MATLAB App Designer is a powerful tool that allows users to create professional apps with interactive controls and visualizations, enabling a smooth user experience through a drag-and-drop interface.
Here's a simple code snippet to create a basic app with a button that displays a message when clicked:
% Create a simple app with a button using App Designer
function startupFcn(app)
% Create Button
app.Button = uibutton(app.UIFigure, 'Text', 'Click Me', ...
'Position', [100, 100, 100, 30], ...
'ButtonPushedFcn', @(btn,event) displayMessage(app));
end
% Display message when button is clicked
function displayMessage(app)
uialert(app.UIFigure, 'Button was clicked!', 'Alert');
end
What is MATLAB App Designer?
MATLAB App Designer is an interactive environment for building applications with rich user interfaces (UIs) in MATLAB. It allows users to create professional apps without deep programming knowledge, making it accessible for engineers and data scientists. The key features that make App Designer valuable include:
- User-Friendly Interface: A drag-and-drop environment that simplifies component organization.
- Comprehensive Component Library: Access to a diverse range of UI components like tables, buttons, sliders, and more.
- Integrated Coding Environment: Seamlessly write and link code to UI actions.
Compared to traditional MATLAB GUIs, App Designer provides a modern approach to building apps, incorporating advanced design best practices and better layout management. This ensures a smoother user experience and a more aesthetically pleasing output.
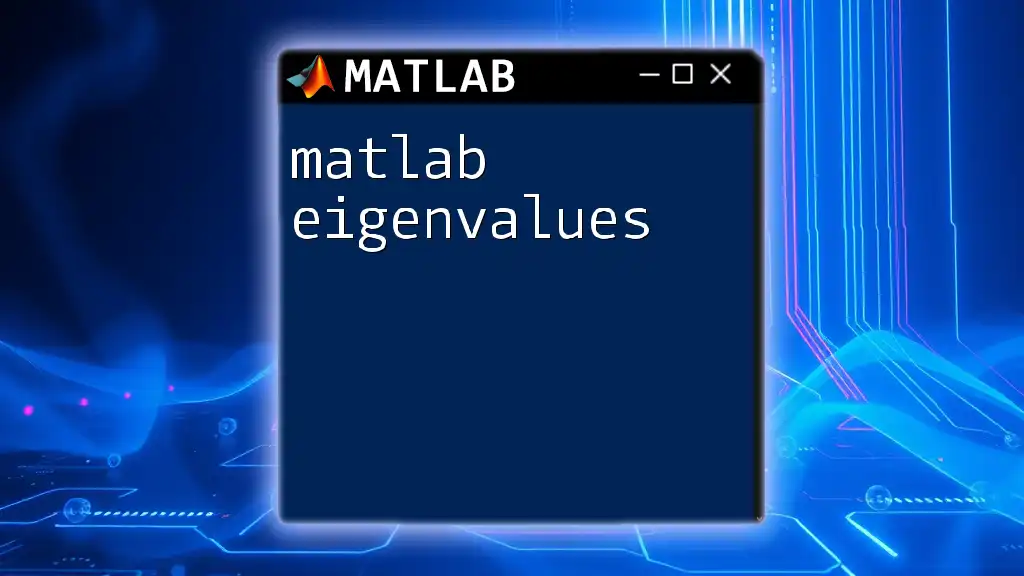
Getting Started with App Designer
Setting Up Your Environment
Before diving into MATLAB App Designer, ensure that you have the correct installation requirements. App Designer comes with recent versions of MATLAB, so make sure you have the latest release. You can access App Designer from the Home tab by selecting "App Designer" under the "Apps" section.
Navigating the Interface
Understanding the layout of App Designer is crucial for efficient app development. The interface consists of several key areas:
- Component Library: Here, you can find all the UI components available for use. Drag components from this library into your design area.
- Design Area: This is where you arrange your UI components visually.
- Code View: Provides access to the underlying code of your app, where you can write and edit callback functions.
- Inspector: A pane that allows you to modify properties of selected UI components, such as labels, colors, and size.
Each section plays a vital role in the development process, allowing you to design visually and code the functionality needed for your app.
