The `fcn` function in MATLAB allows you to create a simple function handle for calling functions with specified input arguments, enabling concise and efficient programming.
Here’s a code snippet demonstrating how to create and use a function handle with `fcn`:
fcn = @(x) x^2; % Create a function handle that squares its input
result = fcn(5); % Call the function with an input of 5
disp(result); % Display the result
What is a Matlab Function?
Definition of Functions
A function in programming is a block of reusable code designed to perform a specific task. Unlike scripts, functions can take inputs and return outputs, enabling them to be highly modular and versatile in a broader application.
Key Characteristics of Matlab Functions
Functions in Matlab are characterized by:
-
Input and Output Arguments: Functions can accept parameters (inputs) and return values (outputs). This allows the same function to be used with different data, making it flexible.
-
Function Naming Conventions: To avoid naming conflicts, it's essential to follow a consistent naming convention. Function names should be descriptive and reflect the task they perform, using only alphanumeric characters and underscores.
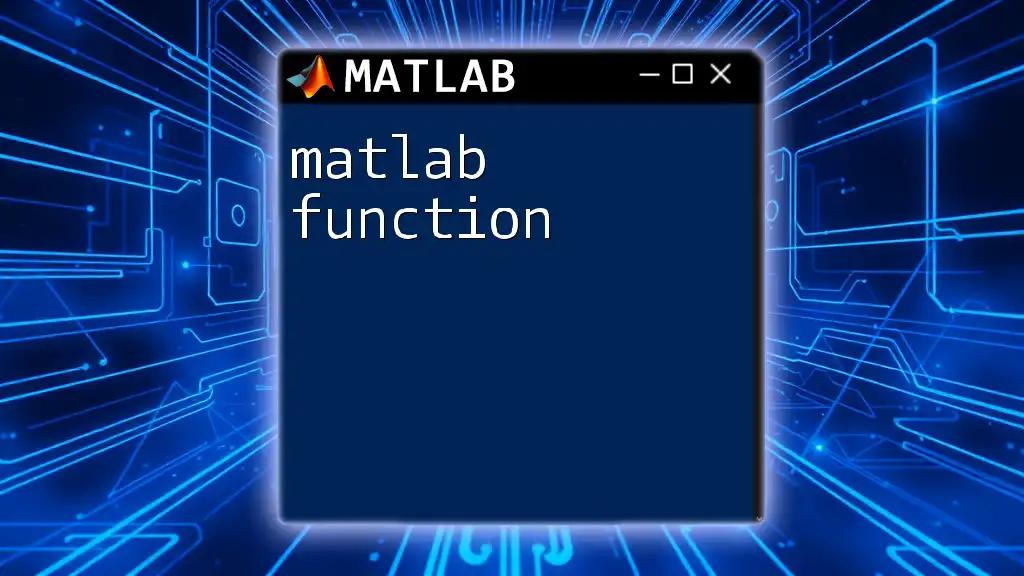
Understanding the 'fcn' Command
What is the 'fcn' Command?
The 'fcn' command is not explicitly defined in Matlab as a dedicated function but serves as a common placeholder to signify function concepts throughout Matlab programming resources. The term often appears when discussing general function-related topics or referring to user-defined functions.
Syntax of 'fcn'
The general syntax for defining a Matlab function follows this structure:
function output = fcn(input)
Components include:
- function: A keyword that initiates the function definition.
- output: The variable(s) that the function will return.
- fcn: The name of the function (this can be any valid identifier).
- input: The variable(s) that the function will accept as parameters.
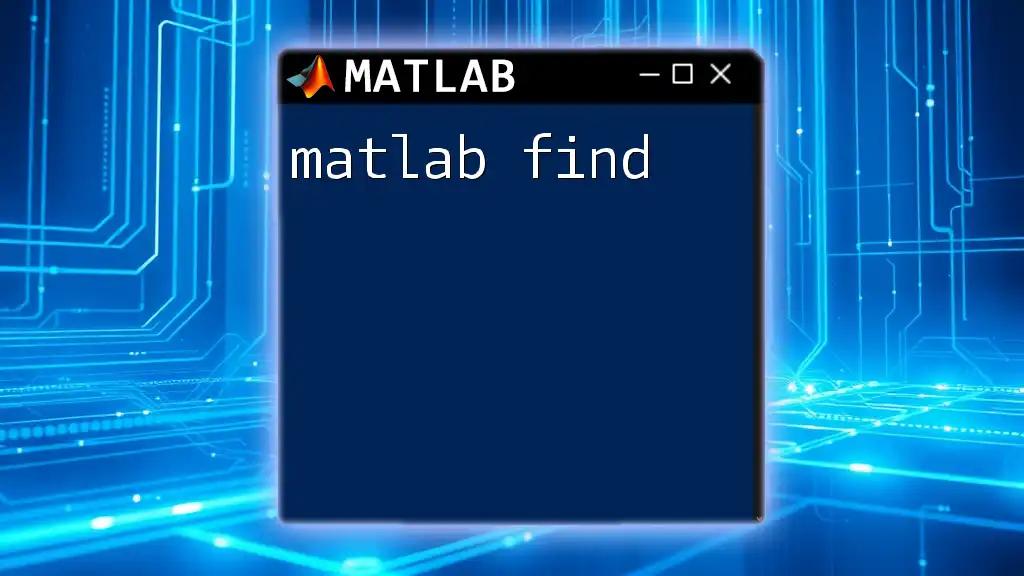
Creating a Simple Matlab Function
Step-by-Step Guide to Creating a Function
- Defining the Function: Start with the `function` keyword.
- Writing the Code: Implement the logic that the function will perform.
- Testing the Function: Ensure it works as expected by calling it with test inputs.
Example: Creating a Function to Calculate Square
To demonstrate the workings of a simple function, consider a function that calculates the square of a number:
function result = squareNumber(num)
result = num^2;
end
In this example, the function `squareNumber` takes a numeric input `num` and returns the square of that number as `result`. Testing this function might involve calling it as follows:
output = squareNumber(4);
disp(output); % This will display 16
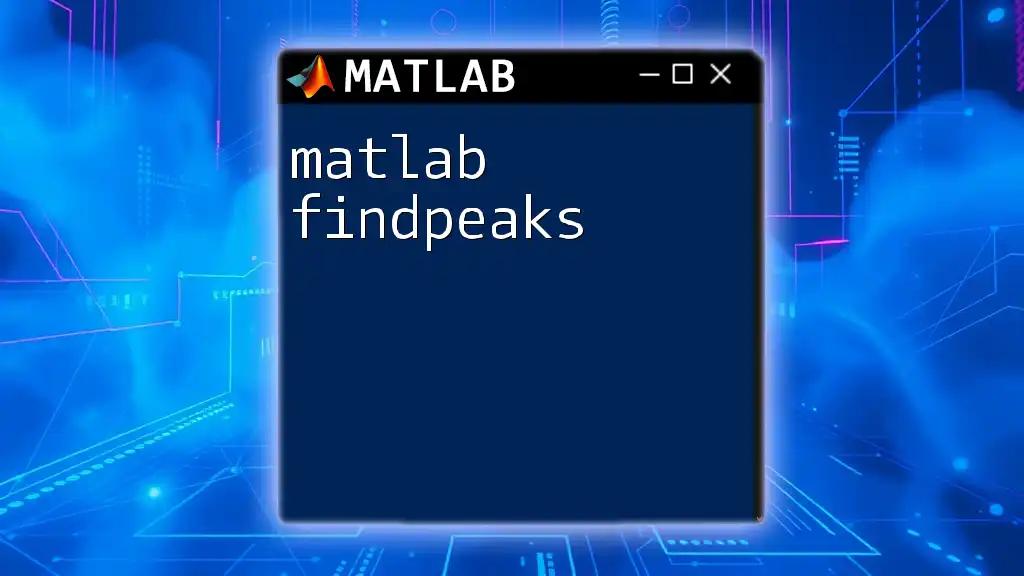
Advanced Usage of Functions
Nested Functions
Nested functions are those defined within another function. They have access to the variables of the outer function, making them useful for specific tasks that are only relevant within that context.
Example:
function outerFunction(x)
function innerFunction(y)
disp(y^2);
end
innerFunction(x);
end
This code defines `outerFunction`, which contains `innerFunction`. Calling `outerFunction(3)` results in displaying `9`, as `innerFunction` calculates the square of `x`.
Anonymous Functions
Anonymous functions are one-liners that are defined without a formal function file. Their simplicity makes them ideal for quick calculations.
The syntax follows this pattern:
name = @(input) expression;
Example:
square = @(x) x^2;
disp(square(5)); % Outputs 25
Here, `square` is defined as an anonymous function that squares its input. This can be used in various contexts such as array operations or with built-in functions like `arrayfun`.
Function Handles
Function handles allow you to reference functions within other functions or pass them as arguments. This increases flexibility, especially in higher-order functions.
Example:
f = @sin; % Create a handle to the sine function
x = 0:0.1:10; % Array of values
y = f(x); % Apply the sine function to x
plot(x, y);
This code snippet creates a function handle for the sine function, applies it to an array of values, and plots the results.

