In MATLAB, a double is a data type used to represent double-precision floating-point numbers, which is the default numeric type for most numerical computations.
Here’s a simple example demonstrating the creation of a double variable in MATLAB:
% Define a double variable
myDouble = 3.14159;
Understanding the Double Data Type
What is a Double in MATLAB?
In MATLAB, double refers to the default data type for representing numerical values as double-precision floating-point numbers. This representation allows for significant computational accuracy and is particularly important in mathematical and engineering applications. The double data type follows the IEEE 754 standard, which ensures that numbers are stored with a precision of approximately 15 decimal digits.
Characteristics of Double
Double variables are versatile and widely used in MATLAB due to their significant precision and range of representable values. A double can represent numbers as large as approximately \(1.8 \times 10^{308}\) and as small as approximately \(5.0 \times 10^{-324}\). This extensive range, coupled with their high precision, makes doubles an ideal choice for applications that require accurate computational results.
To contrast, other numeric types in MATLAB include:
- Single: Uses less memory and provides lower precision (about 7 decimal digits).
- Integer Types: Fixed in size and do not support fractions.
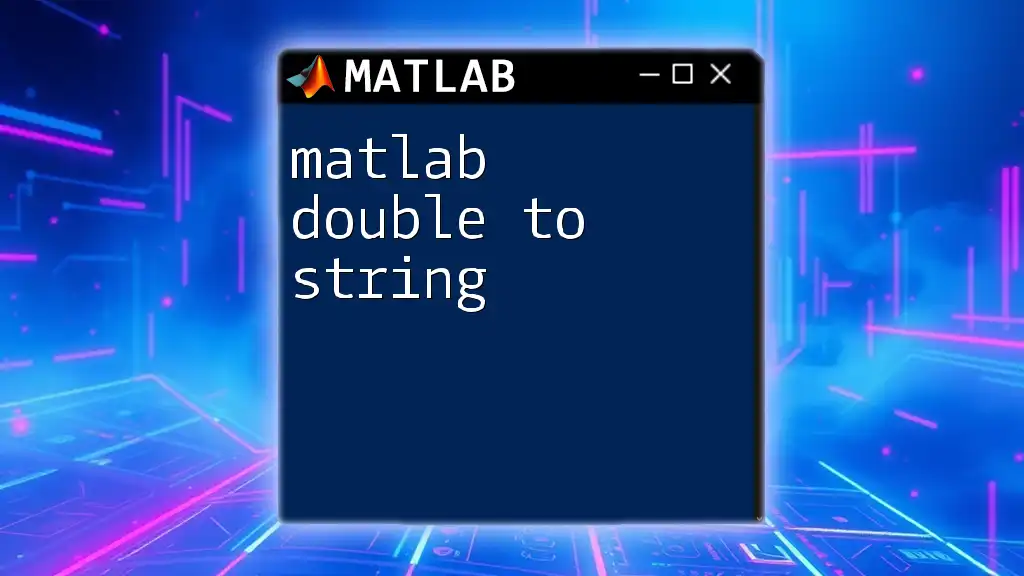
Creating and Using Double Variables
Declaring a Double Variable
Declaring a double variable in MATLAB is straightforward. The syntax allows for the intuitive allocation of numeric values. Here are examples demonstrating how to declare double variables:
a = 5.5; % A simple double variable
b = 3.14e2; % Scientific notation for double
The above code snippets show how to create double variables with regular numbers and scientific notation. The numerical flexibility of doubles serves to enhance the capability to handle a variety of calculations effectively.
Basic Operations with Doubles
Once doubles are declared, they can be manipulated using basic arithmetic operations. These operations are straightforward, enabling quick and efficient calculations:
sum = a + b; % Addition
diff = a - b; % Subtraction
prod = a * b; % Multiplication
quot = a / b; % Division
In these examples, you'll notice how each arithmetic computation is performed directly on double variables, and the results are stored in new double-type variables.
Advanced Operations
Beyond simple arithmetic, MATLAB supports a suite of advanced mathematical operations using double variables, such as trigonometric functions and matrix operations.
Trigonometric Functions
For instance, calculating trigonometric values such as sine and cosine can be achieved effortlessly:
theta = 30; % Angle in degrees
radian = theta * (pi / 180); % Convert degrees to radians
sine_value = sin(radian); % Calculate sine of the angle
In this instance, the conversion from degrees to radians is necessary for the proper functioning of trigonometric functions, illustrating the seamless integration of double values in various mathematical contexts.
Matrix Operations
Doubles are also invaluable in matrix manipulations, a cornerstone of linear algebra and engineering applications. For example, the following illustrates basic matrix multiplication:
A = [1.5, 2.5; 3.5, 4.5]; % Matrix of doubles
B = [1.0, 1.0; 1.0, 1.0]; % Another matrix of doubles
C = A * B; % Matrix multiplication
This example shows how matrix operations can be executed using double-type matrices, producing a resultant matrix that retains the double properties.
