MATLAB GUI (Graphical User Interface) allows users to create visual applications for data analysis, control systems, and simulations with a user-friendly interface.
Here’s a simple example of creating a MATLAB GUI with a push button:
function simple_gui
f = figure('Position', [100, 100, 300, 200]);
btn = uicontrol('Style', 'pushbutton', 'String', 'Click Me', ...
'Position', [100, 80, 100, 40], ...
'Callback', @button_callback);
end
function button_callback(~, ~)
disp('Button clicked!');
end
Understanding MATLAB GUI
What is a GUI?
A Graphical User Interface, commonly known as GUI, provides a visual way for users to interact with software. Unlike traditional command-line interfaces, which require users to type commands, GUIs allow users to click on buttons, sliders, and other elements, making it easier to use for individuals who may not be familiar with programming. The benefits of using GUIs in programming include:
- Ease of Use: GUIs enable users to navigate applications intuitively.
- Visual Feedback: Users get immediate visual responses to their actions.
- Accessibility: GUIs can be more accessible for those without technical backgrounds.
The Role of MATLAB in GUI Development
MATLAB provides a robust environment for creating GUIs, making it an excellent choice for engineers and scientists. Using MATLAB GUIs, you can build applications that facilitate simulations, data visualization, and user interaction. For instance, you might use a MATLAB GUI to develop a data analysis tool that allows users to input parameters and visualize results dynamically.
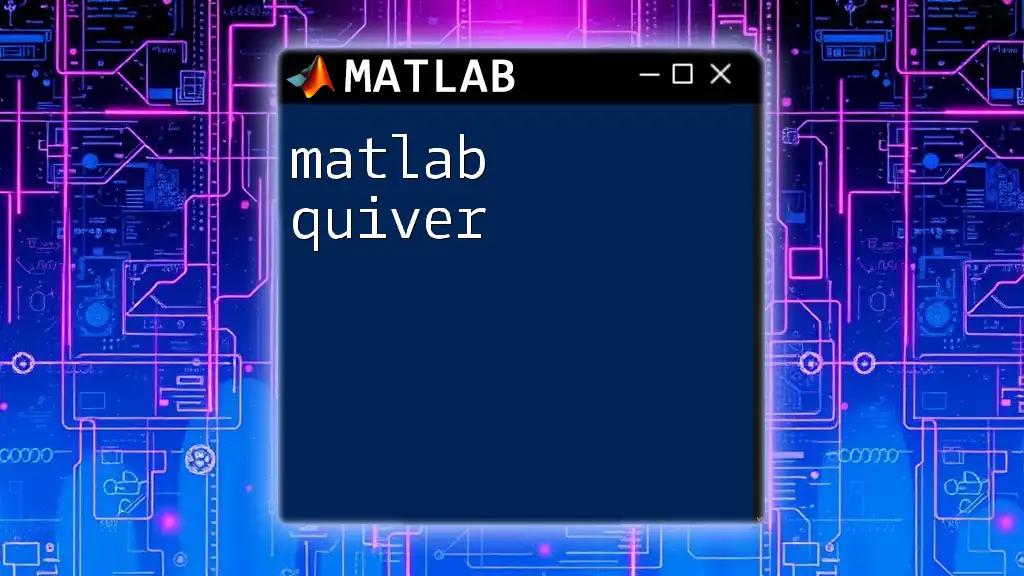
Getting Started with MATLAB GUI
Installing MATLAB and Toolboxes
Before you can start creating GUIs, you will need to have MATLAB installed along with the necessary toolboxes. The App Designer and GUIDE (Graphical User Interface Development Environment) are essential for building interfaces. To install MATLAB:
- Download MATLAB from the official MathWorks website.
- Follow the installation instructions and ensure you select the App Designer toolbox.
Basics of GUI Components
Common GUI Elements
When designing a GUI in MATLAB, there are several components you will commonly employ:
- Buttons: Used to trigger actions—these can be simple commands or complex functions.
- Sliders: Allow users to adjust variables easily by sliding a control along a range.
- Text Boxes: Useful for displaying or collecting text data.
- Panels: Help you organize components logically, enhancing usability.
Layout Management
Well-designed GUIs effectively manage layout to improve user experience. MATLAB provides various layout options, such as grid layouts and absolute positioning. Here is an example of how to create a simple layout using a grid:
% Create a simple grid layout
f = uifigure;
g = uigridlayout(f, [2, 2]);
% Create components
btn1 = uibutton(g, 'Text', 'Button 1');
btn2 = uibutton(g, 'Text', 'Button 2');
slider1 = uislider(g);
txtField = uitextarea(g);
Creating Your First GUI
Step-by-Step Guide
To create your first MATLAB GUI using App Designer, follow this process:
- Open App Designer: Launch MATLAB and click on the App Designer icon.
