The `flip` function in MATLAB reverses the order of elements in an array along a specified dimension, enabling quick manipulation of data orientation.
A = [1, 2, 3; 4, 5, 6];
B = flip(A); % Flips A upside down resulting in B = [4, 5, 6; 1, 2, 3]
Understanding MATLAB Arrays
What is an Array?
In MATLAB, an array is a fundamental data type that stores a collection of values, all of the same type. Arrays can be one-dimensional (1D), two-dimensional (2D), or even multi-dimensional, allowing for flexible and powerful data manipulation.
Why Use Arrays?
Arrays are crucial in various mathematical computations and data manipulations. They can represent everything from simple lists of numbers to complex matrices required in advanced statistical analysis, simulations, or even graphical representations.
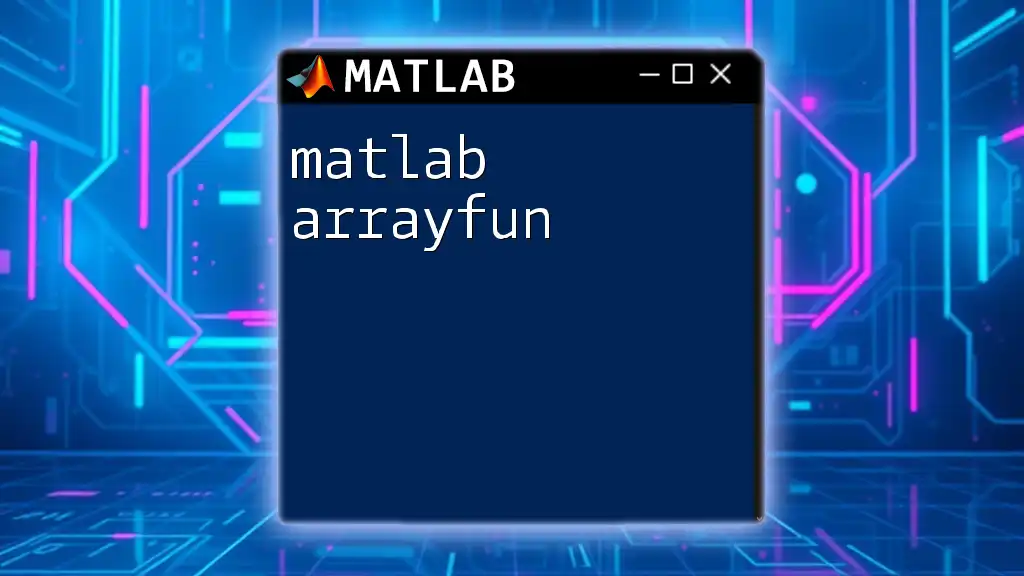
The Flip Array Command
What is the Flip Command?
The flip command in MATLAB is designed to invert the order of the elements in an array. This concept is significant because it allows for unique transformations of data, providing different perspectives on the same information. Flipping differs from other array operations, such as rotation or shifting, by strictly reversing the order of elements without altering their values.
Key Functions for Flipping Arrays
flip
The primary function for flipping arrays is the `flip` function. This command takes an array and reverses the order of its elements along a specified dimension.
Syntax:
B = flip(A)
B = flip(A, dim)
- A is the input array.
- B is the output array.
- dim is the dimension along which to flip the array (optional).
Example of usage:
A = [1, 2, 3; 4, 5, 6];
B = flip(A);
% B will be [4, 5, 6; 1, 2, 3]
flipud
The `flipud` function specifically flips an array upside down. This means that the first row becomes the last row and so on.
Syntax:
B = flipud(A)
Example of usage:
C = flipud(A);
% C will be [4, 5, 6; 1, 2, 3]
fliplr
Conversely, the `fliplr` function flips the array from left to right, reversing the order of columns.
