MATLAB excels at handling matrix operations, allowing users to efficiently perform calculations with high-dimensional data using simple commands.
Here's a basic example of creating a matrix and performing matrix multiplication:
% Create two matrices
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
% Perform matrix multiplication
C = A * B;
Understanding Matrices
What is a Matrix?
A matrix is a rectangular array of numbers, symbols, or expressions, arranged in rows and columns. In MATLAB, matrices serve as the foundation for numerous operations and mathematical models. For example, a simple 2x3 matrix consists of 2 rows and 3 columns, and can be represented as follows:
A = [1, 2, 3; 4, 5, 6];
In this example, `A` has two rows and three columns, with each entry being an element of the matrix.
Types of Matrices
Matrices come in various forms, each serving a unique purpose in computations:
-
Row Matrix: Contains a single row of elements. For example, a 1x4 matrix can be expressed as:
R = [1, 2, 3, 4];
-
Column Matrix: Consists of a single column with multiple elements. A 4x1 matrix can be represented as:
C = [1; 2; 3; 4];
-
Square Matrix: Has an equal number of rows and columns. A common example is a 2x2 matrix:
S = [1, 2; 3, 4];
-
Zero Matrix: Contains all elements as zero. Creating a 3x2 zero matrix can be done using:
Z = zeros(3, 2);
-
Identity Matrix: A special type of square matrix where all diagonal elements are one and all other elements are zero. An example of a 3x3 identity matrix is:
I = eye(3);
-
Diagonal Matrix: A square matrix where all elements outside the main diagonal are zero. For example:
D = [1, 0, 0; 0, 2, 0; 0, 0, 3];
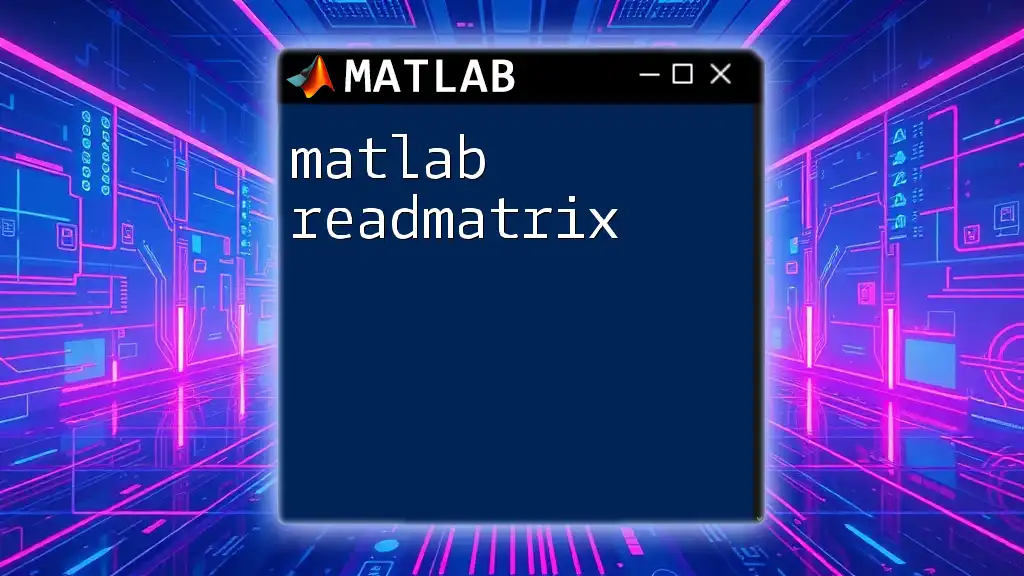
Creating Matrices in MATLAB
Using Brackets
MATLAB utilizes brackets to create matrices directly. You can easily define a matrix by listing the elements within square brackets, separating rows with semicolons and columns with commas. For example, to create a 3x3 identity matrix, you can use:
