The `find` function in MATLAB is used to locate the indices of array elements that meet a specified condition.
% Example: Find the indices of elements greater than 10 in an array
A = [4, 15, 7, 23, 8];
indices = find(A > 10);
Understanding the Basics of the `find` Function
What is the `find` Function?
The MATLAB function `find` is a powerful tool utilized for locating indices of non-zero elements within an array. This function serves as an essential component in data analysis, enabling users to filter and extract values based on certain conditions. The general syntax for the `find` function is:
index = find(condition)
Where `condition` refers to a logical expression evaluated on the array or matrix elements. When the condition holds true, the indices of those true elements are returned.
Key Features of the `find` Function
The `find` function possesses several notable features:
-
Returns indices of non-zero elements: The primary function of `find` is to identify and return the locations of non-zero elements within an array, making it an important tool in numerical computations and data processing.
-
Conditional filtering capabilities: Users can apply logical conditions when calling the `find` function, allowing them to retrieve specific elements based on custom criteria.
-
Output formats: The function can return results in various formats, including linear indices (1-D) or subscript indices (row and column).
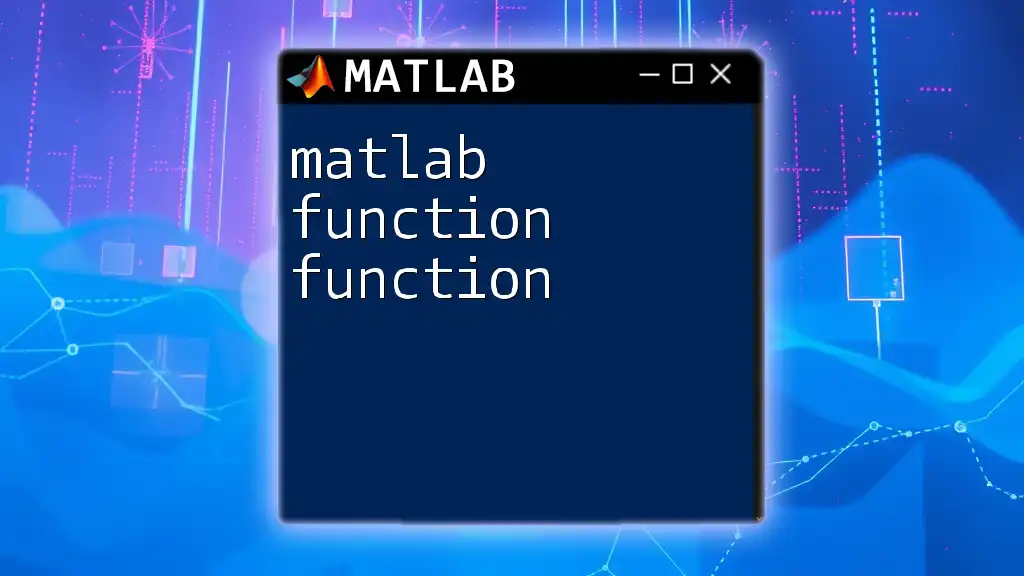
How to Use the `find` Function
Basic Usage
Using the `find` function is straightforward. Here is a simple example where we find elements of an array that are greater than a specified threshold:
A = [1, 4, 7, 3, 8];
indices = find(A > 4);
disp(indices); % Displays: 3, 5
This example demonstrates that elements located at positions 3 and 5 are greater than 4, which corresponds to the values 7 and 8 in the array `A`.
Find with Additional Arguments
Specifying the Number of Outputs
MATLAB allows users to specify the number of outputs from the `find` function, aiming to limit the number of indices returned. The syntax `find(condition, k)` permits users to find the first k indices that meet the specified condition.
For example:
B = [5, 2, 9, 6, 1];
idx = find(B < 5, 2); % Find first 2 indices where values are less than 5
disp(idx); % Displays: 5
In this case, only the index 5 (corresponding to the value 1) is returned, as it is the only value less than 5 within the first 2 checks.
Returning Subscript Indices
You can also use the `find` function to get both row and column indices when applied to a matrix. For instance:
C = [1, 0, 2; 3, 0, 0; 4, 5, 0];
[row, col] = find(C > 0); % Find non-zero elements
disp(row); % Displays: [1; 1; 2; 3; 3]
disp(col); % Displays: [1; 3; 1; 1; 2]
This snippet reveals all positions in matrix `C` where elements are greater than zero. The output will show that non-zero values are located at specific row and column combinations.