Syntax:
B = fliplr(A)
Example of usage:
D = fliplr(A);
% D will be [3, 2, 1; 6, 5, 4]
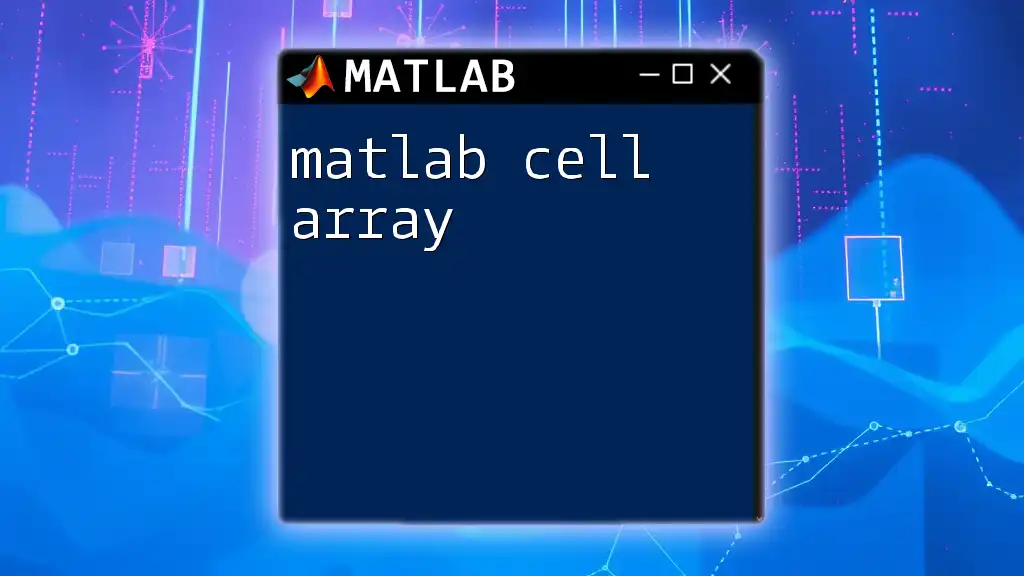
Detailed Breakdown of the Flip Arrays Functions
The flip Function
How it Works
The `flip` function can operate on any dimension of the array. A deep understanding of dimensions is vital, especially when working with higher-dimensional arrays.
Optional Arguments: When you specify the dimension, you can explicitly control how the flipping occurs. The default behavior of `flip` without specifying a dimension is to flip along the first non-singleton dimension.
Practical Examples
-
Example with a vector:
vec = [1, 2, 3, 4]; flippedVec = flip(vec); % flippedVec will be [4, 3, 2, 1]
-
Example with a matrix:
mat = [1, 2; 3, 4; 5, 6]; flippedMat = flip(mat, 1); % Flip along first dimension (vertically) % flippedMat will be [5, 6; 3, 4; 1, 2]
The flipud Function
Usage Scenarios
The `flipud` function is particularly useful in applications where the order of elements is critical, such as reversing time series data or reorganizing a matrix for visual representation.
Practical Examples
- Example with a matrix:
mat = [1, 2; 3, 4; 5, 6]; flippedUD = flipud(mat); % flippedUD will be [5, 6; 3, 4; 1, 2]
The fliplr Function
Usage Scenarios
Use `fliplr` when manipulating data presented in a tabular format. This could involve altering image matrices or datasets where column order is significant.
Practical Examples
- Example with a matrix:
mat = [1, 2; 3, 4; 5, 6]; flippedLR = fliplr(mat); % flippedLR will be [2, 1; 4, 3; 6, 5]
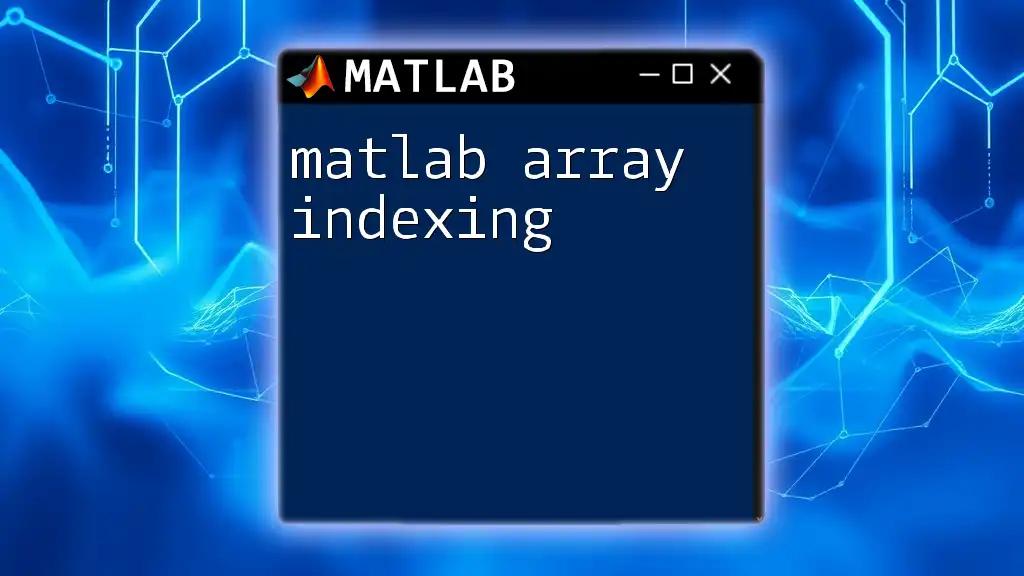
Combining Flip Functions
Chaining Operations
It is possible and often practical to combine multiple flip operations to achieve specific transformation objectives on arrays.
