A MATLAB function handle is a MATLAB data type that allows you to reference a function, enabling you to pass it as an argument, store it in variables, or use it in function operations.
Here’s a simple code snippet demonstrating how to create and use a function handle:
% Define a simple function
f = @(x) x.^2; % Function handle for the square function
% Use the function handle
result = f(5); % This will return 25
disp(result);
What are Function Handles?
Definition
In MATLAB, a function handle is a MATLAB data type that represents a function. Function handles allow you to call a function indirectly and are particularly useful when you want to pass functions as arguments to other functions. By creating a handle to a function, you can execute the function without having to know the function's name explicitly.
Why Use Function Handles?
Using function handles brings several advantages:
- Flexibility: They allow functions to be treated as first-class citizens, which means you can pass them as arguments, return them from other functions, or store them in data structures.
- Increased Code Modularity: Function handles promote code reusability. You can create, modify, and pass functions around without rewriting code.

Creating Function Handles
Syntax of Function Handles
The general syntax for creating a function handle in MATLAB is simple and intuitive:
f = @myFunction;
In this syntax, `@` denotes a function handle. It effectively points to the specified function, `myFunction`, allowing you to call it using the handle `f`.
Examples of Creating Function Handles
Simple Function Handle
Here's an example demonstrating how to create a function handle for a simple function:
function y = square(x)
y = x^2;
end
f = @square; % Create a function handle to the 'square' function
Now, calling `f(5)` will return 25 since it internally calls the `square` function.
Anonymous Functions
Anonymous functions are a compact way to define single-line functions without needing a separate file. Here's how to create one:
f = @(x) x^2; % Anonymous function for squaring a number
You can now use `f(4)` and obtain the result 16.

Using Function Handles
Passing Function Handles to Other Functions
Function handles can be passed to many built-in MATLAB functions, enhancing their functionality. One common use case is in the `arrayfun` function, which applies a function to each element of an array.
Example: Using `arrayfun`
For instance, using the `square` function we created earlier:
result = arrayfun(f, [1, 2, 3, 4, 5]); % Result: [1, 4, 9, 16, 25]
In this example, `arrayfun` calls the function handle `f` for each element in the vector, producing an array of squared values.
Function Handle as Callback Functions
In GUI programming or applications, function handles are often used as callback functions. This allows you to define custom behavior when certain events occur (like button presses). For example, you could define a function handle that executes when a user clicks a button:
function buttonCallback(src, event)
disp('Button clicked!');
end
hButton = uicontrol('Style', 'pushbutton', 'Callback', @buttonCallback);
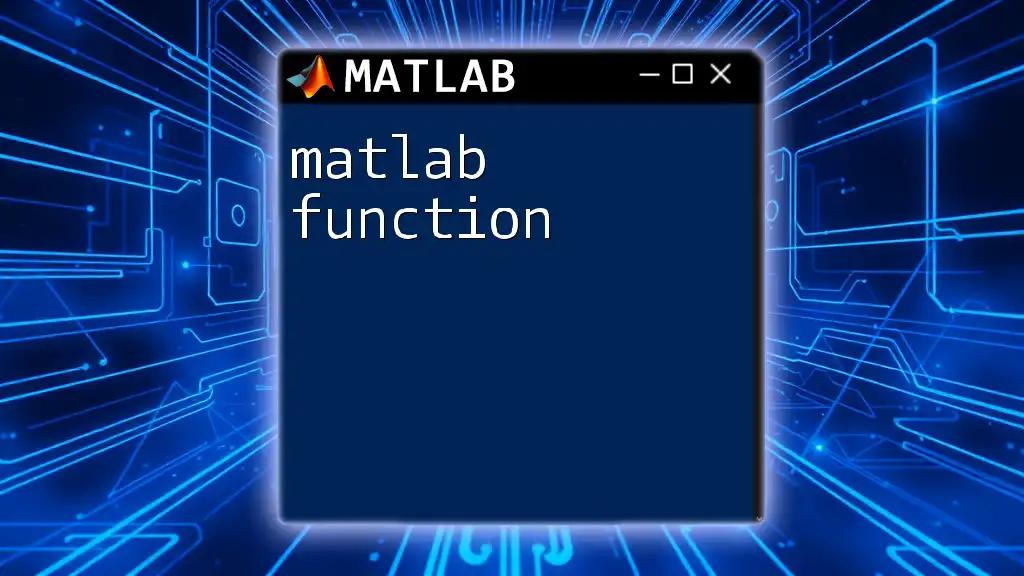
Function Handle with Multiple Inputs
Syntax for Functions with Multiple Inputs
Creating function handles that accept multiple inputs is also straightforward. The syntax looks like this:
g = @(x, y) x + y; % Function handle for adding two numbers
Example of Using Multiple Input Function Handles
You can then call this function handle with multiple arguments:
result = g(3, 5); % Result: 8
This functionality proves useful for complex operations requiring multiple parameters.

