In MATLAB, you can calculate the logarithm of a number to base 10 using the `log10()` function.
Here's a code snippet demonstrating its usage:
% Calculate the log base 10 of a number
number = 1000;
logValue = log10(number);
disp(logValue); % This will display 3 since 10^3 = 1000
Understanding Logarithms
What is a Logarithm?
A logarithm is the inverse operation to exponentiation. That means the logarithm of a number is the exponent to which the base must be raised to produce that number. For example, if you have \( b^y = x \), then \( \log_b(x) = y \). Here, \(b\) is the base of the logarithm.
In practical terms, logarithms allow us to work with very large or very small numbers conveniently. They are paramount in various applications, from computer science to engineering.
Importance of Log Base 10
The log base 10, often denoted as \(\log_{10}\), is particularly significant in several fields:
-
Decibels in Sound: In acoustics, sound intensity is measured in decibels (dB), which is a logarithmic scale based on the power ratio of a given level compared to a reference level.
-
Richter Scale for Earthquakes: Earthquake magnitudes are measured on a log scale, where each unit increase corresponds to about 31.6 times more energy release.
These applications highlight how log base 10 simplifies calculations and makes comparison tractable.
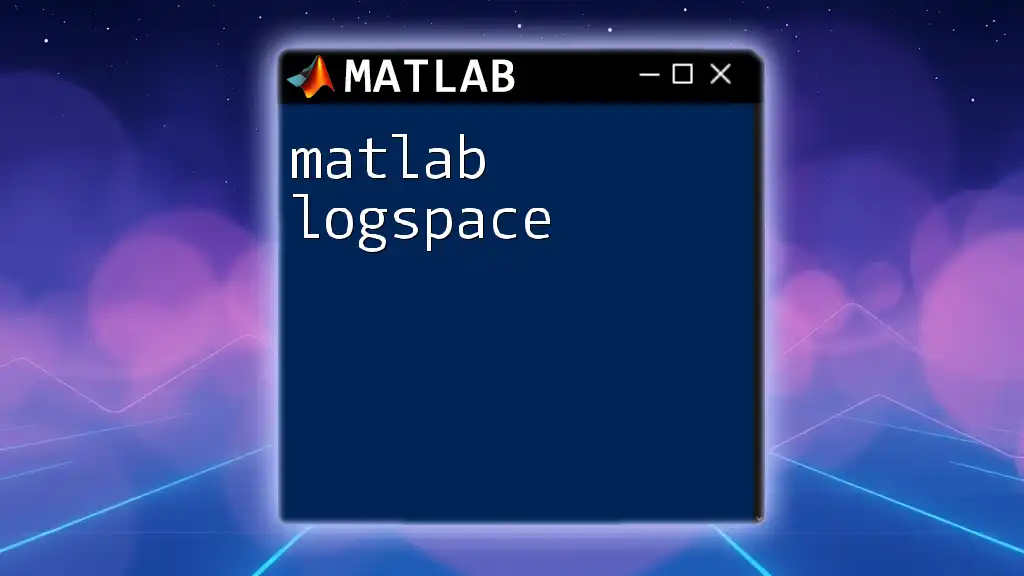
The MATLAB `log10` Function
Overview of the `log10` Function
In MATLAB, calculating the logarithm base 10 of a number is straightforward using the built-in `log10` function. The syntax for the function is:
log10(x)
Where `x` is the input variable, which must be a positive real number. If you input 0 or a negative number, MATLAB will return an error because logarithms for those numbers are not defined.
Basic Usage
Using `log10` is simple. Here are a few basic examples:
result1 = log10(100); % This will set result1 to 2
result2 = log10(10); % result2 will be 1
result3 = log10(1); % result3 will be 0
Notice how the function returns the exponent to which 10 must be raised to get the respective output. For instance, \(10^2 = 100\), therefore `log10(100)` yields 2.