Example of Chaining
result = flipud(fliplr(mat));
% This first flips the matrix left-to-right, then top-to-bottom
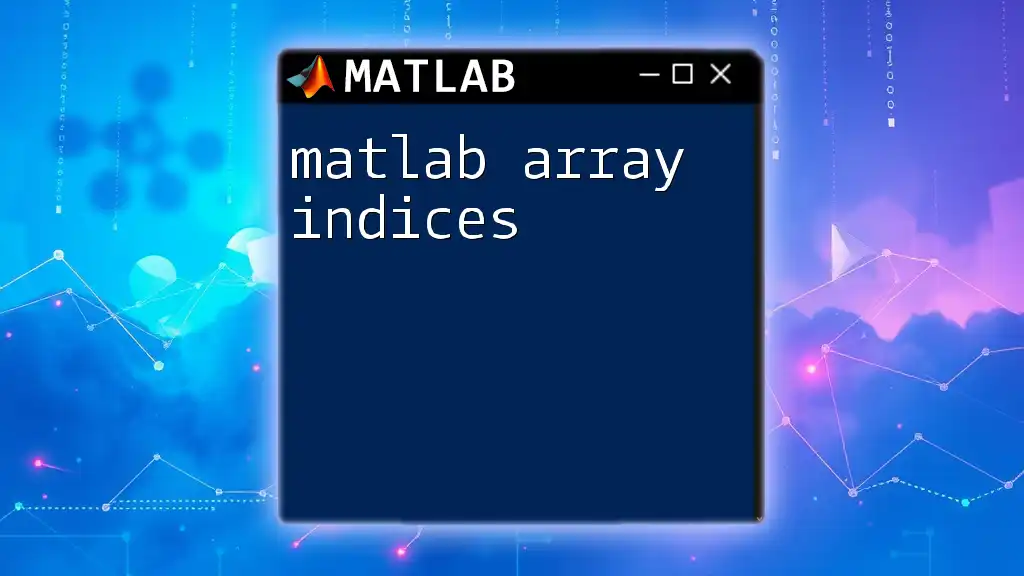
Tips for Effective Use of Flip Functions
General Best Practices
- Choose the Right Function: Understanding when to use `flip`, `flipud`, or `fliplr` can streamline your data processing tasks.
- Test Outputs: After flipping an array, always examine the result to ensure the transformation achieved the desired effect.
Performance Considerations
When working with vast arrays, keep in mind the impact of size on performance. Flipping operations can be computationally intensive, especially with larger datasets. Employing these functions on subarrays or smaller segments can optimize your workflow.
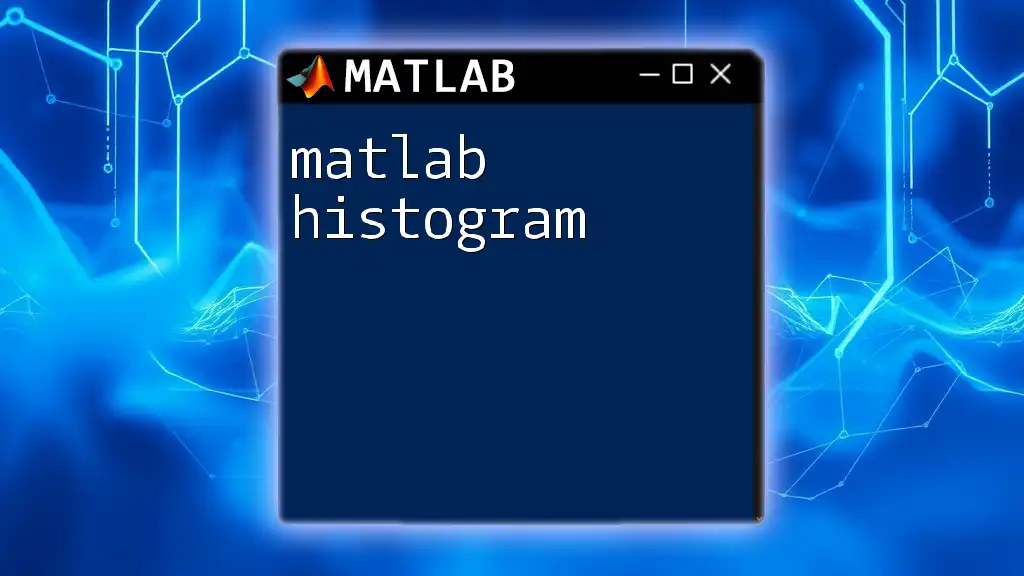
Conclusion
In conclusion, mastering the MATLAB flip array commands can significantly enhance your capabilities in data manipulation and analysis. By effectively using the `flip`, `flipud`, and `fliplr` functions, you will unlock new dimensions of data handling that can be applied across various projects and industries.
