To find the index of a specific value in an array in MATLAB, you can use the `find` function, which returns the indices of array elements that meet a certain condition.
Here's an example code snippet:
% Example: Find the index of value 5 in an array
A = [1, 2, 5, 4, 5];
indices = find(A == 5);
Understanding Arrays in MATLAB
What is an Array?
In MATLAB, an array is a collection of elements organized in a specific structure. You can think of it as a grid or a table that holds multiple values. MATLAB supports different types of arrays, including 1D arrays (also known as vectors), 2D arrays (or matrices), and multi-dimensional arrays. Arrays are essential because they allow you to store large amounts of data efficiently and manipulate that data using powerful matrix operations.
Basic Array Operations
Before delving into methods for finding indices, it is crucial to understand basic array operations. You can create arrays using square brackets, and they can be constructed by:
- Manually specifying values.
- Using built-in functions like `linspace` or `zeros`.
For example, a simple array can be created like this:
array = [1, 2, 3, 4, 5];
Once you have created an array, you can perform several operations, such as reshaping, concatenating, and indexing, which lays the groundwork for using indexing functions like `find`.
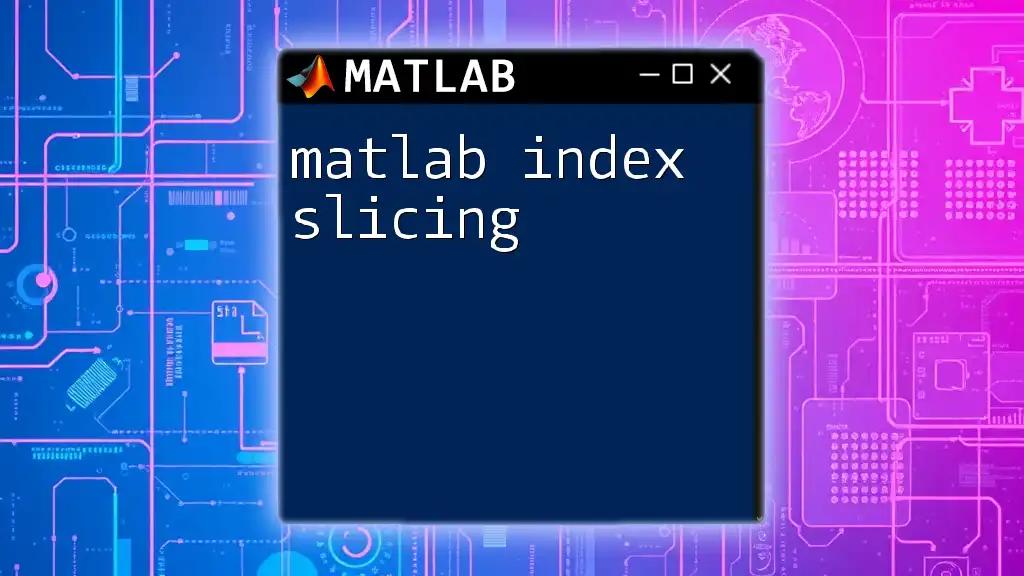
Using the `find` Function
Overview of the `find` Function
The `find` function in MATLAB is a powerful tool to locate the indices of elements that meet specific criteria within an array. Its basic syntax allows you to retrieve the indices of values that either equal a specified value or satisfy a condition.
Basic Syntax
The syntax of the `find` function is straightforward and as follows:
index = find(array == value);
Here, `array` is the input from which you want to find the indices, and `value` is the specific value you’re looking for in that array.
Example 1: Finding a Single Value
Suppose you have an array of integers and you want to find the index of the value 7:
array = [3, 5, 7, 9, 11];
value = 7;
index = find(array == value);
disp(index);
In this case, the output will be `3`, indicating that the value 7 is located at the third position of the array.
Example 2: Finding Multiple Occurrences
If the value appears multiple times in the array, `find` efficiently returns all the indices where the value occurs. Consider the following example:
array = [4, 7, 4, 8, 4];
value = 4;
indices = find(array == value);
disp(indices);
The displayed output will be `1 3 5`, showing that the value 4 appears at the first, third, and fifth indices of the array.
