The MATLAB logical OR operator (`||` for short-circuit or `|` for element-wise) allows you to evaluate two conditions, returning true if at least one of the conditions is true.
Here’s a code snippet demonstrating the logical OR operator:
% Example of logical OR in MATLAB
a = true;
b = false;
result = a || b; % result will be true
What is Logical OR in MATLAB?
The logical OR operation in MATLAB is a fundamental concept that allows the evaluation of multiple conditions to determine if at least one is true. This becomes especially important in programming and data analysis, where conditions often dictate the flow and execution of code. Logical OR is crucial for decision-making processes, data filtering, and more.
Understanding Logical Operators in MATLAB
Logical operators in MATLAB play a vital role in controlling the logic flow in your code. The primary logical operators available are:
- AND (`&&` and `&`): Checks if both conditions are true.
- OR (`||` and `|`): Checks if at least one condition is true.
- NOT (`~`): Reverses the logical state of its operand.
Logical Values
In MATLAB, logical values are treated as true (1) and false (0). Understanding these values is essential because they impact how expressions and conditions are evaluated. You can also convert numeric values to logical, where any non-zero number is considered true, and zero is false.
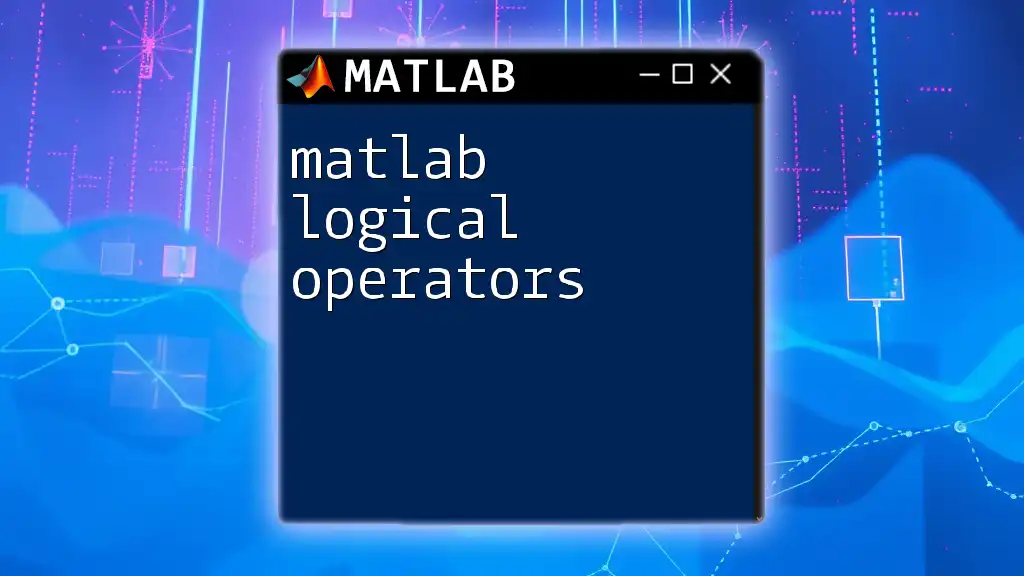
The Logical OR Operator (`||` vs `|`)
In MATLAB, the logical OR operation can be executed using two distinct forms: short-circuit logical OR (`||`) and element-wise logical OR (`|`). It's crucial to understand the differences between them for effective programming.
Short-Circuit Logical OR (`||`)
The short-circuit logical OR operator evaluates conditions in a way that stops evaluating as soon as the result is known. If the first condition is true, MATLAB does not evaluate the second condition, which can enhance performance by avoiding unnecessary computations.
Example of Short-Circuit Logical OR
% Using short-circuit logical OR
a = true;
b = false;
if a || (b = true) % b is not evaluated since a is true
disp('At least one is true');
end
In this example, the statement `(b = true)` doesn't execute because the expression already finds `a` to be true.
Element-wise Logical OR (`|`)
In contrast, the element-wise logical OR operator checks every condition for arrays and matrices. This enables the operation to be performed on corresponding elements across arrays.
Example of Element-wise Logical OR
% Using element-wise logical OR
A = [1, 0, 1];
B = [0, 0, 1];
C = A | B; % Element-wise comparison
disp(C);
The output of this operation would be `[1, 0, 1]`, where the function returns true (1) for elements where either `A` or `B` has a true value.
