In MATLAB, the length of an array can be determined using the `length` function, which returns the size of the largest dimension of the array.
A = [1, 2, 3, 4, 5];
len = length(A); % len will be 5
Understanding Array Length
What Does Length Mean in Arrays?
In MATLAB, the concept of "length" refers to the number of elements in a specific array. Understanding the length of an array is essential for numerous computational tasks, such as data analysis and algorithm implementation. The length can vary depending on the type of array, which can include vectors, matrices, and multidimensional arrays.
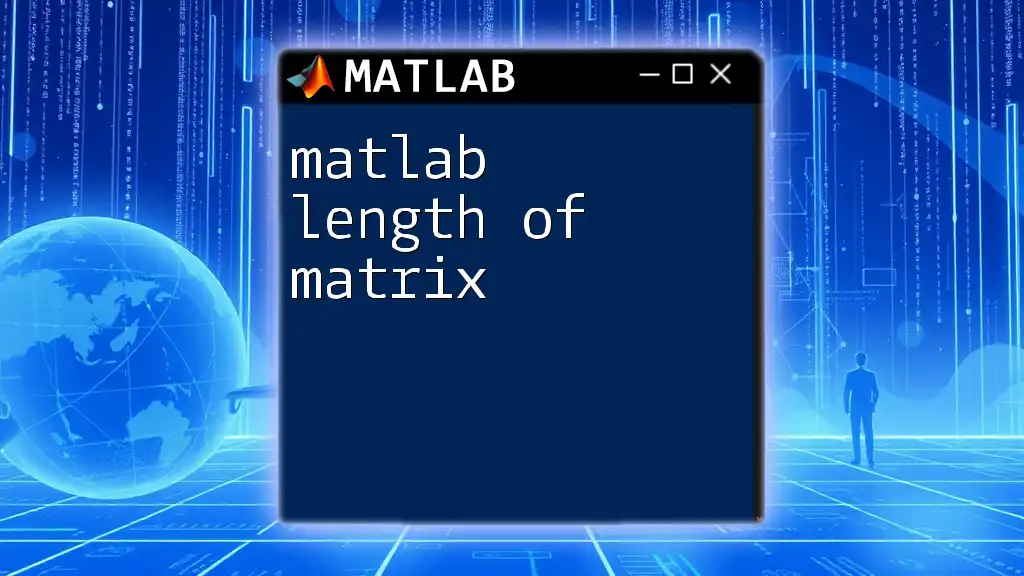
Using the `length` Function
The Basics of the `length` Function
The `length` function is designed to retrieve the length of an array efficiently. The general syntax for the function is:
length(array)
This function is particularly useful because it simplifies the process of determining how many elements are in a given array.
Example of Using `length`
Consider the following example, where we define a simple vector and use the `length` function:
myArray = [1, 2, 3, 4, 5];
len = length(myArray);
disp(['The length of the array is: ' num2str(len)]);
The output would be `The length of the array is: 5`, showing that there are five elements in the array `myArray`.