- Initialize a New Project: Start a new app using the blank app template.
- Add Components: Drag and drop components like buttons and sliders onto the canvas.
Example Project: Simple Calculator GUI
Let’s say we want to create a simple calculator that can add two numbers. Here’s a basic structure of the code for our GUI:
% Create the main UI figure
fig = uifigure('Position', [100 100 300 200]);
% Create UI components
num1 = uieditfield(fig, 'numeric', 'Position', [50 150 100 22]);
num2 = uieditfield(fig, 'numeric', 'Position', [150 150 100 22]);
result = uilabel(fig, 'Position', [100 100 100 22], 'Text', 'Result');
calcButton = uibutton(fig, 'Text', 'Calculate', ...
'Position', [100 50 100 22]);
% Button callback function
calcButton.ButtonPushedFcn = @(src, event) calculateResult(num1.Value, num2.Value, result);
In this code snippet, we set up two edit fields for input, a label to display the result, and a button to trigger the calculation. The calculation logic is encapsulated in the `calculateResult` function that you will define based on your application’s requirements.
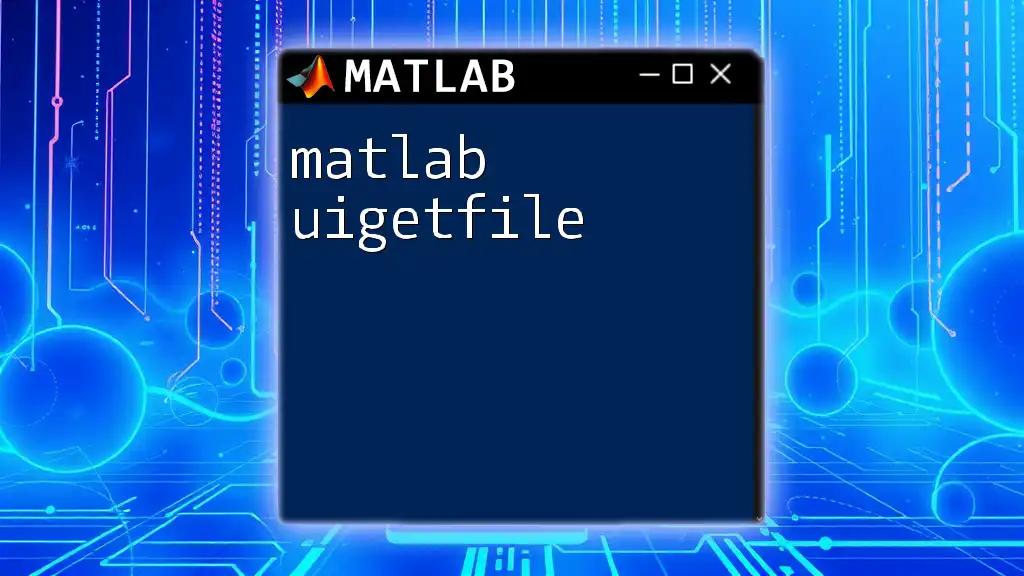
Advanced Features of MATLAB GUI
Customizing the Appearance
Using Properties
Customizing the look of your GUI can greatly enhance usability. In MATLAB, each component has properties like Color, FontSize, and Text that you can modify. For example, to change the background color and the font size of a button, you could use:
btn.BackgroundColor = 'blue';
btn.FontSize = 14;
Adding Icons and Images
Visual elements like icons and images can improve the aesthetics of your GUI. To load and display an image, you can use:
img = imread('image.png');
imageHandle = uiimage(fig, 'ImageSource', img, 'Position', [10 10 100 100]);
Event Handling
Understanding Callbacks
Callbacks are functions that execute in response to user actions, such as clicking a button. They are crucial for creating interactivity in your GUI.
Example: Button Click Events
Here's how to create a callback for the calculator button:
function calculateResult(value1, value2, resultLabel)
total = value1 + value2;
resultLabel.Text = sprintf('Result: %.2f', total);
end
This function takes the two numbers entered by the user, computes the sum, and updates the label displaying the result.