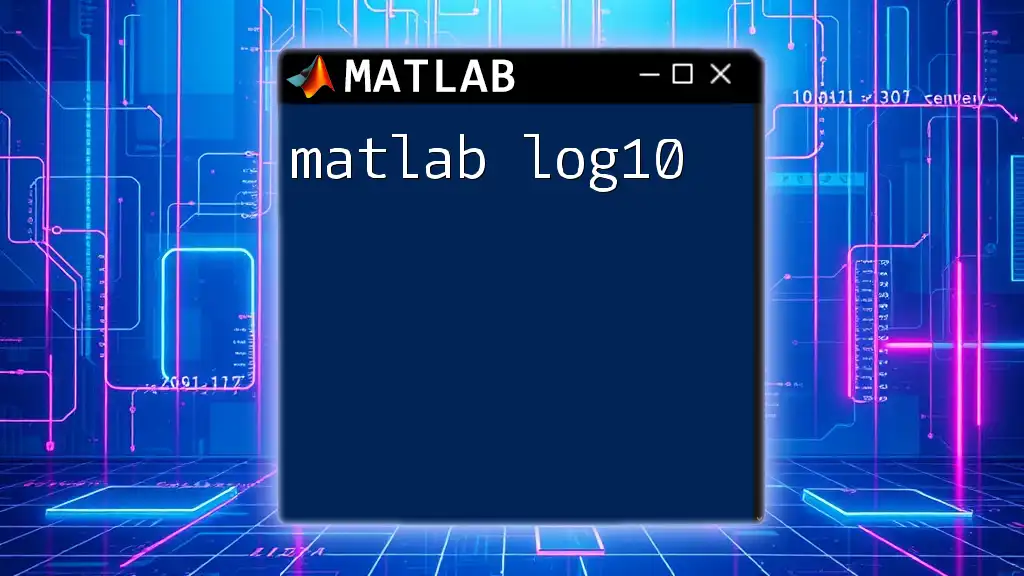
Logarithms of Vectors and Matrices
Working with Arrays
The `log10` function in MATLAB can also be applied to vectors and matrices. When using `log10` on an array, it computes the logarithm for each element separately.
Consider the following example:
A = [10, 100; 1000, 10000];
result = log10(A);
The output matrix `result` would contain:
1 2
3 4
This demonstrates that the function operates element-wise, making it efficient for bulk operations on data sets.
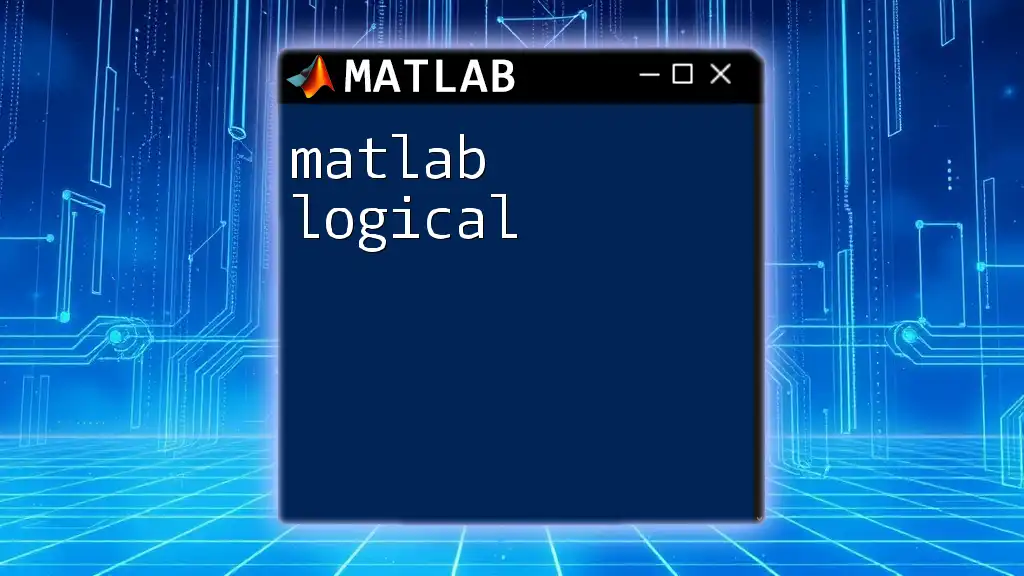
Handling Complex Numbers
Using `log10` with Complex Numbers
In MATLAB, the `log10` function seamlessly handles complex numbers. In mathematical terms, the logarithm of a complex number \(z = a + bi\) can be expressed as:
$$\log_{10}(z) = \log_{10}(|z|) + i \frac{\text{arg}(z)}{\pi}$$
Where \(|z|\) is the magnitude of the complex number and \(\text{arg}(z)\) is the angle.