I = eye(3);
Using MATLAB Functions
You can also create matrices using built-in MATLAB functions:
-
zeros(): Generates a matrix filled with zeros. For instance, to create a 2x3 zero matrix:
Z = zeros(2, 3);
-
ones(): Produces a matrix filled with ones. A 4x2 matrix of ones can be generated with:
O = ones(4, 2);
-
rand(): Creates a matrix with random values uniformly distributed in the interval (0,1). For a 3x3 matrix:
R = rand(3, 3);
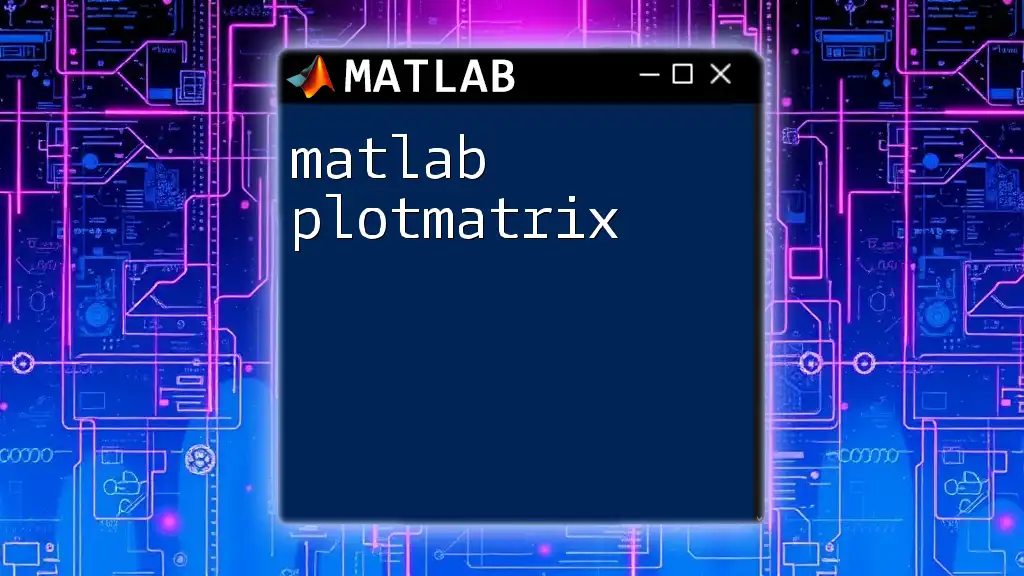
Basic Matrix Operations
Addition and Subtraction
Matrix addition and subtraction require matrices to be of the same dimension. When you add or subtract two matrices, you perform the operation element-wise. Here's an example:
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
C = A + B; % Matrix addition
D = A - B; % Matrix subtraction
Scalar Multiplication
This operation involves multiplying every element of a matrix by a constant (scalar). If you want to multiply matrix `A` by 2, you can do so with:
k = 2;
E = k * A; % Scalar multiplication
Matrix Multiplication
Matrix multiplication is more complex; the number of columns in the first matrix must equal the number of rows in the second. Here’s an example:
F = A * B; % Matrix multiplication
This will yield a new matrix where each element is the result of multiplying and summing the appropriate rows and columns from `A` and `B`.
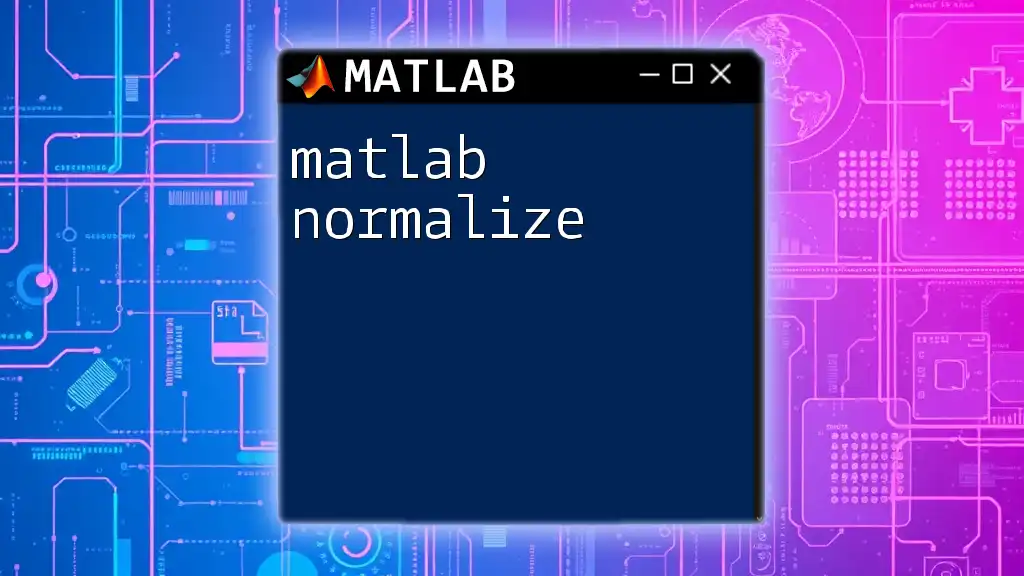
Advanced Matrix Operations
Transposing a Matrix
Transposing a matrix switches its rows and columns. The notation for the transpose operation is a single quote (`'`). For example:
G = A'; % Transpose of A
Inverse of a Matrix
The inverse of a matrix is crucial in solving linear equations. A matrix must be square and non-singular (i.e., must have a non-zero determinant) to have an inverse. Use the `inv` function to compute it:
H = inv(A); % Inverse of A
Determinants and Eigenvalues