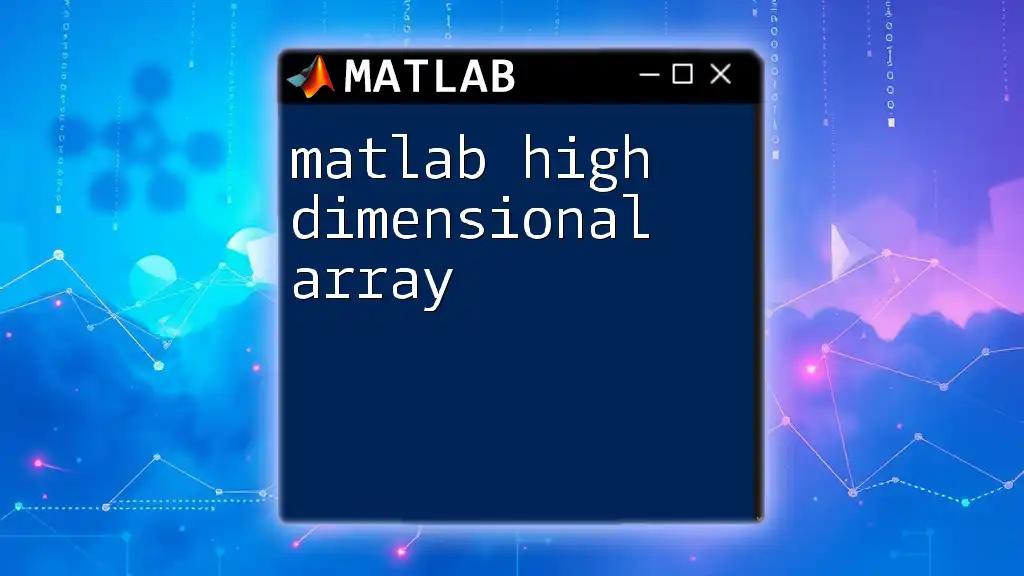
Advanced Usage of the `find` Function
Finding Indices Based on Conditions
Using the `find` function allows you to locate elements based on logical conditions. You can define a condition to identify indices of elements that meet specific criteria.
For instance, if you want to find indices of elements greater than 15 in an array, the code would be:
array = [12, 15, 20, 25];
condition = array > 15;
indices = find(condition);
disp(indices);
The output here will yield `3 4`, indicating that the values 20 and 25 are located at the third and fourth positions, respectively.
Using `find` with Multi-dimensional Arrays
Finding Indices in 2D Arrays
In MATLAB, you can also work with multi-dimensional arrays, particularly 2D arrays (matrices). The `find` function can be applied to these matrices to locate values both in terms of row and column indices.
Consider a matrix and the need to find the index of the value 5:
matrix = [1, 2, 3; 4, 5, 6];
value = 5;
[row, col] = find(matrix == value);
disp([row, col]);
The output here will be `2 2`, indicating that the value 5 is in the second row and second column of the matrix.
Handling Empty Results
It is crucial to handle cases where the specified value is not found within an array. Using the `find` function, if there are no occurrences of the value, it returns an empty array.
For example:
array = [1, 2, 3];
value = 10;
index = find(array == value);
if isempty(index)
disp('Value not found!');
end
The output of this snippet will be `Value not found!` This checks for an empty return and handles it gracefully, which is essential for robust programming.
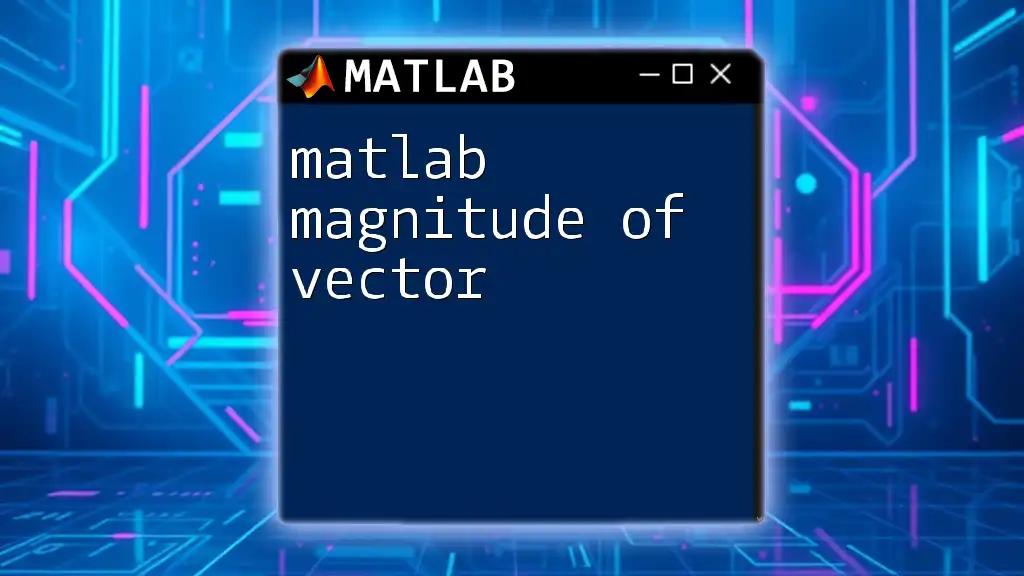
Alternative Methods to Find Indices
Using `ismember`
Another method to locate indices of a specific value within an array is using the `ismember` function, which checks for membership between two arrays. Here's how you can use it:
array = [1, 2, 3, 4];
value = 3;
index = find(ismember(array, value));
disp(index);
This will return `3`, indicating the position of value 3 within the original array. Utilizing `ismember` can be particularly advantageous when comparing two arrays since it also handles multiple occurrences naturally.
Using Logical Indexing
Logical indexing is a powerful feature in MATLAB that allows you to index an array based on a logical condition directly. Here's a great example of using logical indexing:
array = [10, 20, 30, 40, 50];
value = 30;
index = array == value; % Logical index
disp(find(index));
This method offers concise syntax and can be easier to read. While it serves a similar purpose to `find`, it highlights an alternative way to extract indices and helps you to write more expressive code.