Take this example:
z = 10 + 10i;
result = log10(z);
This will yield a complex result, providing both the magnitude and the argument (angle) of the complex logarithm.
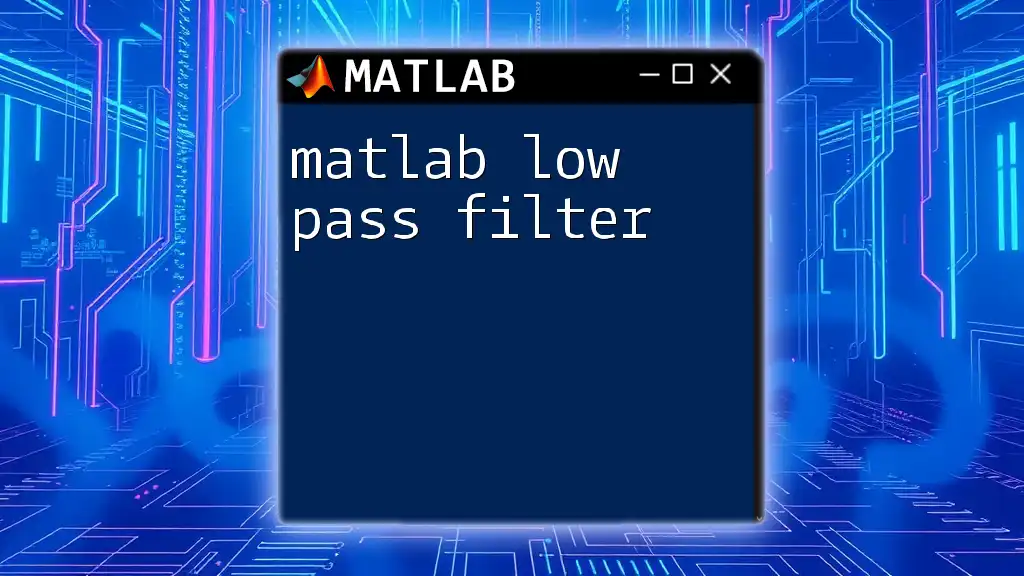
Logarithmic Properties in MATLAB
Key Properties of Logarithms
Understanding the properties of logarithms is critical in simplifying mathematical expressions. Below are some key properties, along with how you can apply them in MATLAB:
-
Product property: \[ \log_{10}(a \cdot b) = \log_{10}(a) + \log_{10}(b) \]
-
Quotient property: \[ \log_{10}\left(\frac{a}{b}\right) = \log_{10}(a) - \log_{10}(b) \]
-
Power property: \[ \log_{10}(a^b) = b \cdot \log_{10}(a) \]
MATLAB Examples Demonstrating Properties
Here are code snippets illustrating each property effectively:
a = 100;
b = 10;
p = 2;
% Product property
result1 = log10(a * b); % This will equal log10(a) + log10(b)
product_property = log10(a) + log10(b);
% Quotient property
result2 = log10(a / b); % This will equal log10(a) - log10(b)
quotient_property = log10(a) - log10(b);
% Power property
result3 = log10(a^p); % This will equal p * log10(a)
power_property = p * log10(a);
Each of these computations should yield equivalent results that confirm the properties.
