A MATLAB low pass filter allows you to remove high-frequency noise from a signal while preserving its low-frequency components.
Here's a simple example of how to implement a low pass filter using the built-in `lowpass` function in MATLAB:
% Define sample rate and cutoff frequency
Fs = 1000; % Sample rate in Hz
Fc = 100; % Cutoff frequency in Hz
% Create a sample signal and a noisy signal
t = 0:1/Fs:1; % Time vector
signal = sin(2*pi*50*t) + randn(size(t))*0.5; % Original signal with noise
% Apply low pass filter
filtered_signal = lowpass(signal, Fc, Fs);
% Plot the results
figure;
subplot(2,1,1);
plot(t, signal); title('Noisy Signal');
subplot(2,1,2);
plot(t, filtered_signal); title('Filtered Signal');
What is a Low Pass Filter?
A low pass filter (LPF) is a fundamental tool in signal processing used to allow signals with a frequency lower than a specified cutoff frequency to pass through while attenuating higher frequencies. This type of filter is essential when you want to remove high-frequency noise from a signal.
By smoothing out rapid variations and fluctuations, low pass filters help in preserving the essential characteristics of the data, making them invaluable across various applications.
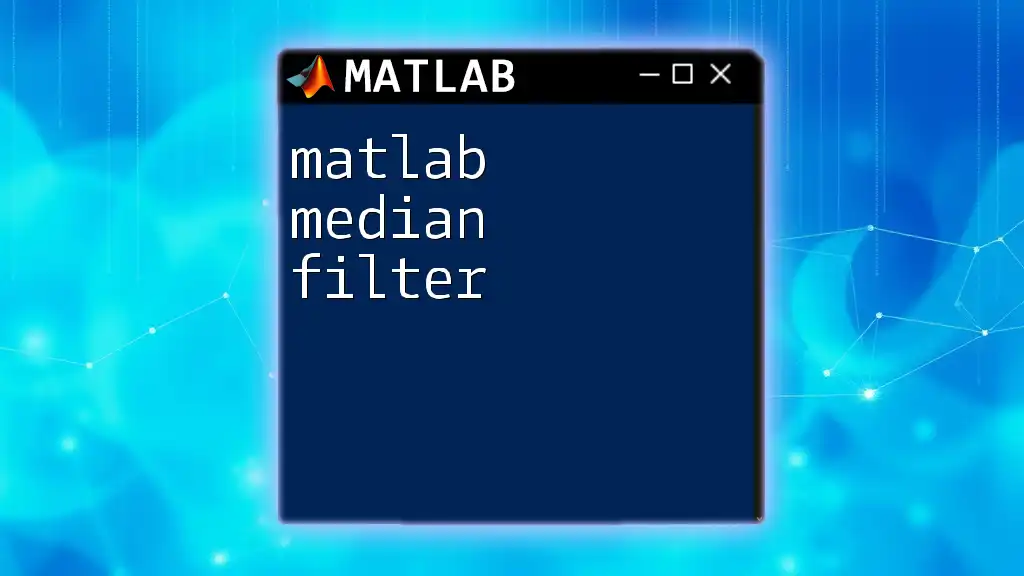
Applications of Low Pass Filters
Low pass filters have widespread applications in several fields:
- Audio Signal Processing: Used in music production to lessen harsh sounds and equalize audio tracks.
- Image Processing: Helps in reducing the noise and smoothing graphical images, making them more visually appealing.
- Data Smoothing: Utilized in economy, finance, and control systems to clarify trends and eliminate volatility.