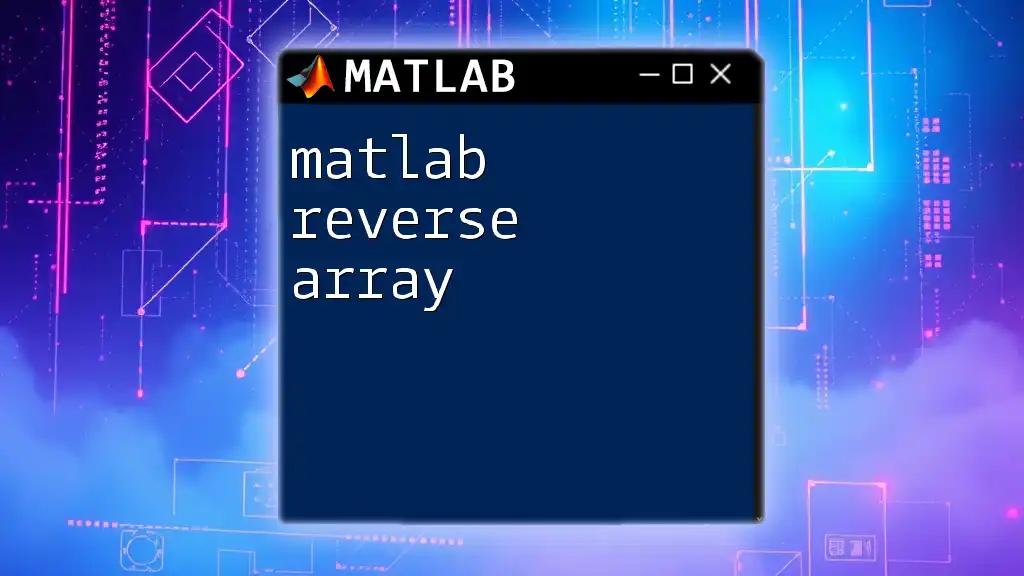
Common Pitfalls and Best Practices
Typical Mistakes When Using `find`
Some common mistakes while using the `find` function could include:
- Comparing incompatible types, such as trying to compare a string with a numeric array.
- Not accounting for empty results, leading to errors in further computations.
Always ensure that you check the dimensions of your arrays and handle potential empty results gracefully to avoid runtime errors.
Best Practices
To maximize the efficiency and readability of your MATLAB code while working with arrays:
- Employ clear variable naming to indicate what each array or value represents.
- Comment your code adequately to assist others (or your future self) in understanding your logic.
- Utilize vectorized operations whenever possible, as they are typically faster than using loops in MATLAB.
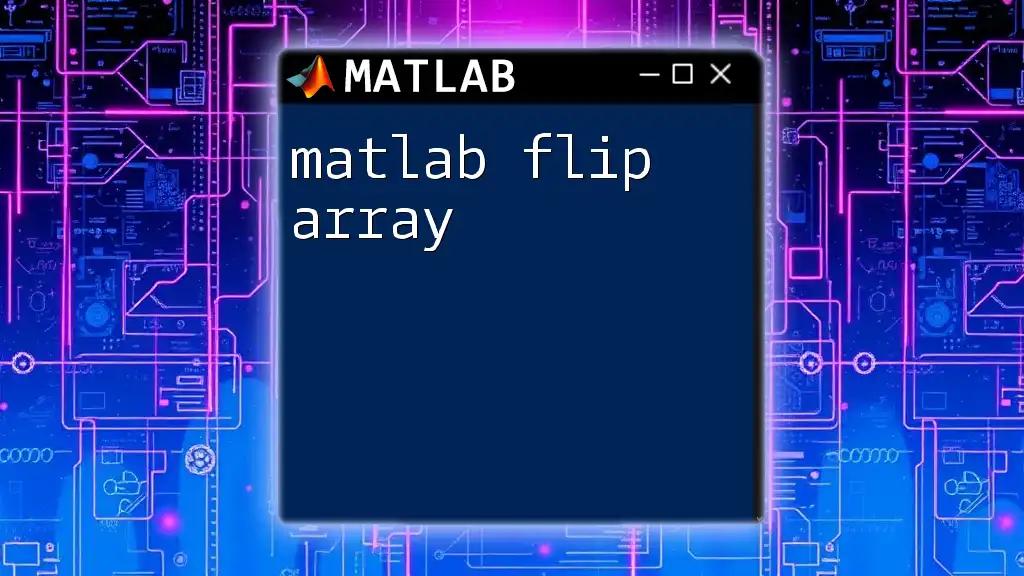
Conclusion
Finding the index of a value in an array is a critical skill when using MATLAB, especially for tasks involving data analysis and manipulation. By mastering the `find` function, along with alternative methods such as `ismember` and logical indexing, you can navigate and manipulate arrays with ease. Practice using various examples to become proficient, and leverage the additional resources available for continued learning on MATLAB commands. Each method has its strengths and it's essential to explore different approaches to determine which is best suited for your specific programming needs.