The `max` function in MATLAB returns the largest element in an array or the largest element along a specified dimension, enabling efficient data analysis.
A = [3, 5, 1; 8, 2, 9];
maxValue = max(A(:)); % Finds the maximum value in the array A
Understanding the Basics of `max`
What is `max` in MATLAB?
The `max` function in MATLAB is a built-in function used to determine the maximum value in an array or set of values. Its main purpose is to provide a straightforward way to extract the highest numerical value from various types of data structures.
Syntax Overview: The basic syntax of the `max` function is as follows:
M = max(A)
Here, `A` represents the input array, and `M` is the output that contains the maximum value found in `A`.
Types of Inputs Accepted by `max`
The `max` function is versatile and can handle different data types, including:
-
Vectors: A one-dimensional array, such as:
vector = [1, 5, 3, 9, 2]; max_value = max(vector); % Output: 9
-
Matrices: A two-dimensional array, like:
matrix = [1, 5, 3; 9, 2, 7]; max_value_matrix = max(matrix); % Output: [9, 5, 7]
-
Multidimensional arrays: The `max` function can also be utilized for arrays with more than two dimensions, allowing for greater flexibility in data analysis.
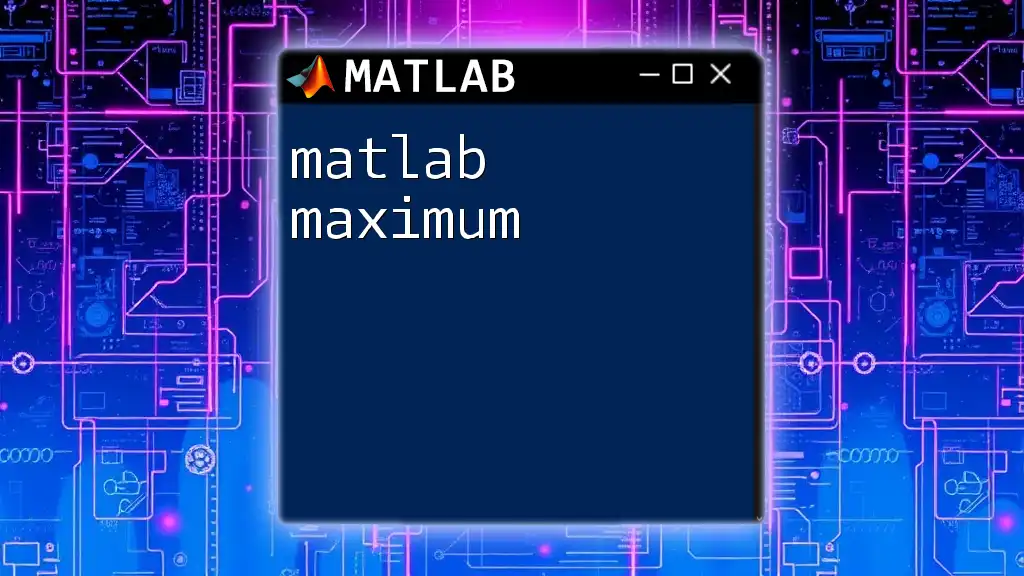
Using the `max` Function
Basic Usage of `max`
One-Dimensional Vectors
For one-dimensional arrays, `max` simply scans through the elements to find the highest value.
vector = [1, 5, 3, 9, 2];
max_value = max(vector); % Output: 9
The output is straightforward: `max_value` holds the maximum from the given vector.
Two-Dimensional Matrices
When working with 2D matrices, `max` returns the maximum value for each column.
matrix = [1, 5, 3; 9, 2, 7];
max_values_per_column = max(matrix); % Output: [9, 5, 7]
The result here is that each column's maximum is returned as a row vector.
The `max` Function with Multiple Dimensions
When using `max` on multidimensional arrays, you can take advantage of its flexibility.