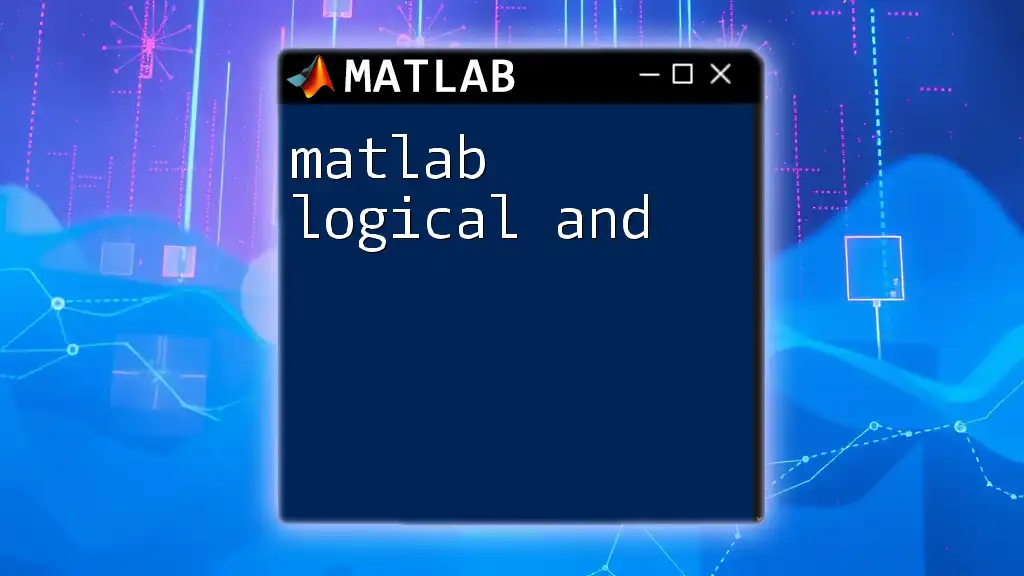
Common Errors and Troubleshooting
What to Avoid When Using `log10`
New users may encounter errors while using the `log10` function in MATLAB, primarily when the input is not within the acceptable range. The function requires positive non-zero inputs; otherwise, you’ll get an error indicating the input must be positive.
Error Messages Explanation
For example, running:
result = log10(-5); % This will return an error
You’ll receive an error similar to “Error using log10. Input must be a positive real number.” Always ensure your input data meets the requirements.
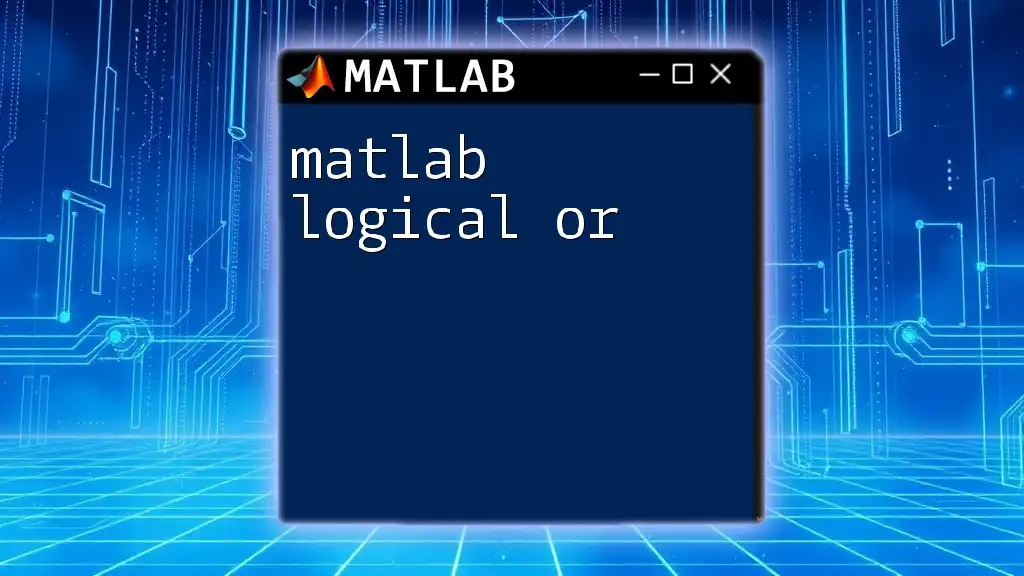
Practical Applications of `log10` in MATLAB
Engineering and Scientific Applications
Logarithms play a significant role across many scientific and engineering disciplines. In data analysis, log transformations often help normalize distributions, making data easier to work with, especially in large datasets.
