In MATLAB, plot markers are symbols used to denote individual data points in plots, enhancing the visualization of datasets.
Here’s a code snippet demonstrating how to create a simple plot with different markers:
x = 0:0.1:10; % Define x data
y = sin(x); % Define y data
figure; % Create a new figure
plot(x, y, 'o', 'MarkerFaceColor', 'r'); % Plot with red circle markers
hold on; % Keep the current plot
plot(x, cos(x), 's', 'MarkerFaceColor', 'b'); % Plot with blue square markers
title('Plot with Different Markers'); % Title for the plot
xlabel('X-axis'); % Label for x-axis
ylabel('Y-axis'); % Label for y-axis
legend('sin(x)', 'cos(x)'); % Legend to describe the plots
grid on; % Turn on the grid
What are Plot Markers in Matlab?
In Matlab, plot markers serve as visual representations for data points in a graphical plot. They are essential for differentiating points in scatter plots, adding many elements to line plots, and enhancing the overall readability of graphical representations. Knowing when to use markers instead of just lines can significantly improve the communication of data insights.
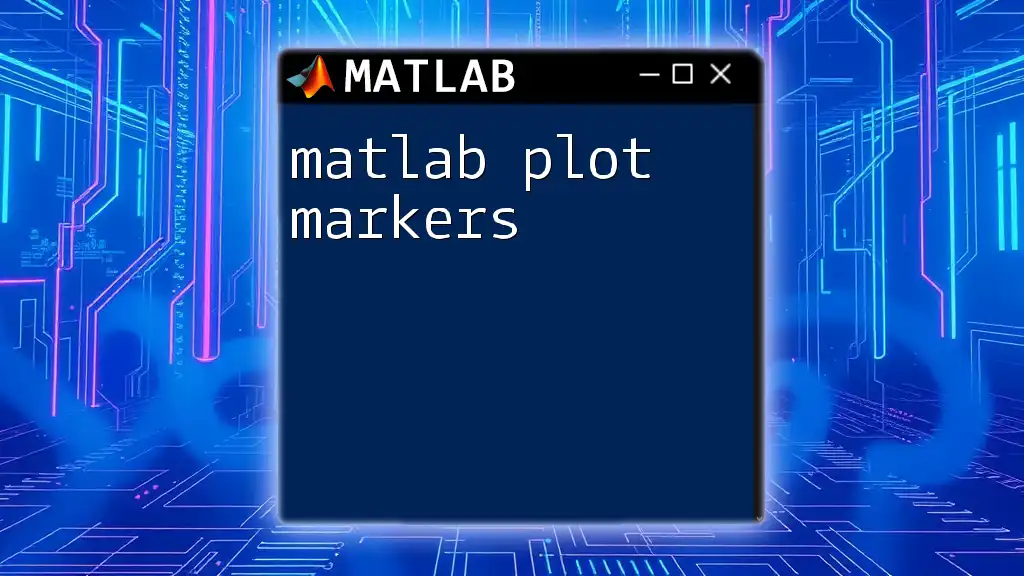
Types of Plot Markers
Commonly Used Markers in Matlab
Various markers exist to cater to different visualization needs, each serving specific purposes. Below are some of the most commonly used plot markers along with visual representations that help to communicate different datasets effectively:
- Circle ('o')
- Square ('s')
- Diamond ('d')
- Plus ('+')
- Cross ('x')
- Star ('*')
Each marker has a distinctive shape, allowing viewers to quickly identify and differentiate between data series in a plot.
Custom Markers
In addition to standard markers, Matlab allows for custom markers. You can specify a marker’s shape using a three-column matrix that defines the coordinates of the marker points. This flexibility enables you to create unique markers that align with your visualization style or project requirements.
