The `if` statement in MATLAB allows you to execute a block of code conditionally based on whether a specified logical condition evaluates to true.
Here's a simple example of how to use the `if` statement in MATLAB:
x = 5;
if x > 0
disp('x is positive');
end
Understanding Conditional Statements in MATLAB
What is a Conditional Statement?
A conditional statement is a fundamental concept in programming that allows your code to make decisions based on certain conditions. Conditional statements check whether a specific condition is true or false and control the flow of execution accordingly. In MATLAB, conditional statements help to create dynamic and responsive scripts that can adapt to various data inputs and scenarios.
Why Use `if` Statements?
The `if` statement is one of the most widely used conditional statements in MATLAB. It provides a way to execute specific blocks of code based on whether a condition evaluates to true. This flexibility is vital in numerous applications, such as:
- Data analysis: Dynamically adjusting processing based on the values of input data.
- Algorithm implementation: Implementing decision-making logic in algorithms, such as searching or sorting.
- User input handling: Tailoring responses to the user's choices during script execution.
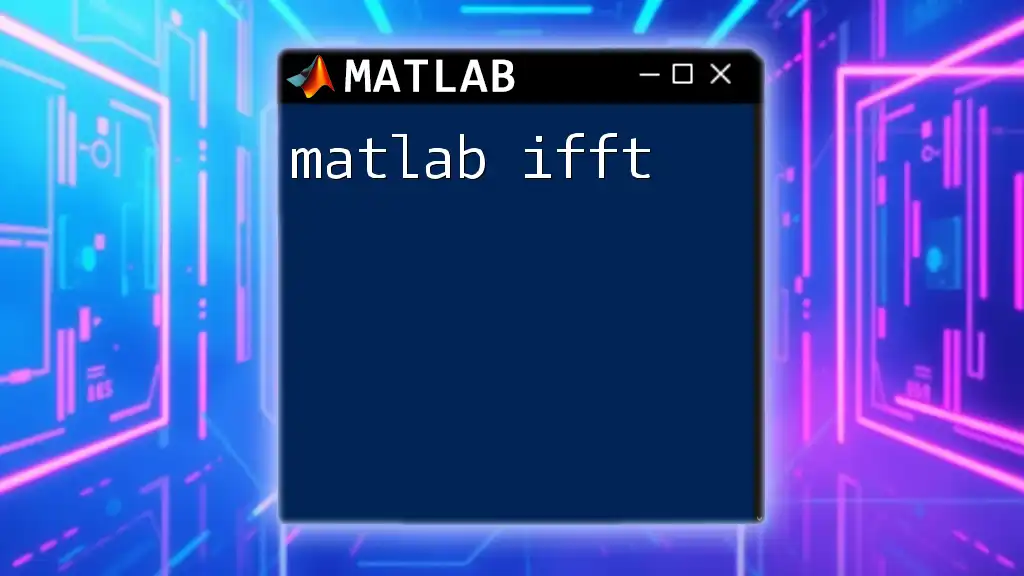
The Basic Syntax of `if` Statements in MATLAB
Structure of an `if` Statement
The basic syntax for an `if` statement in MATLAB is as follows:
if condition
% Code to execute if condition is true
end
Components of the Syntax
-
Condition: This is a logical expression that MATLAB evaluates. It can involve comparisons (e.g., `x > 5`, `y == 10`) and must return a true or false value.
-
Code block: This part contains the commands that will be executed only if the condition evaluates to true. Without any commands here, the `if` statement is effectively empty when the condition is met.
-
End statement: The `end` keyword is crucial as it indicates the termination of the `if` block, marking where the flow of execution will continue if the condition is not met.
