MATLAB software is a powerful platform for numerical computing and algorithm development that allows users to analyze data, develop algorithms, and create models and applications efficiently.
Here's a simple code snippet to compute the sum of an array in MATLAB:
array = [1, 2, 3, 4, 5];
total = sum(array);
disp(total);
Getting Started with MATLAB
Installation and Setup
Downloading MATLAB
To start your journey with MATLAB software, you need to obtain the software. You can download it from the official [MathWorks website](https://www.mathworks.com/). Ensure that you check the system requirements to confirm compatibility with your operating system.
Installation Steps
The installation process is straightforward. After downloading the installer, simply follow the prompts to install MATLAB on your computer. It is advisable to opt for the default settings for beginners. Once installed, you can launch MATLAB from your applications menu.
Navigating the MATLAB Interface
The MATLAB Desktop
Upon launching MATLAB, you will be greeted with its user interface, commonly referred to as the MATLAB Desktop. Here, you'll find several key components:
- Command Window: This is where you type commands and see output.
- Workspace: This displays all the variables you have created during your session.
- Editor: This area is where you can write and save scripts and functions.
To enhance your productivity, you can customize the MATLAB interface by arranging panels as per your preferences.
Basic Commands
Begin by familiarizing yourself with some essential commands. In the Command Window, you can execute straightforward commands. Here are some foundational commands:
- `clc`: Clears the Command Window.
- `clear`: Removes all variables from the Workspace.
- `close all`: Closes all open figures.
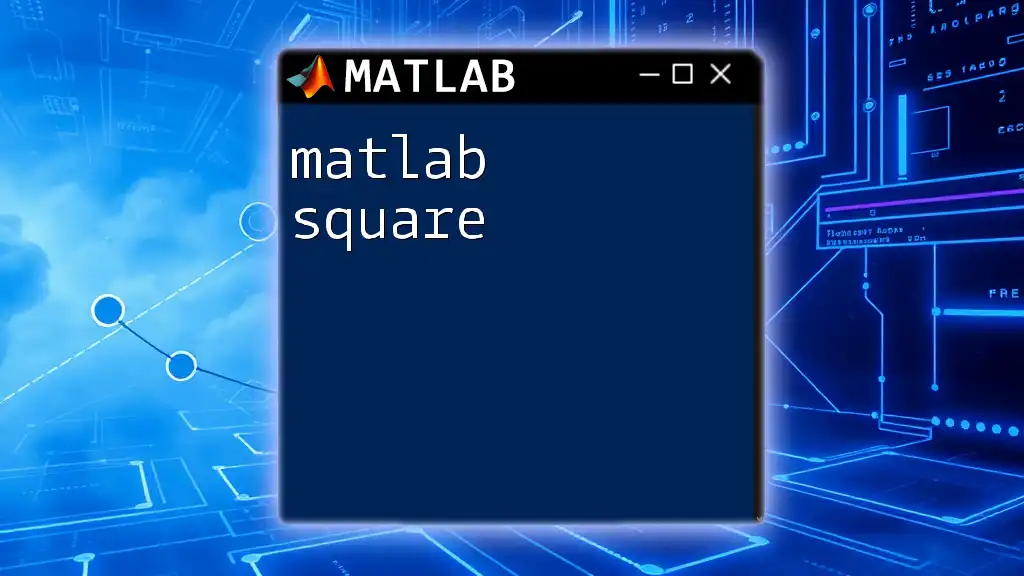
Basic MATLAB Commands and Functions
Working with Variables
Defining Variables
In MATLAB, creating variables is as simple as assigning a value to a name. For example, you can define a variable like this:
a = 5;
This assigns the value 5 to the variable `a`. MATLAB allows you to use descriptive variable names, aiding in code readability.
Basic Data Types in MATLAB
MATLAB supports several data types, including:
- Numeric: This is used for numbers, both integers and decimals.
- Character: Typically used for strings, which are sequences of characters.
- Logical: This data type can only represent true (1) or false (0).
Here’s a code snippet demonstrating various data type definitions:
integerVar = 42; % Numeric
charVar = 'Hello, MATLAB!'; % Character
logicalVar = true; % Logical
Mathematical Operations
Elementary Operations
MATLAB allows you to perform basic mathematical operations seamlessly. Here are some operations:
addition = 2 + 3;
subtraction = 7 - 5;
multiplication = 4 * 5;
division = 10 / 2;
MATLAB also supports element-wise operations for arrays, enabling you to perform operations on individual elements.
