"MATLAB logicals are data types that represent true and false values, enabling users to perform conditional checks and logical operations efficiently."
Here’s a simple example of using logical operations in MATLAB:
% Define two vectors
A = [1, 2, 3, 4, 5];
B = [2, 3, 6, 7, 8];
% Find common elements using logical indexing
commonElements = A(ismember(A, B));
Understanding Logical Data Types
What is a Logical Array?
In MATLAB, a logical array is a data structure that contains boolean values, specifically true (1) or false (0). Unlike numeric arrays that may contain a variety of numbers, logical arrays serve a distinct purpose in decision-making processes within scripts and functions. Logical arrays are particularly useful for condition-checking or filtering operations, allowing for efficient manipulation of data.
Creating Logical Arrays in MATLAB
Creating logical arrays is straightforward using the `true` and `false` functions. For instance, you can generate an array where all values are true or all are false:
logicalArray1 = true(3, 3); % Creates a 3x3 array of true values
logicalArray2 = false(2, 5); % Creates a 2x5 array of false values
In this example, `logicalArray1` is a 3x3 matrix filled entirely with true values, while `logicalArray2` is a 2x5 matrix filled with false values. This ability to create logical arrays with specific dimensions is vital for setting up conditions later in your program.
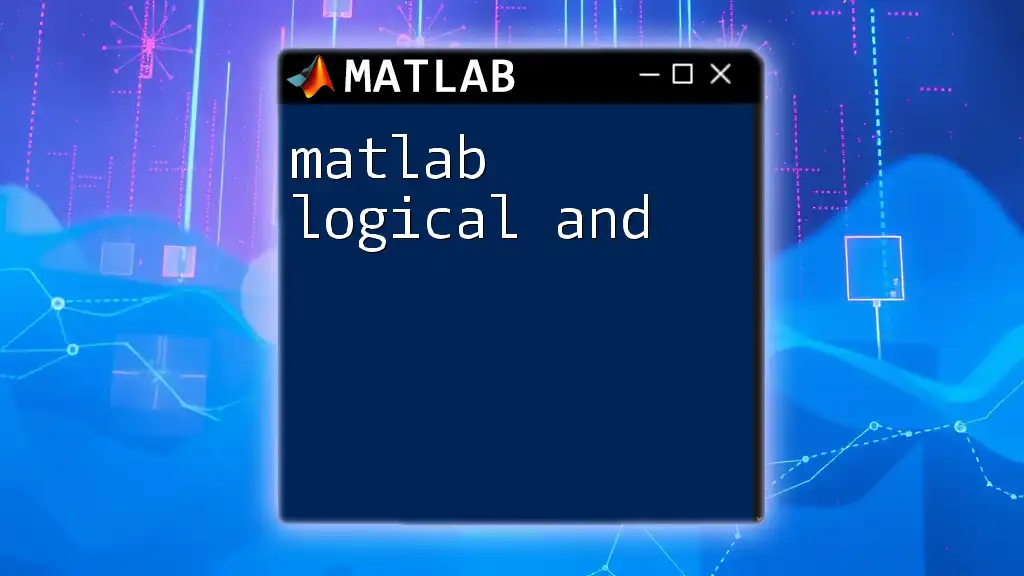
Basic Logical Operations
The AND Operation
Logical operations frequently involve the AND condition, represented by `&` for element-wise operations and `&&` for short-circuiting evaluations. To showcase this, consider the following example:
a = [1, 2, 3];
b = [0, 1, 1];
andResult = a > 1 & b == 1; % Element-wise logical AND
In this case, `andResult` will yield the result: `[false, true, false]`. The operation checks both arrays against the specified conditions, returning true only when both conditions hold true for the corresponding elements.
The OR Operation
The OR operation functions similarly, using `|` for element-wise evaluation and `||` for short-circuiting. Here's how it works:
orResult = a < 2 | b == 1; % Element-wise logical OR
For the given variables, `orResult` will evaluate to `[true, true, true]`. The OR operator confirms true when at least one of the conditions, comparing elements from both arrays, is met.
The NOT Operation
To invert a logical condition, we employ the NOT operation, represented by `~`. This operator allows for a straightforward means to negate values:
notResult = ~(a > 2); % Logical NOT operation
In this example, `notResult` becomes `[true, true, false]`, effectively reversing the truth values of the original comparison.