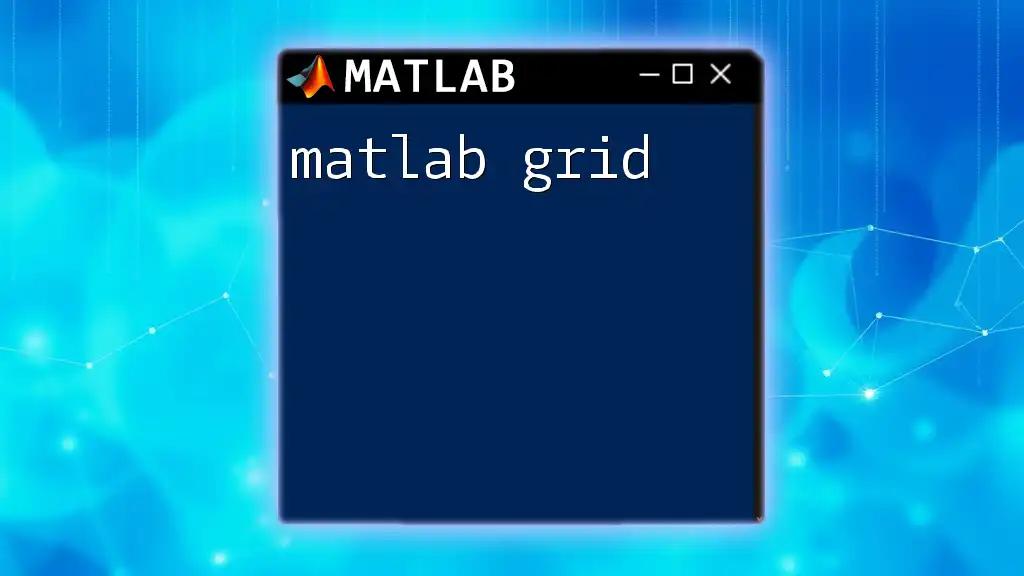
Debugging and Testing Your GUI
Common Issues and Solutions
While developing your MATLAB GUI, you may encounter various issues such as unresponsive buttons or incorrect displays. Common problems often stem from callback misconfigurations or issues with component properties. Always double-check your event functions and available properties.
Tools for Testing
MATLAB offers built-in debugging tools that allow you to step through your application line-by-line. Utilize breakpoints to examine variable states and ensure that your GUI behaves as expected. Regular testing during development can save time and frustration later.
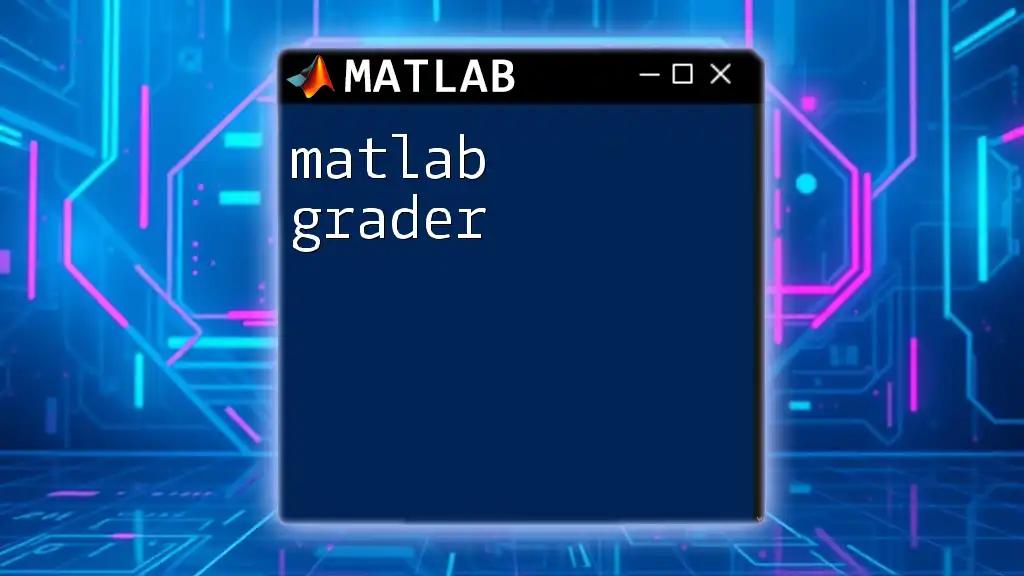
Deploying Your MATLAB GUI
Sharing Your GUI
Once you've created a MATLAB GUI, you may want to share it with others. MATLAB provides options for packaging your application for users who do not have MATLAB installed, which is essential for wider distribution.
Packaging Your GUI Application
To package your GUI application for deployment:
- Use MATLAB Compiler: This tool allows you to create standalone applications.
- Configure export settings according to your target audience's requirements, ensuring all necessary files and dependencies are included.