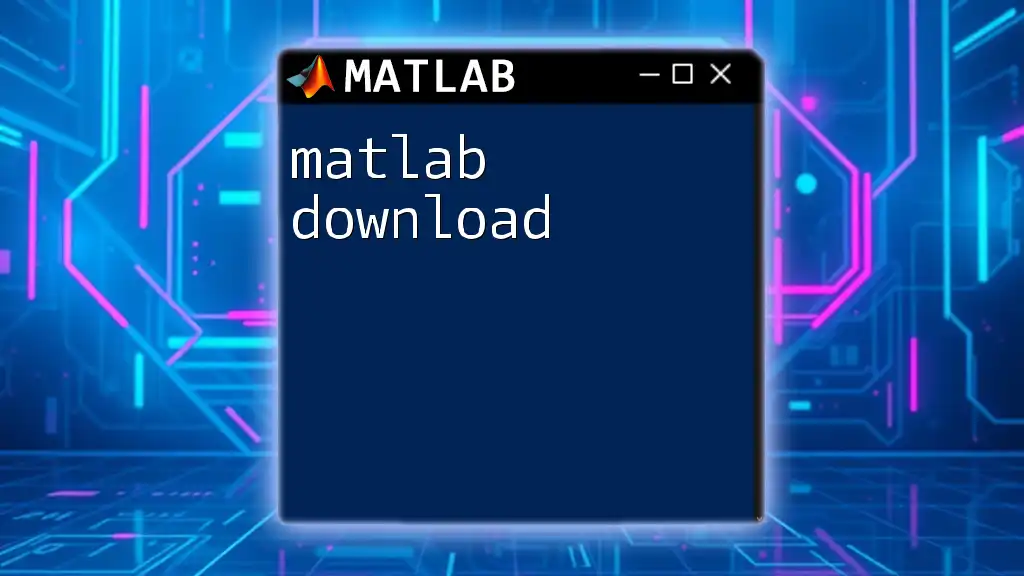
Manipulating Doubles
Rounding and Precision Control
Working with doubles may occasionally require adjustments in precision. MATLAB provides several built-in functions to control rounding:
a = 3.75;
rounded_value = round(a); % Rounding to nearest integer
Functions such as `round`, `floor`, `ceil`, and `fix` help in managing numerical precision effectively. Each function serves a specific purpose in rounding, enabling users to manipulate double values as needed for their computational tasks.
Converting Between Data Types
MATLAB allows the conversion of doubles to other types and vice versa using type casting, which is essential for interoperability among various data types:
int_value = int32(a); % Converting double to int32
In this scenario, the double variable `a` is converted to an integer type, demonstrating flexibility in data manipulation.
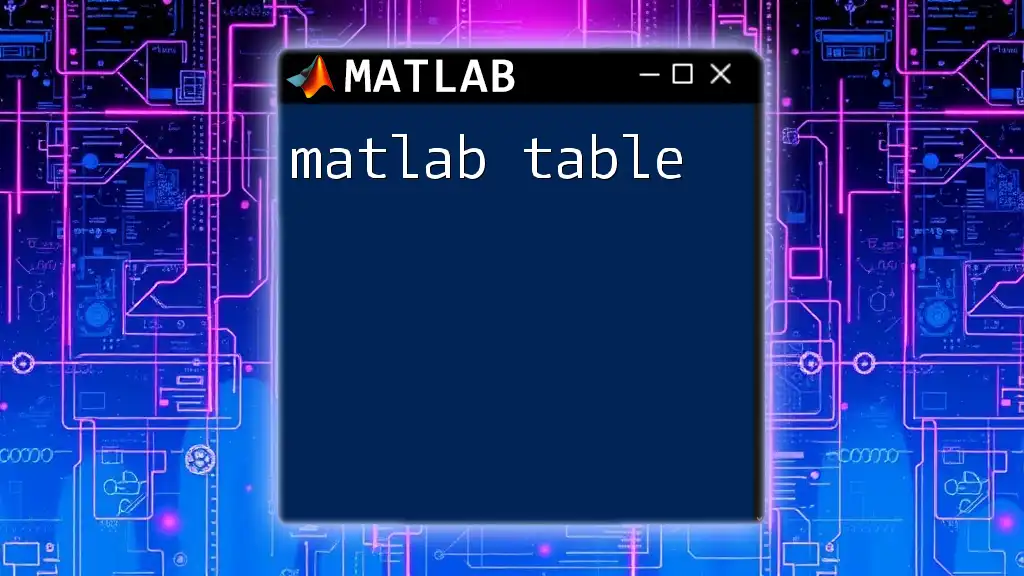
Common Issues and How to Avoid Them
Precision Errors in Calculations
When performing calculations involving doubles, it's crucial to understand the potential for precision errors. Floating-point arithmetic can introduce inaccuracies, especially when dealing with very large or very small numbers.
To mitigate these errors, it's recommended to implement comparison tolerance when validating equality between doubles. For example:
if abs(a - b) < 1e-10
% Considered equal within tolerance
end
Overflows and Underflows
The limitations inherent in double precision can sometimes lead to overflows (when numbers exceed the upper representational limit) or underflows (when numbers are too small). To handle these issues, you can use MATLAB functions like `isfinite` to check for valid double values, ensuring your computations remain stable.
