In MATLAB, you can multiply matrices or arrays using the `` operator for matrix multiplication or the `.` operator for element-wise multiplication. Here's a code snippet for both types:
% Matrix multiplication
A = [1, 2; 3, 4];
B = [5; 6];
result_matrix_multiply = A * B;
% Element-wise multiplication
C = [1, 2; 3, 4];
D = [5, 6; 7, 8];
result_elementwise_multiply = C .* D;
Types of Multiplication in MATLAB
Element-wise Multiplication
Element-wise multiplication is a common operation in MATLAB where each element of one array is multiplied by the corresponding element of another array. This operation is crucial for element-wise mathematical computations, making matrix manipulations intuitive and straightforward.
Syntax and Usage
The syntax for element-wise multiplication is straightforward, using the `.*` operator.
Example Code Snippet
A = [1, 2, 3; 4, 5, 6];
B = [7, 8, 9; 10, 11, 12];
result = A .* B; % Multiplies corresponding elements
In this example, `result` will be computed as follows:
- \(1 \times 7 = 7\)
- \(2 \times 8 = 16\)
- \(3 \times 9 = 27\)
- \(4 \times 10 = 40\)
- \(5 \times 11 = 55\)
- \(6 \times 12 = 72\)
The Output Explanation shows that the resulting matrix will be:
result = [7, 16, 27;
40, 55, 72]
Matrix Multiplication
Matrix multiplication differs from element-wise multiplication as it adheres to the mathematical rules of linear algebra. For matrix multiplication to occur, the number of columns in the first matrix must equal the number of rows in the second matrix.
Syntax and Usage
Matrix multiplication is performed using the `*` operator.
Example Code Snippet
C = [1, 2; 3, 4];
D = [5, 6; 7, 8];
result = C * D; % Standard matrix multiplication
For the above example, the calculations are as follows:
- \( (1 \times 5 + 2 \times 7) = 19 \)
- \( (1 \times 6 + 2 \times 8) = 22 \)
- \( (3 \times 5 + 4 \times 7) = 43 \)
- \( (3 \times 6 + 4 \times 8) = 50 \)
The resulting matrix is:
result = [19, 22;
43, 50]
Using the `times` and `mtimes` Functions
MATLAB also provides functions for performing element-wise multiplication and matrix multiplication. The `times` function serves the same purpose as `.`, while the `mtimes` function substitutes for the `` operator.
Example Code Snippet for `times`
result = times(A, B);
Example Code Snippet for `mtimes`
result = mtimes(C, D);
Both commands effectively perform the multiplications described earlier, offering a functional approach to matrix operations.
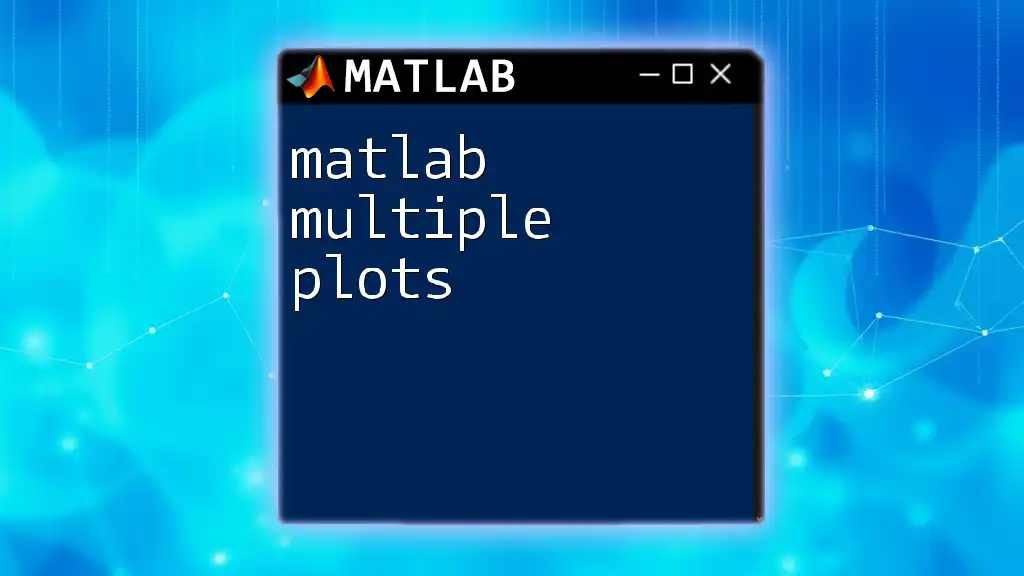
Multiplying Higher-Dimensional Arrays
Understanding N-Dimensional Arrays
MATLAB allows operations on N-dimensional arrays, expanding the potential for computations beyond just two dimensions. This capability is essential in data analysis where multidimensional data structures are common.