For example:
array_3d = cat(3, [1, 2; 3, 4], [5, 6; 7, 8]);
max_value_3d = max(array_3d(:)); % Output: 8
In this case, we flattened the array into a single column to find the maximum value efficiently.
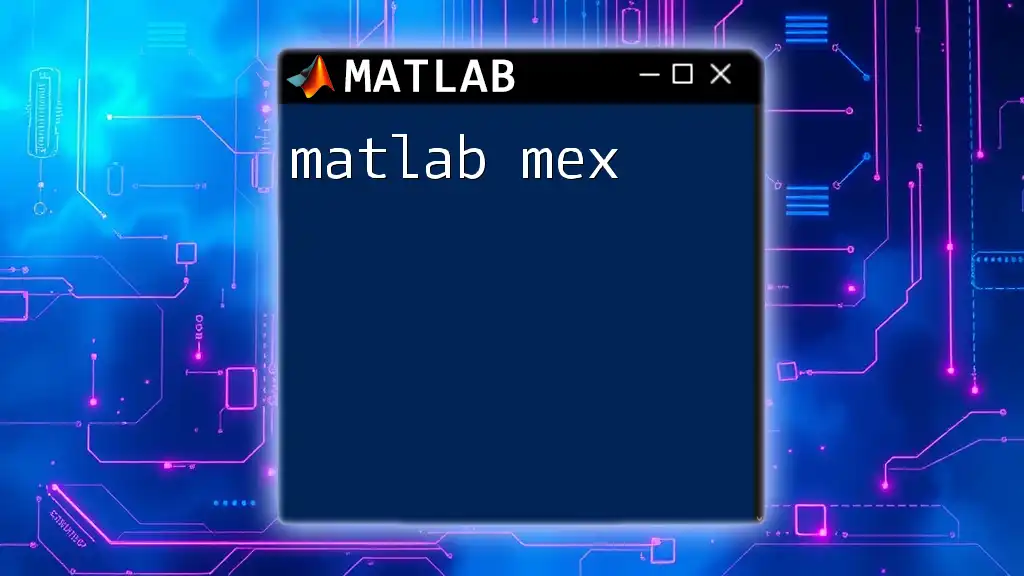
Advanced Features of `max`
Using `max` with Additional Output Arguments
The `max` function can also return the index of the maximum value, which is particularly useful for tracking the position of the maximum in your data.
vector = [1, 5, 3, 9, 2];
[max_value, index] = max(vector); % Output: max_value = 9, index = 4
In this example, `index` tells us that the maximum value of `9` is located at the fourth position in the vector.
Finding Maximum Values Along Specific Dimensions
The `max` function can be specified to return the maximum along a certain dimension in a matrix.
Suppose we have the following 2D array:
matrix = [1, 5, 3; 9, 2, 7];
max_value_rows = max(matrix, [], 1); % Maximum values for each column
max_value_columns = max(matrix, [], 2); % Maximum values for each row
In this case, `max_value_rows` would return `[9, 5, 7]`, while `max_value_columns` would give `[5; 9]`, allowing for tailored data analysis.
