In MATLAB, a high-dimensional array is an array that contains more than two dimensions, allowing for complex data structures and operations on multi-dimensional datasets.
Here's a simple code snippet to create a 3D array and access its elements:
% Create a 3D array of size 3x4x2
A = rand(3, 4, 2);
% Access the element at row 2, column 3, depth 1
element = A(2, 3, 1);
What are High Dimensional Arrays?
High dimensional arrays are fundamental data structures in MATLAB, allowing users to store and manipulate data across multiple dimensions. An array with more than two dimensions (e.g., three or four) is considered a high dimensional array. These structures are incredibly useful for various applications, such as image processing, scientific computing, and simulations, as they allow for complex data representation that captures relationships between multiple variables.
In MATLAB, dimensions can be thought of as the "size" of the array in various directions. A one-dimensional array could represent a list of numbers, while a two-dimensional array (or matrix) might represent a table of data. When we move to three dimensions or higher, the complexity of data representation increases. High dimensional arrays can represent everything from video data (where each frame can be seen as a 2D array over time) to multi-channel sensor data.
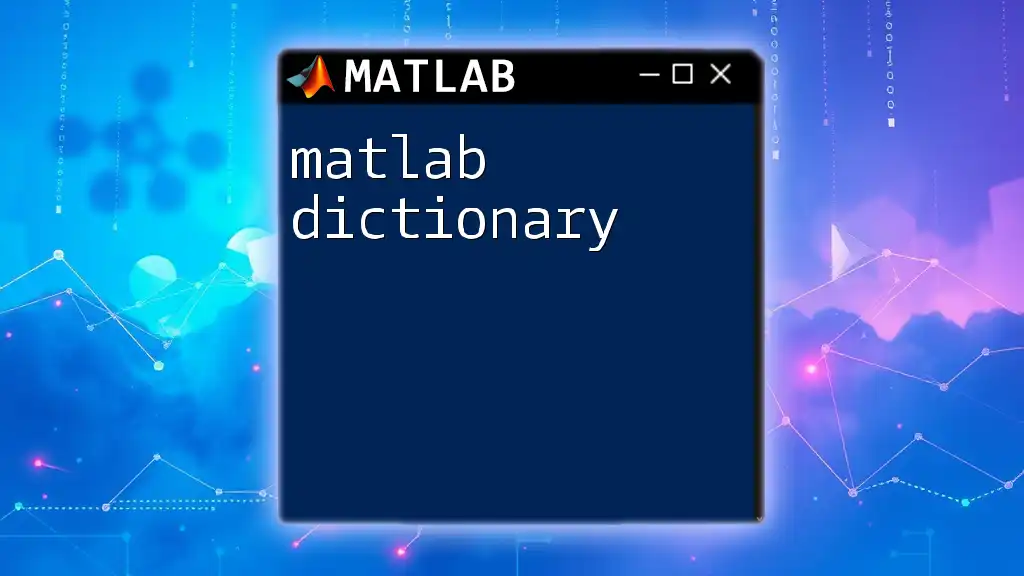
Creating High Dimensional Arrays
Using Built-in Functions
MATLAB provides a variety of built-in functions to create high dimensional arrays with minimal effort.
- `zeros`: Creates an array filled with zeros.
- `ones`: Generates an array filled with ones.
- `rand`: Generates an array with random values.
For example, to create a simple 3D array filled with ones, you can use:
A = ones(3,3,3); % Creates a 3x3x3 array filled with ones
This single line of code generates a uniform 3D matrix where each element is 1. Understanding the properties of the created array, such as its dimensions and data type, is crucial because these properties inform the operations and manipulations you can perform on the data.
Using the `reshape` Function
The `reshape` function is highly versatile, allowing users to convert matrices into different dimensions as required. This function enables you to take a 1D vector and reshape it into any dimensional format you need.
For instance, consider reshaping a vector containing numbers 1 through 27 into a 3D array:
B = reshape(1:27, [3, 3, 3]); % Reshape a 1D array to a 3D array
Here, a 1D array containing integers from 1 to 27 is transformed into a 3D matrix of size 3x3x3. The ability to reshape arrays is particularly useful in data analysis scenarios where new dimensions may be required for operations or visualizations.