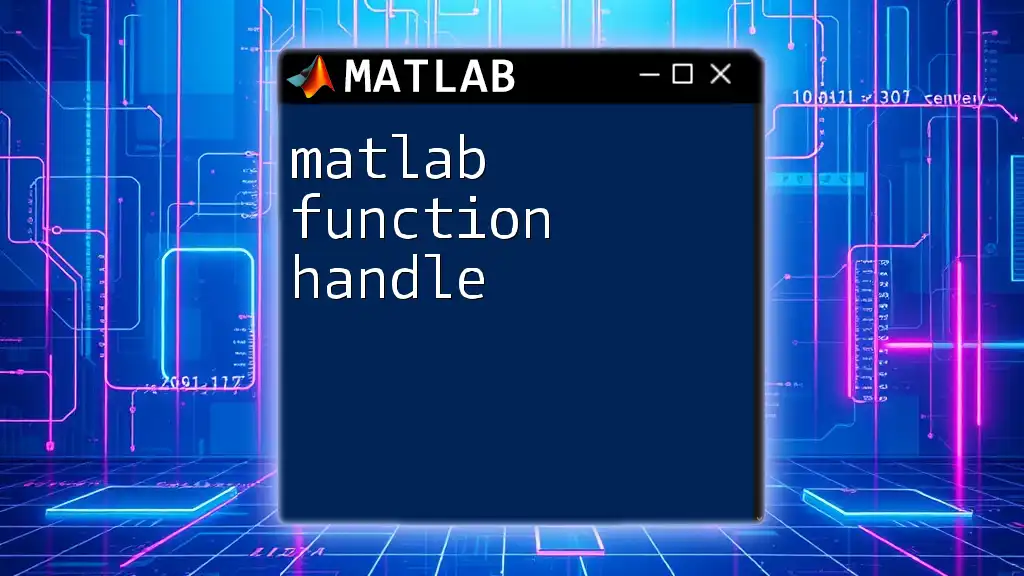
Advanced Usage of the `find` Function
Using Logical Arrays
The convenience of the `find` function is amplified when combined with logical arrays. A logical condition can be expressed directly to find indices of elements that return true.
For example:
D = logical([0, 1, 0, 1, 1]);
indices = find(D); % Finds where true
disp(indices); % Displays: 2, 4, 5
In this case, the function returns indices 2, 4, and 5, which correspond to the true entries in the logical array.
Combining `find` with Other Functions
Integration with `sort`
The `find` function can be seamlessly integrated with other MATLAB capabilities, such as `sort`. This combination is useful for sorting datasets while maintaining an association with the original indices. For example:
E = [4, 2, 3, 1];
[sorted_vals, original_indices] = sort(E);
disp(original_indices); % Displays: 4, 2, 3, 1
The `sort` function arranges the values in increasing order while simultaneously tracking where each original value migrated within the new sorted array.
Avoiding Common Pitfalls
Even experienced users of the `find` function may encounter common issues. One such oversight is using `find` on an empty matrix, which can yield unexpected results. Always check for the presence of data before deploying this function.
When uncertain about conditions, leveraging other functions like `isempty` can help maintain smoother workflows.