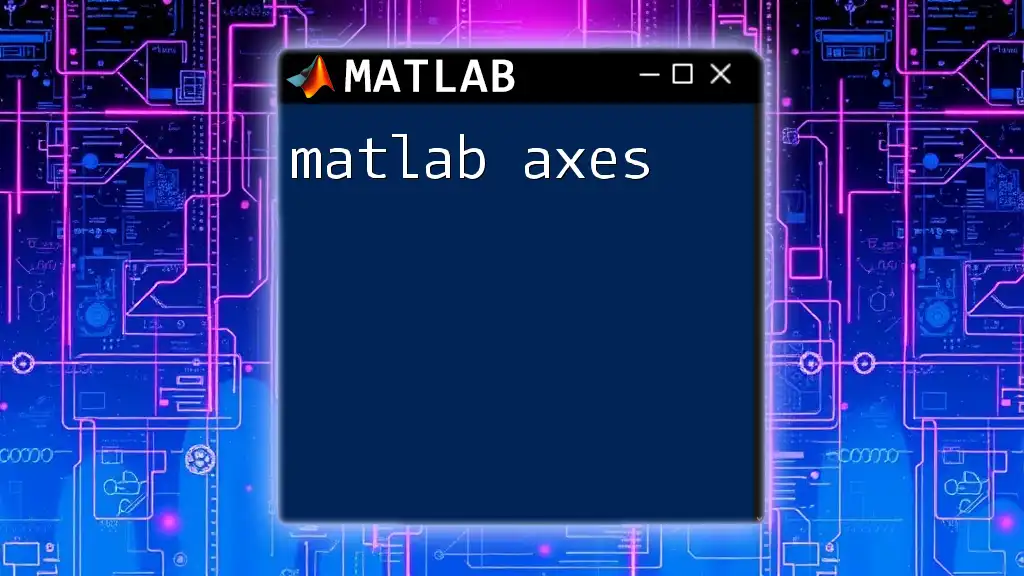
Combining `max` with Other Functions
Using `max` in Data Analysis
In data analysis, the `max` function can be combined with other statistical functions to derive meaningful insights. For example:
data = [5, 20, 15; 10, 30, 25];
max_value = max(data(:)); % Find the overall max value
mean_value = mean(data(:)); % Compute the mean value
This snippet retrieves the maximum and mean values from a dataset, allowing for a quick summary of the data.
Logical Arrays with `max`
Interestingly, `max` can also handle logical arrays. For instance:
logical_array = [0, 1, 0; 1, 0, 1];
max_logical = max(logical_array); % Output: 1
In this case, `max_logical` evaluates to `1`, indicating the presence of true values (non-zero) in the logical array.
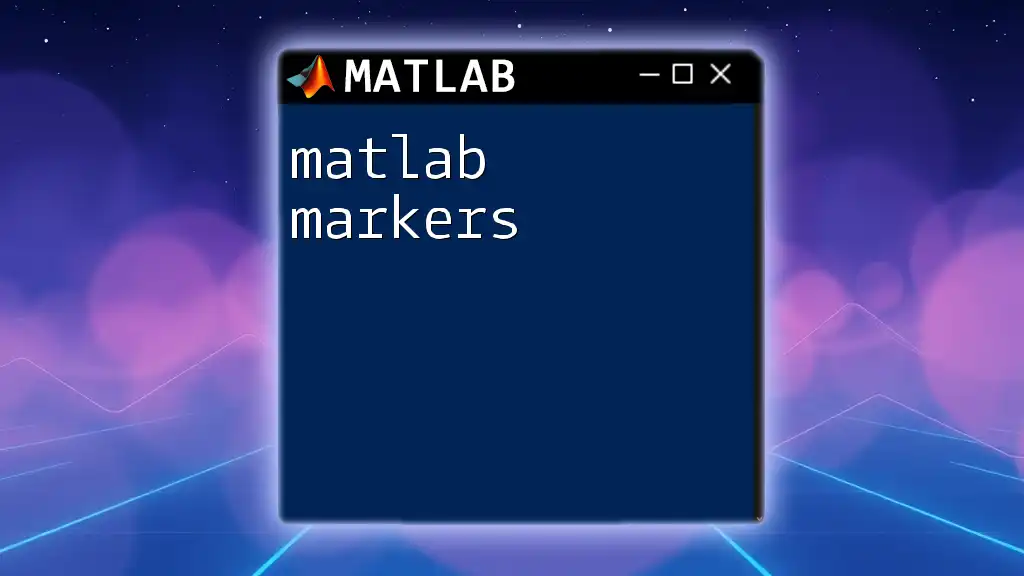
Common Pitfalls and Errors
Errors Related to `max`
While using `max`, programmers may encounter some common issues, such as:
-
Mismatched Dimensions: If the dimensions of the arrays being compared do not match, MATLAB will throw an error.