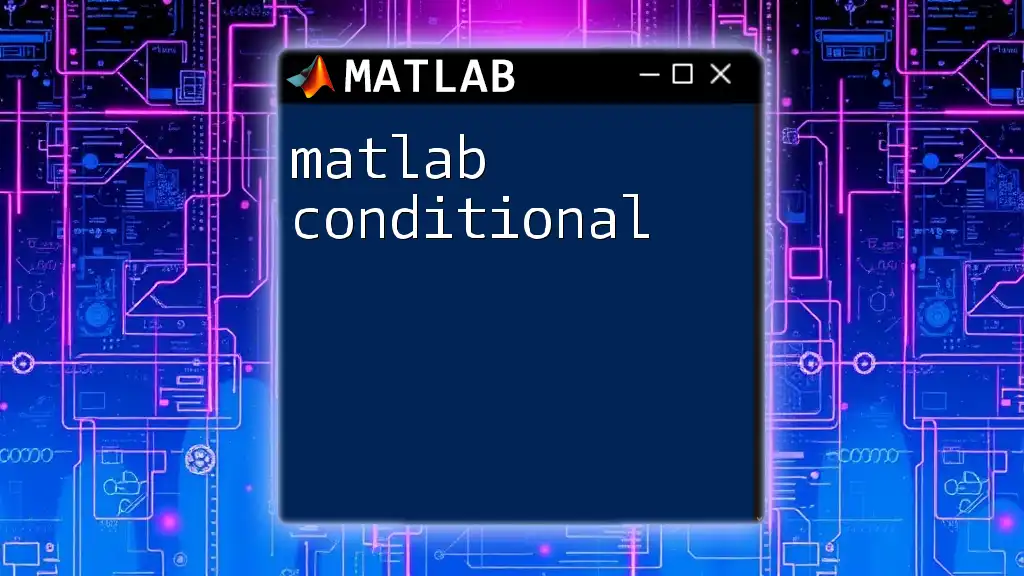
Indexing and Accessing Elements
Linear Indexing in Multi-Dimensional Arrays
One of the powerful features of MATLAB is its linear indexing capability, allowing users to access elements in high dimensional arrays using a single index.
For example, given a 4x4x4 random array:
C = rand(4,4,4);
elem = C(10); % Accessing the 10th element
In this code, `elem` retrieves the 10th element of the 3D array `C`. When using linear indexing, MATLAB treats the array as a single column vector, simplifying the access of specific elements without needing to reference multiple dimensions explicitly.
Logical Indexing Techniques
Logical indexing is a powerful feature that can simplify data manipulation within high dimensional arrays. By creating a logical array, you can easily find and manipulate elements that meet a specific condition.
For example, to find all elements greater than 0.5 in a 3D array:
D = rand(3,3,3);
idx = D > 0.5; % Logical index for elements greater than 0.5
In this example, `idx` generates a logical array with the same dimensions as `D`, where each position indicates whether the corresponding element in `D` satisfies the condition. This technique can greatly enhance data filtering and analysis processes.
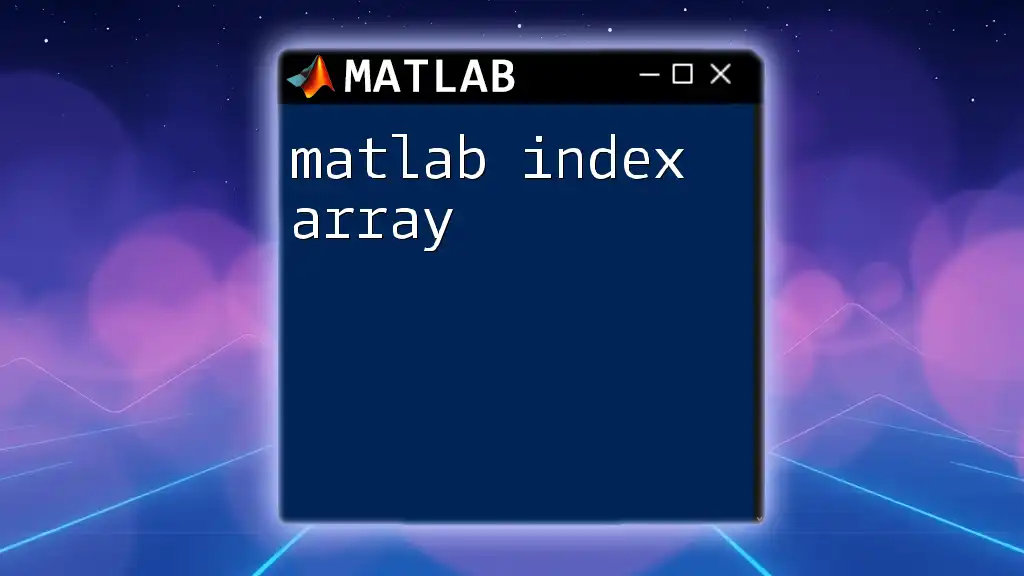
Slicing and Manipulating High Dimensional Arrays
Extracting Subarrays
Slicing allows users to extract specific portions or "slices" of high dimensional arrays. This method is especially useful when working with matrices that contain significant volumes of data across multiple dimensions.