Case Study
Consider analyzing sound levels measured in decibels:
sound_levels = [0, 10, 20, 30, 40, 50]; % Levels in decibels
powers = 10.^(sound_levels / 10); % Convert dB to power
logPowers = log10(powers); % Calculate log base 10 of power
% Analyze results for understanding sound intensity conversion
disp(logPowers);
In this case, transforming the sound levels into power and their logs allows clearer insight into variations in intensity.
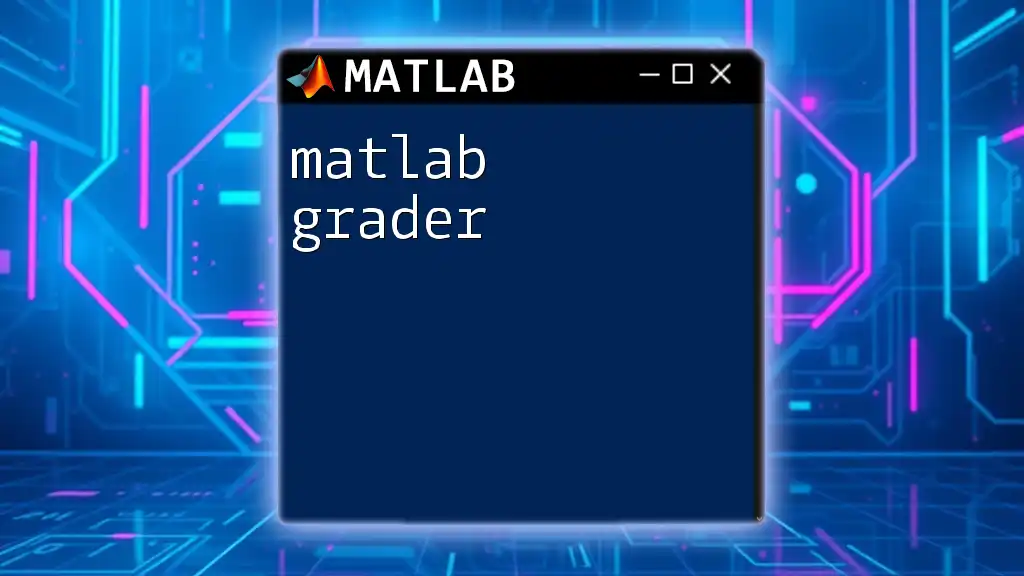
Conclusion
The MATLAB log base 10 function, `log10`, is a powerful tool for mathematicians, engineers, and scientists alike. With its user-friendly syntax and ability to handle vectors, matrices, and complex numbers, this function simplifies calculations and enhances data analysis significantly. By understanding its properties and applications, you can leverage logarithms to bring clarity and efficiency to your mathematical tasks.
