The MATLAB `if` statement allows you to execute code conditionally based on whether a specified condition is true, and the `&&` operator can be used to check multiple conditions simultaneously.
Here's a code snippet demonstrating the use of `if` with `&&`:
a = 5;
b = 10;
if a > 0 && b > 0
disp('Both a and b are positive numbers.');
end
Understanding Conditional Statements in MATLAB
What is a Conditional Statement?
In programming, conditional statements enable the execution of certain code based on whether a condition evaluates to true or false. This allows for decision-making in code, enabling the program to act differently under varying circumstances. In MATLAB, the primary conditional statement is the `if` statement, which can be utilized alongside logical operators such as AND to create more complex conditions.
The Syntax of the If Statement
The basic syntax of an `if` statement in MATLAB is as follows:
if condition
% Code to execute if the condition is true
end
When the specified condition evaluates to true, the code within the `if` block will execute. If it is false, the code is bypassed.
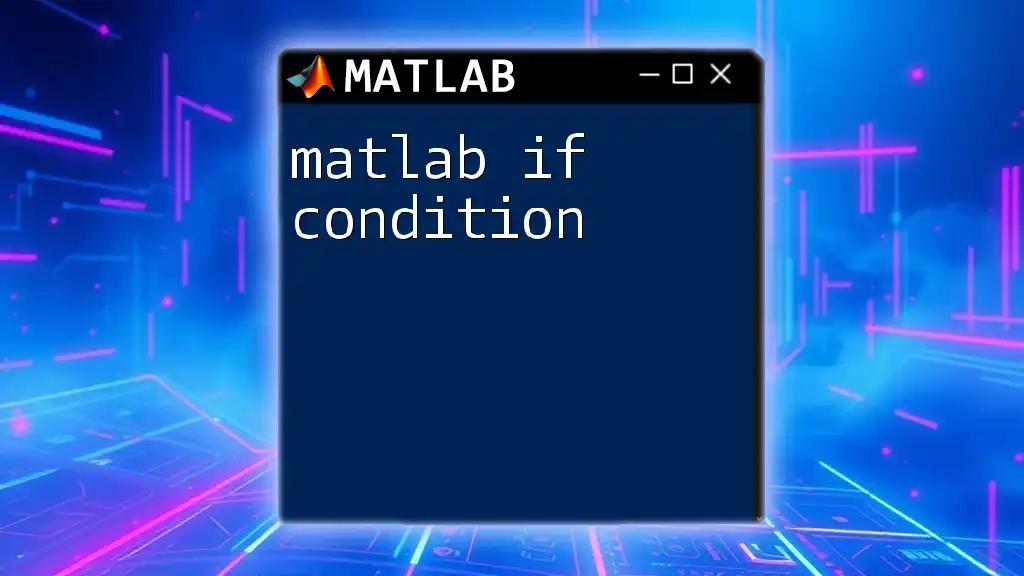
Using the If Statement in MATLAB
Simple If Statement
A simple `if` statement checks a single condition. For example, if we want to check if a variable `x` is greater than 5:
x = 10;
if x > 5
disp('X is greater than 5');
end
In this case, since 10 is greater than 5, the output will be "X is greater than 5."
If-Else Statement
When you want to perform an action based on whether a condition is true or false, you can use the `if-else` statement. The structure looks like this:
if condition
% Code to execute if the condition is true
else
% Code to execute if the condition is false
end
For instance:
x = 3;
if x > 5
disp('X is greater than 5');
else
disp('X is 5 or less');
end
Here, since 3 is not greater than 5, the output will be "X is 5 or less."
Nested If Statements
You can nest `if` statements within each other to create more complex decision trees. This is especially useful for checking multiple layers of conditions.
For example:
x = 7;
if x > 5
disp('X is greater than 5');
if x > 10
disp('X is also greater than 10');
end
end
In this scenario, only the first message will be printed, since 7 is greater than 5 but not greater than 10.