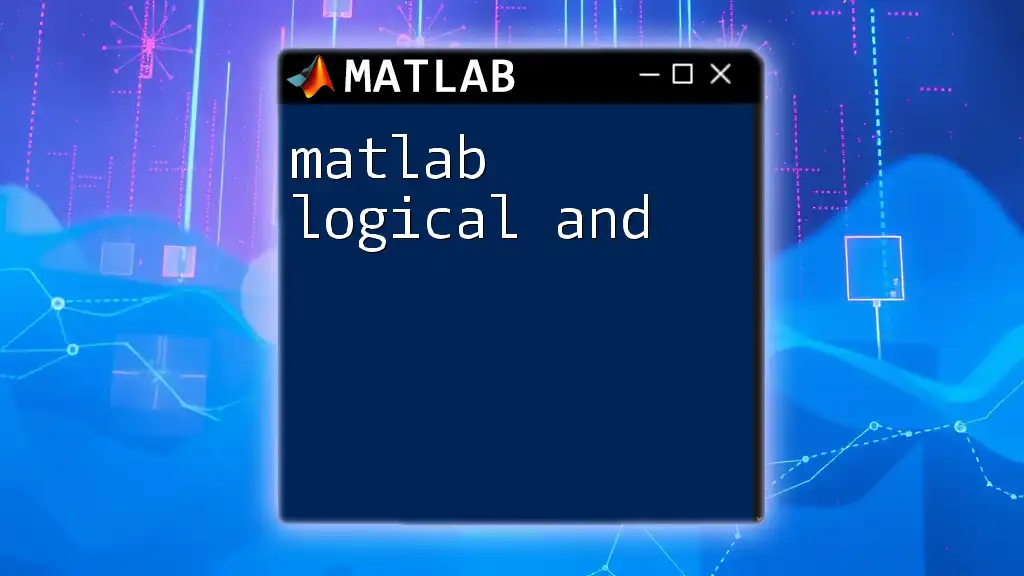
How to Use Logical OR in Conditions
Using logical OR in conditional statements can significantly streamline your code by combining multiple criteria into a single logical expression.
Using Logical OR in if Statements
It's common to use logical OR in if statements to check for conditions that might be true.
Simple Condition Example
x = 5;
if x < 3 || x > 7
disp('x is outside the range of 3 to 7.');
else
disp('x is within the range.');
end
In this example, the program evaluates whether `x` falls outside the specified range. If so, it displays the corresponding message.
Combining Multiple Conditions
You can also combine multiple conditions for more complex validations.
Example of Multiple Conditions
y = -4;
if y < -10 || y > 10 || mod(y, 2) == 0
disp('y meets one or more criteria.');
end
Here, the program checks not just if `y` is out of the defined range but also whether it is even. The flexibility of logical OR allows for more comprehensive condition checks.
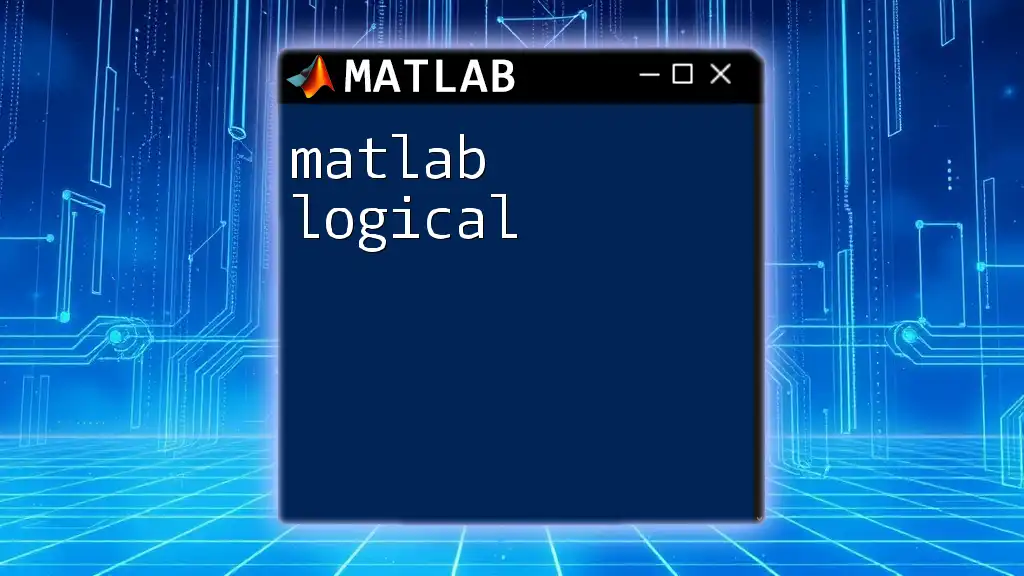
Practical Applications of Logical OR
The matlab logical or is widely applicable, especially in data analysis tasks.
Data Filtering
One of the most practical uses of logical OR is in logical indexing for filtering datasets. This approach allows us to extract specific subsets of data based on conditions.
data = [1, 2, 3, 4, 5, 6];
filteredData = data(data < 3 | data > 5);
In this code snippet, `filteredData` contains values that are either less than 3 or greater than 5, demonstrating how logical OR can efficiently filter data points.
