In MATLAB, you can easily add a title to your plot using the `title` function to enhance the readability and provide context for your visual data representation.
Here's a code snippet demonstrating how to use the `title` function:
x = 0:0.1:10; % Create a range of x values
y = sin(x); % Compute the sine of x values
plot(x, y); % Generate the plot
title('Sine Wave from 0 to 10'); % Add a title to the plot
Understanding Plot Titles in MATLAB
What is a Plot Title?
A plot title serves as a brief description that encapsulates what the plot visually represents. It is typically displayed at the top of a plot and is crucial for providing context to the data being presented. An effective title not only captures the essence of the information but also enhances the audience's understanding, allowing them to derive insights quickly.
The Syntax of a Plot Title
In MATLAB, adding a title to your plot is straightforward, thanks to the built-in `title()` function. The basic syntax follows this structure:
title('Your Plot Title Here');
Here, the string you provide as an argument in the `title()` function becomes the title of your plot. This simple function is the key to presenting your data meaningfully.
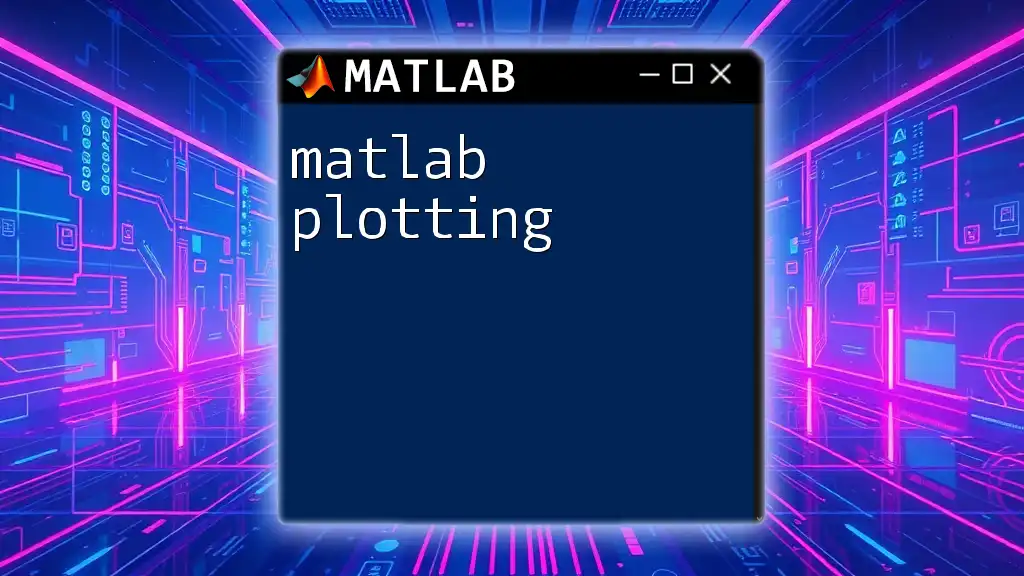
Creating Your First Plot Title
Step-by-Step Guide to Adding a Title
Let's create our first plot and add a title. Below is a basic example that demonstrates this process:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Sine Wave');
Explanation of Each Part:
- `x = 0:0.1:10;`: This line generates a vector of values from 0 to 10, incremented by 0.1, which will serve as the x-axis data.
- `y = sin(x);`: Here, we compute the sine of each element in the vector `x`, creating the data points for the y-axis.
- `plot(x, y);`: This command creates the plot with `x` on the horizontal axis and `y` on the vertical axis.
- `title('Sine Wave');`: Finally, we add the title "Sine Wave" to our plot.
Customizing Your Title
Titles are more impactful when customized. Here are some ways to enhance the appearance of your plot title.
Font Properties
Changing the title's font size, style, and color can add clarity and emphasis. The following code snippet demonstrates how to apply these modifications:
title('Sine Wave', 'FontSize', 14, 'FontWeight', 'bold', 'Color', 'blue');
Explanation of Properties:
- FontSize: Specifies the size of the font.
- FontWeight: Determines the thickness of the text (e.g., bold).
- Color: Changes the color of the title text (e.g., blue) to attract attention.
Adding Linebreaks
For more complex titles, line breaks can improve readability. Use curly braces `{}` to create a multi-line title:
title({'Sine Wave'; 'Amplitude vs Time'});
Best Practices: Use line breaks for titles that consist of multiple parts or where additional context is needed. This helps maintain viewer focus and ensures clarity.