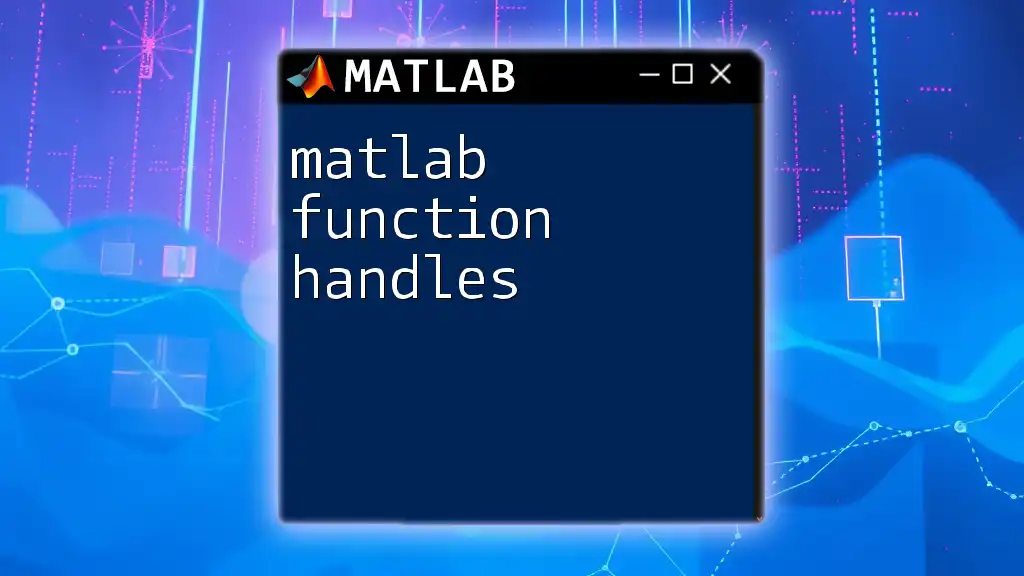
Practical Applications of the `find` Function
Data Analysis
In real-world data analysis tasks, the `find` function proves invaluable. For exampl,e it can be utilized to identify outlier values within a dataset, helping analysts hone in on potentially erroneous or interesting data points.
Here’s how you can find outliers in a simple dataset:
data = [10, 12, 15, 100, 14, 13]; % Outlier at 100
outlier_indices = find(data > 50);
disp(outlier_indices); % Displays: 4
In this scenario, the `find` function pinpoints the 4th index, indicating the outlier value of 100.
Image Processing
In the field of image processing, the `find` function can be employed to locate non-zero pixels in a binary image. This application is crucial for tasks like edge detection, feature extraction, or any other analytical tasks requiring focus on active pixels.
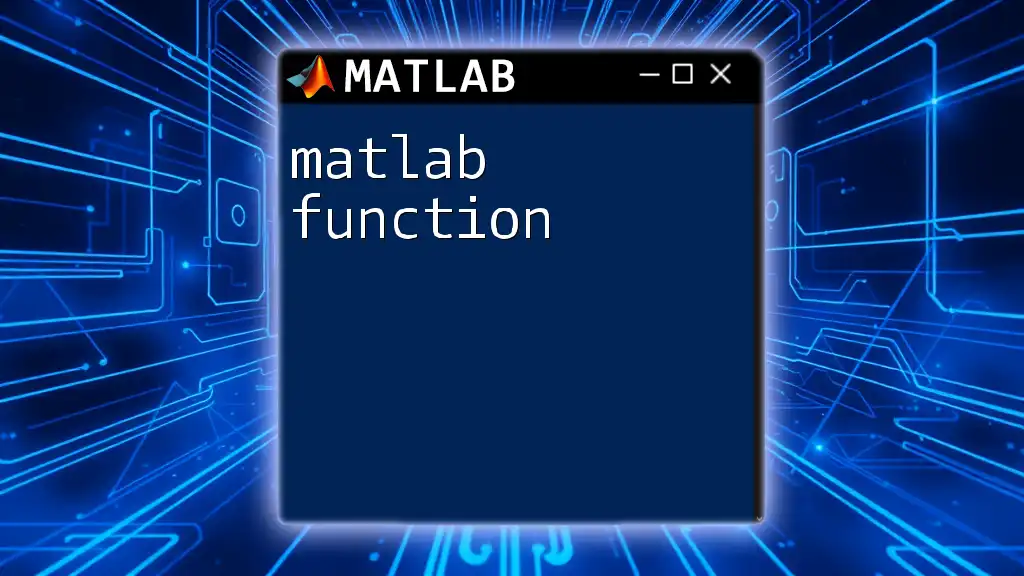
Conclusion
Mastering the MATLAB function `find` is essential for anyone interested in data analysis and programming within the MATLAB environment. By employing this function, users can effectively filter data, identify critical values, and manipulate matrices, making it a powerful asset in any MATLAB programmer's toolkit.