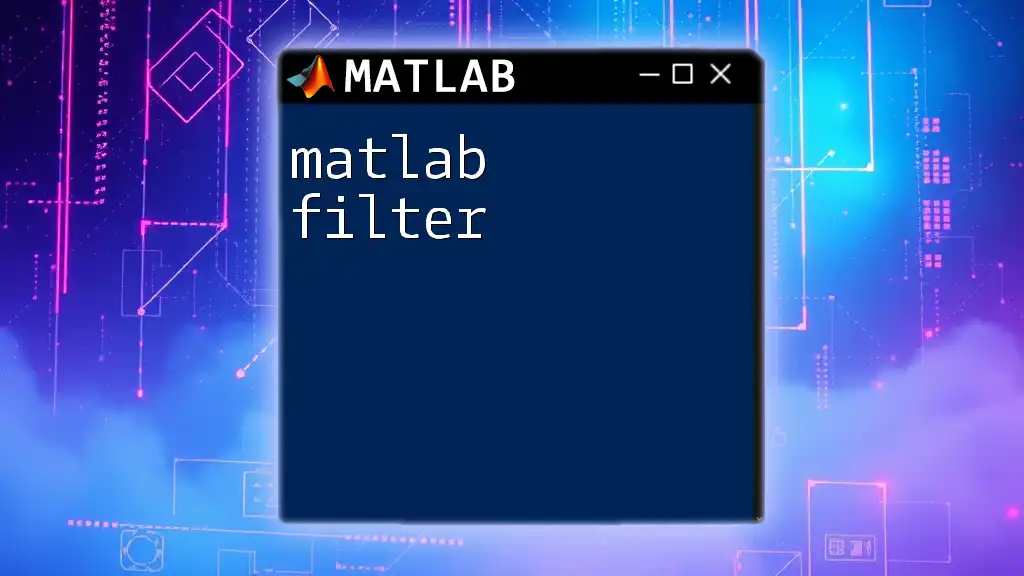
Understanding the Basics of MATLAB
Overview of MATLAB Toolboxes
To effectively create a MATLAB low pass filter, familiarity with the Signal Processing Toolbox is crucial. This toolbox contains functions tailored for designing and implementing filters—ensuring that users can rapidly apply low pass filters with just a few commands. Ensure you have the toolbox installed, which can often be done directly from the MATLAB interface via the Add-Ons Manager.
MATLAB Environment: A Quick Primer
Before diving into filter design, understanding the MATLAB environment is vital. The key components include the Command Window, Editor, and Workspace. The command window allows direct input of MATLAB commands, while the editor enables you to write and save scripts for complex operations. You should be proficient with basic commands such as `plot()`, `fft()`, and `filter()` to navigate through your low pass filter tasks seamlessly.
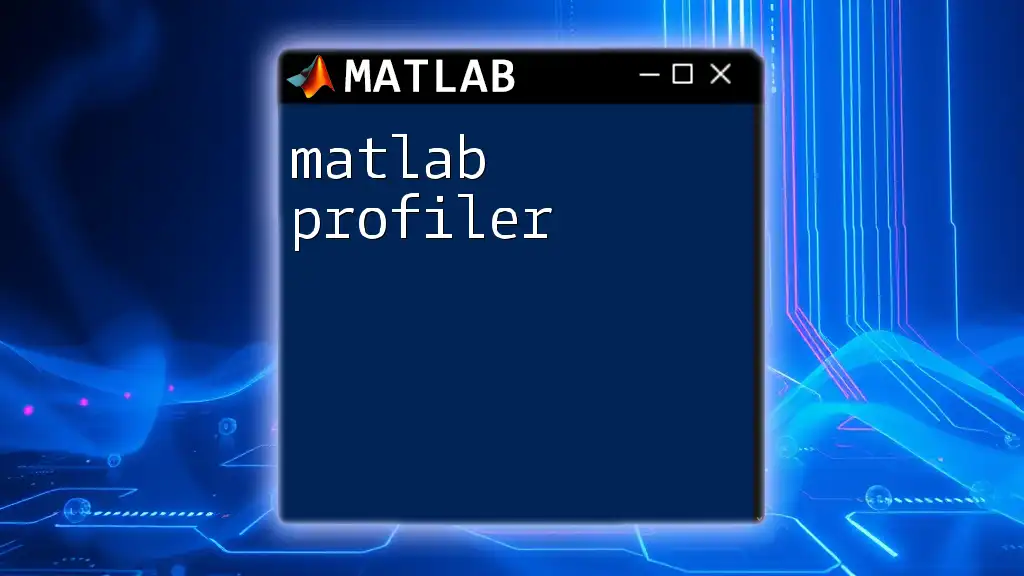
Designing a Low Pass Filter in MATLAB
Choosing the Right Filter Type
When designing a low pass filter, you may choose between two major types: Finite Impulse Response (FIR) and Infinite Impulse Response (IIR) filters.
FIR Filters
FIR filters are known for their stability and straightforward design, making them ideal for applications where phase linearity is required. They rely on a finite set of coefficients for the filter's output. Here is an example of designing a simple FIR low pass filter:
Fs = 1000; % Sampling frequency
Fc = 100; % Cutoff frequency
N = 20; % Filter order
h = fir1(N, Fc/(Fs/2), 'low'); % FIR filter design
In this code, `fir1()` creates an FIR filter of order N that will attenuate frequencies above the cutoff frequency.
IIR Filters
IIR filters, on the other hand, use feedback and can achieve a sharper cutoff with fewer coefficients, but they may have stability issues due to their recursive nature. Here’s how you might design a simple IIR low pass filter:
Fc = 100; % Cutoff frequency
[b, a] = butter(2, Fc/(Fs/2), 'low'); % IIR filter design
The `butter()` function applies a Butterworth design, which provides a smooth frequency response.