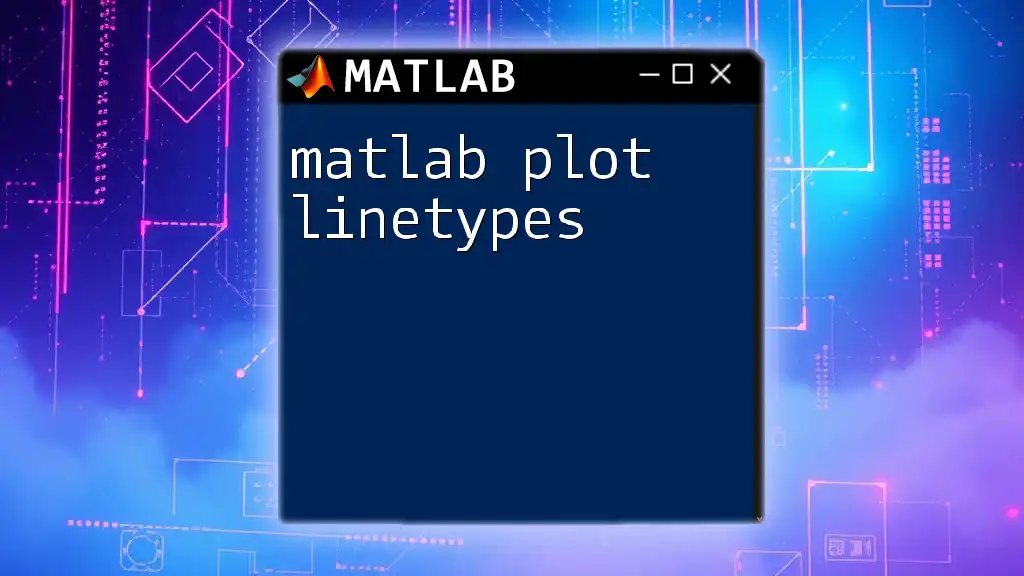
Advanced Title Features
Adding Mathematical Expressions
MATLAB allows users to include mathematical expressions in titles using the TeX or LaTeX interpreter. This feature is particularly useful for scientific plots.
Using TeX Interpreter
Here’s how to use the TeX interpreter for title formatting:
title('y = sin(x)', 'Interpreter', 'latex');
By specifying `Interpreter` as `latex`, MATLAB recognizes LaTeX commands, allowing you to create beautifully formatted equations within your plot titles.
Multi-line Titles
Creating complex multi-line titles can add depth to the information presented. Here's an example:
title({'Sine Wave'; 'y = sin(x)'; 'Frequency: 1 Hz'});
Guidelines for Multi-line Titles:
- Maintain clarity by avoiding excessive wording.
- Ensure each line contributes meaningful context to the title.
- Use consistent formatting throughout the title to maintain a professional appearance.
Dynamic Titles
Dynamic titles are a powerful way to enhance interactivity in your plots. You can incorporate variables or values directly into your title for a personalized touch.
Using Variables in Titles
Consider this example where a frequency variable is included in the title:
frequency = 1;
title(['Sine Wave with Frequency: ', num2str(frequency), ' Hz']);
Benefits of Dynamic Titles:
- Adjust the title automatically based on variable changes, enhancing the plot's informativeness.
- Facilitate more dynamic presentations where the title reflects real-time data or user inputs.
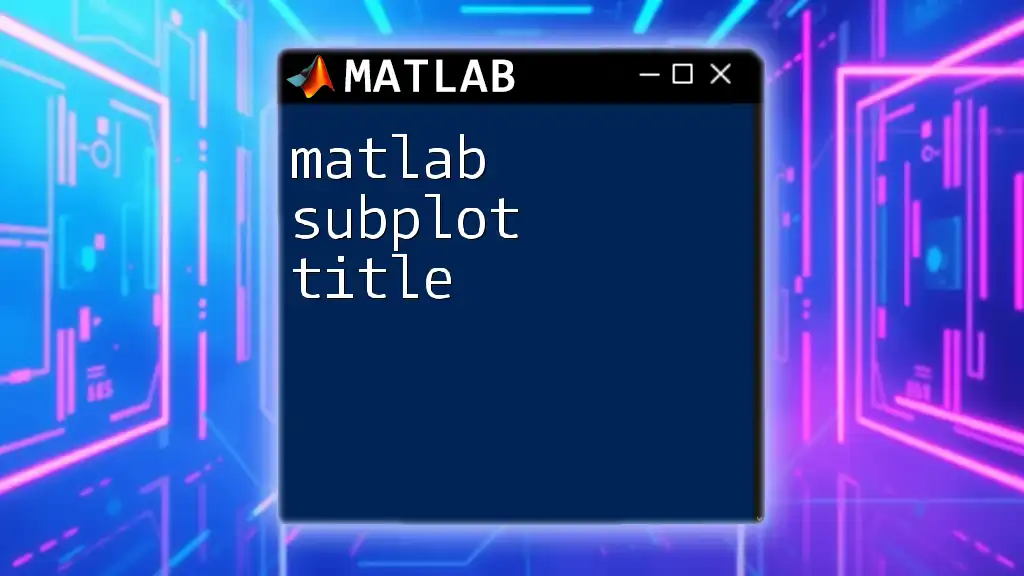
Common Mistakes to Avoid
Overcomplicating Titles
While it's tempting to include all possible details in your title, it’s essential to strike a balance. A title should be concise yet informative. Excessive wording can clutter the plot and distract viewers from the data itself.