Matrix Operations
Matrices are fundamental in MATLAB. To create and manipulate matrices, you can use the following syntax:
A = [1, 2, 3; 4, 5, 6]; % Create a 2x3 matrix
B = [7; 8; 9]; % Create a 3x1 matrix
C = A * B; % Matrix multiplication
Understanding matrices is crucial, as they are extensively used in engineering, data analysis, and various scientific applications.
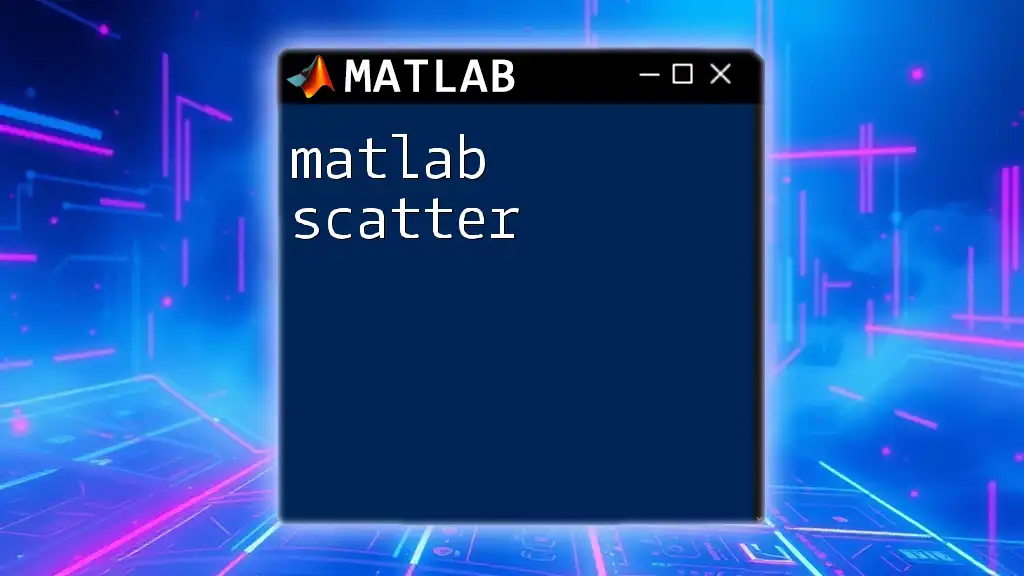
Intermediate Commands and Programming Concepts
Control Flow
Using Conditional Statements
Control flow statements allow you to execute code conditionally. The `if`, `else`, and `elseif` statements are used for this purpose. Here’s an example:
x = 10;
if x > 0
disp('x is positive');
elseif x < 0
disp('x is negative');
else
disp('x is zero');
end
In this snippet, MATLAB will display whether `x` is positive, negative, or zero based on the condition evaluated.
Loops in MATLAB
Loops are essential for executing repetitive tasks. The `for` and `while` loops are commonly used. An example of a `for loop` iterating through an array:
for i = 1:5
disp(['Loop iteration: ', num2str(i)]);
end
This code will display the loop iteration numbers from 1 to 5.
Functions
Creating Custom Functions
Functions are a way to encapsulate code for reuse. A simple function can be written as follows:
function result = myFunction(x)
result = x^2 + 5;
end
This defines a function that takes an input `x`, computes it squared plus five, and returns the result.
Function Inputs and Outputs
This structure allows you to specify multiple inputs and outputs, enhancing the modularity of your code. For example:
function [square, cube] = calculatePowers(x)
square = x^2;
cube = x^3;
end
In this function, both the squared and cubed values of the input are returned.