Storing and Manipulating Function Handles
Storing Function Handles in Arrays
Function handles can be stored in arrays or cell arrays for greater organization and flexibility. For example:
functions = {@square, @(x) x^3, @(x) sin(x)};
Here, we've created a cell array containing different function handles.
Executing Function Handles in a Loop
You can easily execute these function handles using a loop, as shown below:
for i = 1:length(functions)
disp(functions{i}(2)); % Executes each function handle with input 2
end
This snippet showcases how to apply all stored functions with the input value of 2, allowing you to see how each function responds.
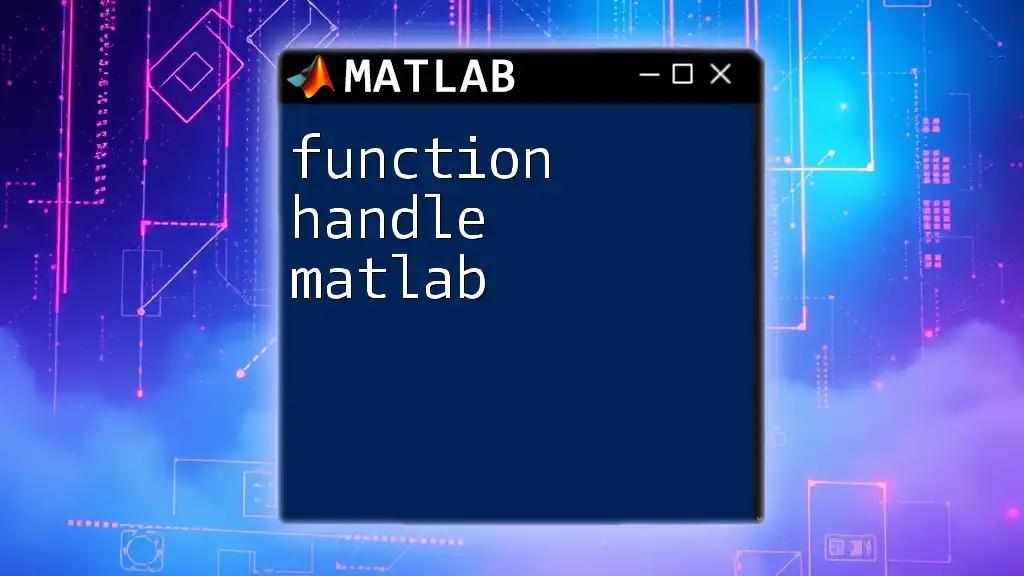
Advanced Topics in Function Handles
Handling Anonymous Functions
While anonymous functions can be very useful for short, specific tasks, they do have limitations. For example, they cannot span multiple lines or have local variables unless you declare them as nested functions. When using them, consider the complexity of the operations; if they're too intricate, opt for a standard function.
Nested Functions and Function Handles
Another powerful feature of MATLAB involves nested functions. Nesting allows a function to access the workspace of its parent function. When combined with function handles, this opens diverse possibilities. Here’s a simple example:
function parentFunction()
a = 10;
nestedFunction = @(x) x + a; % Access 'a' from the parent function
disp(nestedFunction(5)); % Result: 15
end
In this code, the nested function can access and utilize the variable `a` defined in the parent function efficiently.
