The MATLAB logical `and` operator is used to perform element-wise logical conjunction on arrays, returning `true` only when both operands are `true`.
Here's a code snippet demonstrating its usage:
A = [true, false, true];
B = [true, true, false];
C = A & B; % C will be [true, false, false]
Understanding Logical AND in MATLAB
What is Logical AND?
The logical AND operation is a fundamental construct in programming that evaluates multiple conditions simultaneously. In MATLAB, logical AND is used to combine two boolean expressions, returning `true` only if both expressions are `true`.
Syntax of the Logical AND Command
In MATLAB, you can perform logical AND operations using two different operators: `&` and `&&`.
- The `&` operator performs element-wise AND operations between arrays, meaning if you apply it to arrays, each corresponding element will be evaluated.
- The `&& operator is used for short-circuit evaluation only with scalar logical values. This means that if the first condition is `false`, the second condition won’t be evaluated because the overall outcome cannot be true.
Understanding when to use each operator can help in writing efficient and effective code.
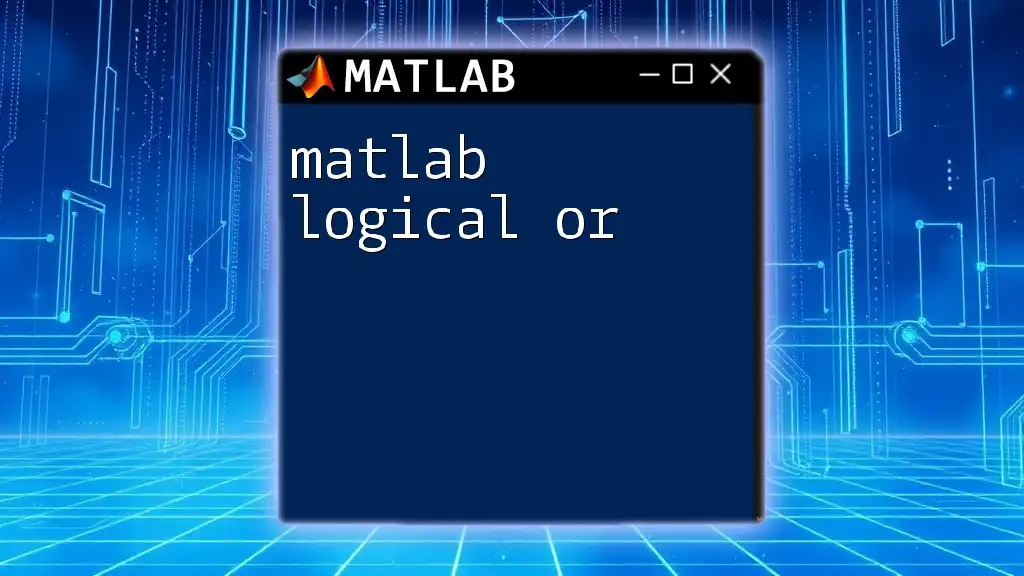
Using Logical AND with Boolean Arrays
Creating Boolean Arrays
Before diving into logical AND operations, it's crucial to understand how to create boolean arrays in MATLAB. You can create boolean arrays by applying relational operators, such as `<`, `>`, `==`, etc.
For example:
A = [1, 2, 3, 4];
B = [2, 3, 4, 5];
logicalArray = (A > 1) & (B < 5);
In this example, `logicalArray` will evaluate to `[0, 1, 0, 1]`, representing `false`, `true`, `false`, `true`, respectively, since only the second and fourth elements satisfy both conditions.
Performing Logical AND on Boolean Arrays
Once you have boolean arrays, you can apply the logical AND operation directly to them. For instance:
C = logical([0, 1, 0, 1]); % Example boolean vector
D = logical([1, 1, 0, 0]);
result = C & D;
The output, `result`, will be `[0, 1, 0, 0]`, showing that only the second element in both arrays is true.