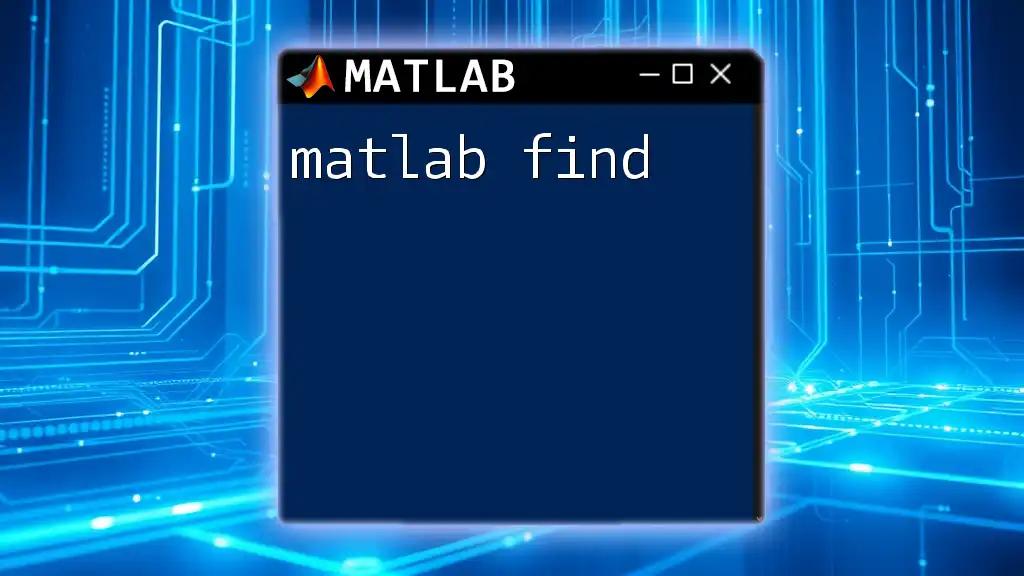
Introduction to Logical Operators
The Role of Logical Operators
Logical operators, such as AND, OR, and NOT, are fundamental in programming, especially for combining multiple conditions. These operators help create complex logical expressions that dictate the flow of the program based on multiple criteria.
The AND Operator in MATLAB
The AND operator is critical in situations where you want all specified conditions to be true. In MATLAB, the AND operator can be represented as short-circuit (`&&`) or element-wise (`&`) depending on the context.
The Short-Circuit AND Operator (&&)
The short-circuit AND operator (`&&`) evaluates the second condition only if the first condition is true. This is useful for improving efficiency in scenarios where the second condition might cause errors if evaluated alone.
For example:
x = 7;
y = 10;
if (x > 5) && (y > 5)
disp('Both conditions are true');
end
In this case, both conditions are evaluated, and since 7 and 10 are both greater than 5, the message "Both conditions are true" will be displayed.
The Element-wise AND Operator (&)
Conversely, the element-wise AND operator (`&`) checks conditions across arrays or matrices and is useful for comparing corresponding elements.
A = [1, 2, 3];
B = [0, 1, 1];
C = A > 1 & B > 0; % Element-wise comparison
disp(C); % Outputs [0 1 1], indicating where both conditions hold true
This operation will yield a vector indicating for each element if both conditions are satisfied.
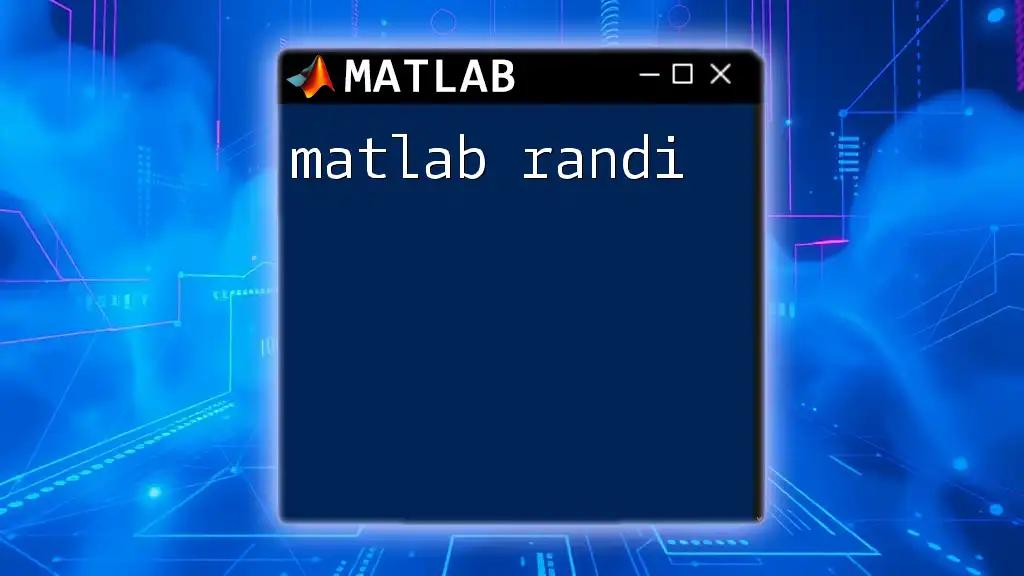
Combining If and AND Statements
Using AND in If Statements
By combining the `if` statement with the AND operator, you can check multiple conditions in a single expression. For instance, if you want to assess both temperature and humidity:
temperature = 75;
humidity = 40;
if (temperature > 70) && (humidity < 50)
disp('Ideal weather conditions!');
end
Here, both conditions are true, leading to the output: "Ideal weather conditions!"