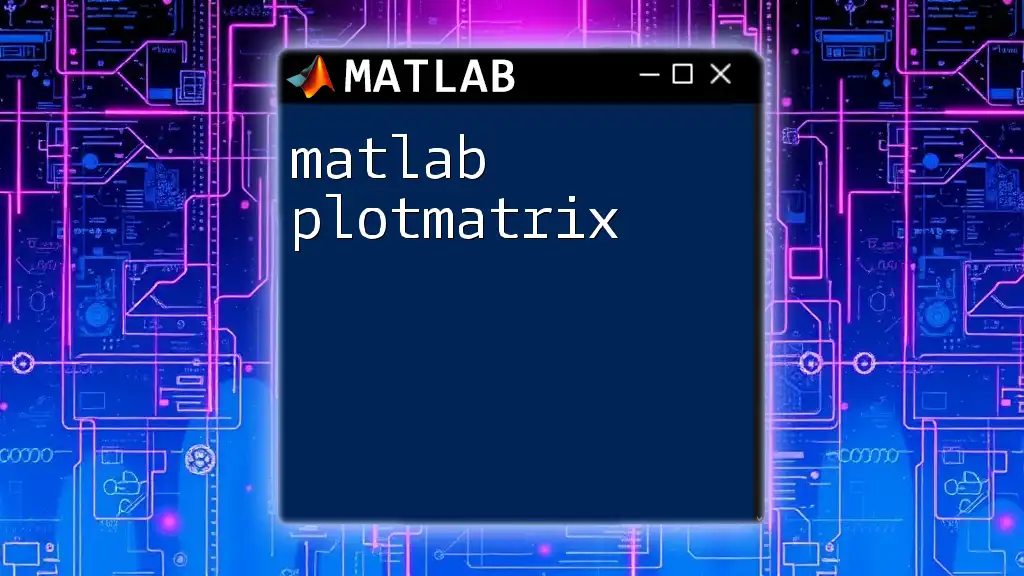
Basic Syntax for Plotting with Markers
The `plot()` Function
The primary function for creating plots in Matlab is the `plot()` function. It allows for easy customization of how data points are represented, including the use of markers.
The basic syntax to incorporate markers in a plot is as follows:
plot(X, Y, 'MarkerType')
Here's a simple example illustrating how to plot data points using a circle marker:
x = 0:0.1:10;
y = sin(x);
plot(x, y, 'o') % Plot with circle markers
In this example, the sine function is graphed, and each point along the curve is marked with a circle, providing a clear visual reference for the value of the sine function at different points.
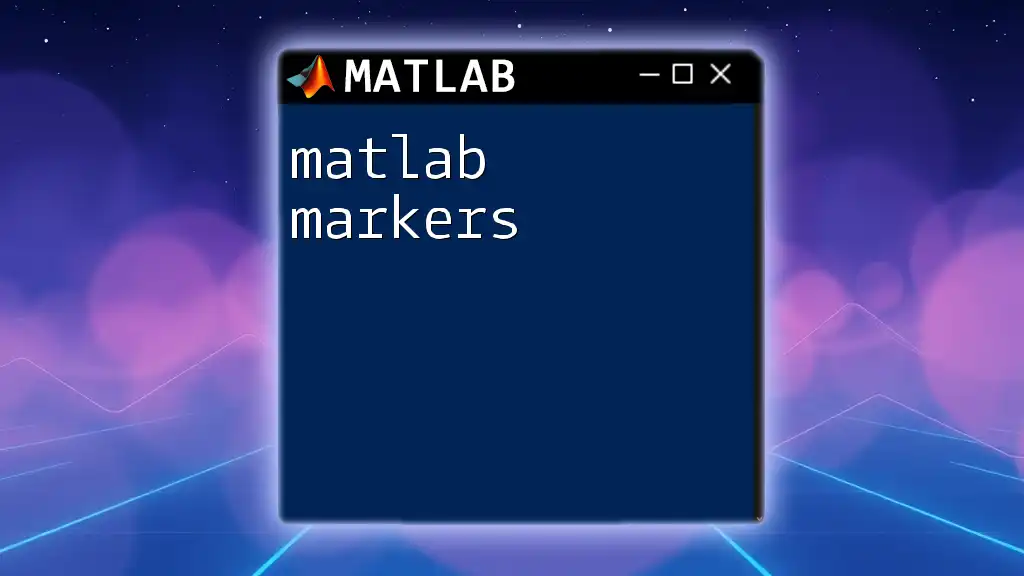
Detailed Explanation of Marker Properties
Marker Size
One important aspect to consider when using markers is their size. Adjusting the marker size can significantly impact the visibility and clarity of your plots. You can easily change the marker size using the `MarkerSize` property:
plot(x, y, 'o', 'MarkerSize', 10)
In this command, the size of the circle markers is increased, making them more prominent and easier to identify.