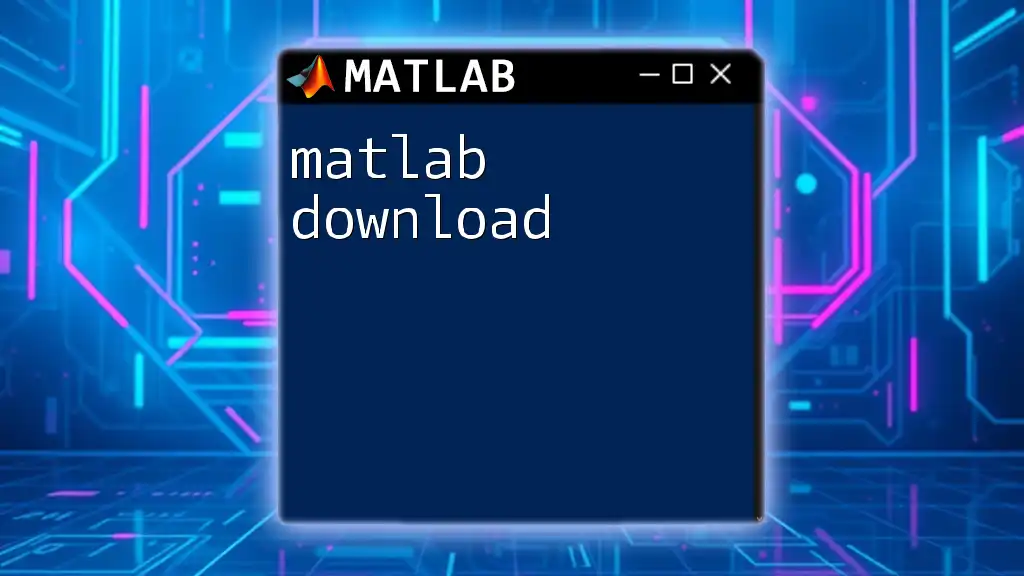
Conditional Statements and Logical AND
Using Logical AND in If Statements
Logical AND can be invaluable in conditional statements, providing a way to ensure that multiple criteria are met before executing a block of code.
Consider this example:
x = 5;
y = 10;
if (x > 0) && (y > 5)
disp('Both conditions are true');
end
This code will display the message since both conditions evaluate to `true`.
Practical Example: Filtering Data
Logical AND operations are particularly useful when filtering datasets. For example, let’s say you have a matrix and you want to extract certain rows based on conditions:
data = [1, 2; 3, 4; 5, 6; 7, 8];
filter = (data(:,1) > 2) & (data(:,2) < 7);
filteredData = data(filter, :);
In this case, `filteredData` will contain rows from `data` where the first column is greater than `2` and the second column is less than `7`. It’s a practical application of combining multiple conditions to finely filter data.
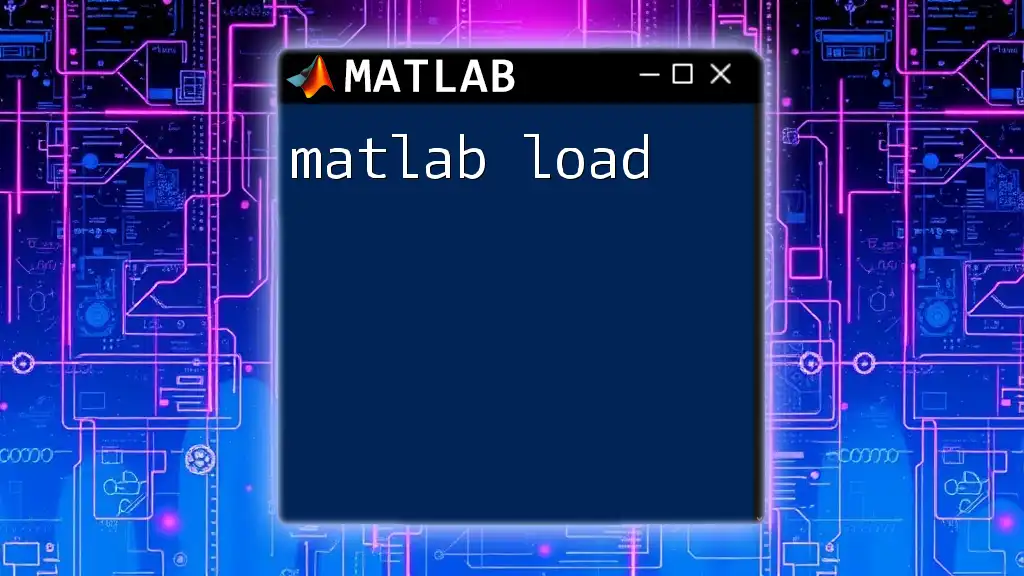
Debugging Common Issues with Logical AND
Common Pitfalls
When using logical AND, many beginners may encounter some common mistakes, particularly with the usage of operators. For instance:
% Incorrect
if (x > 0 & y > 5)
disp('Condition may not behave as expected');
end
In this case, using a single `&` with non-logical outputs may lead to unexpected behavior. It's crucial to ensure all expressions return boolean values.