Creating Logical Masks
Logical masks are another valuable application of the logical OR operator. A logical mask can determine which elements in an array meet any given criteria.
mask = (data < 2) | (data > 5);
disp(data(mask)); % Only shows values outside the range
This filter shows the versatility of the logical OR in isolating data points that meet specific conditions, further improving data manipulation capabilities.
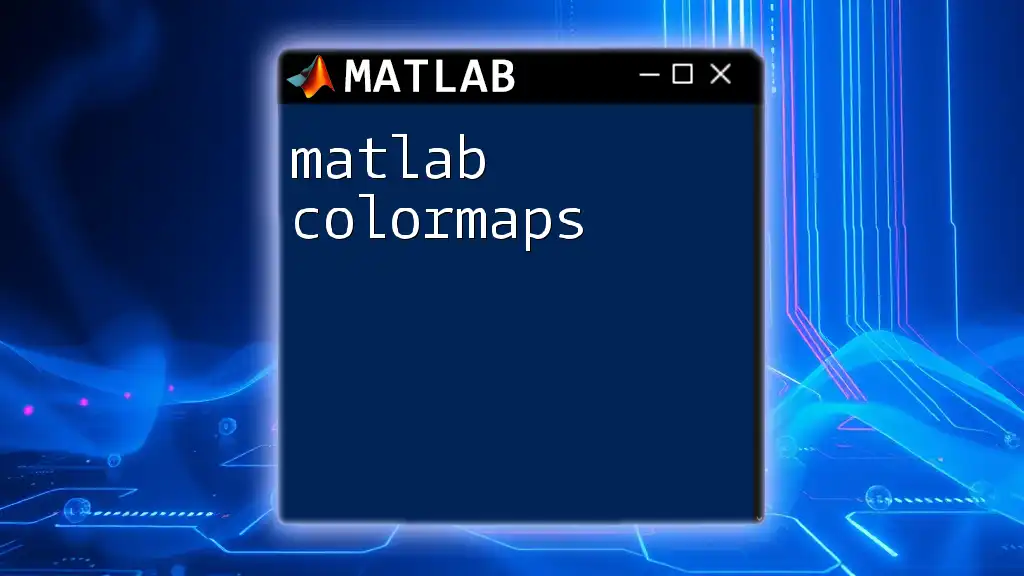
Best Practices for Using Logical OR in MATLAB
When utilizing the matlab logical or, consider these best practices:
Readability Matters
Always strive for clarity in your conditionals. For instance, break complex conditions into multiple lines or use intermediate variables to ensure that your code is easy to understand.
Performance Considerations
Knowing when to use `||` versus `|` is crucial. Use `||` for simple, boolean evaluations and `|` when performing element-wise operations on arrays. This choice can impact your code’s performance, especially when processing large datasets.
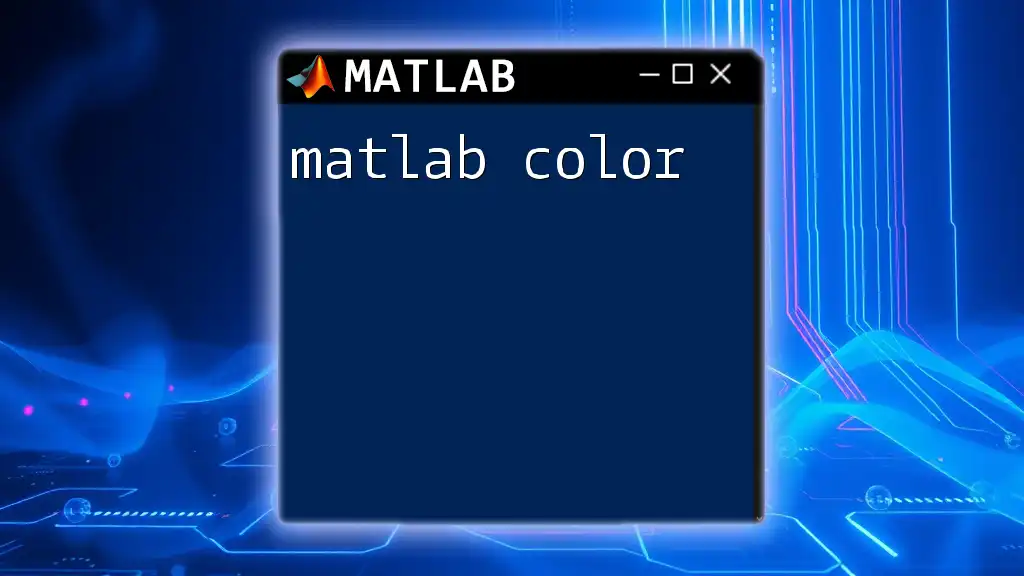
Common Pitfalls and How to Avoid Them
As with any programming concept, there are several pitfalls to be aware of when using logical OR.