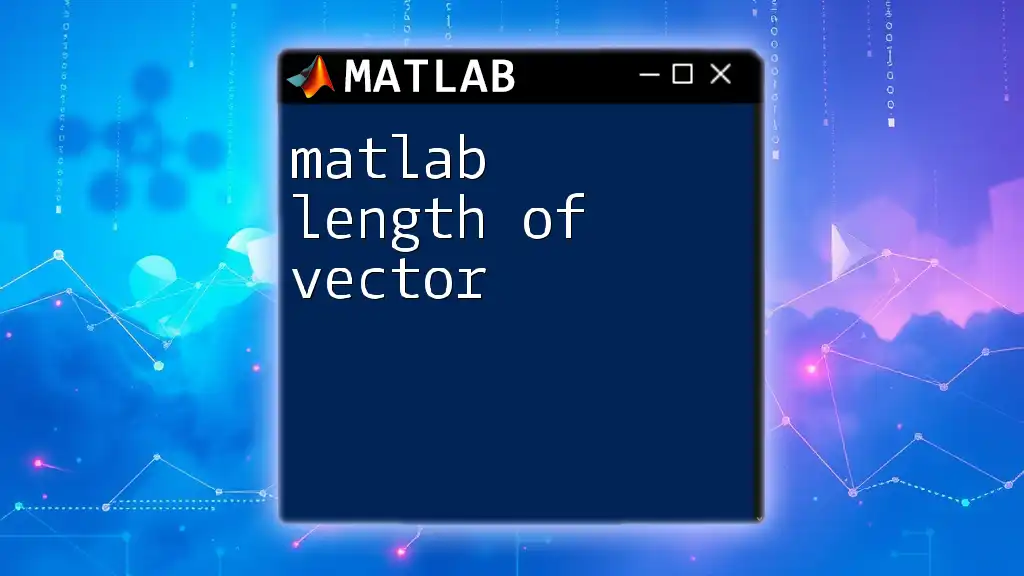
Alternative Ways to Determine Length
Using the `size` Function
The `size` function provides an alternative method for assessing the dimensions of an array. This function returns two values: the number of rows and the number of columns in a matrix or the dimensions of a multidimensional array. The syntax for the `size` function is as follows:
size(array)
While `length` returns the largest dimension, `size` provides more granular detail.
Example Usage
For instance, if we define a matrix, we can find its size:
myMatrix = [1, 2; 3, 4; 5, 6];
[rows, cols] = size(myMatrix);
disp(['Number of rows: ' num2str(rows) ', Number of columns: ' num2str(cols)]);
The output here will indicate `Number of rows: 5, Number of columns: 2`, offering clarity on the structure of the `myMatrix`.
Using the `numel` Function
Another useful function for determining the total number of elements in an array is `numel`. This function counts all the elements in an array, regardless of its dimensionality. The syntax is:
numel(array)
When to Use `numel` Instead of `length`
While `length` is limited to returning the largest dimension, `numel` provides a comprehensive count of all elements. This distinction is critical, especially with multidimensional arrays.
Code Example
Here’s how you would use `numel`:
myVector = [1, 2, 3, 4];
elements = numel(myVector);
disp(['Total elements in the vector: ' num2str(elements)]);
This will output `Total elements in the vector: 4`, effectively showcasing the total count in `myVector`.
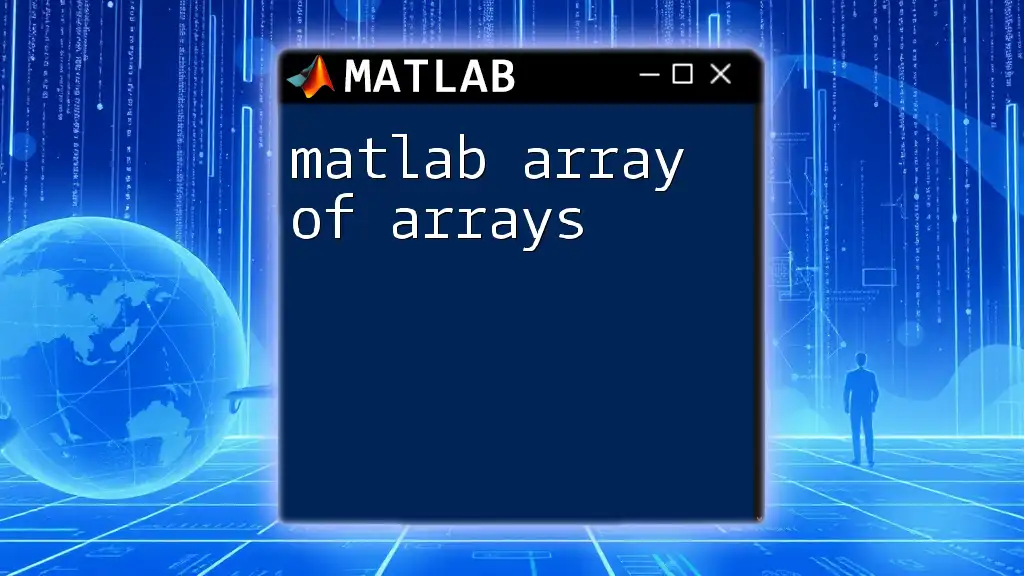
Length of Different Array Types
Vectors and Their Length
In MATLAB, the calculation of length is straightforward for both row and column vectors. The `length` function returns the number of elements in either form.