Troubleshooting Techniques
When debugging logical conditions, make use of MATLAB's built-in debugging tools. Step through your logic visually and evaluate variables at different stages to ensure they hold the expected boolean values.
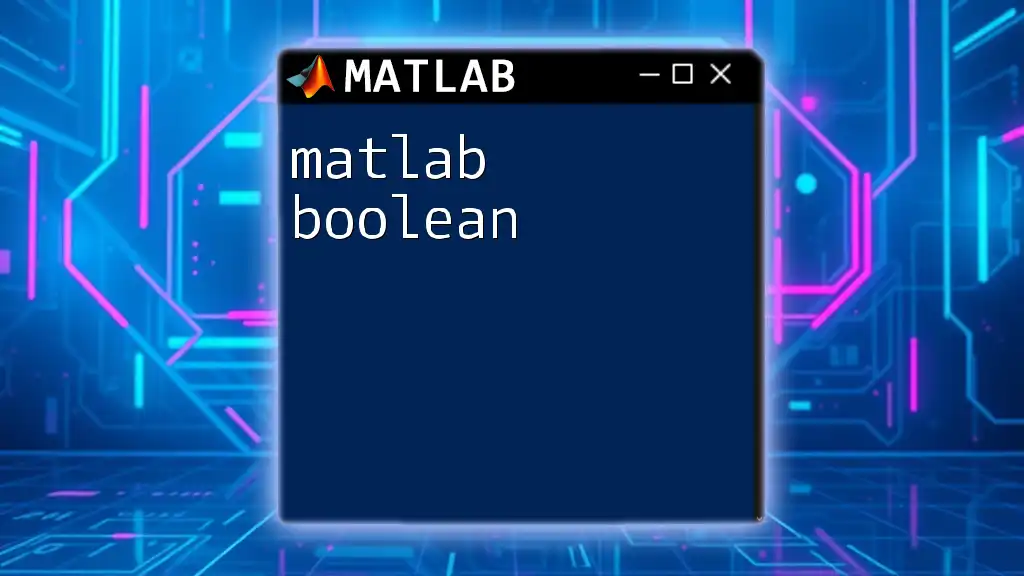
Performance Considerations
Efficiency of Logical Operators
When writing code, making informed choices about which logical operator to use can significantly impact performance. The `&&` operator, while slightly faster due to short-circuiting, can only be used with scalar logical values. On the other hand, `&` can handle array operations effectively.
Vectorization Benefits
Using logical AND within vectorized operations contributes to overall code speed by avoiding explicit loops. For example, comparing two datasets directly with logical conditions can be done in one concise statement, enhancing both readability and performance.
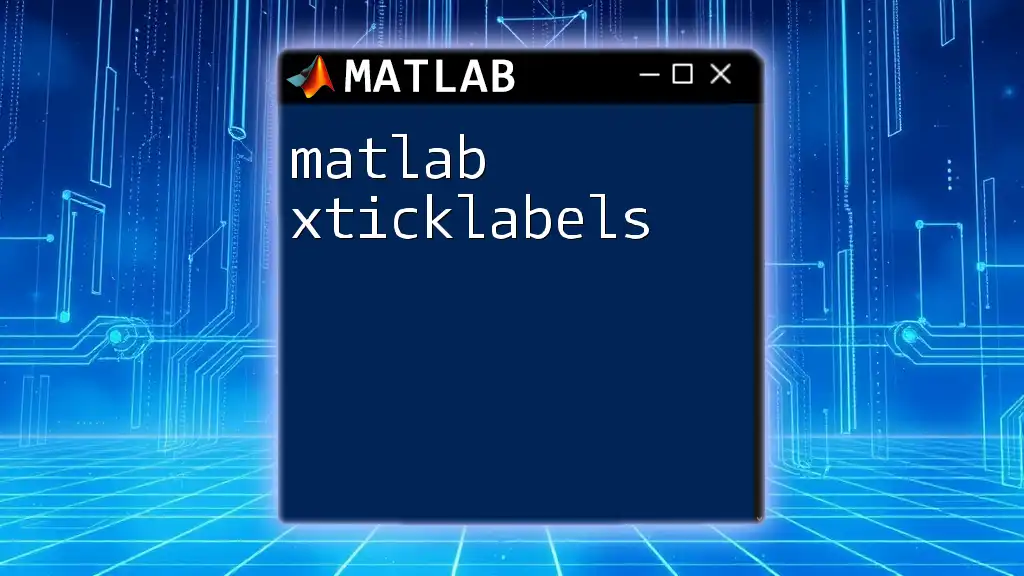
Conclusion
Understanding and mastering the concept of matlab logical and is crucial for effective programming in MATLAB. As you delve deeper into logical operations, you realize their importance in writing conditions, filtering data, and controlling program flow. By fully grasping logical AND's capabilities, you pave the way for more complex programming tasks in MATLAB.