Best Practices for Writing Matlab Functions
Code Readability
Readable code is crucial for maintainability and collaboration. Using clear and descriptive function names, consistent indentation, and logical organization significantly improves the clarity of your code.
Commenting and Documentation
Comments provide context and explanations for your functions, making them easier to understand. Use comments to explain why certain decisions were made, describe parameters, and provide examples of usage.
Optimization
To ensure efficient performance, utilize built-in Matlab functions whenever applicable. They are often more optimized than custom implementations. Additionally, consider vectorizing your code to reduce loops, enhancing speed and performance.

Debugging Functions
Common Errors in Functions
Errors can arise from various sources:
- Syntax errors: Typos or incorrect structure.
- Logic errors: Mistakes in the coding logic that produce incorrect results.
- Runtime errors: Issues that occur when the function is executed, often related to input types or values.
Debugging Techniques
Matlab provides powerful debugging tools, including breakpoints and the workspace window, to help identify and troubleshoot issues within functions. Use breakpoints to pause execution and inspect variables, which can clarify the function's behavior and pinpoint where problems occur.

Conclusion
The 'fcn' concept in Matlab underscores the importance of functions in programming. Functions empower you to write reusable and modular code, enhancing flexibility and maintainability. With a comprehensive understanding of function creation, advanced usage, best practices, and debugging techniques, you’re well on your way to mastering Matlab functions.

Call to Action
Share your experiences with Matlab functions in the comments below. If you’re looking to deepen your understanding, consider enrolling in our specialized training courses to hone your skills in writing efficient and effective Matlab functions.