The MATLAB modulus operator, denoted by `mod`, returns the remainder after division of one number by another.
Here's a code snippet demonstrating its use:
result = mod(17, 5); % This will return 2 since 17 divided by 5 has a remainder of 2
What is Modulus in MATLAB?
Definition of Modulus
The modulus operation, often denoted as "mod," is an essential mathematical function that provides the remainder of a division between two numbers. In MATLAB, this operation allows us to determine how much is "left over" after dividing one number by another. It plays a critical role in programming, particularly in scenarios involving cycles, even-odd checks, and more complex algorithms.
Understanding Modulus Operation
In its simplest form, the modulus operation is akin to performing integer division and retaining the remainder. When dividing a number \(a\) by another number \(b\), the modulus operation gives us the value that remains after \(a\) is divided by \(b\). This is particularly useful in various mathematical and programming contexts, assisting in scenarios ranging from looping through array indices to managing time-based calculations.
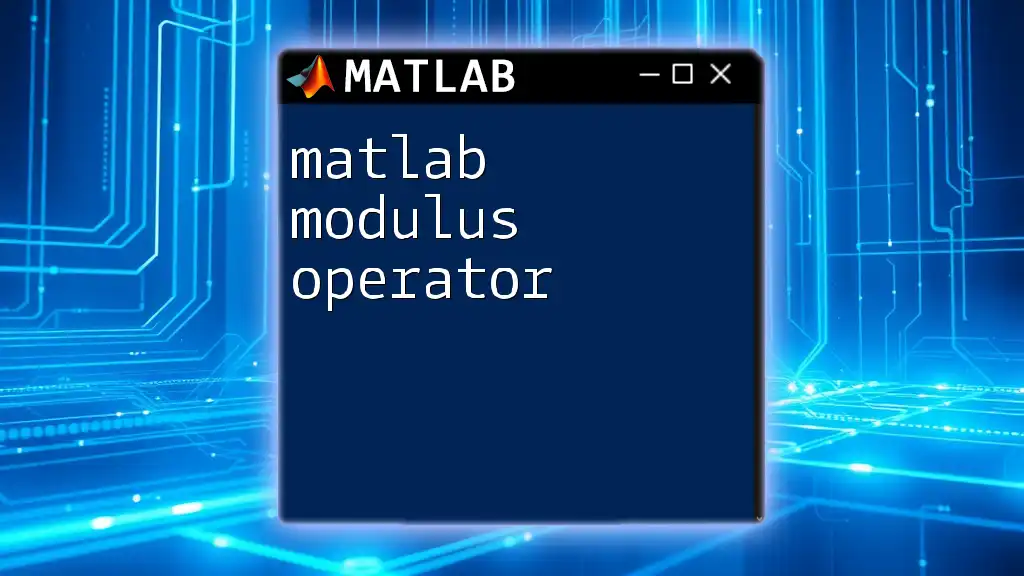
Basics of Modulus in MATLAB
Syntax of Modulus in MATLAB
The MATLAB syntax for the modulus function is straightforward:
remainder = mod(a, b)
In this syntax:
- `a` is the dividend (the number to be divided).
- `b` is the divisor (the number by which we are dividing).
Parameters of the Modulus Function
The `mod` function in MATLAB can accept both integer and floating-point values. You can also use negative numbers. It behaves differently with negative dividends and divisors, as we will explore later.
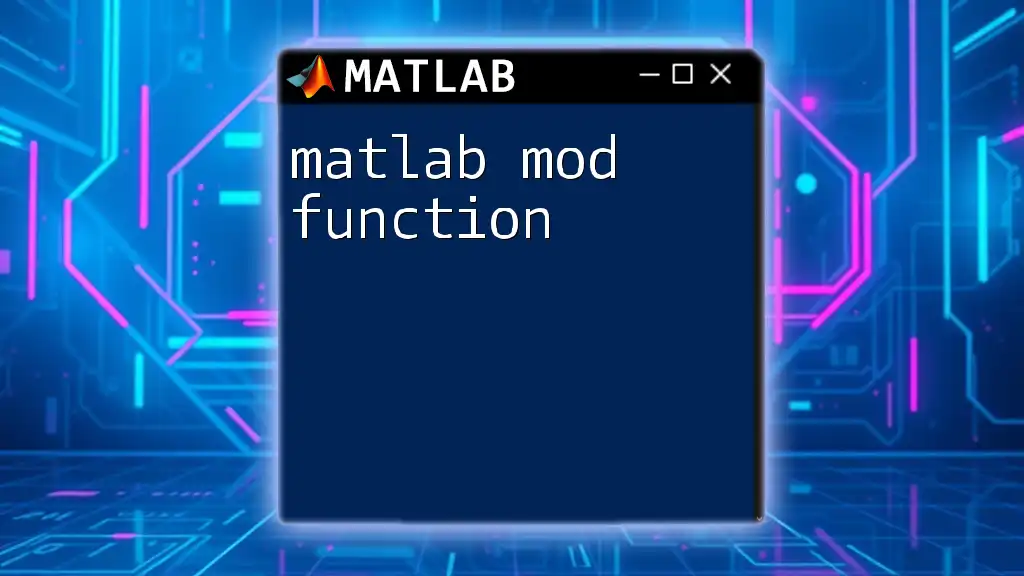
How to Use the Modulus Function in MATLAB
Simple Examples
Let’s look at some elementary examples to illustrate the modulus operation.