Filter Specifications
Cutoff Frequency
The cutoff frequency is a critical parameter of a low pass filter, determining the threshold at which higher-frequency components are attenuated. Setting an appropriate cutoff frequency is essential, as it directly influences how the filter performs and the quality of the output signal.
Filter Order
The filter order defines how many coefficients or taps the filter will use in its design. A higher order results in better frequency response and sharper cutoff characteristics but may introduce delays and computational complexity. It is important to balance filter performance against computational efficiency when selecting your filter order.
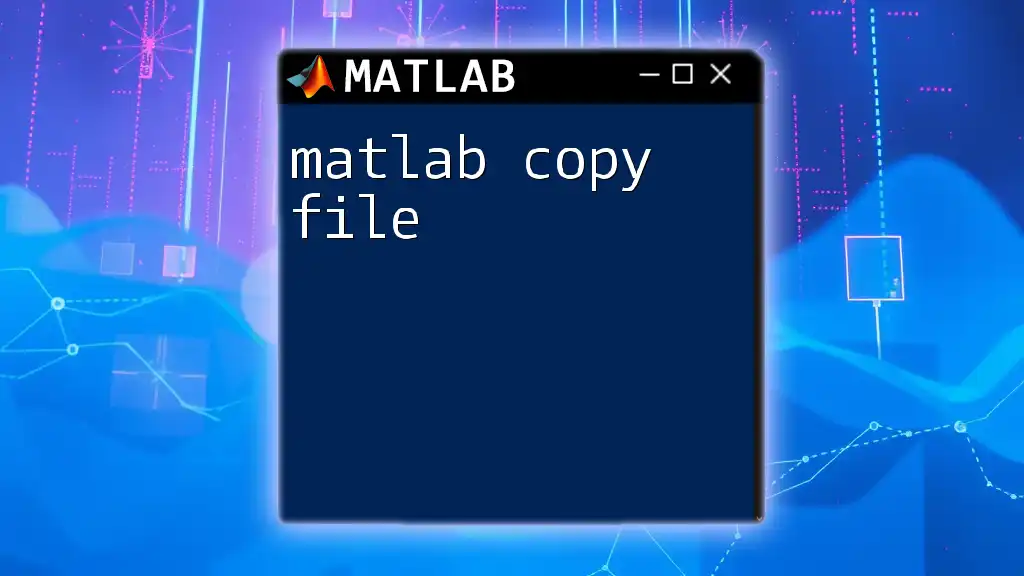
Implementing Low Pass Filters in MATLAB
Step-by-Step Filter Implementation
Step 1: Generate a Sample Signal
To test your low pass filter, begin by generating a sample signal. In this example, we’ll create a noisy sine wave to demonstrate how the filter performs.
t = 0:1/Fs:1; % Time vector
signal = sin(2*pi*50*t) + randn(size(t)); % Sine wave with noise
This code generates a sine wave at 50 Hz and adds Gaussian white noise, creating a signal that is perfect for filtering.
Step 2: Apply the Low Pass Filter
Next, you can apply the low pass filter designed earlier to mitigate the noise in your signal:
filtered_signal = filter(b, a, signal); % Apply IIR filter
This command uses the transfer function coefficients `b` and `a` calculated from the earlier filter design to filter the noisy signal.
Visualizing Filter Performance
Time Domain Analysis
After filtering, it's effective to visualize the results to understand the performance of your low pass filter. You can plot both the original and filtered signals:
figure;
subplot(2,1,1);
plot(t, signal);
title('Original Signal');
subplot(2,1,2);
plot(t, filtered_signal);
title('Filtered Signal');
This will allow for an at-a-glance comparison, making it easy to spot the filtering effects.
Frequency Domain Analysis
To further analyze the filter's impact, it's beneficial to scrutinize the frequency content of both the original and filtered signals using the Fast Fourier Transform (FFT):
Y = fft(signal);
Yf = fft(filtered_signal);
f = (0:length(Y)-1) * Fs/length(Y); % Frequency vector
figure;
plot(f, abs(Y), 'r', f, abs(Yf), 'b');
legend('Original Signal', 'Filtered Signal');
This will provide a clear representation of how the high-frequency components have been suppressed in the filtered signal.