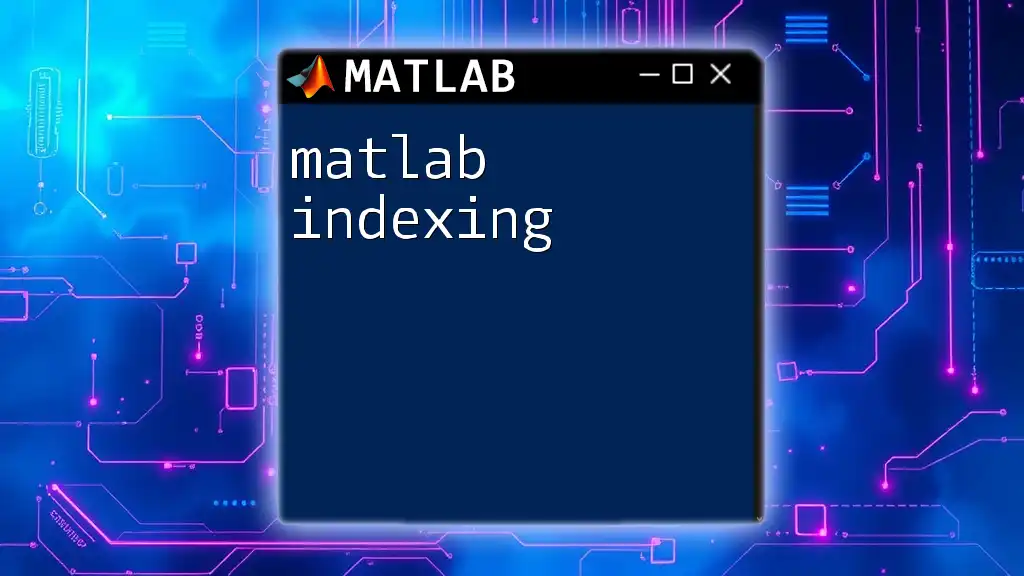
Building Your First App
Creating a New App
To start creating an app, simply open App Designer, and you’ll be prompted to create a new layout. Follow this step-by-step guide:
- Click on "New" and select "App" from the options.
- A blank app environment opens where you can begin designing.
Once your app interface is created using the design area, you can utilize MATLAB code to add functionality.
% Example code snippet for creating a new app
app = uifigure; % Creating a figure for the app
Adding UI Components
Choosing the Right Components
Choosing the right UI components is essential to create a functional app. Common choices include:
- Buttons: For user actions.
- Sliders: To allow users to adjust parameters.
- Dropdowns: For selection options from predefined lists.
Simply drag the desired component from the Component Library onto the Design Area.
Customizing Components
Once you've added components, you can customize their appearance and functionality using the Inspector. For example, you can change a button’s label and background color. To modify the button text programmatically, refer to the following example:
% Example of changing button text in the code view
app.MyButton.Text = 'Click Me';
Organizing Layout
A well-structured layout enhances user experience. You can organize components using:
- Grid Layout: This helps in aligning components harmoniously.
- Absolute Positioning: Allows free positioning of components but may affect responsiveness.
By thoughtfully placing components, you ensure that your app is user-friendly and visually appealing.
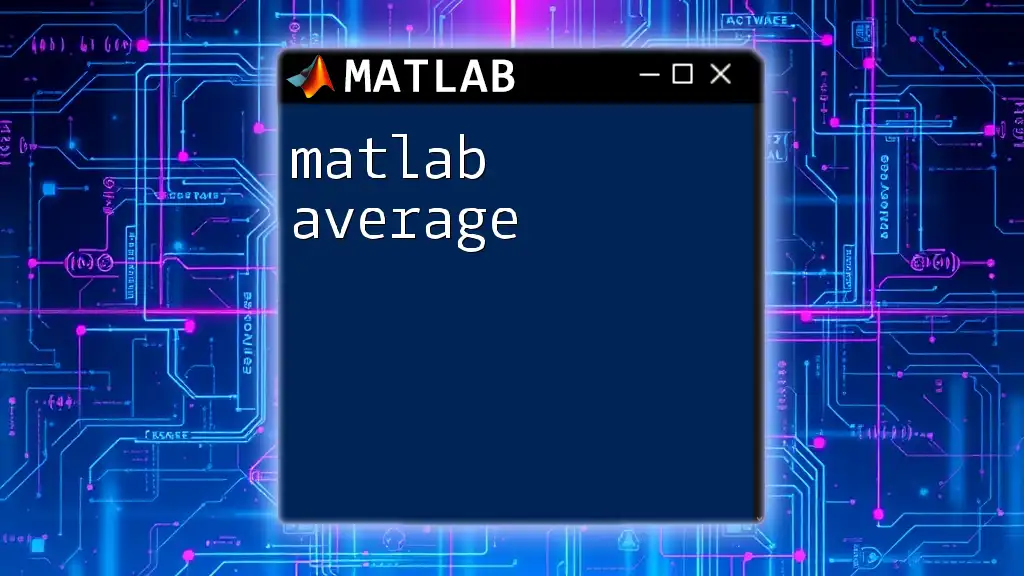
Coding Your App
Introduction to Callbacks
Callbacks are crucial in App Designer, as they define the behavior of your app based on user interactions. When an event (like a button click) occurs, the callback associated with that event is executed, thus allowing your app to react dynamically.