Example 1: Basic division and modulus
result = mod(10, 3); % Output: 1
In this example, dividing 10 by 3 yields 3 with a remainder of 1, which is precisely what the modulus function returns.
Example 2: Modulus with negative numbers
result = mod(-10, 3); % Output: 2
Here, the remainder of negative ten divided by three is two, which might seem counterintuitive at first. However, this behavior ensures that the result maintains a non-negative remainder.
Using Modulus with Arrays
The `mod` function can also be applied to arrays, allowing element-wise calculations that make processing data efficient.
Example: Element-wise modulus operation
A = [4, 5, 6];
B = [2, 3, 4];
result = mod(A, B); % Output: [0, 2, 2]
In this case, MATLAB computes the modulus for each corresponding pair of elements in arrays A and B, demonstrating the power of array operations.
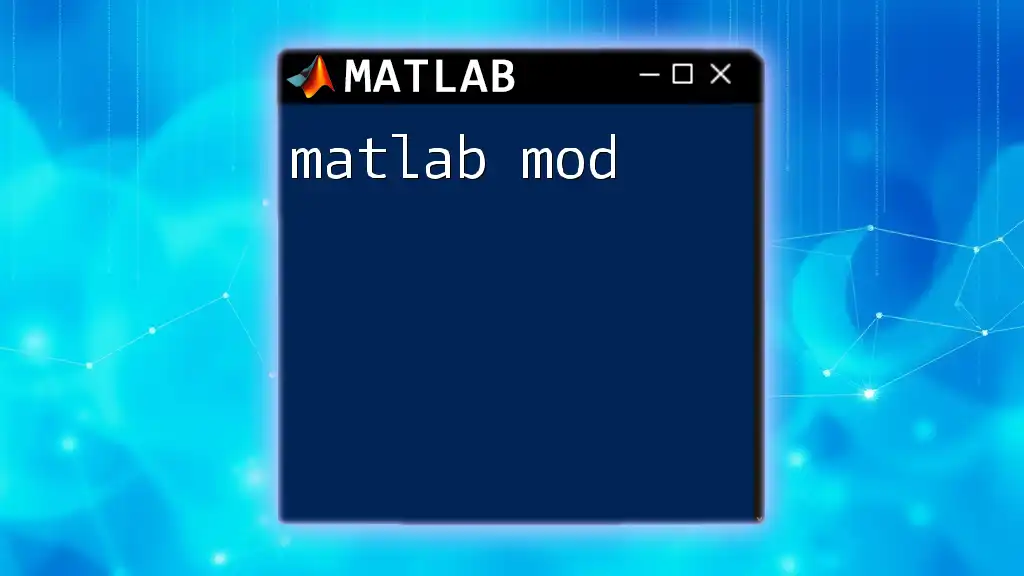
Advanced Applications of Modulus in MATLAB
Conditional Statements
The modulus function is often used in conditional statements to distinguish between even and odd numbers—an application that highlights its practical utility.
Example
if mod(x, 2) == 0
disp('Even number');
else
disp('Odd number');
end
In this snippet, if `x` is divisible by 2 with no remainder (i.e., even), the code will display "Even number"; otherwise, it will return "Odd number." This is a classic use case of the modulus operator.
Modular Arithmetic
Modular arithmetic involves performing calculations where numbers wrap around upon reaching a certain value, much like a clock.
Example of a simple implementation:
n = 12; % Modulus
a = 5;
result = mod(a, n); % Output: 5
In this example, since 5 is less than 12, the output remains 5. If `a` were, say, 15, the result would return 3 (as 15 - 12 = 3).