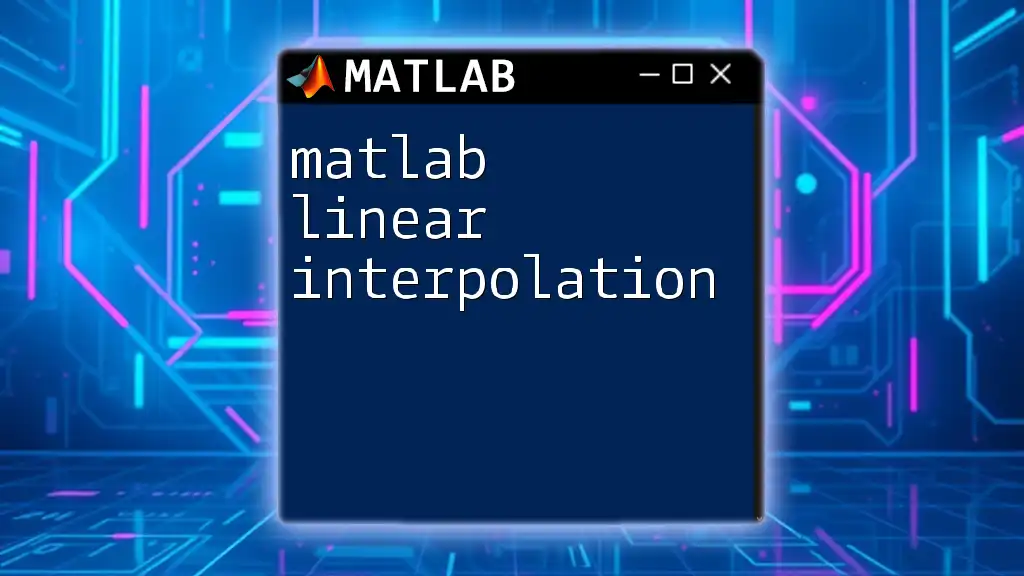
Evaluating Filter Performance
Common Evaluation Metrics
To assess the effectiveness of your low pass filter, consider various evaluation metrics such as Signal-to-Noise Ratio (SNR) and various distortion measures. These metrics will indicate how effectively your filter has improved the signal quality.
Troubleshooting Common Issues
Users may encounter challenges while implementing filters, such as over-filtering or under-filtering. Over-filtering can lead to loss of important signal characteristics, while under-filtering may fail to sufficiently clean up the noise. Awareness of these effects is vital for achieving optimal performance in your MATLAB low pass filter implementations.
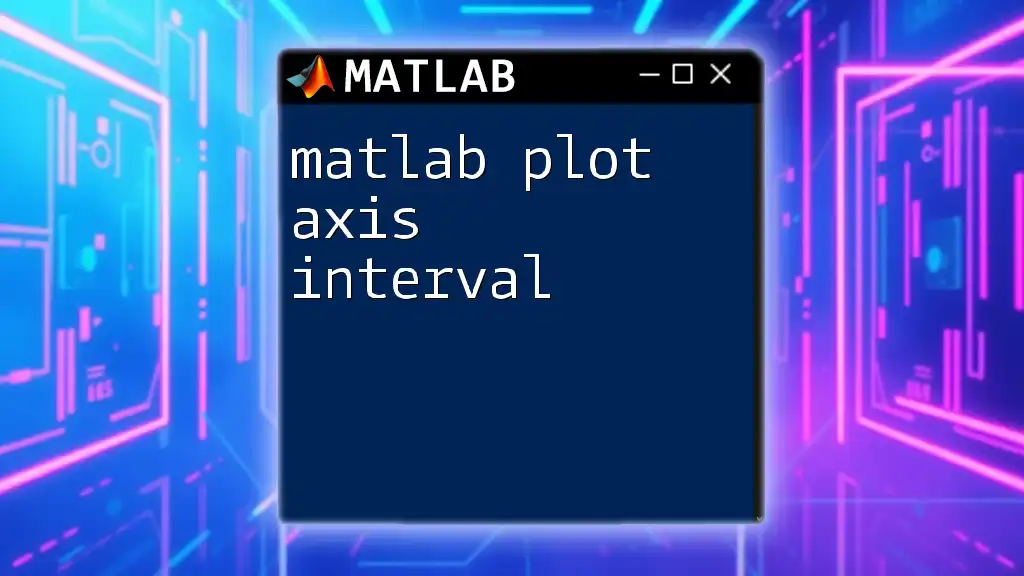
Advanced Topics in Low Pass Filtering
Multiple Filter Design Techniques
Advanced users may explore other methods of designing low pass filters, including the Kaiser window method and the Parks-McClellan algorithm. These approaches involve more sophisticated strategies for determining filter coefficients and can lead to substantial improvements in filter performance.
Adaptive Filters
An exciting frontier in filter design is adaptive filtering. This technique adjusts filter parameters dynamically in response to changing signal characteristics, making it suitable for real-time applications. Beginners can start by exploring simple implementations of adaptive low pass filters and gradually evolve their understanding.