Writing Callback Functions
To create interactivity, you need to write callback functions for your UI components. Each component can have specific callbacks. Here’s an example of a callback for a button that displays a message:
% Callback function example
function MyButtonPushed(app, event)
uialert(app.UIFigure, 'Button was pressed!', 'Alert');
end
This function creates an alert when the button is pressed, adding interactivity to your app.
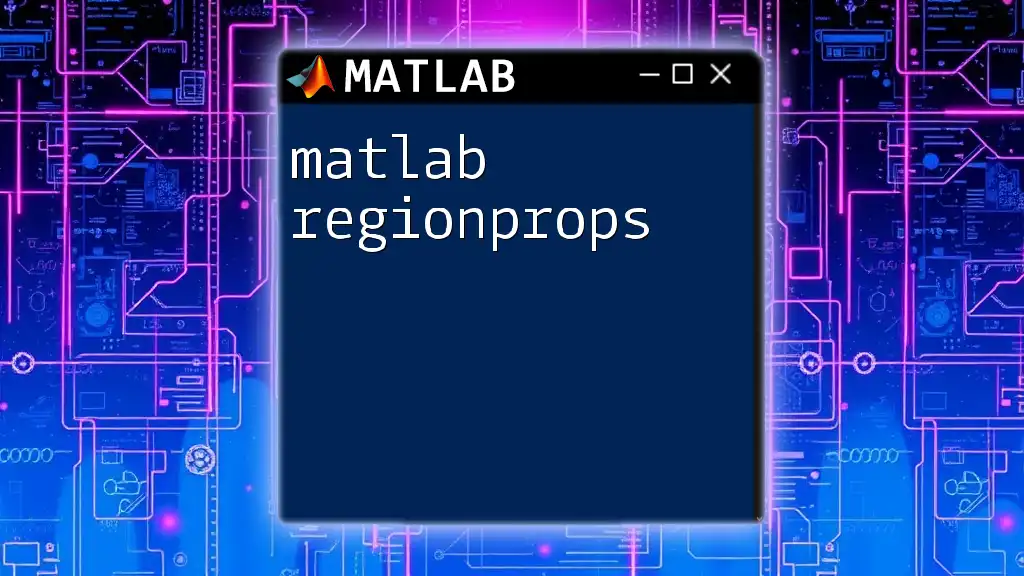
Advanced Features
Adding Data Visualizations
Integrating data visualizations can tremendously enhance the functionality of your app. MATLAB provides a variety of plotting functions that can be easily included in your app. For instance, you can add a simple plot as follows:
% Example of plotting in an app
plot(app.UIAxes, x, y); % Where app.UIAxes is an axes component in the App
This allows users to visualize data dynamically based on their input.
Incorporating App Interactivity
Beyond basic interactions, you can use input fields to collect user data and update the UI accordingly. For example, if you have a slider, you might want the plot to update as the user moves the slider. Here’s how you can manage that:
% Example of updating plot based on user input
function MySliderChanged(app, event)
val = app.MySlider.Value;
plot(app.UIAxes, x, val * y);
end
This approach creates a more engaging experience where users can see the immediate effect of their inputs.