Example Code Snippet
E = rand(2, 3, 4); % 3-D array
F = rand(2, 3, 4);
result = E .* F; % Element-wise multiplication
In this case, the operation conducts element-wise multiplication across all three dimensions of the arrays `E` and `F`, maintaining the integrity of the results as it processes.
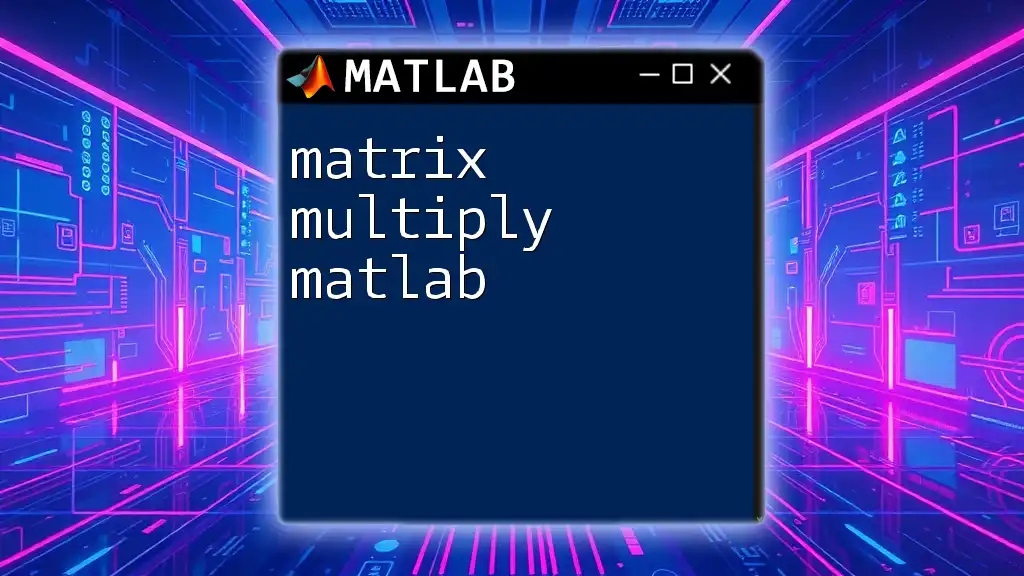
Advanced Multiplication Techniques
Batch Matrix Multiplication
MATLAB provides options for performing batch matrix multiplications efficiently. This is particularly useful for applications where multiple datasets need to be processed simultaneously.
Using `pagefun` for Batch Operations
The `pagefun` function can be instrumental when handling batch operations.
Example Code Snippet
A = rand(2, 3, 4); % 3D matrix
B = rand(3, 2, 4);
result = pagefun(@mtimes, A, B); % Batch matrix multiplication
This method will effectively perform matrix multiplication across the third dimension for each 2D slice of the arrays.
Utilizing GPU for Multiplication
To enhance computational efficiency, particularly with large datasets, MATLAB supports GPU computing, which significantly speeds up processes such as multiplication.
Example Code Snippet
A = gpuArray(rand(1000));
B = gpuArray(rand(1000));
result = A * B; % Matrix multiplication on GPU
By leveraging the graphics processing unit, calculations that traditionally require a considerable amount of time on standard CPUs can be executed much faster.