Marker Color
Another essential property is the marker color. Customizing the colors of markers can help convey additional information or create more visually appealing plots. You can set both edge and face colors using:
plot(x, y, 'o', 'MarkerEdgeColor', 'red', 'MarkerFaceColor', 'yellow')
This code sets the edge color of the markers to red and the inside (face) of the markers to yellow, enabling quick visual differentiation.
Combining Markers with Line Styles
Combining markers with lines can provide additional context to your plots. The ability to blend these styles enhances the interpretability of visual data. Here's an example of plotting a sine function with both a line and markers:
plot(x, y, '-o', 'MarkerSize', 8, 'MarkerEdgeColor', 'blue')
In this plot, the solid line indicates the change in the sine function, while the circular markers highlight specific data points, offering a comprehensive perspective of the function's behavior.
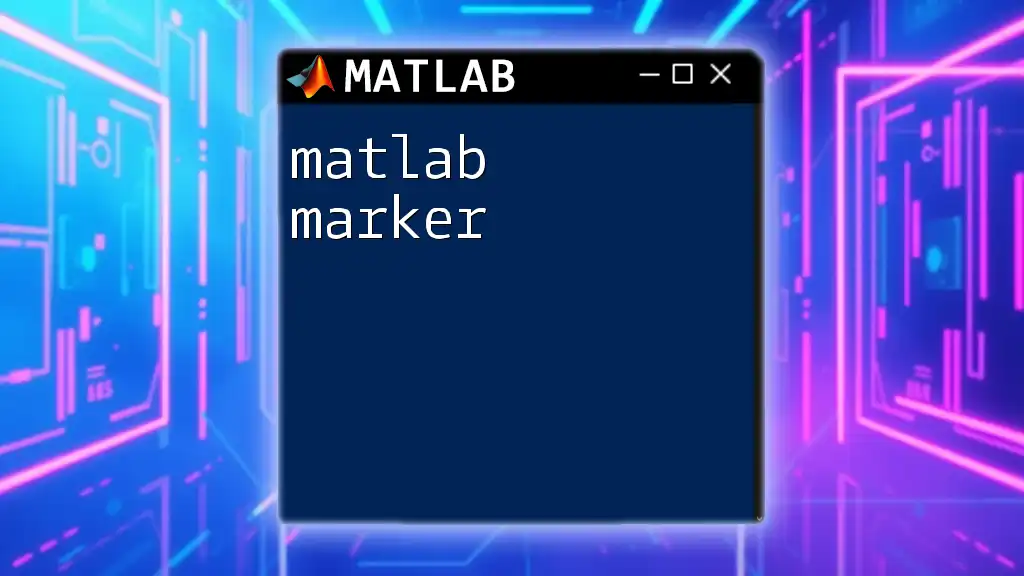
Using Markers in Different Plotting Functions
Scatter Plots
The `scatter()` function is specifically designed for detailed scatter plots, facilitating more robust usage of markers. Here is how you can create a scatter plot with customized markers:
scatter(x, y, 100, 'filled', 'MarkerEdgeColor', 'black')
In this example, the `100` specifies the size of the markers, and `filled` makes the markers solid, enabling a clear view of data density.
Other Plot Types
Markers are not limited to simple plots. They can be effectively used in various other types such as bar plots or plots with error bars. For instance, when using error bars, markers can further inform viewers about the reliability of the data:
errorbar(x, y, 0.1, 'o')
In this command, error bars are added to the data points, each represented by circle markers, thus providing both the data value and its associated uncertainty.