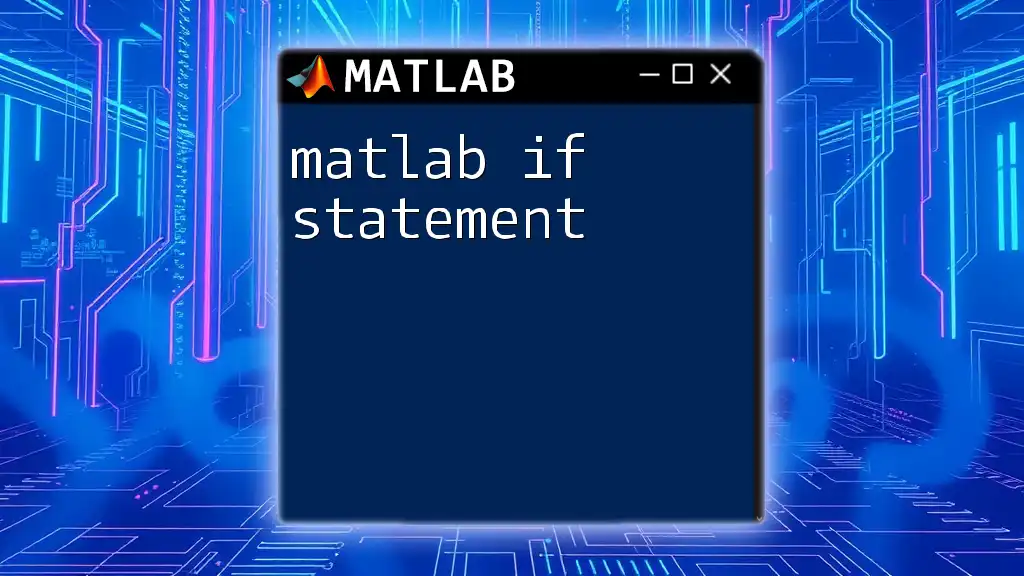
Types of `if` Statements in MATLAB
Simple `if` Statement
A simple `if` statement executes code only when a specific condition is true. For example:
x = 10;
if x > 5
disp('x is greater than 5');
end
In this example, since the value of `x` is indeed greater than 5, the code block inside the `if` statement executes, outputting "x is greater than 5".
`if-else` Statements
An `if-else` statement provides an additional pathway for execution when the initial `if` condition is false. The syntax is as follows:
if condition
% Code for true condition
else
% Code for false condition
end
For instance:
x = 3;
if x > 5
disp('x is greater than 5');
else
disp('x is 5 or less');
end
In this case, since `x` is not greater than 5, the output will be "x is 5 or less". This usage allows for more flexible decision-making in your scripts.
`if-elseif-else` Ladder
The `if-elseif-else` structure is employed when multiple conditions need to be evaluated. It allows for a sequence of conditions to be checked, executing the first true condition’s block. The syntax is outlined below:
if condition1
% Code for condition1
elseif condition2
% Code for condition2
else
% Code if none of the conditions are true
end
For example:
score = 85;
if score >= 90
grade = 'A';
elseif score >= 80
grade = 'B';
else
grade = 'C';
end
disp(['Grade: ', grade]);
In this example, `score` is evaluated against multiple cutoffs to determine the corresponding grade. As `score` is 85, it outputs "Grade: B".
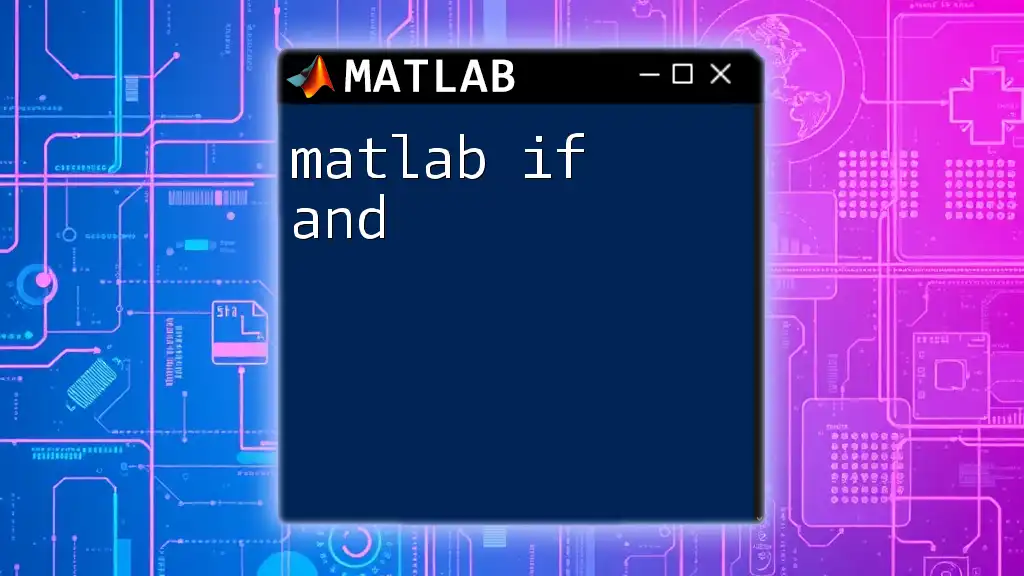
Nested `if` Statements
What are Nested `if` Statements?
Nested `if` statements occur when an `if` statement is placed within another `if` block. This allows for more complex decision-making, where you can refine your conditions even further.
Example of a Nested `if`
Consider the following example:
num = 10;
if num > 0
disp('Positive number');
if mod(num, 2) == 0
disp('Even number');
else
disp('Odd number');
end
end
In this case, the outer `if` checks if `num` is positive. If true, it prints "Positive number" and enters another `if` statement to check whether the number is even or odd. This structure offers a powerful way to handle multiple layers of logic.