To extract a 2D slice from a 3D array, you can specify the appropriate indices:
E = rand(4,4,4);
slice = E(:,:,2); % Extracting the 2nd layer from a 3D array
This line of code accesses the second layer of the 3D array `E`, providing a 4x4 matrix. Slicing can be utilized to focus on and manipulate specific subsets of data effectively.
Modifying Values
Manipulating high dimensional arrays is straightforward in MATLAB. You can modify specific elements, entire layers, or sections of a 3D array seamlessly.
For example, to change a specific element in the 3D array:
E(2,2,3) = 10; % Setting a specific element to 10
This line assigns the value `10` to the element located at the 2nd row, 2nd column, and 3rd layer of the 3D matrix `E`. Such modifications enable tailored data analysis and insights.
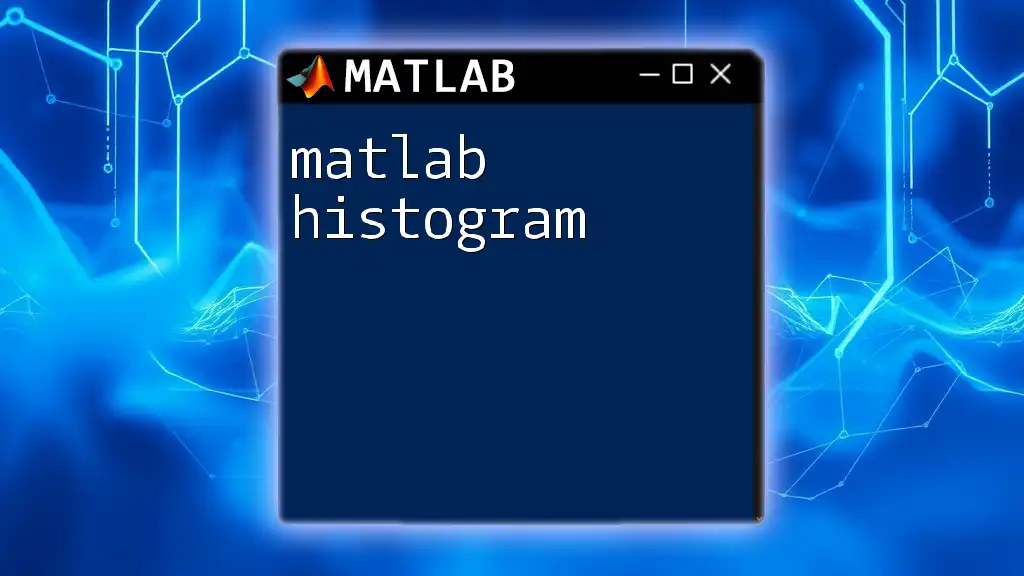
Mathematical Operations on High Dimensional Arrays
Element-wise Operations
MATLAB supports element-wise operations that can simplify calculations for high dimensional arrays. These operations include addition, subtraction, multiplication, and division performed at the individual element level.
For instance, to add a constant value to every element in a 3D array, you can use:
F = rand(2,2,2);
G = F + 5; % Adding 5 to all elements of a 3D array
This operation applies a uniform transformation across the entire array, easily adjusting values as needed for analysis.
