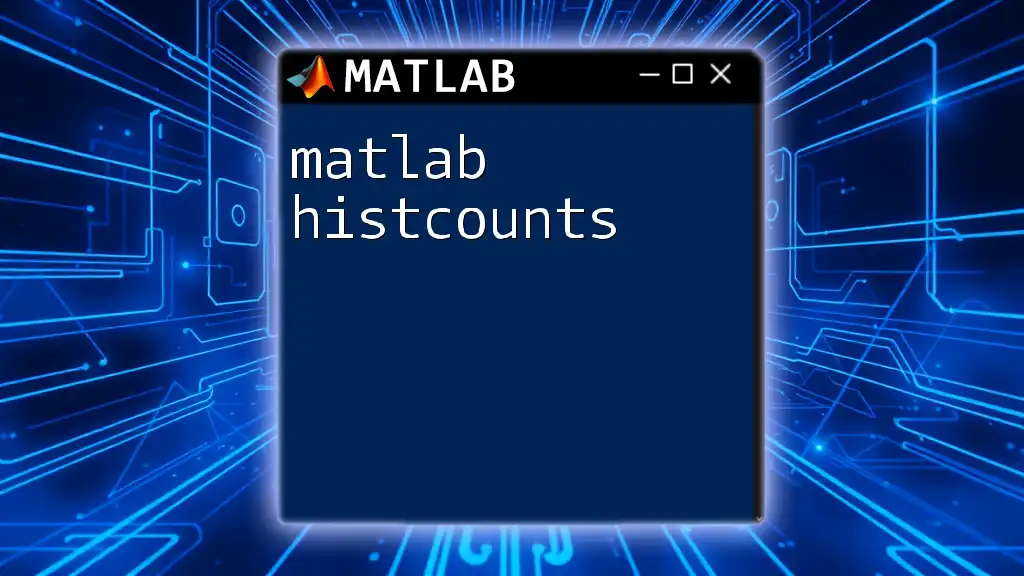
Common Functions for List Operations
Built-in Functions for Lists
MATLAB has robust built-in functions that help you manipulate lists effectively. Functions like `cellfun`, `structfun`, and `table2array` can streamline operations on lists.
For example:
-
Using `cellfun` for applying a function to each cell of a cell array:
lengths = cellfun(@length, myCellArray); % Calculate the length of each cell entry
-
Using `structfun` to apply a function to each field of a struct:
ages = structfun(@num2str, student, 'UniformOutput', false); % Convert ages to strings
-
Using `table2array` to convert a table column to an array:
ageArray = table2array(T(:, 'Age')); % Extract Age column as an array
Custom Functions for Lists
Creating custom functions to operate on lists enhances productivity. Writing a function allows you to encapsulate frequently used operations.
Here is a simple custom function to extract names from a struct array:
function output = getNames(studentStruct)
output = {studentStruct.name}; % Extract names into a cell array
end
This function can be called with the `student` struct array to easily gather names into a single variable.