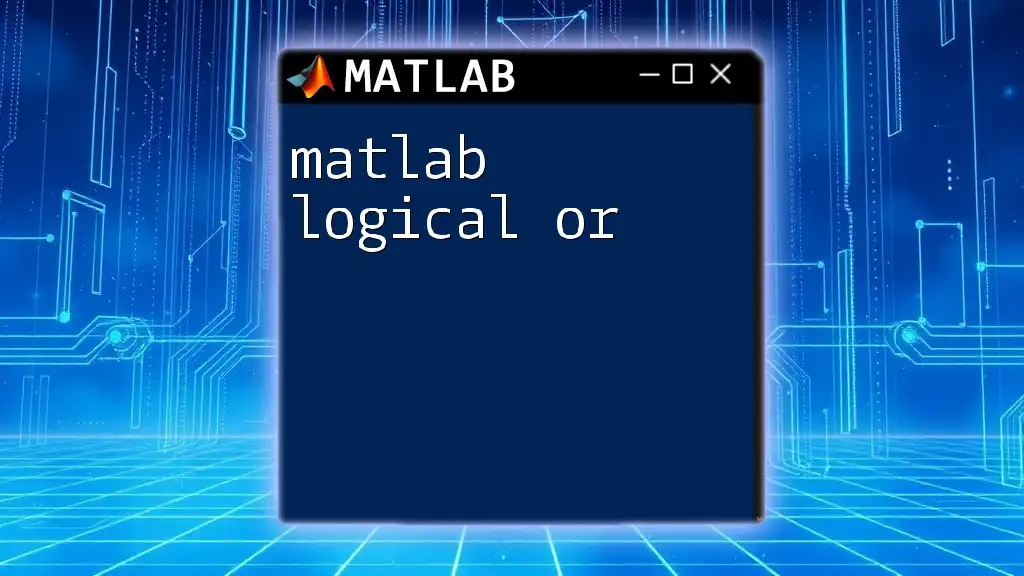
Advanced Logical Operations
Using `xor` for Exclusive OR
The `xor` function in MATLAB stands for "exclusive OR." It is useful when you want to test that either one condition or another is true, but not both. Consider the following scenario:
a = [true, false, true];
b = [false, false, true];
xorResult = xor(a, b);
In this example, `xorResult` evaluates to `[true, false, false]`, confirming that the first element is true when only one of the conditions is true.
Logical Short-Circuiting
Logical short-circuiting is a behavior exhibited by the operators `&&` and `||`. When using these operators, if the first condition evaluates to determinatively true or false, MATLAB will not evaluate the second condition. Here's an example:
x = 0;
result = (x ~= 0) && (10 / x > 1); % No division by zero occurs
In this scenario, since `x` equals zero, the first condition evaluates as false, and MATLAB skips the division, which safeguards against potential errors.
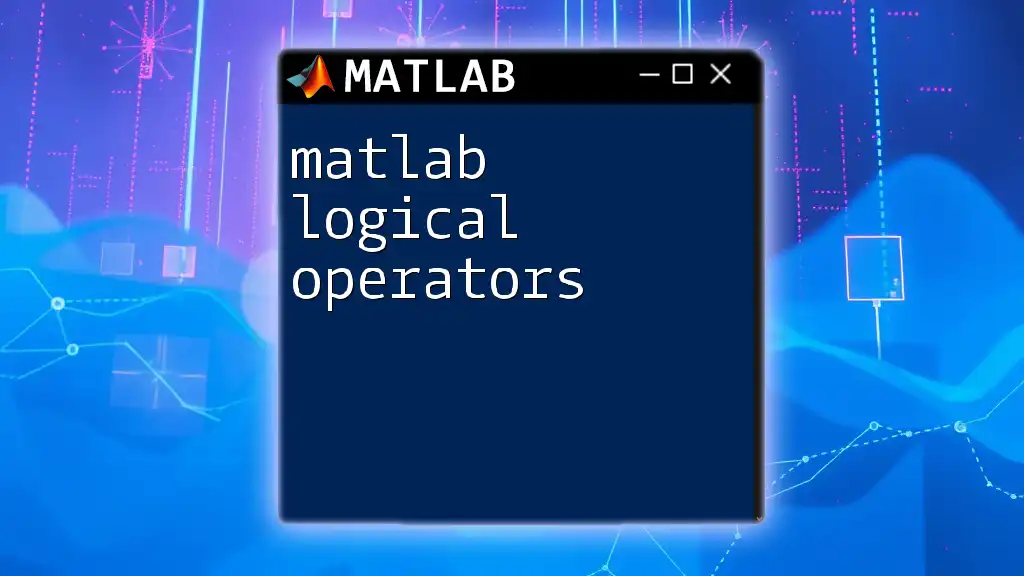
Logical Indexing
Introduction to Logical Indexing
Logical indexing is a powerful feature in MATLAB where logical arrays can be used to index other arrays. This allows for selective data manipulation based on specific conditions.