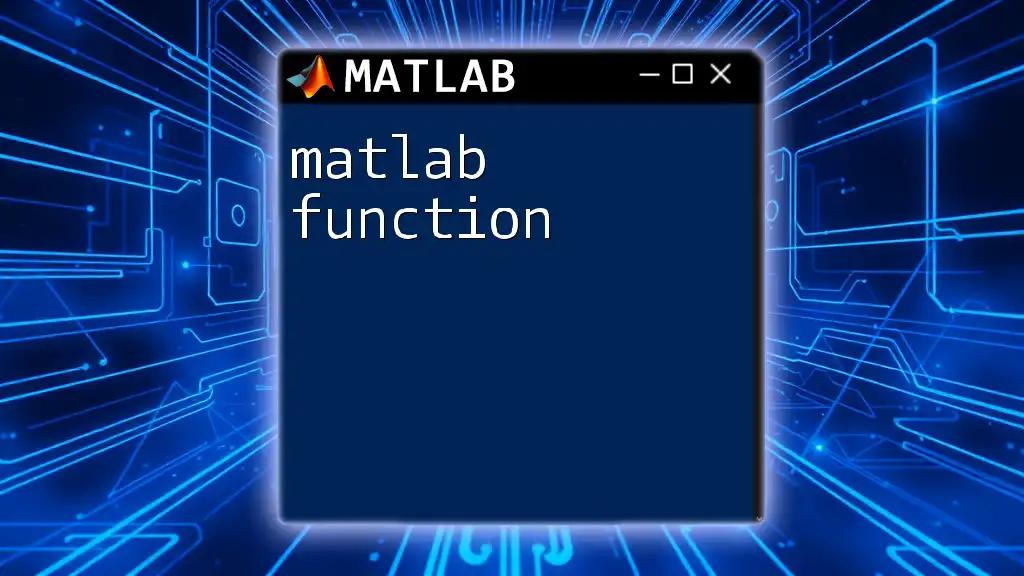
Conclusion
In this comprehensive overview on creating a MATLAB GUI, we explored the foundations of GUI components, the process of creating basic and advanced interfaces, and ways to debug, test, and deploy your applications. MATLAB GUIs are powerful tools that can greatly enhance user interaction and data manipulation capabilities. With practice and creativity, you can develop advanced applications tailored to your specific needs.
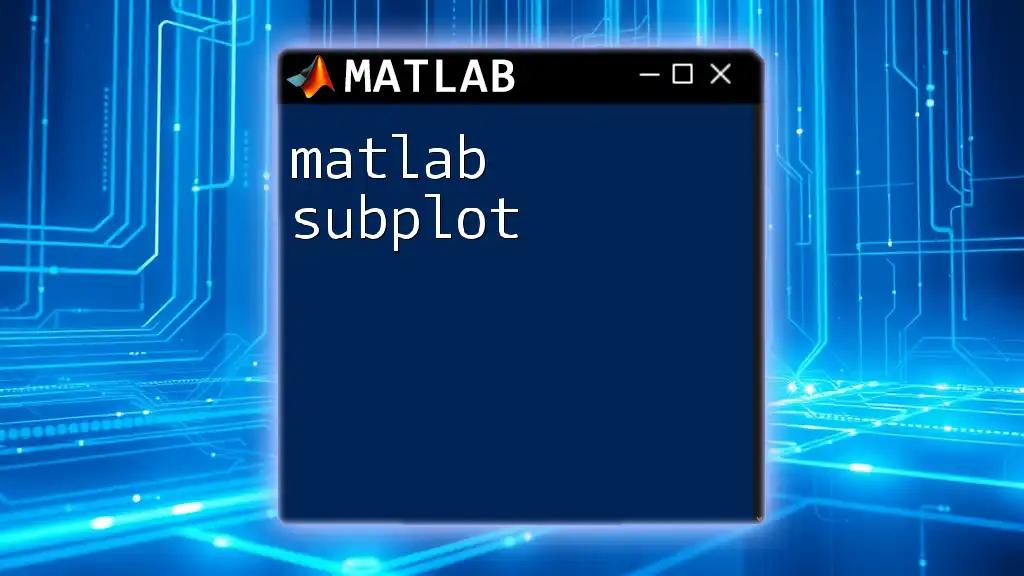
Call to Action
If you are interested in deepening your understanding and skills in MATLAB GUI, consider joining a workshop or reaching out for personal consultation. For further resources and tutorials, stay engaged with our content, as we continuously update our platform with the latest insights on MATLAB development.