-
Non-Numeric Input: It is crucial to ensure that the input to `max` is numeric, as other data types (e.g., strings or cell arrays) will lead to errors.
Best Practices for Using `max`
To maintain code efficiency and readability, consider the following best practices:
- Use descriptive variable names alongside concise functions to simplify your code.
- Ensure proper documentation and comments in your code, making it easier for others (or yourself) to understand later.
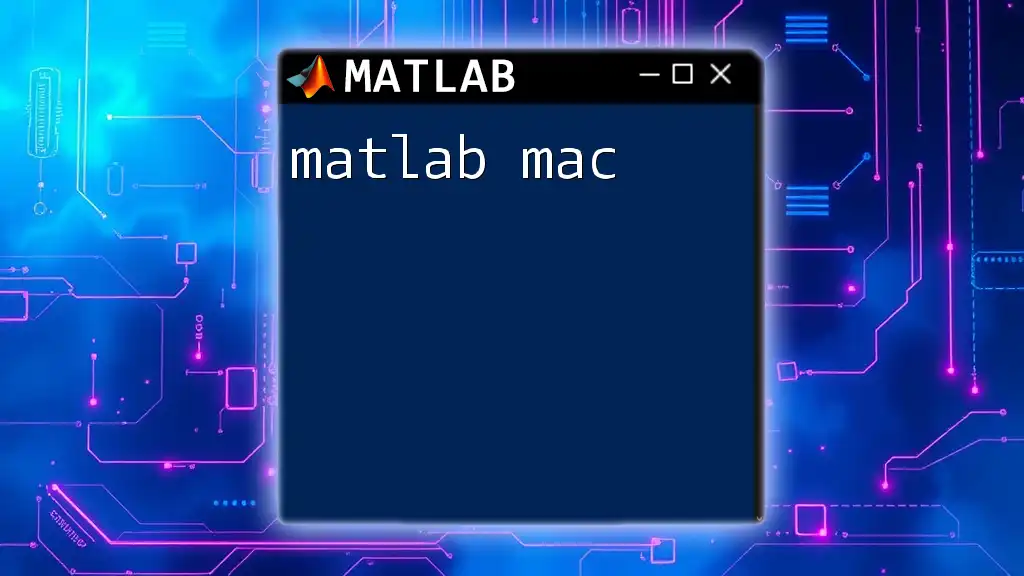
Real-World Applications of `max`
Engineering Applications
In signal processing, the `max` function serves a vital role. For instance, determining the peak level of a signal is crucial in many engineering fields, such as telecommunications and audio engineering.
By applying `max` to a series of sampled values from a signal, engineers can assess the maximum amplitude, crucial for optimizing transmission quality and integrity.
Statistical Analysis
In data science, the `max` function is indispensable when interpreting datasets. For instance, if you are analyzing survey results, you can easily extract the highest ratings or scores, which can help identify top-performing products or services.
survey_results = [4.5, 3.5, 5.0, 4.0];
highest_rating = max(survey_results); % Output: 5.0
Here, extracting the maximum rating allows you to quickly identify the best-rated item.