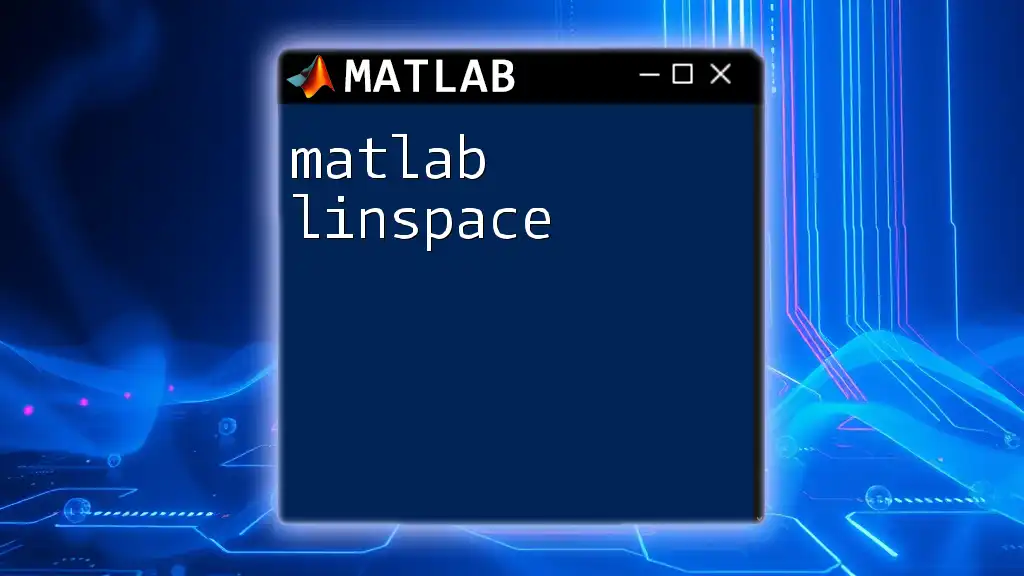
Practical Applications of Lists
Data Organization and Analysis
Lists in MATLAB facilitate better data organization, especially when dealing with large datasets. With the ability to store mixed types (numbers, strings, matrices), lists serve as the backbone for data analysis tasks. You can group related information in struct arrays or analyze datasets efficiently using tables.
Real-World Examples
There are countless scenarios where MATLAB lists shine. For example, in engineering, cell arrays might be used to store results from various simulations, while in finance, tables can organize stock data, despite differing entry types.
Using lists not only improves data management but also contributes to more efficient coding and analysis, allowing professionals to focus on more complex tasks.
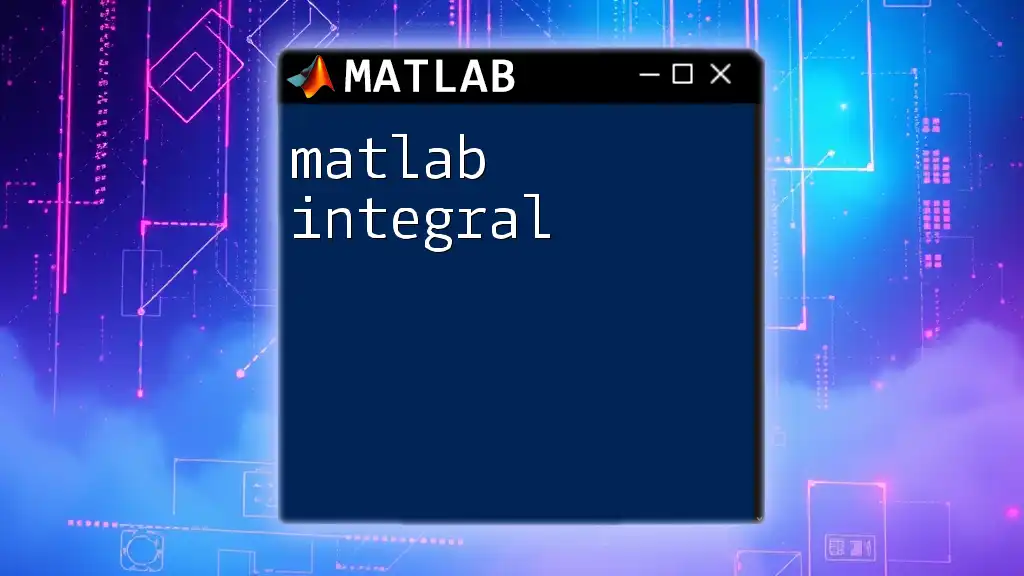
Conclusion
In this guide, we explored the various types of lists available in MATLAB, from cell arrays to struct arrays and tables. We covered how to create, modify, and access these lists, as well as practical applications in real-world scenarios. Understanding how to effectively use lists in MATLAB will empower you to tackle complex data analysis tasks with ease.