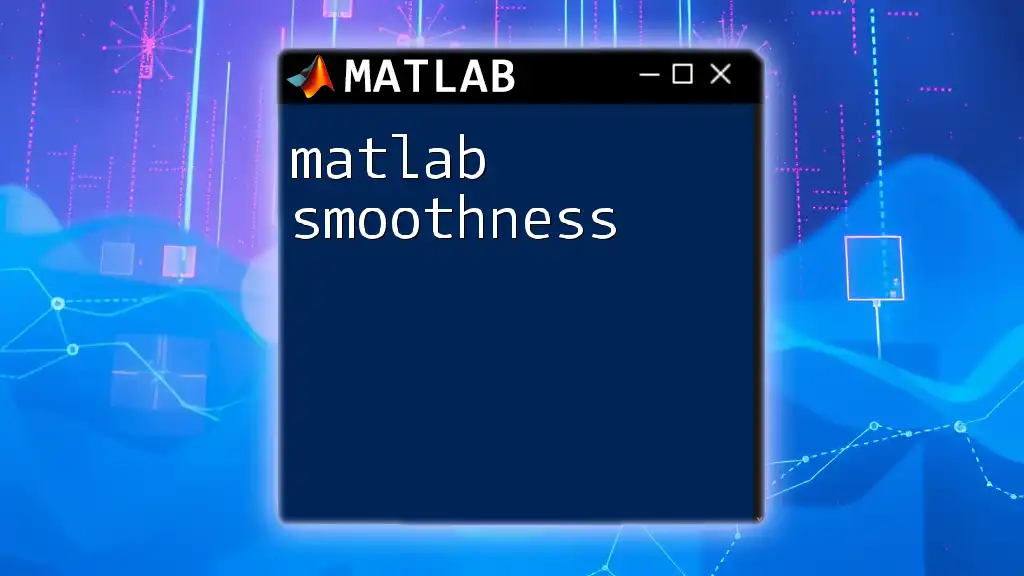
Advanced MATLAB Features
Data Visualization
Plotting in MATLAB
Visual representation of data is a powerful way to understand it. MATLAB provides built-in functions for creating plots. Here’s how to create a simple sine wave plot:
x = 0:0.1:10; % Generate values from 0 to 10 in steps of 0.1
y = sin(x); % Compute the sine of each value
plot(x, y); % Create the plot
title('Sine Wave');
xlabel('x-axis');
ylabel('y-axis');
This code snippet demonstrates generating data and visualizing it through MATLAB's plotting capabilities, which are crucial in data analysis.
Advanced Plotting Techniques
MATLAB allows you to customize your plots extensively. You can modify colors, line styles, and add legends:
plot(x, y, 'r--', 'LineWidth', 2); % Red dashed line
legend('Sine');
grid on; % Adding a grid to the plot
Such customizations enhance the clarity and aesthetics of the plots.
Toolboxes and Add-Ons
What are Toolboxes?
MATLAB offers specialized toolboxes that cater to various domains, such as robotics, image processing, and machine learning. These toolboxes come with functions optimized for specific tasks, allowing you to extend the capabilities of MATLAB.
Popular Toolboxes Overview
Some notable toolboxes include:
- Signal Processing Toolbox: Tools for analyzing, preprocessing, and filtering signals.
- Image Processing Toolbox: Functions for image analysis, enhancement, and object detection.
Familiarizing yourself with these toolboxes can significantly enhance your productivity and expand your horizons in MATLAB software applications.
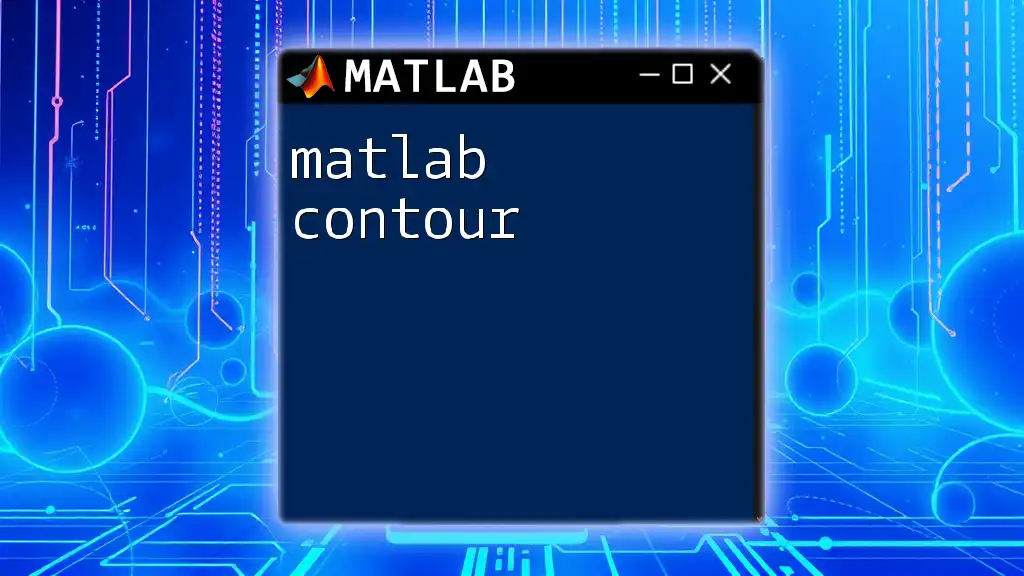
MATLAB for Data Analysis and Machine Learning
Data Import and Export
Working with Data Files
One of MATLAB's strengths is its ability to handle data easily. You can import data from various file formats, such as CSV or Excel. For instance, to read a CSV file, you can use:
data = readtable('data.csv');
This command imports the data from `data.csv` into a table format, enabling you to manipulate it conveniently.