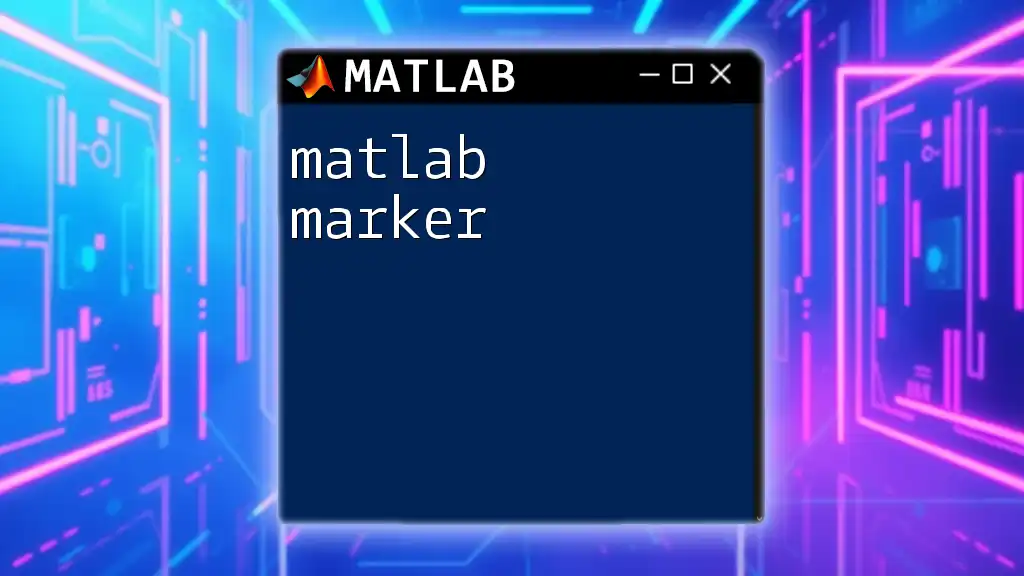
Conclusion
Recap of the `max` Function
The `max` function in MATLAB is a versatile tool for identifying maximum values across various data types, thereby enhancing data analysis in engineering, statistics, and many other fields.
Encourage Further Exploration
In addition to `max`, MATLAB has a rich array of functions, including `min`, `sort`, and `find`, which can complement your data analysis toolkit. Exploring these functions further can greatly expand your MATLAB capabilities.
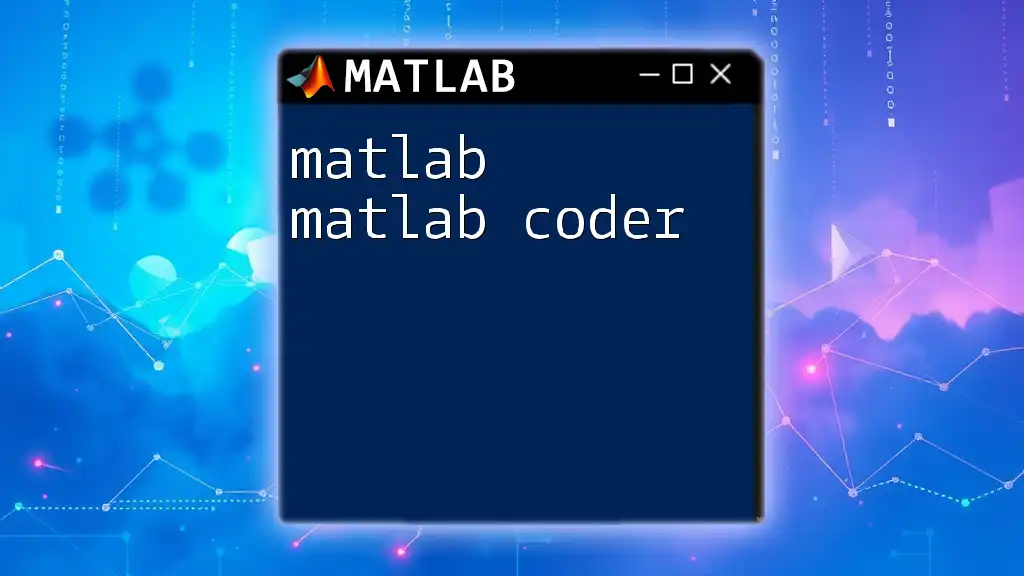
Additional Resources
Links to MATLAB Documentation
For further reading and more detailed explanations, consider visiting the official [MATLAB documentation for `max`](https://www.mathworks.com/help/matlab/ref/max.html).
Recommended Tutorials
You might also find helpful tutorials on platforms like Coursera, edX, and YouTube, covering various aspects of MATLAB programming.
Community Contributions
Feel free to share your own examples or questions about using the `max` function in MATLAB in the comments section below. Engaging with the community can provide valuable insights and different approaches!