Sample Code and Output
For a demonstration:
rowVector = [1, 2, 3];
colVector = [1; 2; 3];
disp(['Row vector length: ' num2str(length(rowVector))]);
disp(['Column vector length: ' num2str(length(colVector))]);
The output will indicate:
Row vector length: 3
Column vector length: 3
This reveals that the length is determined solely by the number of elements.
Matrices and Their Length
When applied to two-dimensional matrices, the `length` function provides the size of the longest dimension, which may not divide the total elements cleanly into rows and columns.
Example Code
Consider the following matrix:
myMatrix = [1, 2; 3, 4; 5, 6];
disp(['Length of the matrix: ' num2str(length(myMatrix))]);
Output will be `Length of the matrix: 6`, since it accounts for the maximum length, which in this case corresponds to the total number of elements.
Multidimensional Arrays
When working with three-dimensional arrays or higher, the `length` function continues to return the length of the largest dimension.
Code Example
Here’s how you could check the length of a 3D array:
my3DArray = rand(3, 4, 5); % Creates a random 3D array
disp(['Length of the 3D array: ' num2str(length(my3DArray))]);
The output reflects that all 60 elements are accounted for, returning the largest dimension's length result.
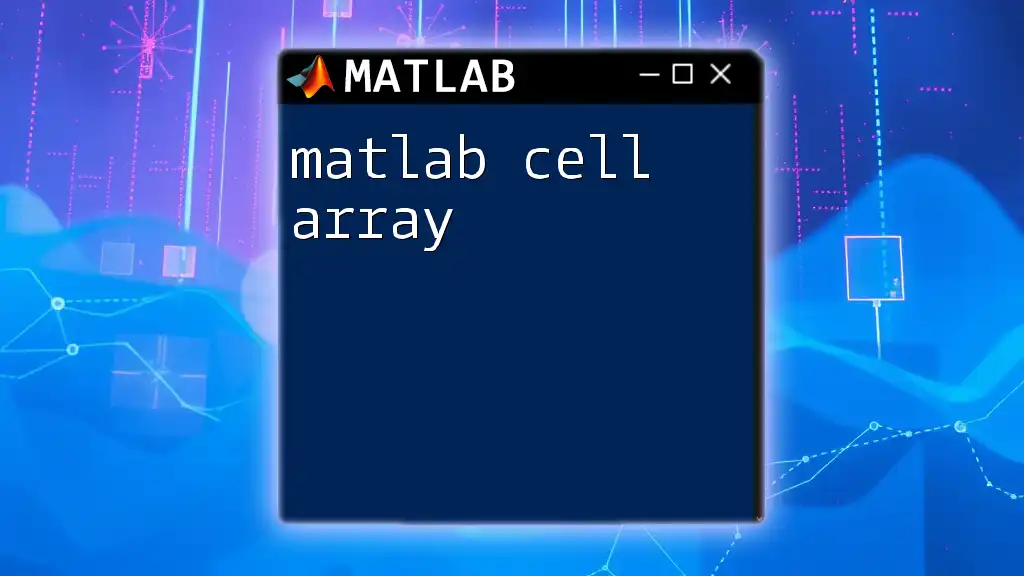
Best Practices and Tips
Common Mistakes to Avoid
A prevalent mistake is misunderstanding the value returned by `length`. Many beginners assume that `length` provides the total number of elements in all cases, whereas it only gives the largest dimension.
Performance Considerations
When optimizing code, it's wise to be thoughtful about which function to choose. Using `length` is very efficient for 1D arrays, while `numel` is more suitable for aggregating overall element counts in multidimensional datasets.