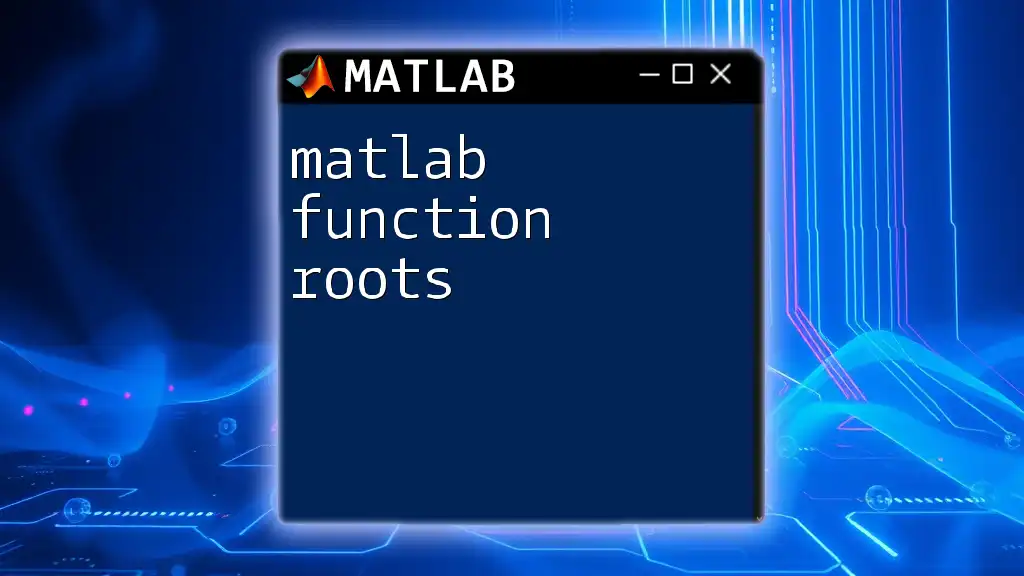
Additional Resources
For further enhancement of your understanding, refer to the official MATLAB documentation, where in-depth descriptions and additional examples are readily available. Explore online tutorials and courses centered around MATLAB to accelerate your learning journey.
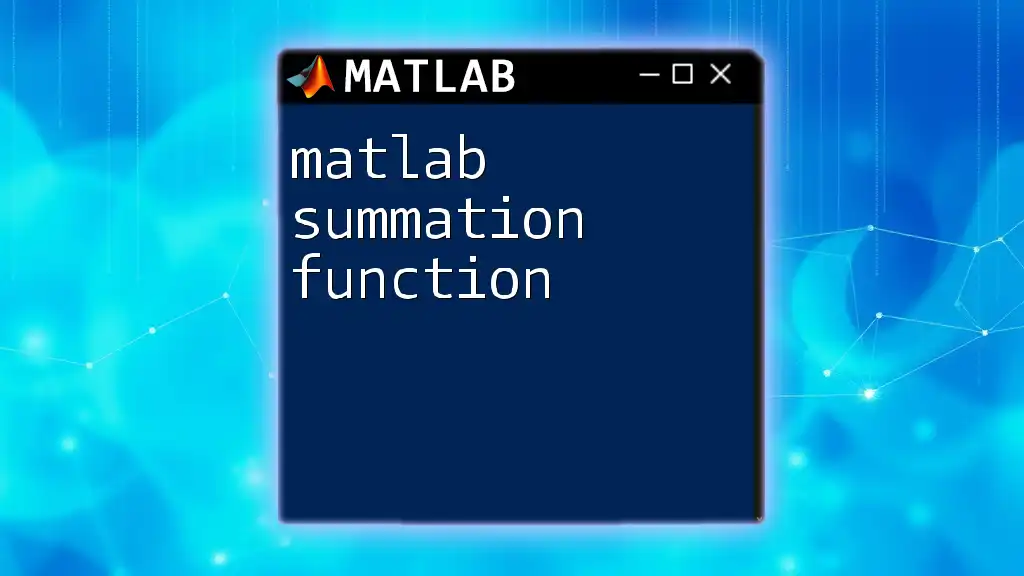
Call to Action
If you found this guide helpful, consider subscribing for more informative posts and updates on MATLAB functions. We're eager to hear your experiences and insights regarding the usage of the `find` function, so please feel free to share your thoughts in the comments!