Matrix Operations and Broadcasting
Broadcasting allows for the application of operations over arrays of different dimensions when appropriate. MATLAB automates this process to align and operate on these arrays efficiently.
For example, if you have a 2D matrix and a 3D array, you can perform matrix multiplication:
H = rand(2,3);
J = rand(3,2,2);
result = H * J; % Matrix multiplication across dimensions
The result of this code utilizes H's 2D structure and applies it across the higher-dimensional array J, producing insightful outcomes when analyzing combined datasets.
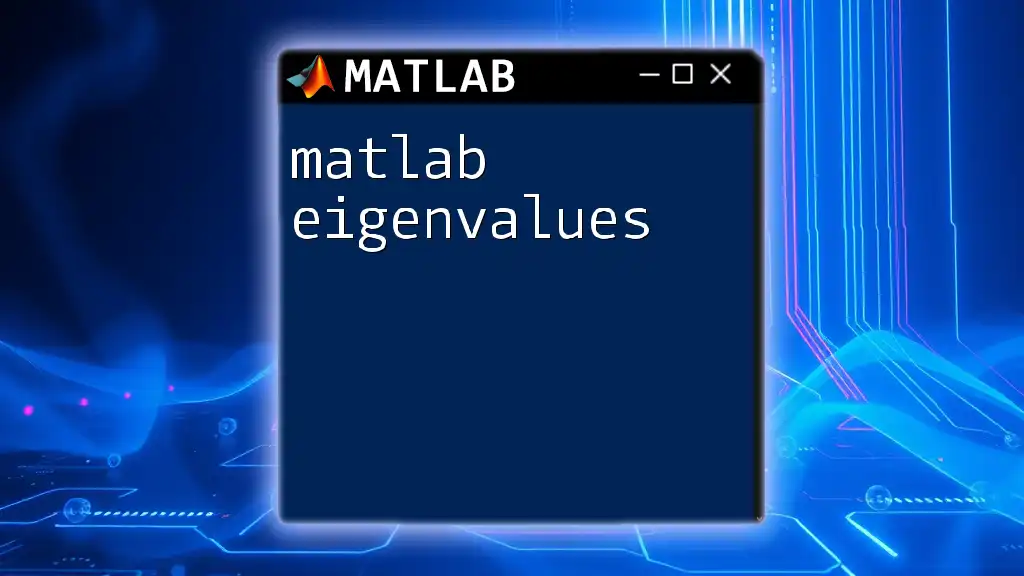
Applications of High Dimensional Arrays
Storing Image Data
High dimensional arrays are particularly prominent in the realm of image processing. Images can be represented as 3D arrays where each pixel's color is defined by three channels: red, green, and blue (RGB).
In this context, the three dimensions correspond to height, width, and color channels, allowing for comprehensive manipulation and analysis of visual data. MATLAB's image processing toolbox provides extensive functions aimed at manipulating image data stored in these arrays.
Working with Time-Series Data
High dimensional arrays are also crucial when handling complex datasets, such as multi-channel time series data. For example, consider multiple sensors collecting data over time across different locations. Each sensor’s reading can be represented as a separate "layer" in a 3D array, capturing diverse measurements for analysis.
By organizing these data points thoughtfully, it becomes easier to perform comparative analyses, detect patterns, and derive actionable insights across multiple dimensions.