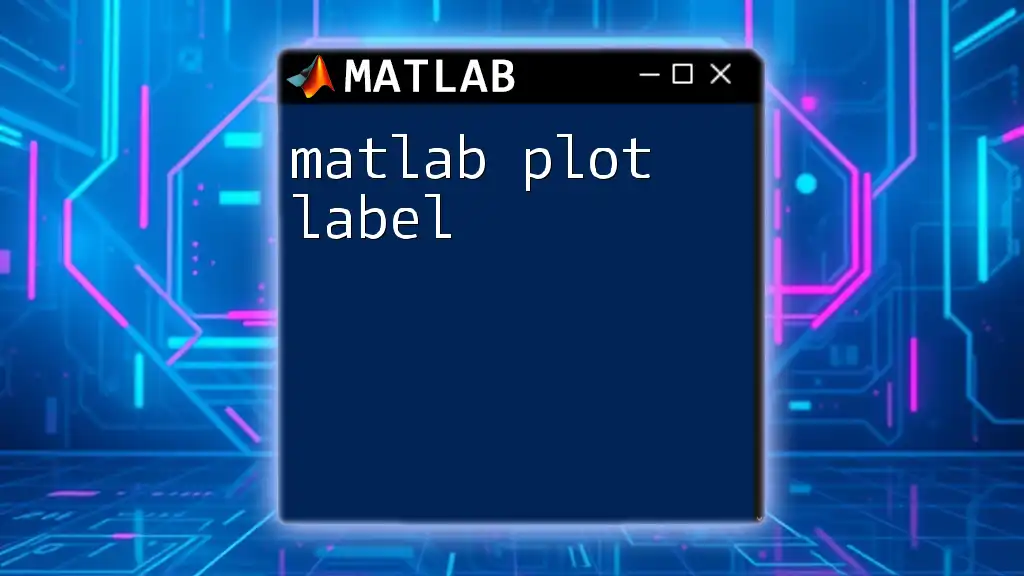
Best Practices for Using Plot Markers
Choosing the Right Marker
When selecting markers for your plots, it’s crucial to think about the context of your data. Opt for markers that enhance readability and differentiate datasets effectively. For example, use larger markers for significant data points, or consider custom shapes for specific classifications within your data.
Maintaining Consistency
Consistency in the use and style of markers across multiple plots is essential. Adopting a uniform approach helps the audience follow your work more easily and recognize patterns across different datasets. For instance, if you choose square markers for one set, consider using the same shape across other plots unless there’s a compelling reason to change.
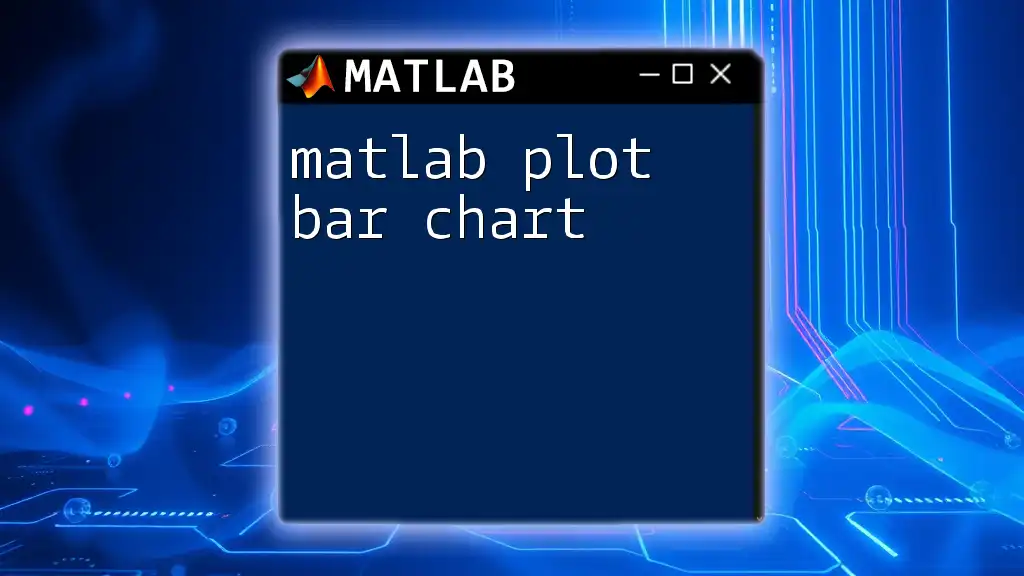
Troubleshooting Common Issues with Markers
Marker Overlap and Clarity
When too many data points overlap in a plot, markers can become indistinguishable. A few strategies include adjusting marker size or using transparency features to differentiate overlapping points for better clarity.
Compatibility with Other Graphic Elements
It’s important to ensure that markers complement other graphical elements without causing clutter. Whenever possible, maintain a clean design by balancing markers, lines, and annotations. Clear labeling of axes and using legends can further enhance the overall readability.