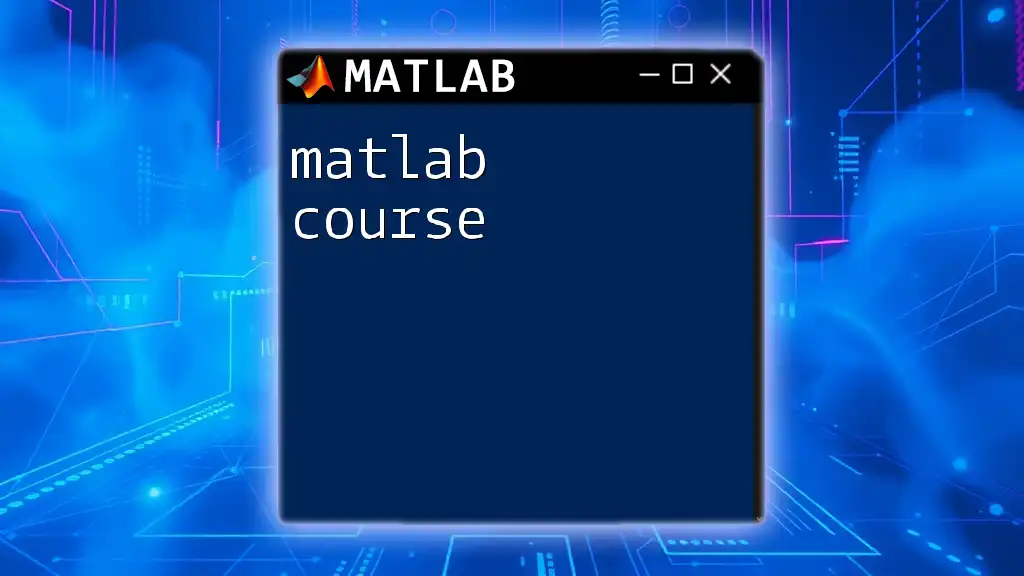
Common Mistakes When Using Modulus in MATLAB
Confusion with Division
A common error many beginners make is confusing the modulus operation with simple division. It’s crucial to understand that:
mod(5, 2) % Returns 1, the remainder
5 / 2 % Returns 2.5, the quotient
While both operations involve division, they serve very different purposes and yield different results.
Negative Values
Negative values can also lead to confusion. The behavior of the modulus function with negative inputs must be carefully understood.
result = mod(-7, 3); % Output: 2
In this example, the result still guarantees a positive remainder, reflecting the unique properties of the modulus operation.
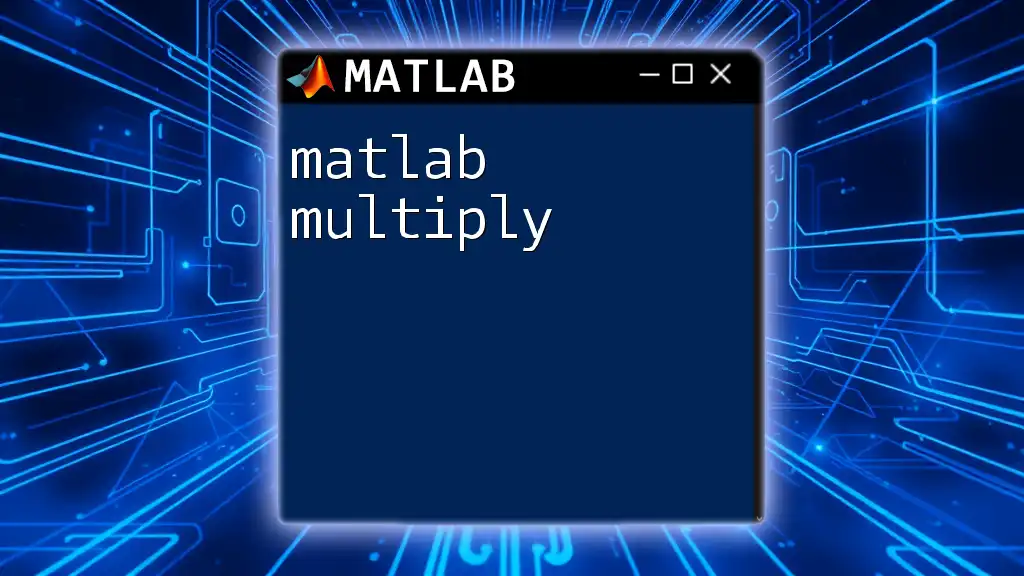
Performance Considerations
Computational Cost of Modulus
While the modulus operation is typically computationally efficient, its performance can be impacted when used excessively, such as in loops with large datasets.
When implementing algorithms that require frequent use of modulus, consider using modulo operations judiciously, particularly in high-performance applications or time-critical scenarios. Caching results and minimizing calls within loops can enhance overall performance.