More Complex Conditional Logic
Often, several conditions are involved in decision-making, especially in applications like eligibility checks. An example of this might look like:
age = 25;
income = 50000;
if (age > 18) && (income > 40000)
disp('Eligible for the loan');
else
disp('Not eligible for the loan');
end
In this case, if the age is above 18 and the income exceeds 40,000, the individual is deemed eligible for the loan.
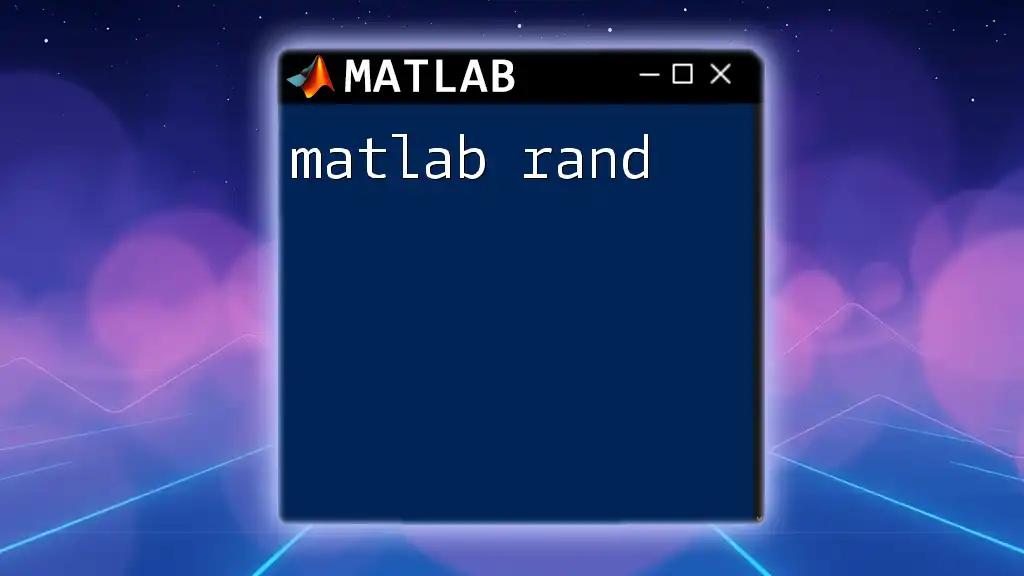
Common Mistakes with If and AND in MATLAB
Pitfalls to Avoid
One common mistake in using `if` statements with AND is neglecting to properly group conditions with parentheses. For example, the following logic might lead to an unexpected result:
x = 10;
if x > 5 && x < 15 || x == 20
disp('Condition met');
end
Without proper use of parentheses, the program might misinterpret the conditions, leading to logic errors. A corrected version would be:
if (x > 5 && x < 15) || (x == 20)
disp('Condition met');
end
Importance of Parentheses for Clarity
Using parentheses not only avoids errors but also enhances readability by clearly defining the logical structure of your conditions.

Best Practices for Writing If Statements
Readability and Maintainability
When writing `if` statements, employing clear and descriptive variable names is crucial. Rather than using generic names such as `a` or `x`, try to use context-specific names like `temperature` or `userAge`. This practice enhances code readability and maintainability, making it easier for others (and yourself) to understand the logic later on.
Testing Conditions
Always consider testing boundary conditions to ensure your conditions hold true under all scenarios. For example, if your logic checks for values greater than a threshold, test it with exactly that threshold to see how your code behaves.