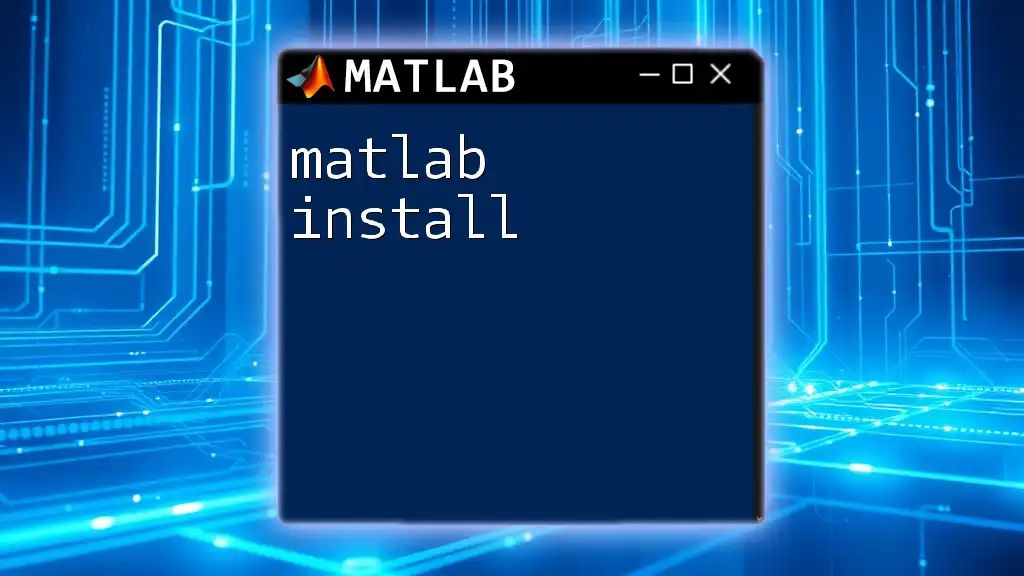
Additional Resources
For further exploration, check out the official MATLAB documentation on cell arrays, struct arrays, and tables. Additionally, consider engaging with books and online courses to deepen your understanding of MATLAB programming.
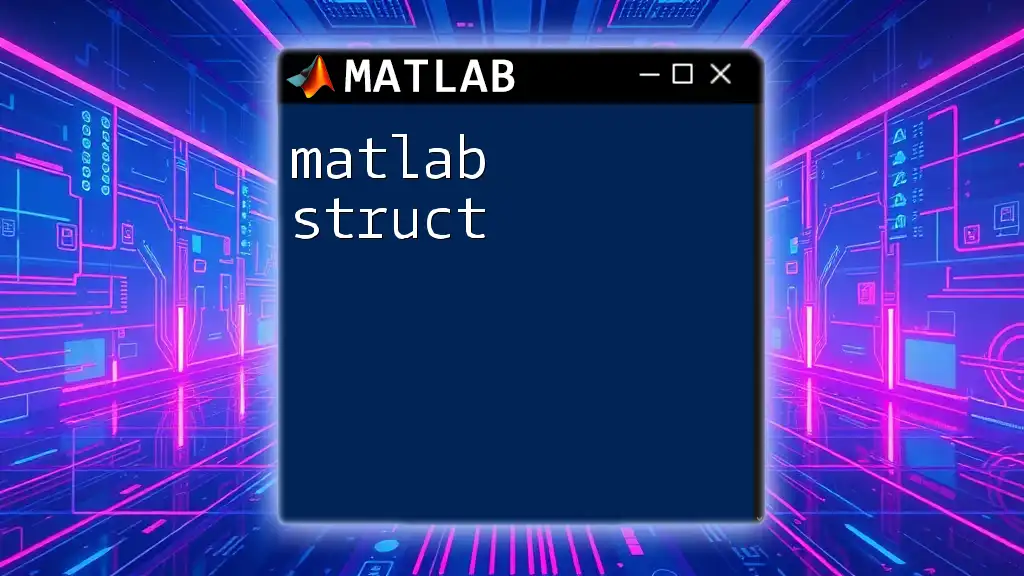
Call to Action
Start experimenting with MATLAB lists today. Create your own cell arrays, struct arrays, and tables, and share your experiences or questions in the comments. Engaging in practical exercises is the best way to master MATLAB!