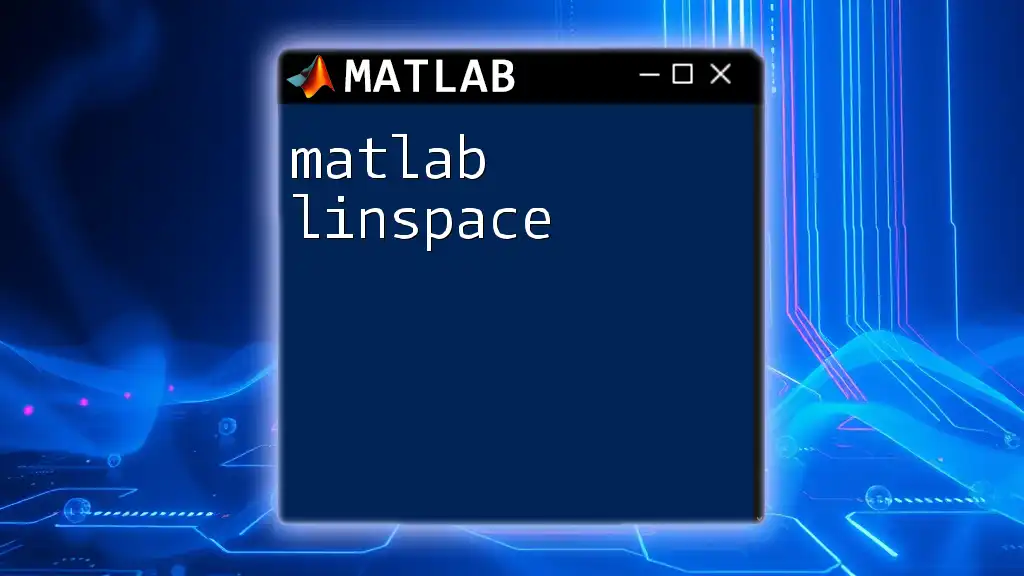
Additional Resources
Recommended Reading
For further exploration, consult the official MATLAB documentation and various online resources that provide tutorials on array manipulations.
Suggested Exercises
Challenge yourself with exercises that involve flipping different types of arrays, gradually increasing complexity as you become more familiar with the concepts.
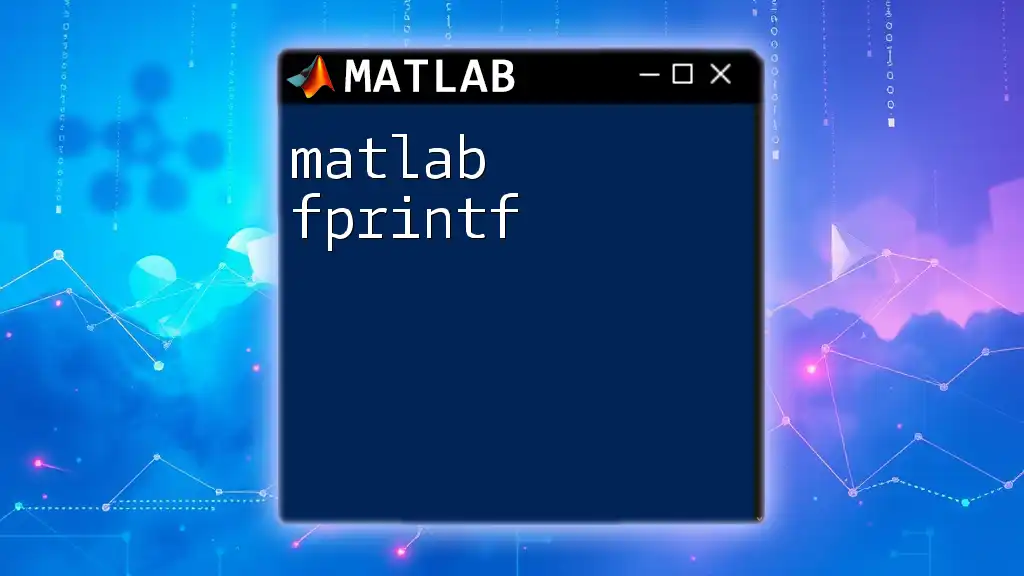
Call to Action
Join our community for more tips and tricks on mastering MATLAB! Subscribe to our newsletter for the latest updates and insights on optimizing your programming skills.