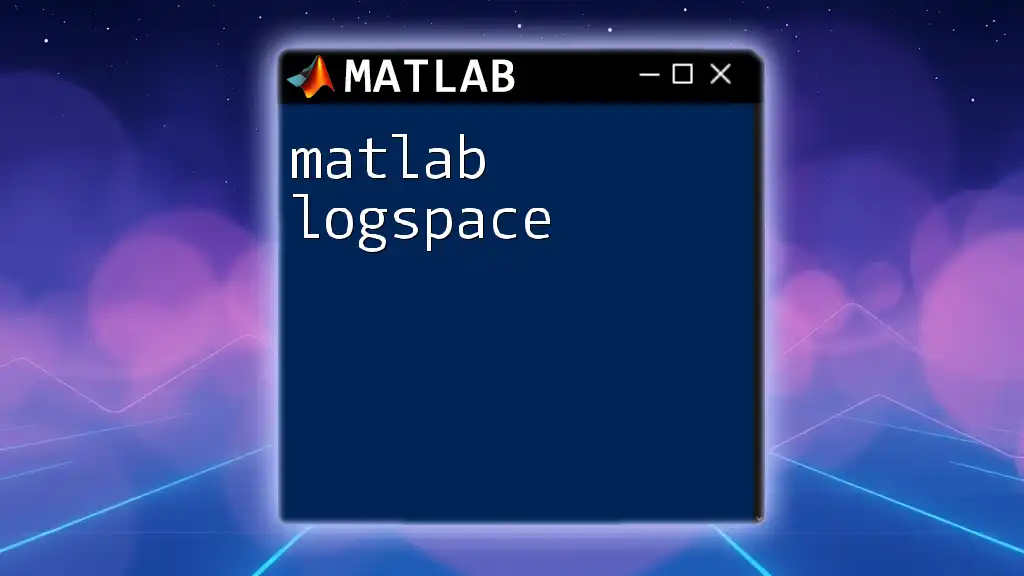
Additional Resources
For those looking to further their knowledge regarding logical operations, consider reading advanced MATLAB documentation or exploring specialized MATLAB programming courses.
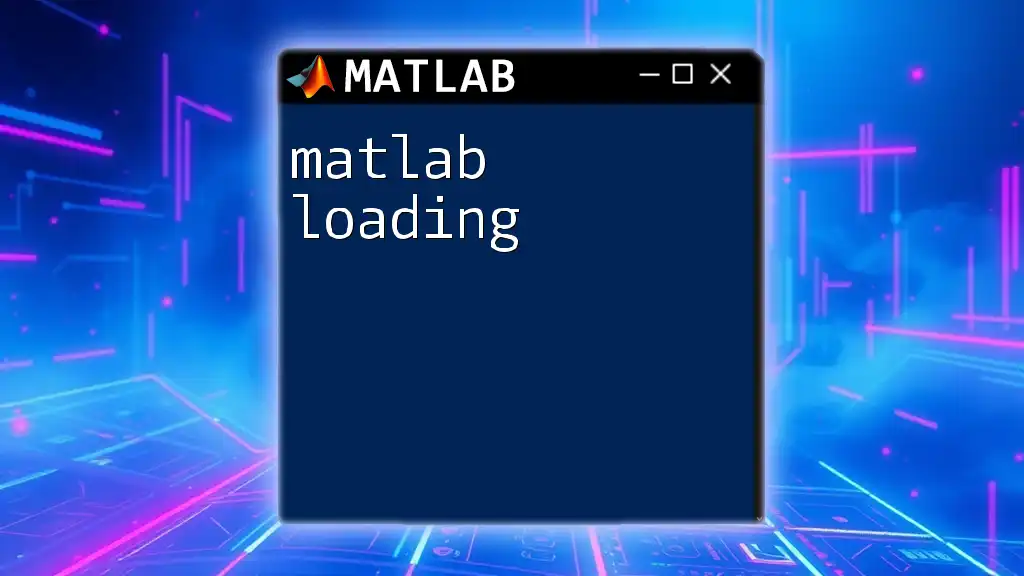
Call to Action
We encourage you to experiment with your logical AND examples and share your experiences. Join our courses to enhance your MATLAB skills and transform your programming abilities!