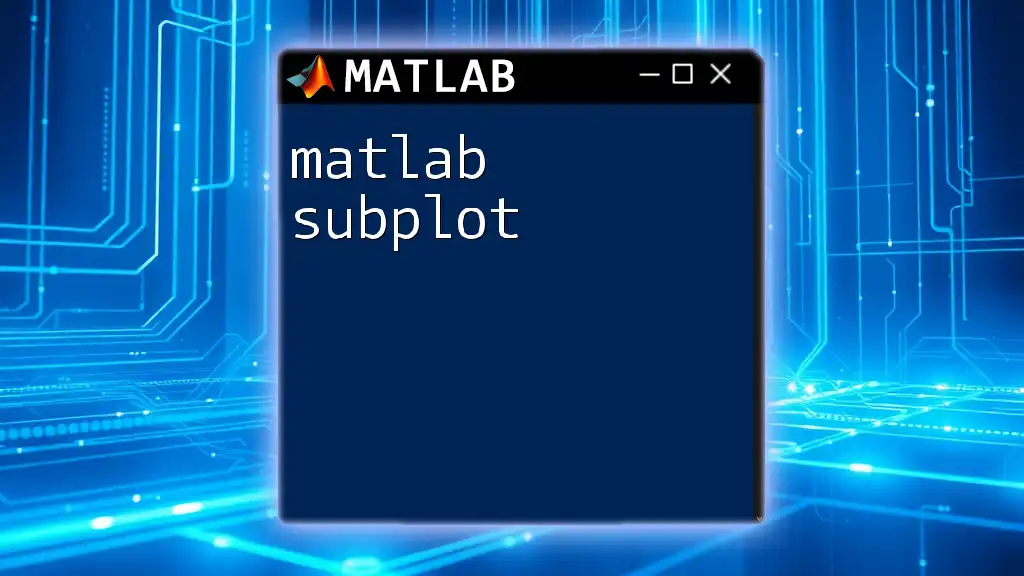
Common Errors and Troubleshooting
Dimension Mismatch
One of the most common issues encountered when performing multiplication in MATLAB is dimension mismatch. This occurs when attempting to multiply matrices that do not conform to the required dimensional rules. Often, users will encounter error messages that indicate the size of the matrices involved.
Solution Techniques
To identify and resolve this issue, always check the dimensions of the matrices using the `size()` function prior to multiplication. For example:
size(A) % Outputs dimensions of A
size(B) % Outputs dimensions of B
Type Compatibility
Another key aspect to consider is ensuring that the data types of the matrices are compatible. Mixing different types (e.g., integer and float) without proper typecasting can lead to unexpected results or errors.
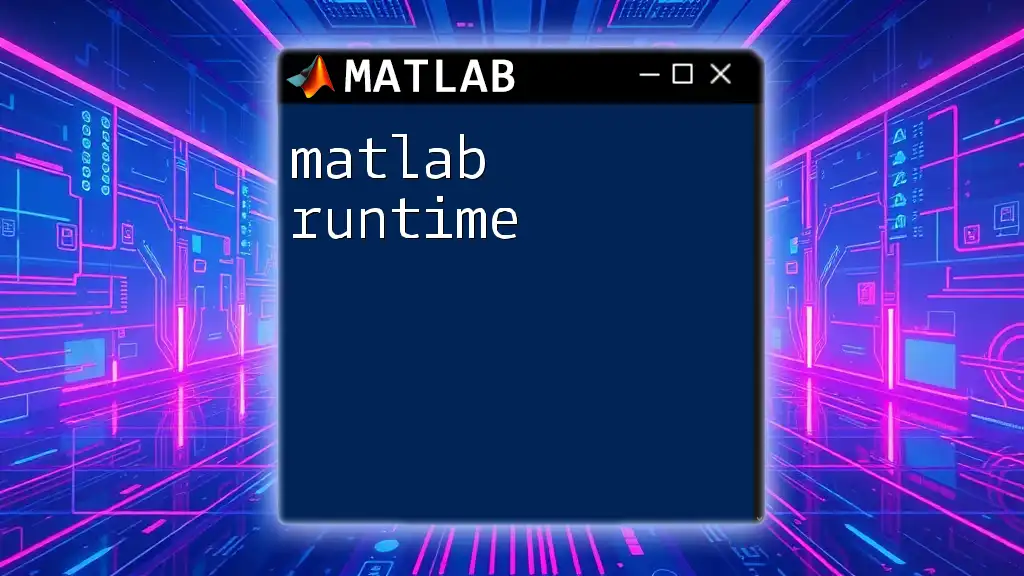
Practical Applications of Multiplication
Data Analytics and Visualization
Multiplication in MATLAB is significantly used in data analytics, where it helps in tasks such as data scaling or matrix transformations.
Example Application in Statistics
Here’s a simple application in predictive modeling:
dataMatrix = rand(100, 5);
weights = rand(5, 1);
predictedValues = dataMatrix * weights; % Producing predictions
In this context, `dataMatrix` is multiplied by `weights` to produce a vector of predicted values, demonstrating how multiplication plays a crucial role in statistical models.
Engineering and Scientific Computation
In fields like engineering and scientific computation, MATLAB’s multiplication capabilities simplify complex mathematical models. Use cases include signal processing, control systems, and simulation modeling where multiple matrices are frequently used to analyze system behaviors or define relationships.