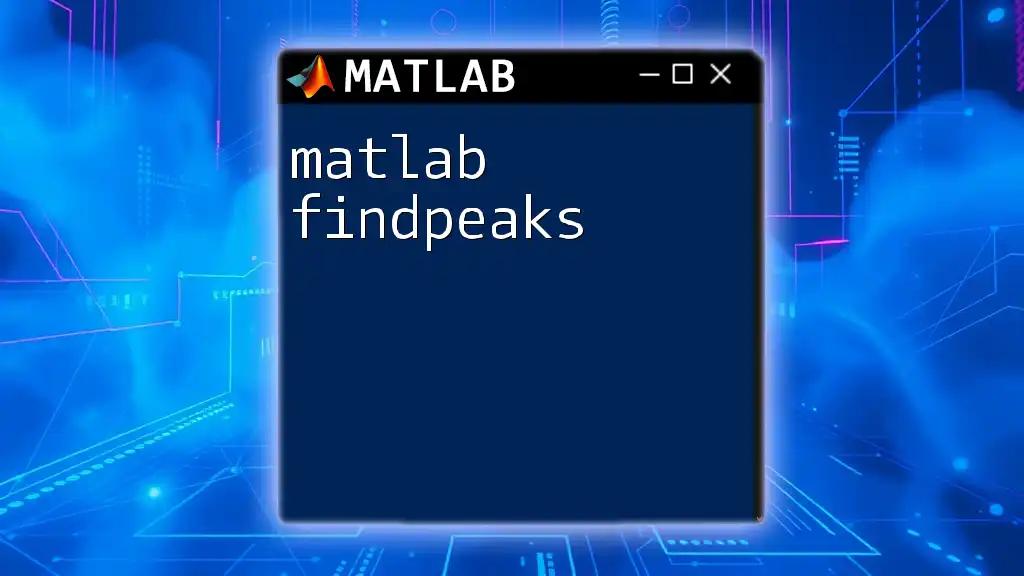
Conclusion
The combination of the `if` statement with the AND operator in MATLAB is a powerful toolkit for creating complex logic flows in your scripts. Understanding how to effectively utilize these tools will enhance your programming skills and allow for efficient decision-making in your code. By practicing and experimenting with these concepts, you can become proficient in using conditional statements in MATLAB.
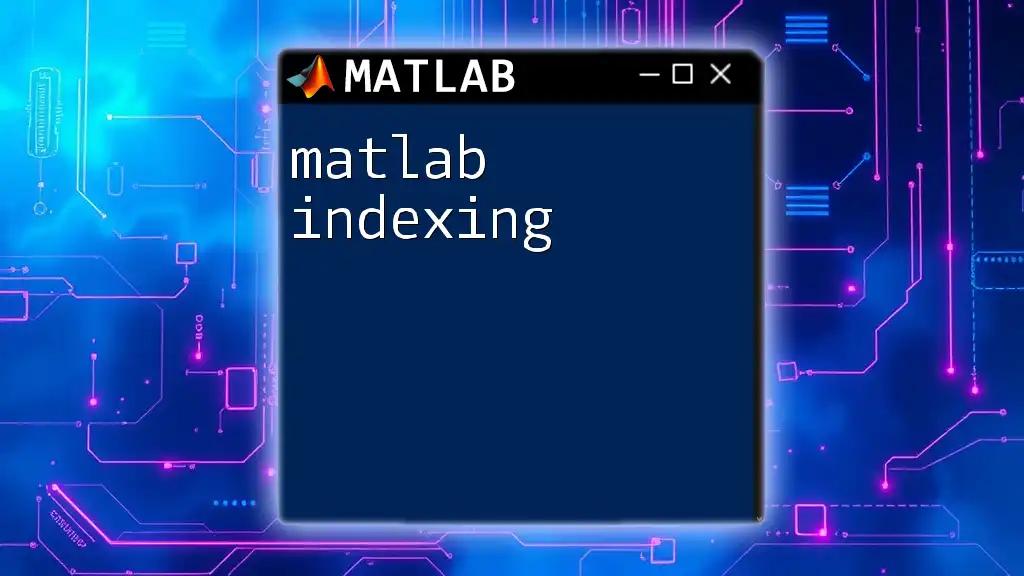
Additional Resources
Further Reading and References
For more detailed information, consider checking the official MATLAB documentation, which offers extensive insights into conditional statements and logical operators. Online tutorials and courses can also provide further practice opportunities.
Practice Exercises
Engage with practical exercises to reinforce your learning. Consider creating your own conditions and testing them against various scenarios to gain a deeper understanding of how MATLAB handles conditional expressions.