Ignoring Audience
Understanding your audience is key. Tailor your titles to match the knowledge level of your viewers. For technical audiences, more complex terms might be appropriate, while a general audience may benefit from simpler language and clearer descriptions.
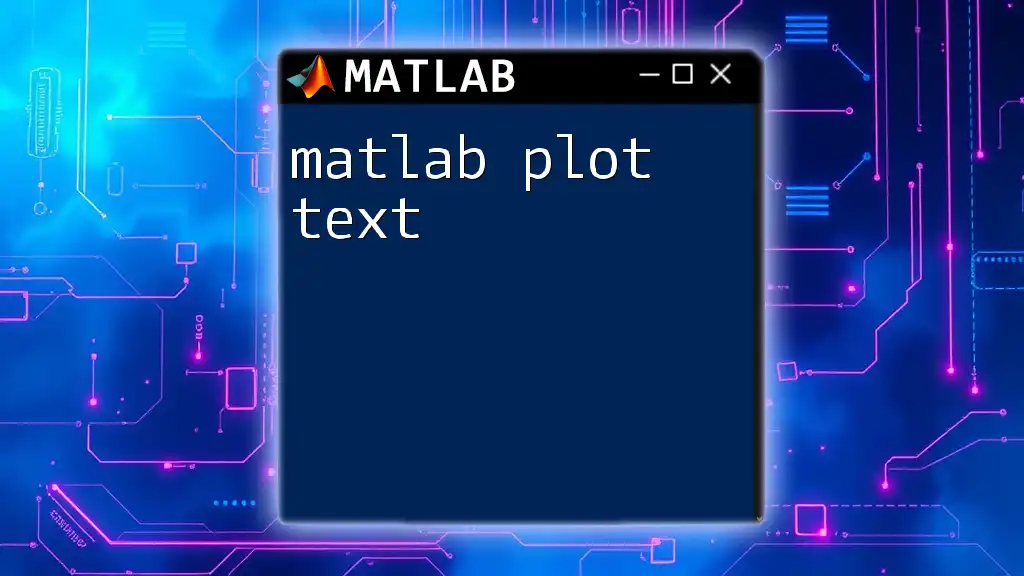
Conclusion
Creating effective plot titles in MATLAB enhances your data visualization significantly. By utilizing the `title()` function and customizing its features—such as font properties and dynamic elements—you can create titles that inform, engage, and clarify the content of your plots.
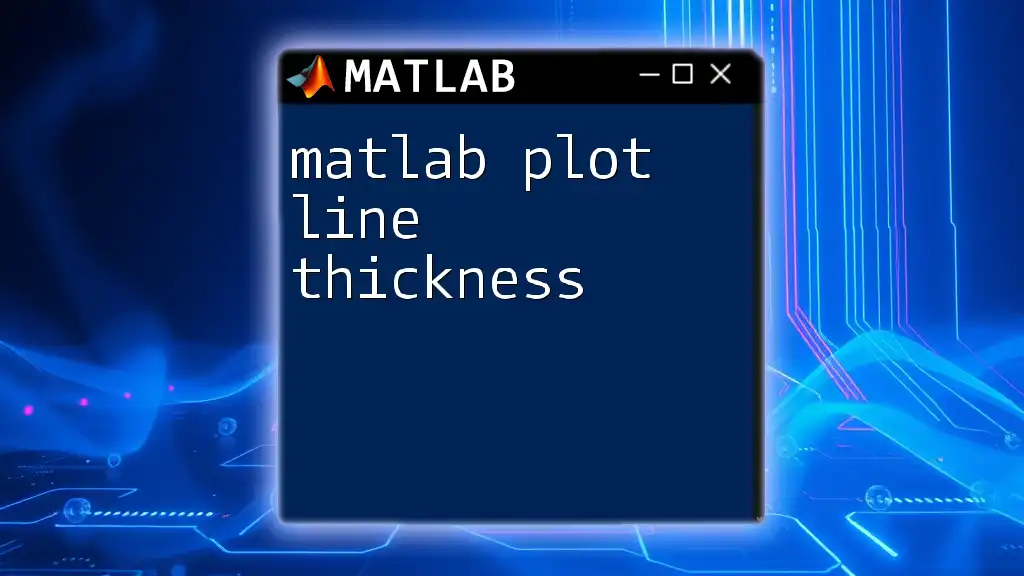
Additional Resources
Books and Tutorials
For those looking to dive deeper into MATLAB plotting techniques, consider exploring specialized books and online tutorials. They can provide additional insights and advanced techniques for effective data visualization.
Online Communities
Joining forums and communities can offer valuable support and collaborative opportunities. Engage with MATLAB enthusiasts, share ideas, and seek help as you refine your plotting skills. This shared learning can be an enriching experience on your journey to mastering MATLAB.