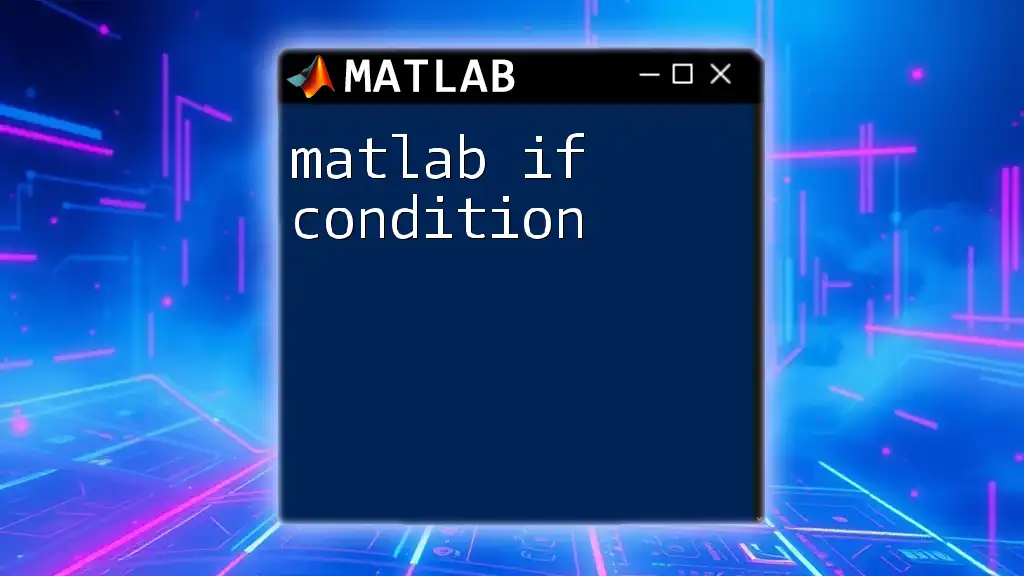
Conclusion
Summarizing Key Points
The `if` statement in MATLAB is a crucial tool for controlling the flow of execution in scripts. Understanding how to effectively implement simple, `if-else`, and nested `if` statements can enhance the functionality of your programming skills and allow for more dynamic and responsive code.
Further Reading and Resources
To deepen your knowledge about the `if` statements and other MATLAB functionalities, it's beneficial to explore the official MATLAB documentation and various MATLAB communities online, where you can find additional examples and discussions.
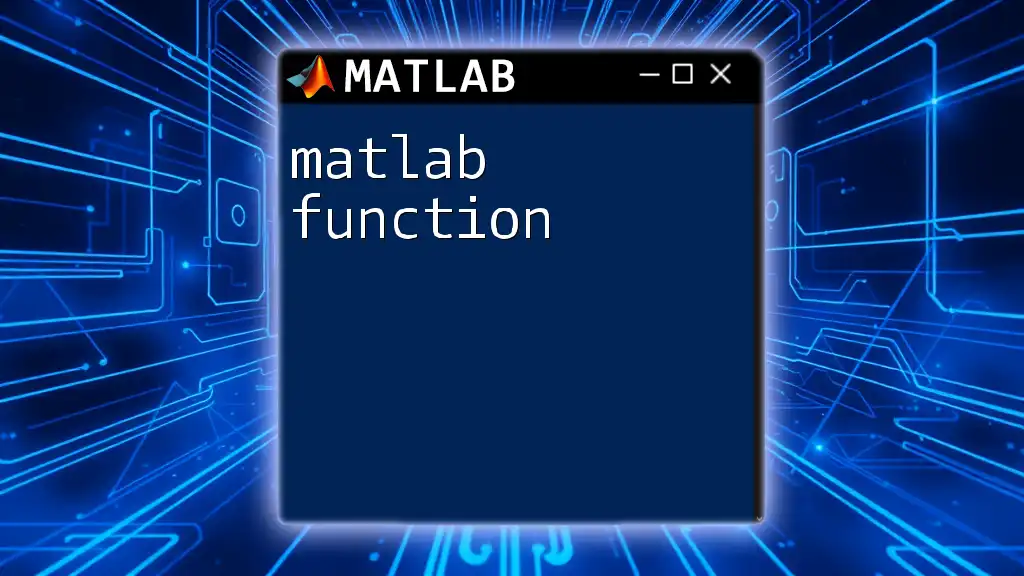
Call to Action
For those eager to advance their MATLAB skills further, we invite you to explore our MATLAB courses designed for quick and concise learning. Discover how to master MATLAB commands, including conditional statements, for effective programming!