Example of Logical Indexing
A demonstration of logical indexing can be illustrated as follows:
data = [10, 20, 30, 40, 50];
logicalIdx = data > 30;
filteredData = data(logicalIdx); % Returns elements greater than 30
Here, `filteredData` will contain `[40, 50]`, as these are the values from the original array that satisfy the condition of being greater than 30. This technique is invaluable for extracting pertinent information from larger datasets.
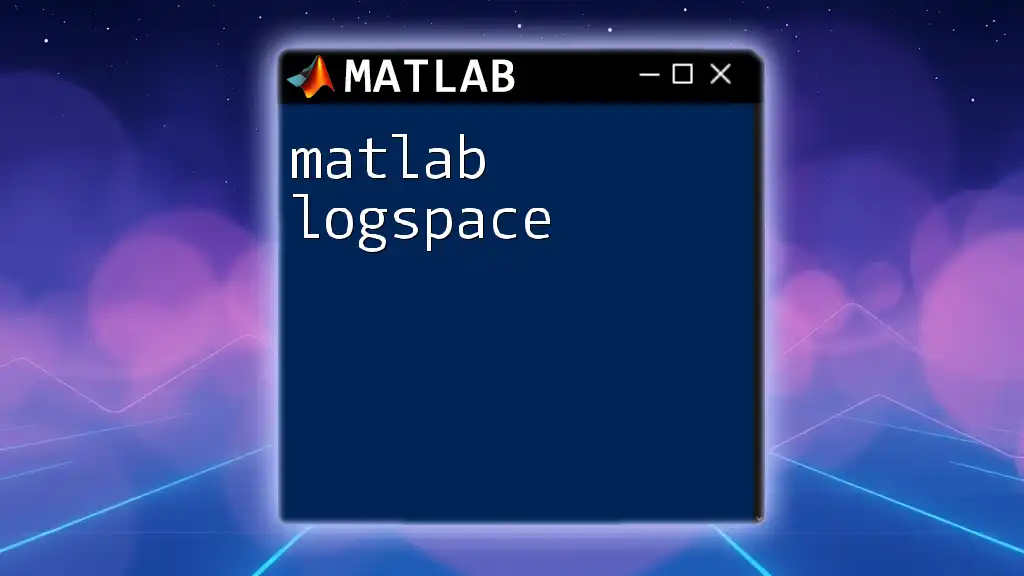
Conditional Statements with Logical Commands
Using Logical Commands in `if` Statements
Logical commands can be seamlessly integrated within conditional statements such as `if`, enabling dynamic decision-making within your code:
value = 15;
if value > 10 && value < 20
disp('Value is between 10 and 20.');
end
This will display the message only if the specified logical condition is true, showcasing the utility of logical operators in controlling program flow.