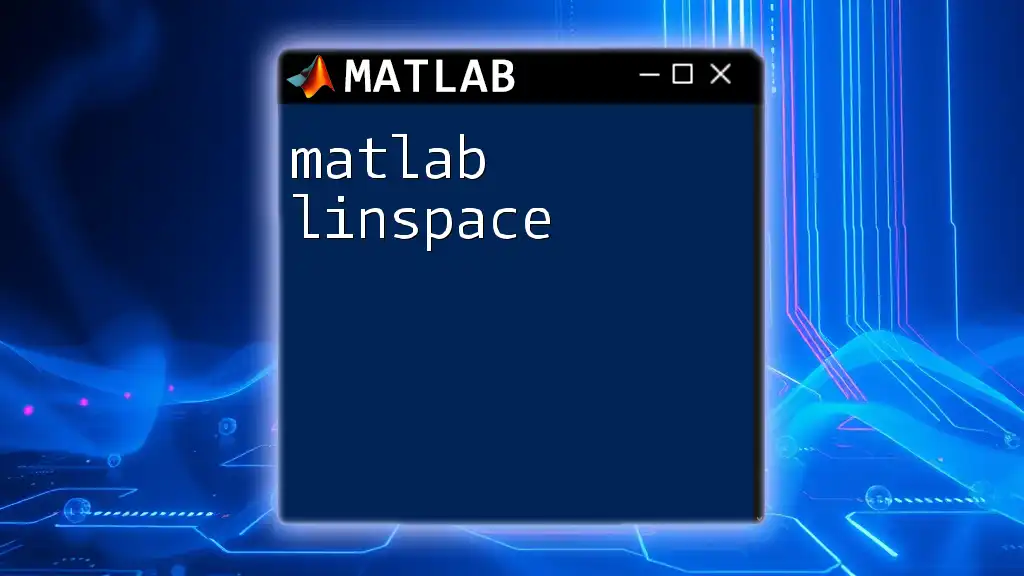
Additional Resources
To dig deeper into MATLAB's offerings related to logarithmic functions, consider referring to the official MATLAB documentation. Books and tutorials on MATLAB offer additional hands-on learning opportunities to maximize your proficiency in this powerful software.
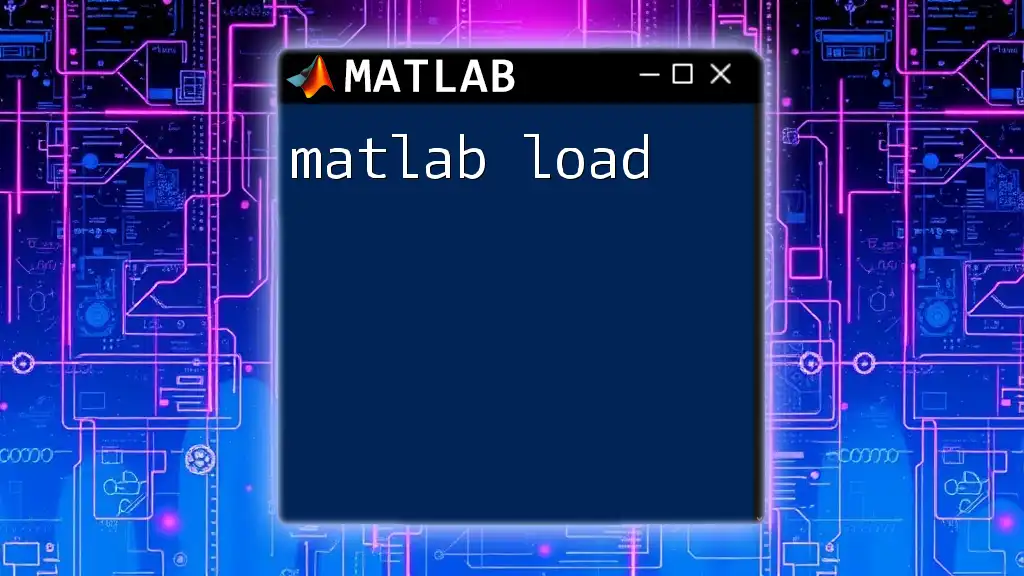
Call to Action
Join our MATLAB tutorials today to explore how the matlab log base 10 function—and more—can enhance your programming and mathematical analysis skills!