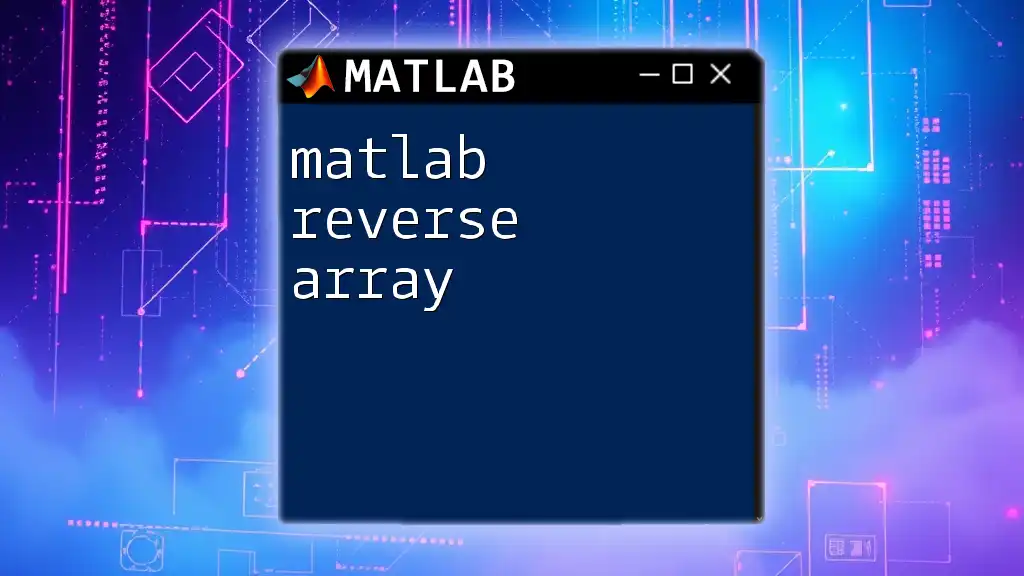
Conclusion
Understanding the various methods for obtaining the MATLAB length of an array is foundational for anyone working with data in MATLAB. From utilizing the `length` function to exploring alternatives like `size` and `numel`, mastering these commands will significantly enhance your programming efficiency.
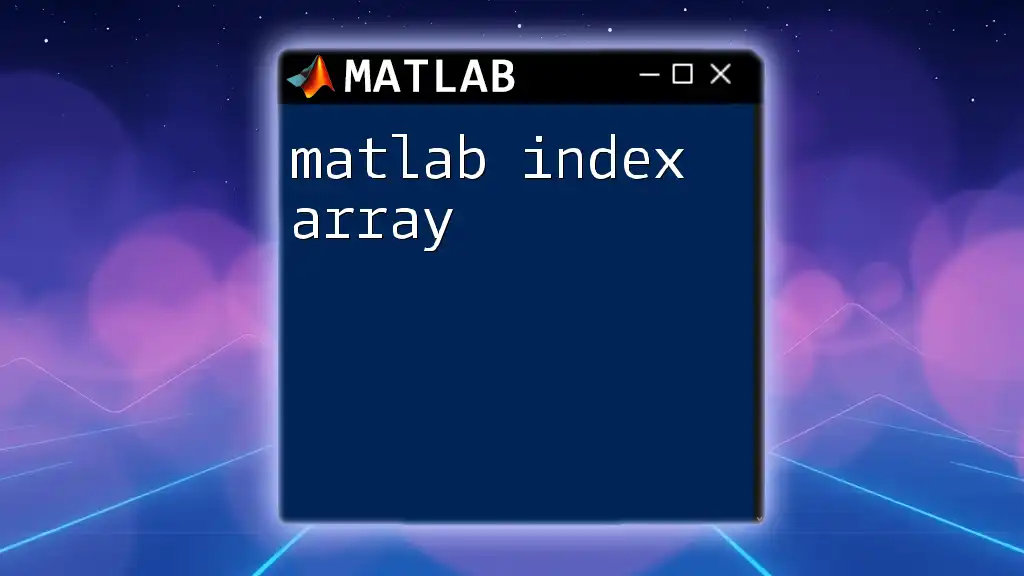
Additional Resources
For those looking for deeper insights into these functions, consider referring to the official MATLAB documentation, which offers extensive guidance and examples. Additionally, practicing with different types of arrays will solidify your understanding and proficiency in employing these commands effectively.