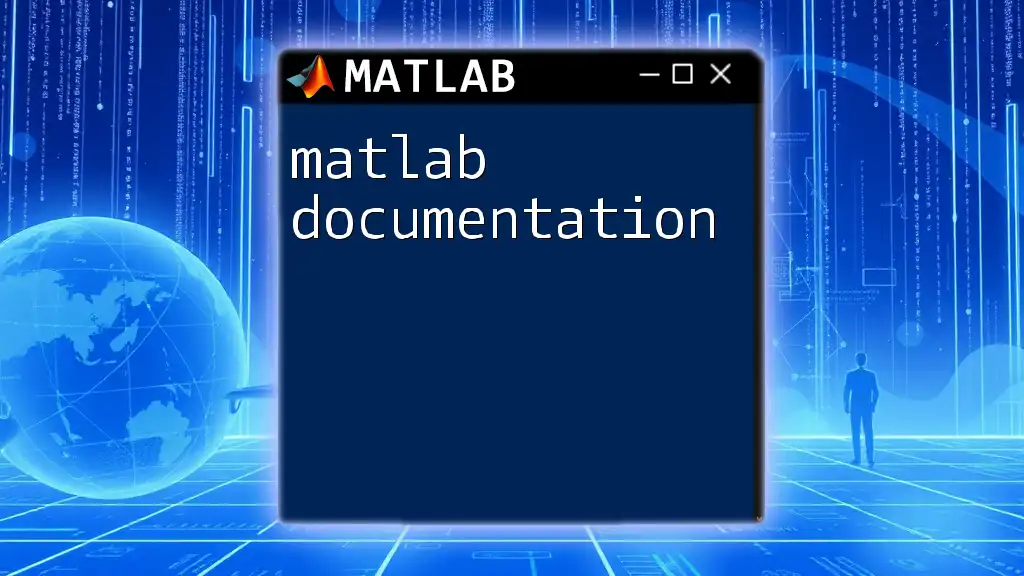
Practical Applications of Doubles
Real-World Examples
Doubles are extensively utilized in various real-world applications. In engineering simulations, for example, they enable precise calculations required for modeling systems, performing optimizations, and analyzing performance metrics.
When conducting data analysis, the flexibility of doubles allows for robust statistical computation, helping researchers derive conclusions and insights from numerical data effectively.
Performance Considerations
As you work with doubles, be aware of their impact on computational performance. Although doubles provide superior precision, using lower precision types in scenarios that don't require extensive accuracy can enhance computational speed and optimize memory usage. Consider this carefully when designing algorithms or applications in MATLAB.
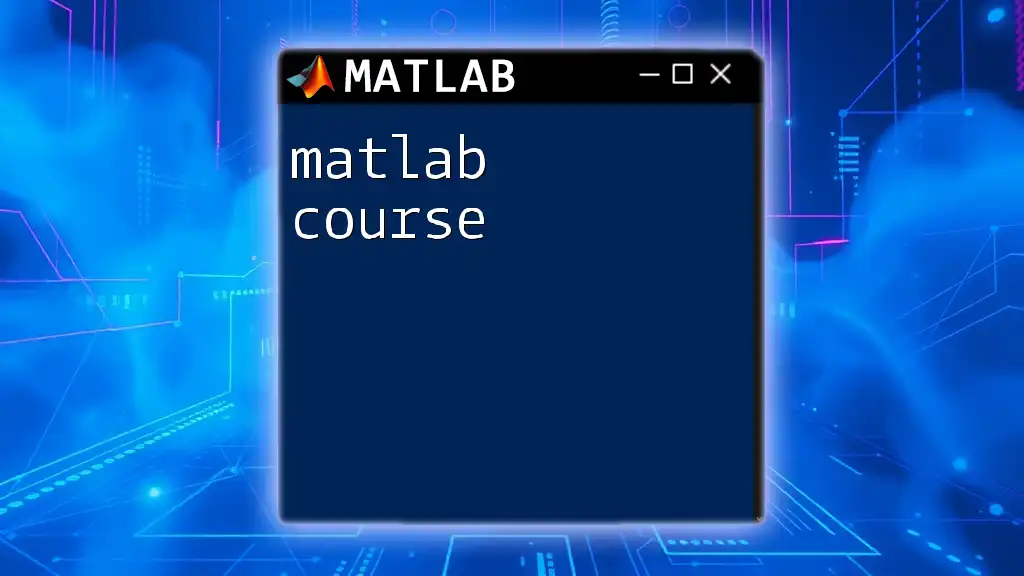
Conclusion
The double data type in MATLAB is a powerful tool that enables accurate numerical computation across various applications. Understanding its characteristics, operations, and best practices can significantly enhance your programming efficiency and effectiveness.
Encourage your growth with MATLAB by practicing the skills outlined in this guide. Dive deeper into the intricacies of double variables and share your projects or queries with the community. Whether you are an engineer, scientist, or student, mastering the double type will equip you with essential tools to tackle the complexities of numerical data.