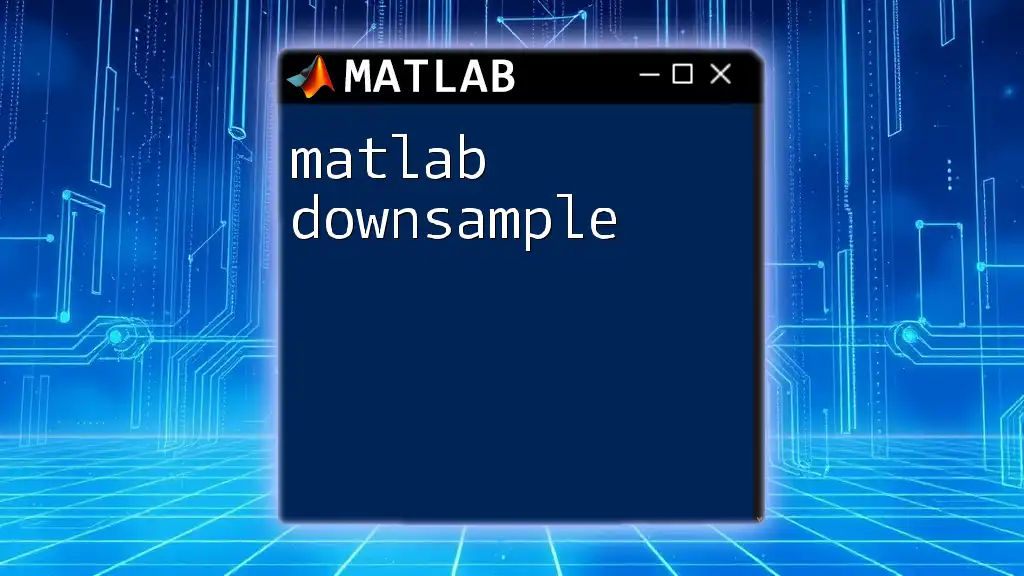
Conclusion
Understanding MATLAB low pass filters is paramount for anyone engaged in signal processing, whether you're working with audio signals, processing images, or smoothing data. By mastering the steps required to design, implement, and evaluate these filters using MATLAB, you can effectively enhance the quality of your data analysis and improve signal integrity.
Further Reading and Resources
For those eager to expand their knowledge further, a plethora of textbooks, online courses, and MATLAB documentation are available to enrich your journey into the world of signal processing.
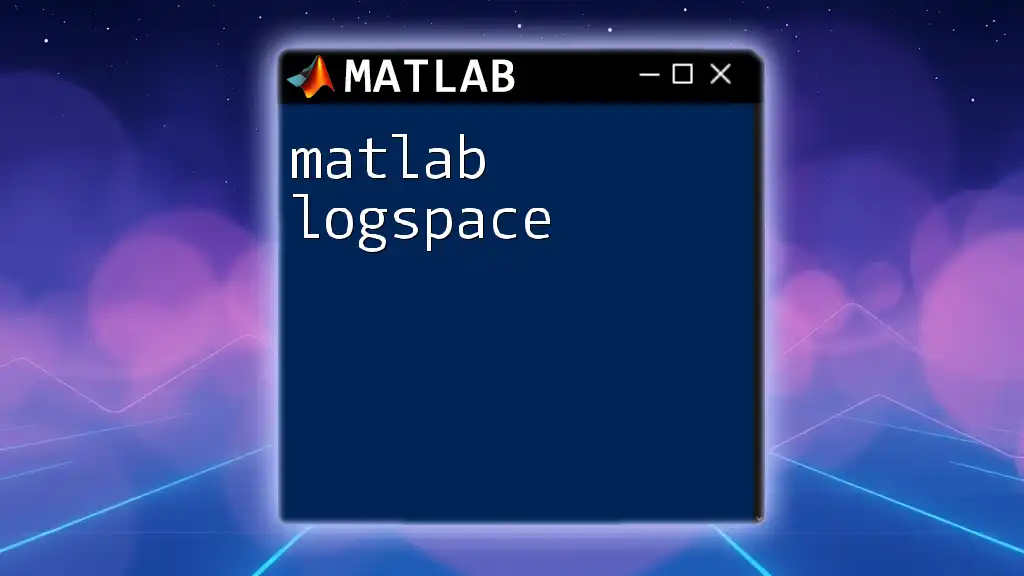
Call to Action
Explore MATLAB’s low pass filter capabilities today and push the boundaries of your projects. Try implementing your unique filters and take your understanding of signal processing to the next level!