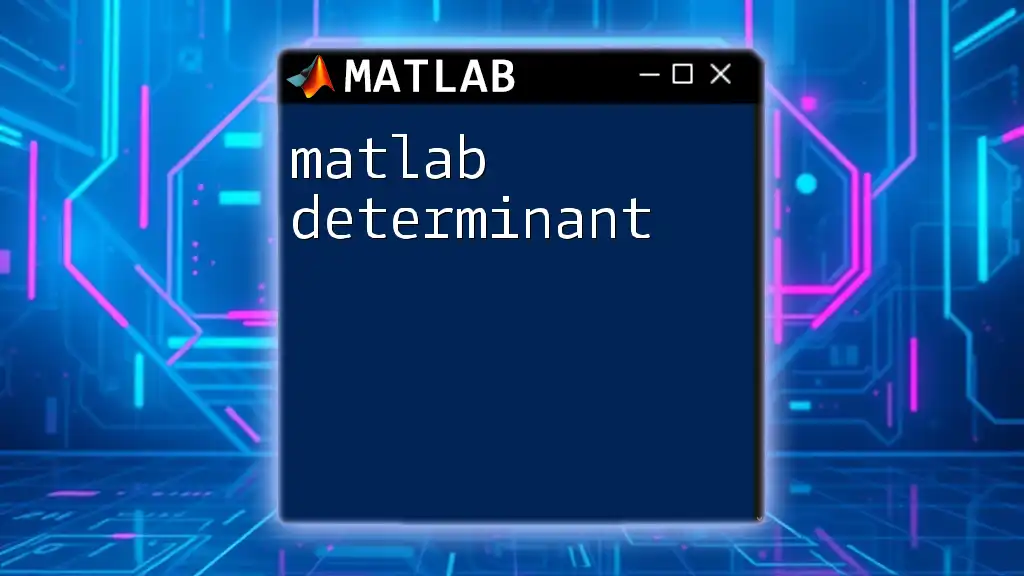
Testing Your App
Importance of Testing
Testing is a fundamental aspect of app development. It ensures that your app operates reliably and meets user expectations. You can conduct internal tests by running your app directly in App Designer.
Running Your App
To run your app, simply press the Run button located at the top of the App Designer environment. This allows you to immediately see your app in action and test its functionality, making it easier to identify and correct any issues.
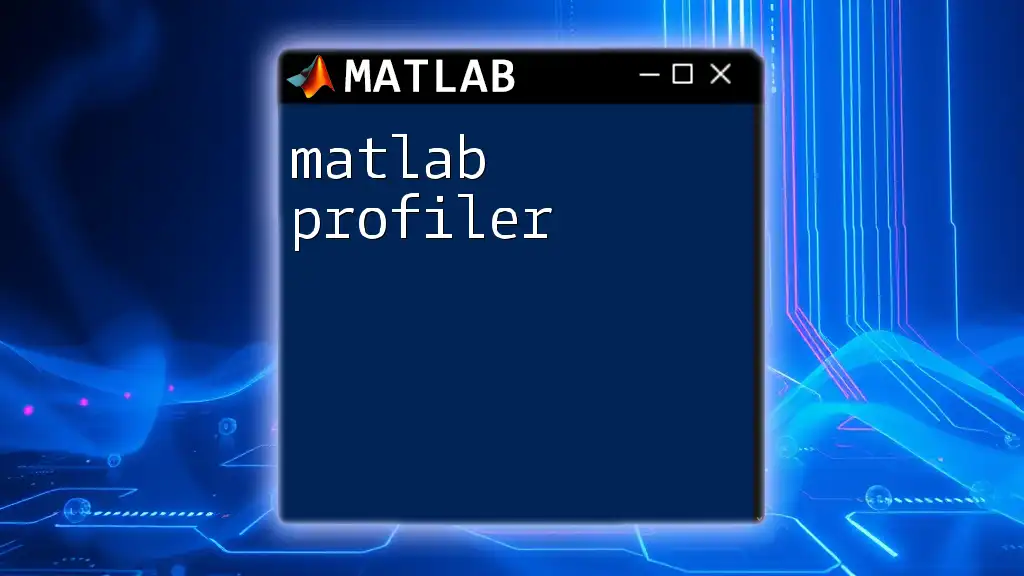
Deploying Your App
Sharing Your App
Once your app development is complete, you have several options to share it. You can use the MATLAB Compiler to package your app for distribution. This allows users without MATLAB to run your app as a standalone application.
Best Practices for App Deployment
To ensure effective deployment:
- User-Friendly Designs: Consider the layout and usability; users should easily navigate your app.
- Documentation and Support: Provide clear instructions and troubleshooting information to assist users.
By following these best practices, you ensure a smooth app experience for your end-users.