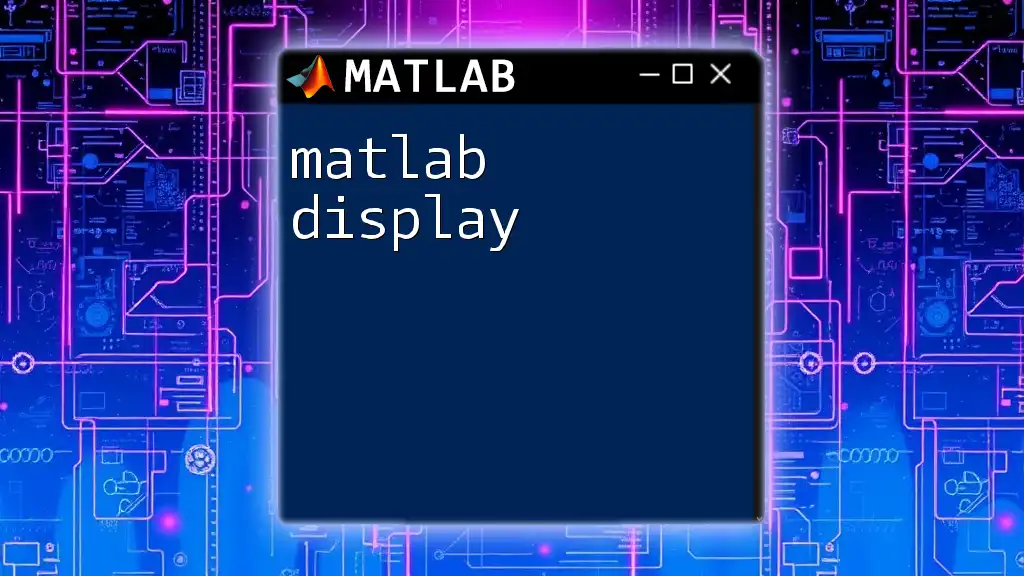
Best Practices for Working with High Dimensional Arrays
Optimizing Memory Usage
High dimensional arrays can consume significant amounts of memory, especially in large datasets. It's important to adopt strategies for optimizing memory usage, such as preallocating arrays, using the appropriate data type (e.g., single vs. double), and breaking down large arrays into manageable chunks when possible.
Effective Data Visualization
Visualizing results from high dimensional arrays can enhance understanding and decision-making. Techniques such as 2D slices, 3D surface plots, and heat maps facilitate the exploration of complex datasets. MATLAB offers numerous functions, such as `slice`, `surf`, and `imagesc`, to aid in effective data visualization.
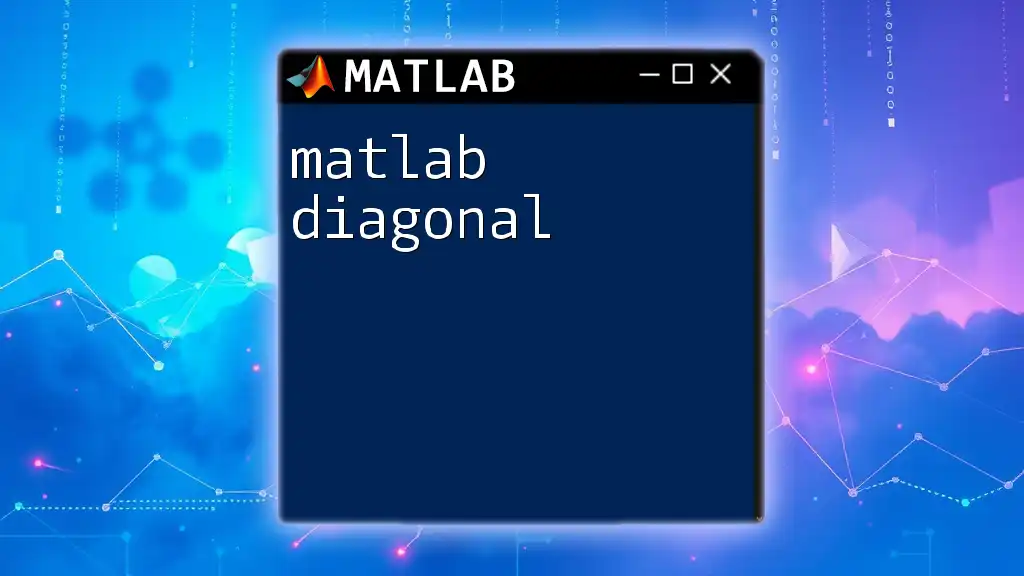
Conclusion
MATLAB high dimensional arrays present a powerful and versatile means to manage complex datasets, offering users the ability to create, manipulate, and analyze data in multiple dimensions. By mastering their construction, indexing, manipulation, and mathematical applications, users can unlock significant analytical capabilities that extend across various domains, from scientific research to engineering applications.
Further Resources
To deepen your understanding and skills with MATLAB high dimensional arrays, consider exploring recommended books, online tutorials, and courses dedicated to MATLAB programming. Additionally, engaging with community forums can provide valuable support and insight as you continue your learning journey.