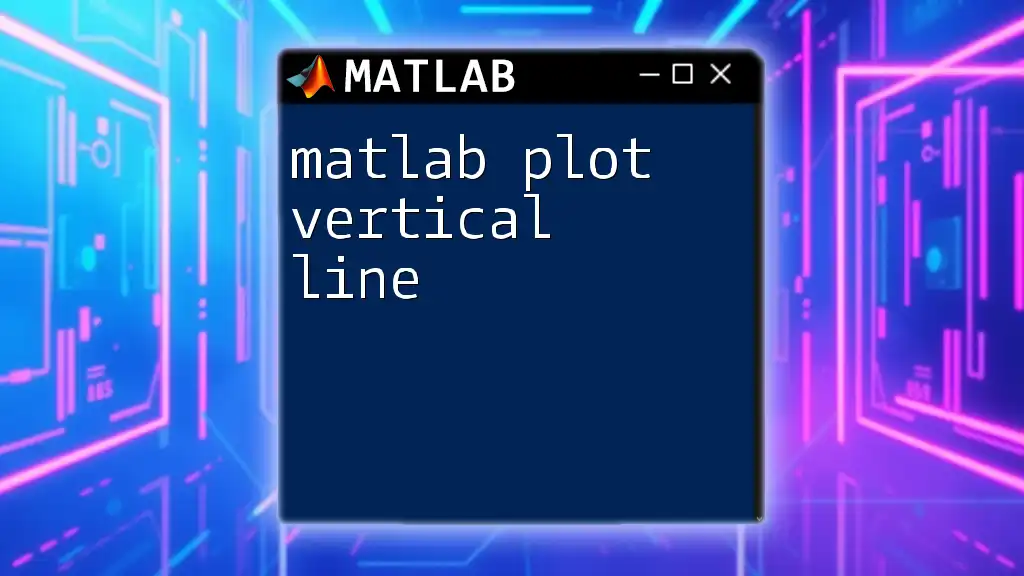
Conclusion
Utilizing effective Matlab plot markers is vital for creating informative and visually appealing plots. By experimenting with different styles and properties, you can significantly enhance your understanding and presentation of data. Engaging with various marker options not only improves your plotting skills but also promotes better communication of data insights. Share your experiences and questions regarding Matlab plots, and connect with the community to learn more!
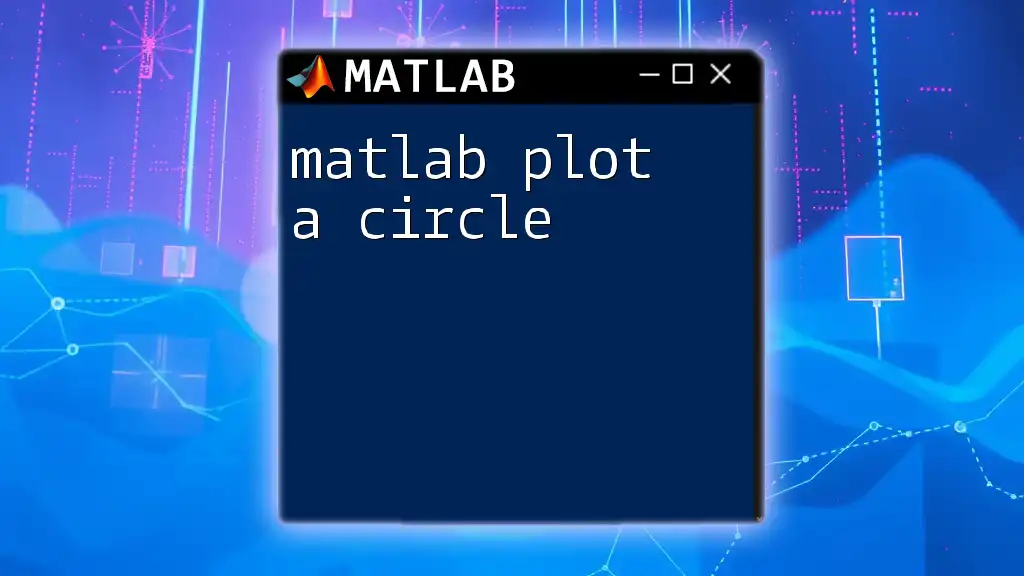
Additional Resources
For further exploration, it's beneficial to access the official Matlab documentation on plotting and seek out tutorials and courses that will expand your knowledge on the subject. Engaging with online forums and user communities can also provide support in your journey to master Matlab and its plotting capabilities.