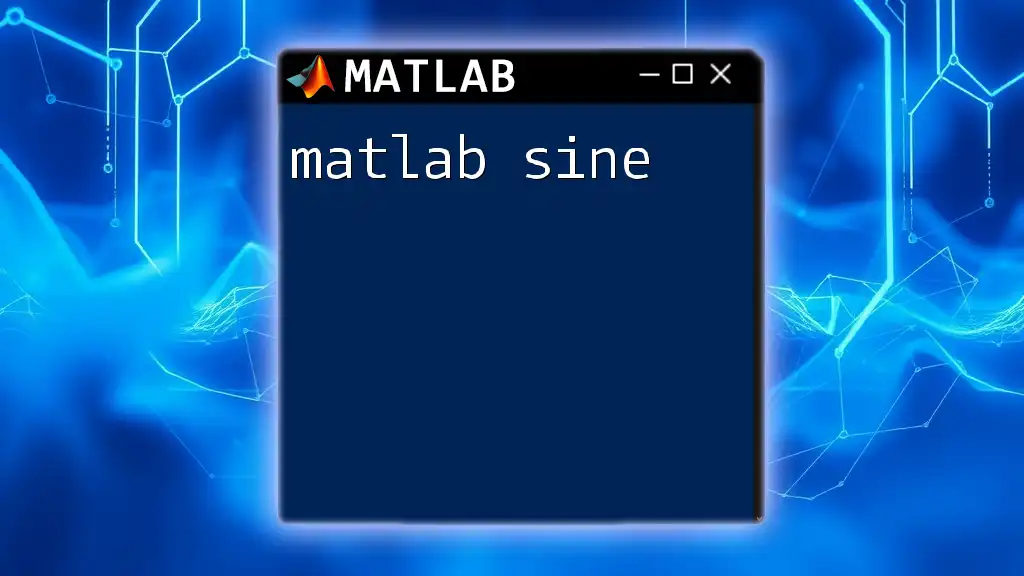
Conclusion
In this guide, we have explored the key components of MATLAB App Designer, from basic setup to advanced features. Understanding how to effectively utilize App Designer can significantly expand your capabilities, enabling you to create powerful applications that enhance data analysis and visualization. We encourage you to practice using the features discussed and constantly experiment to unlock the full potential of MATLAB App Designer. Join our classes or tutorials to deepen your learning and transform your ideas into functional applications!
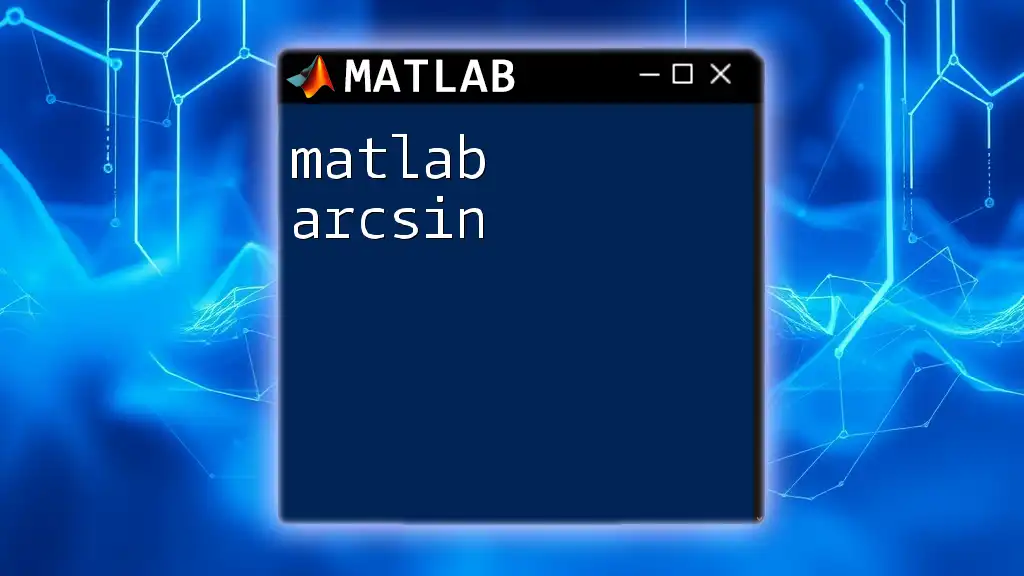
Resources
To continue your learning journey, explore the official MATLAB documentation on App Designer, attend recommended webinars, or participate in community forums for support. Engaging with these resources will help you refine your skills and connect with other MATLAB enthusiasts.