Switch Case with Logical Conditions
While less common, logical conditions can also be used in `switch` statements, enhancing the clarity and control over the flow of logic:
status = true;
switch status
case true
disp('Status is true');
case false
disp('Status is false');
end
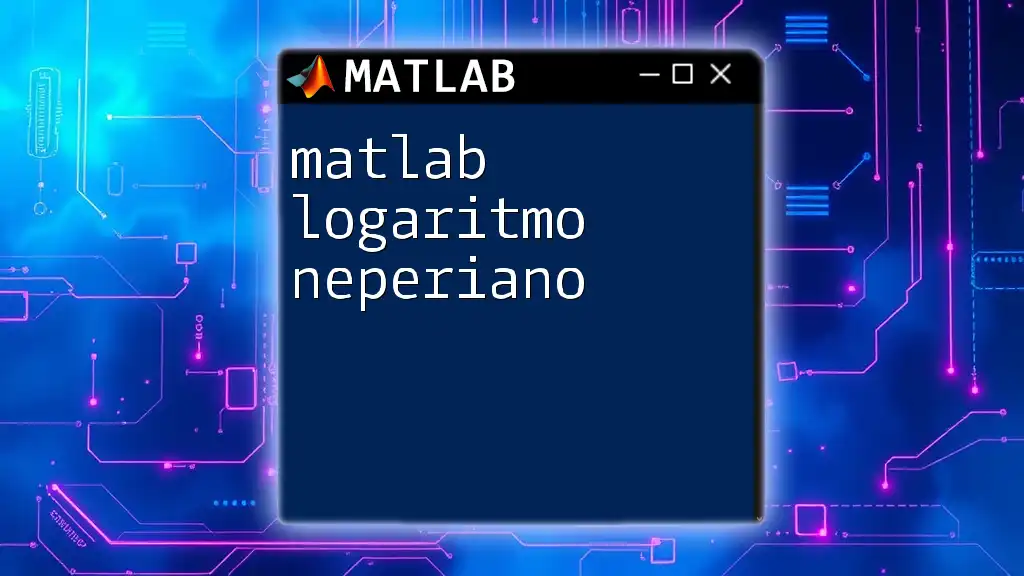
Logical Functions in MATLAB
`any`, `all`, and `find`
MATLAB offers built-in functions like `any()`, `all()`, and `find()` for performing logical operations on arrays effectively.
Here’s how they are utilized:
vec = [false, true, true];
anyResult = any(vec); % Checks if any are true
allResult = all(vec); % Checks if all are true
indices = find(vec); % Finds indices of true values
- `anyResult`: Returns true if there is at least one true value in `vec`.
- `allResult`: Returns true if all values in `vec` are true.
- `indices`: Returns the index positions of the true values, making it easy to locate where conditions are met.
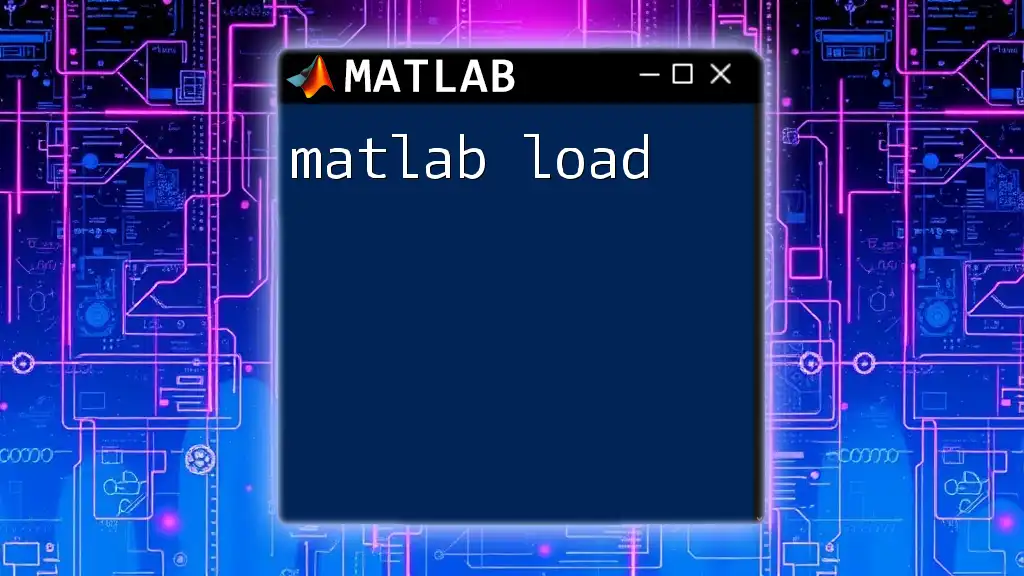
Best Practices and Common Pitfalls
Writing Efficient Logical Code
To enhance the efficiency of your logical code, aim for clarity and simplicity. Avoid unnecessary nesting of conditions. Instead, consider breaking larger conditional statements into smaller, more manageable parts.
Testing Logical Conditions
Thoroughly test your logical conditions to catch potential issues. Utilize MATLAB's debugging tools to trace logic flow and ensure conditions behave as expected. Regular checks not only prevent errors but lead to more robust and maintainable code.