For instance, misusing logical operators can lead to unintended logic errors. Always test the edge cases of your conditions to ensure they behave as expected.
Debugging Tips
When troubleshooting logic errors, utilize MATLAB’s debugging tools. Set breakpoints to examine variable values during execution, helping isolate where the logic might be faulty.
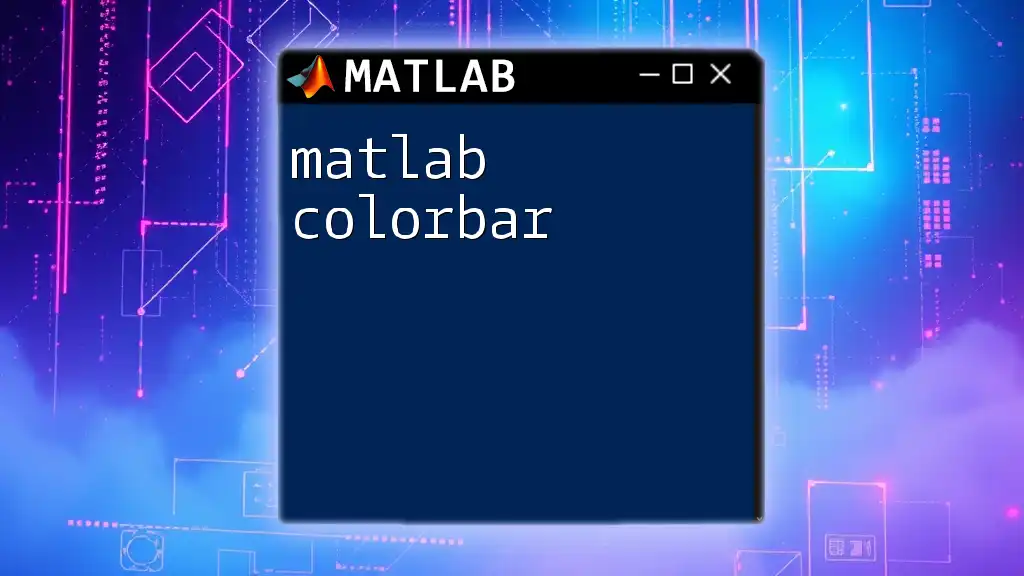
Conclusion
The matlab logical or operation is a crucial component in programming that allows you to evaluate multiple conditions effectively. Understanding the differences between short-circuit and element-wise logical OR can refine your coding techniques, improve your programming performance, and enhance the accuracy of your data analysis. By incorporating these principles into your MATLAB practices, you become more proficient and agile in manipulating data and controlling program flow.
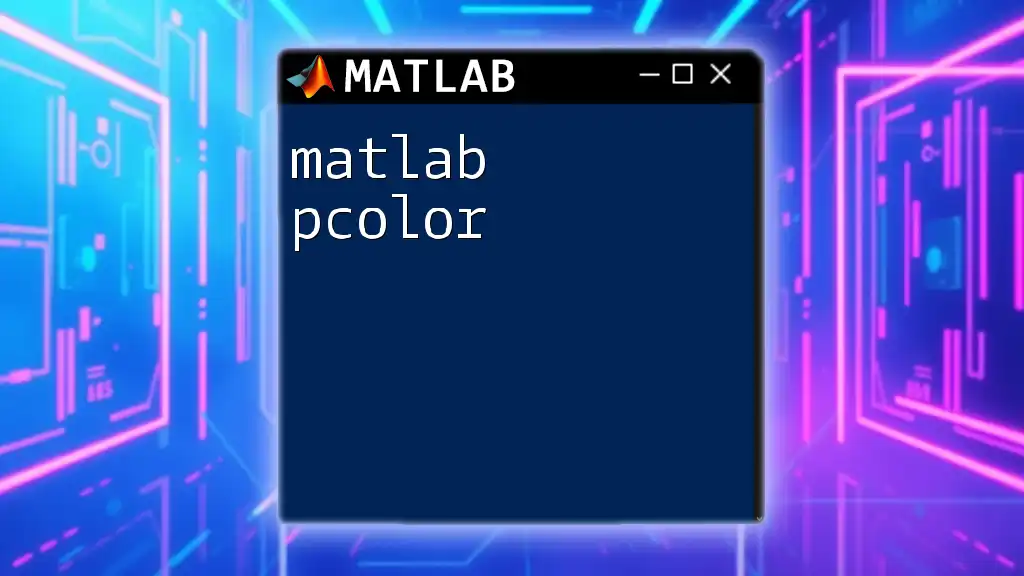
Additional Resources
For deeper understanding, explore links to official MATLAB documentation, recommended tutorials, and forums where you can engage with other MATLAB users to further enhance your skills.