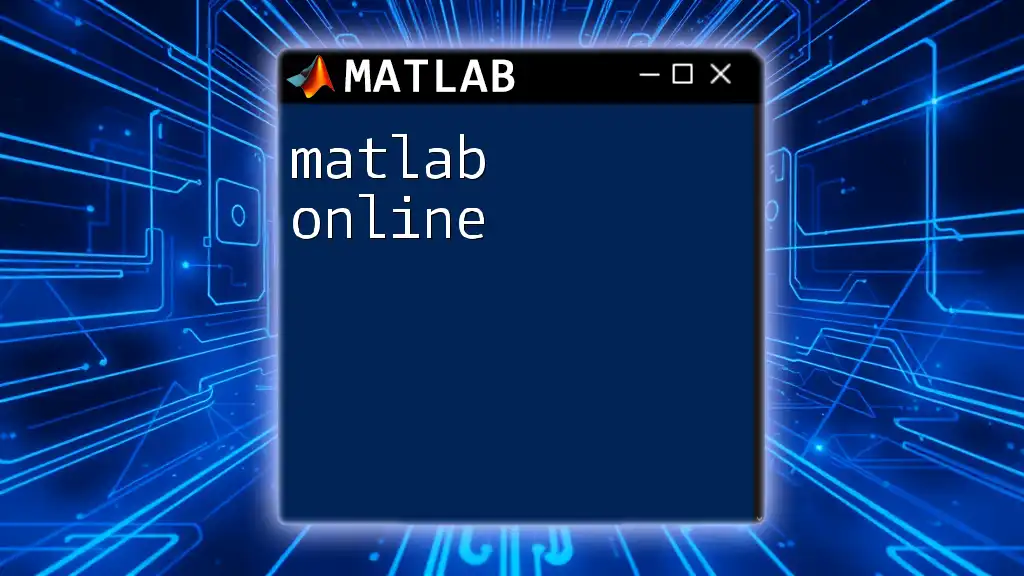
Summary and Conclusion
In summary, the MATLAB modulus function serves as a foundational tool for performing division-related calculations. From basic arithmetic to complex algorithms, understanding how to use the modulus operation can vastly improve programming efficiency and effectiveness.
As you cultivate your MATLAB skills, experimenting with the `mod` command will deepen your comprehension of numerical operations. Don't hesitate to seek out practice opportunities to solidify this essential concept!
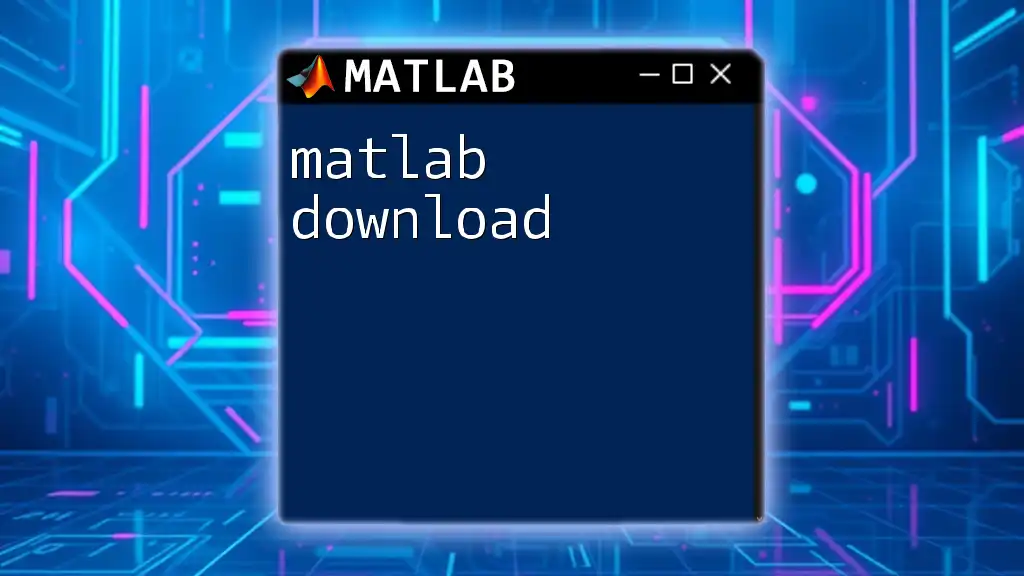
Additional Resources
Useful Links
For those looking to dive deeper into the workings of the modulus function in MATLAB, check out:
- [MATLAB Official Documentation on mod](https://www.mathworks.com/help/matlab/ref/mod.html).
Practice Problems
To enhance your understanding, consider tackling the following practice problems involving the modulus operation:
- Create a script to classify numbers from a list as even or odd using modulus.
- Implement a function that wraps numbers within a specified range (similar to a clock).
- Use modulus in calculations to find the greatest common divisor.
By mastering the MATLAB modulus, you will significantly sharpen your coding capabilities, paving the way for more advanced projects in mathematical computing. Happy coding!