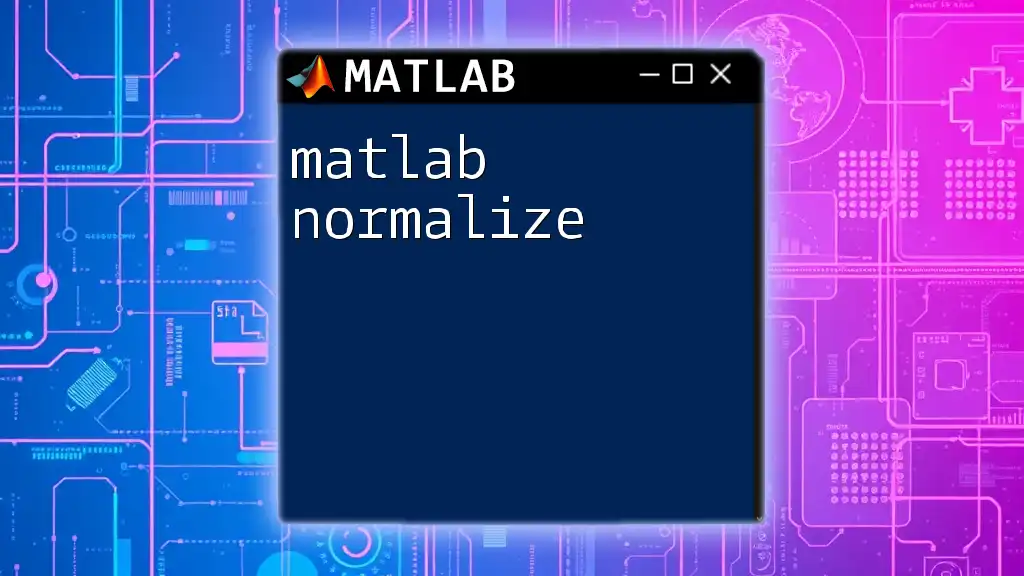
Conclusion
This comprehensive guide on MATLAB logical commands illuminates the essential principles behind logical operations, indexing, and their role in condition checks. Empowering oneself with these tools can significantly enhance both efficiency and clarity in programming tasks. By practicing with the examples provided and diving deeper into MATLAB’s vast functionalities, one can master logical commands quickly and effectively.
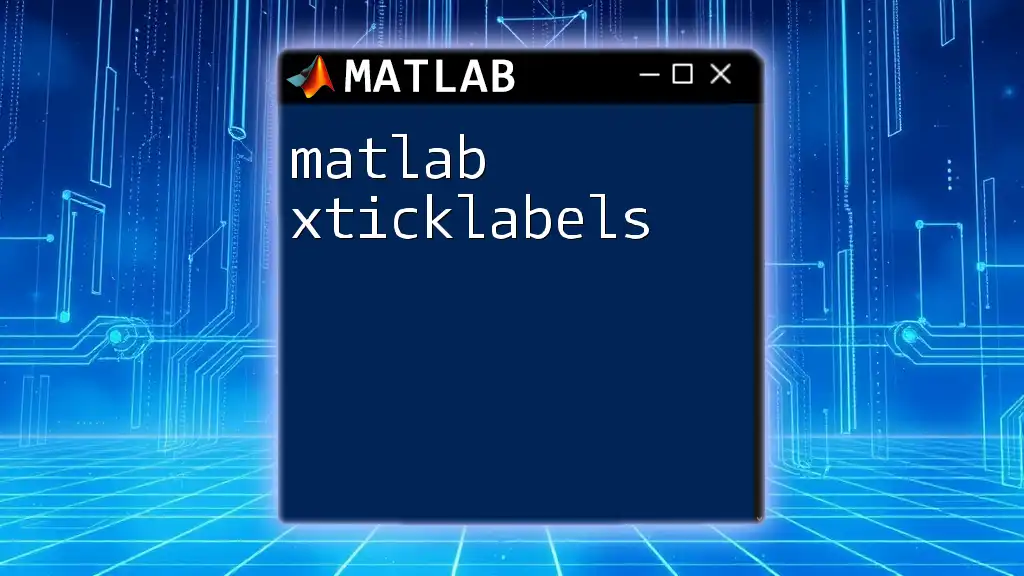
Additional Resources
For further exploration, consider visiting the official MATLAB documentation and exploring comprehensive programming guides available in bookstores or online platforms.