The determinant provides information about matrix properties, such as whether it is invertible. You can calculate it using the `det` function, while eigenvalues and eigenvectors can be derived using the `eig` function:
detA = det(A); % Determinant of A
[V, D] = eig(A); % Eigenvalues and eigenvectors
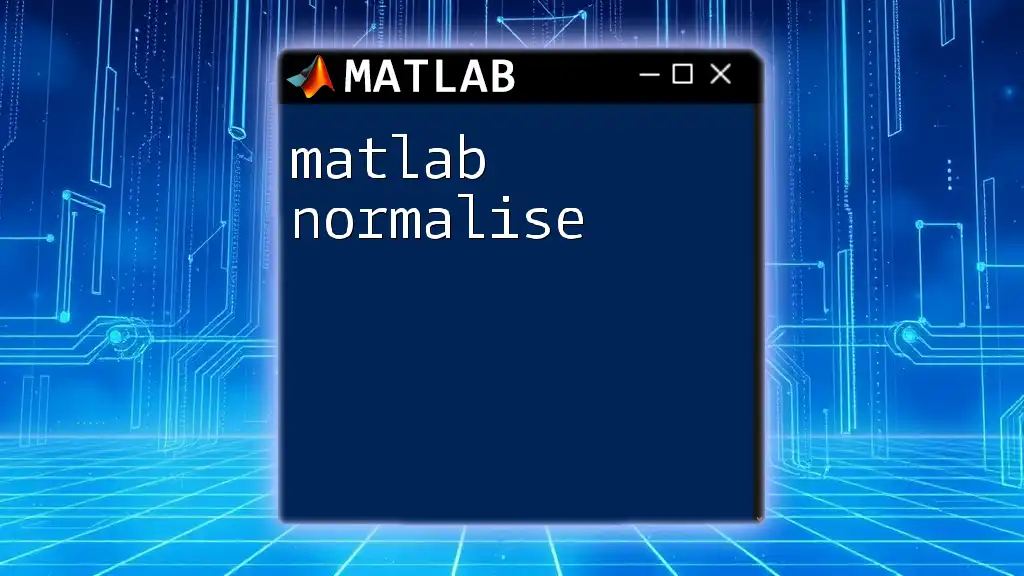
Practical Applications of Matrices in MATLAB
Data Representation
Matrices are fundamental in representing large data sets. You can utilize 2D matrices to store data points, measurements, or any numerical input efficiently. This capability allows easy access and manipulation of data.
Solving Linear Systems
Matrices play an essential role in solving systems of linear equations. For instance, to solve a system represented by `Ax = b`, where `A` is a matrix of coefficients and `b` is a column vector, you can use MATLAB's backslash operator, which provides a straightforward solution:
% Solve Ax = b
A = [3, 2; 1, 2];
b = [12; 8];
x = A\b; % MATLAB backslash operator for solving linear equations
This provides a vector `x` containing the solution to the linear system.
Image Processing
In the realm of image processing, images are represented as matrices where each element corresponds to a pixel intensity value. MATLAB offers various functions to manipulate these matrices easily, enabling operations like filtering, edge detection, and transformations. For example, reading and displaying an image can be performed with:
img = imread('example.jpg');
imshow(img);
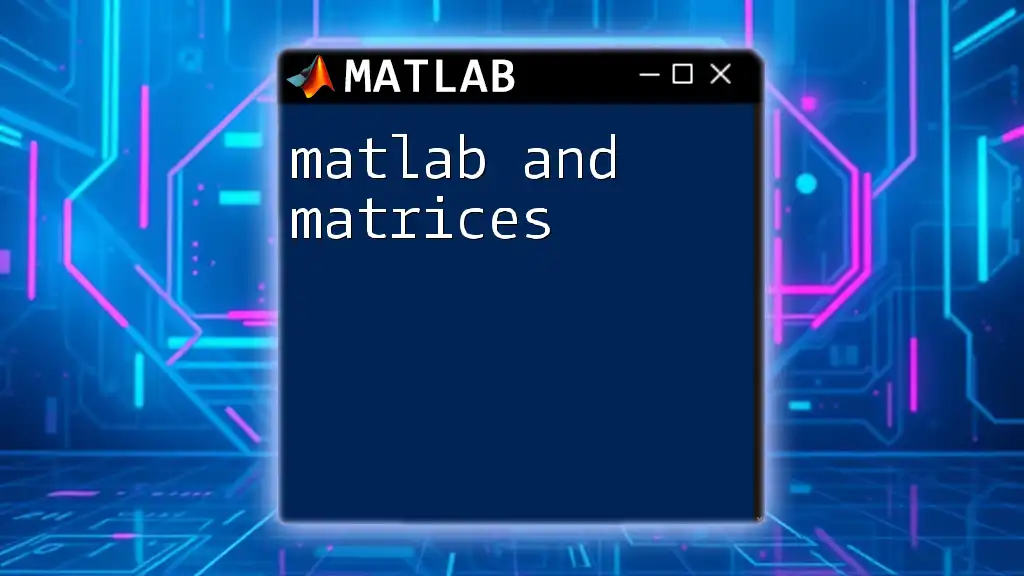
Conclusion
Mastering matrix operations in MATLAB is essential for anyone looking to harness the software's full potential for scientific computing, data analysis, and algorithm development. By comprehensively understanding the creation, manipulation, and application of matrices, you empower yourself with the tools necessary for tackling complex mathematical challenges. Embrace the practice with additional examples to boost your confidence and proficiency in MATLAB for matrix operations.