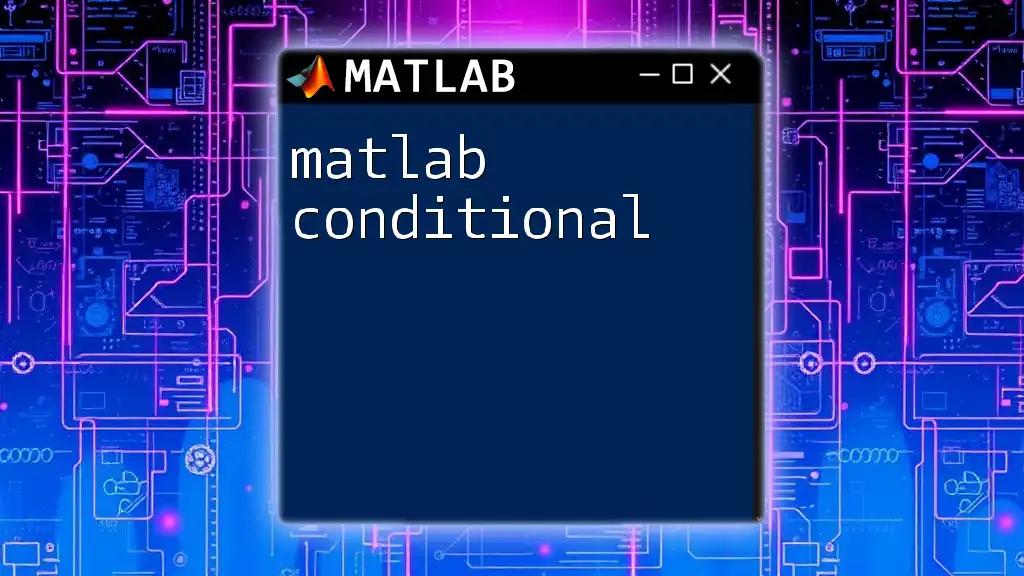
Common Pitfalls and Best Practices
Common Mistakes with Function Handles
Users often encounter pitfalls when creating or using function handles, such as:
- Forgetting to include the `@` symbol, resulting in errors when trying to call a function.
- Confusing the input order of multi-input functions within function handles.
Best Practices for Function Handle Usage
To ensure you maximize the power of function handles, follow these guidelines:
- Consistent Naming Conventions: Use descriptive names for your function handles to improve code readability.
- Documentation: Always include comments describing the purpose of each function handle.
- Use Anonymous Functions Sparingly: Reserve anonymous functions for simple expressions; for anything complex, define a full function.
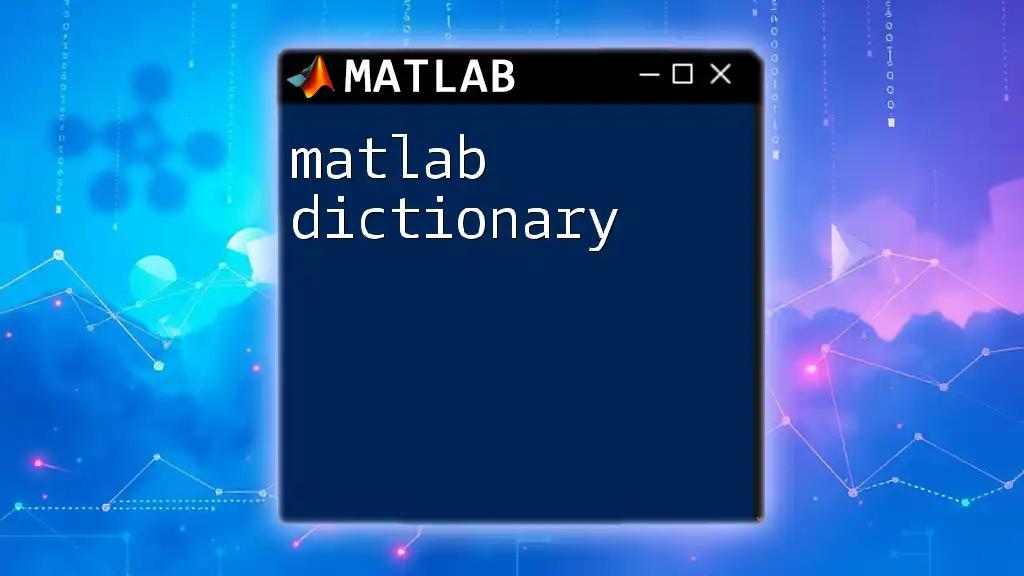
Conclusion
In summary, mastering the use of MATLAB function handles constitutes an essential skill for any MATLAB programmer. These handles enhance your coding efficiency, modularize your functions, and provide the flexibility needed for advanced programming techniques. As you practice creating and employing function handles, you will find numerous ways to streamline your workflow and improve your MATLAB projects. Start exploring and engaging more with the expansive possibilities MATLAB offers beyond just function handles!