Exporting Results
After analysis, you might want to share your results. Exporting data from MATLAB is simple. For instance, to write data back to a CSV file, you can use:
writetable(data, 'results.csv');
This allows seamless communication between MATLAB and other software tools.
Building Machine Learning Models
Introduction to Machine Learning in MATLAB
MATLAB is well-equipped for machine learning applications. With built-in functions, you can build, train, and test machine learning models efficiently. For instance, you can create a linear regression model with just a few lines of code:
mdl = fitlm(X, Y); % Fit a linear model to the data
This code snippet illustrates how straightforward it is to get started with machine learning in MATLAB software.
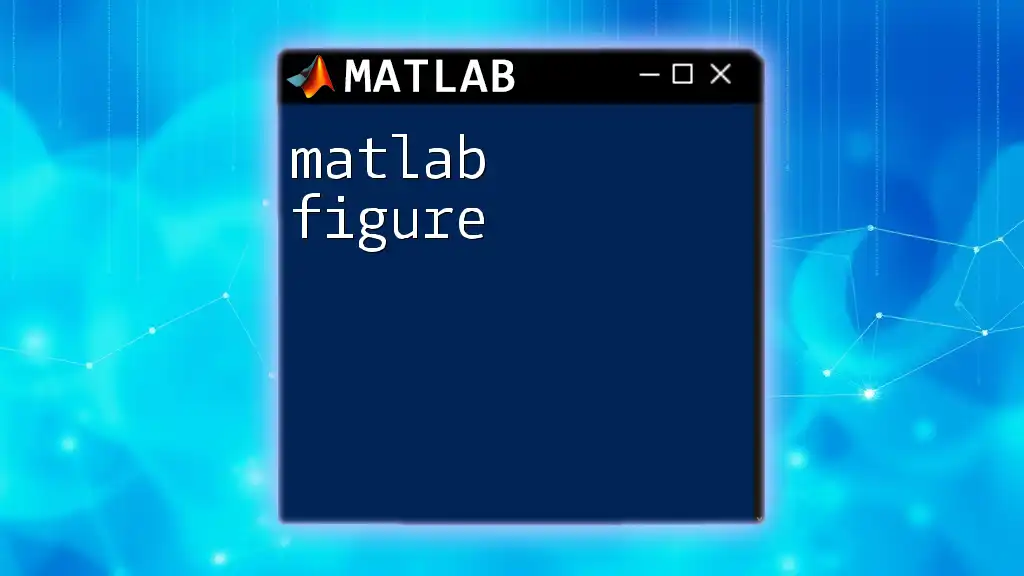
Conclusion
Throughout this comprehensive guide, we’ve emphasized the power and versatility of MATLAB software. From basic commands and functions to advanced concepts like data visualization and machine learning, mastering these elements can pave the way for numerous opportunities in engineering, data analysis, and research fields. Continue practicing with your projects, and leverage the vast resources available, ensuring your skills remain sharp and up-to-date in the ever-evolving landscape of technology. As you delve deeper, you will find MATLAB not only a tool but also a gateway to innovative solutions and discoveries.