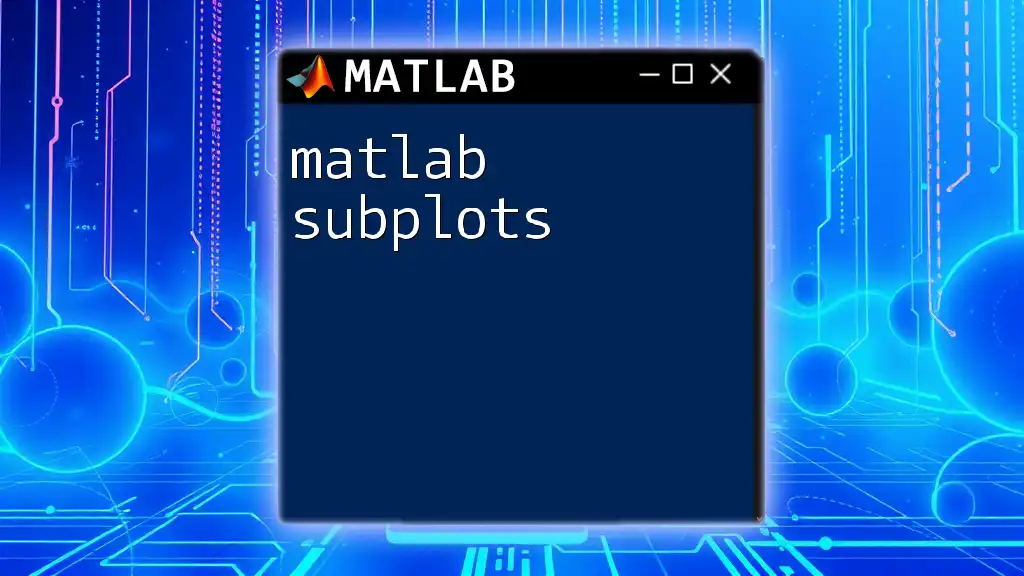
Conclusion
This comprehensive guide has detailed the various multiplication techniques available in MATLAB, providing clarity on when and how to use each method effectively. Whether you need to perform basic element-wise operations or complex batch matrix multiplications, understanding how to matlab multiply will significantly enhance your proficiency in MATLAB. It's encouraged to experiment with these techniques to become adept at utilizing MATLAB's powerful computational capabilities.
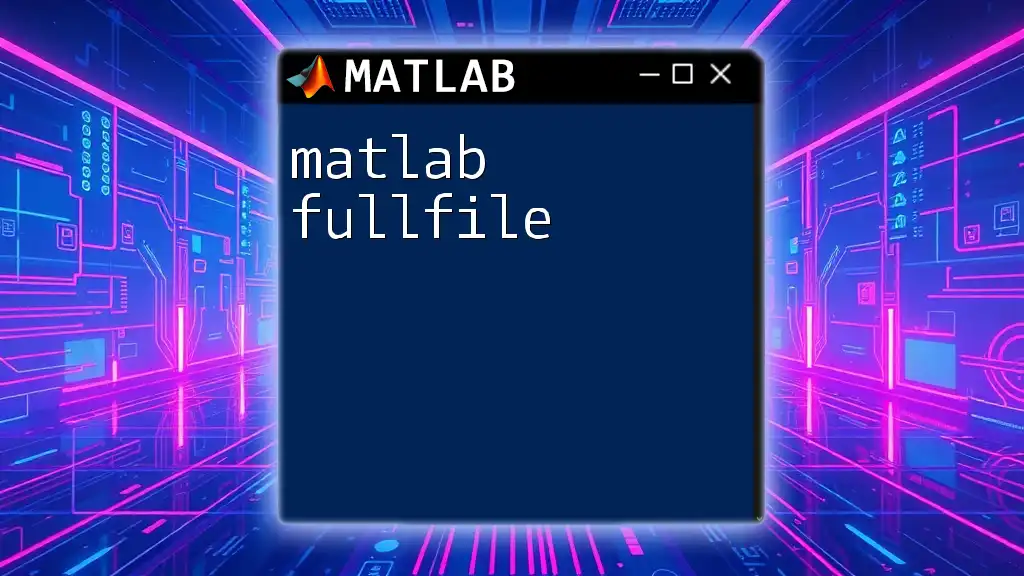
Additional Resources
For further expertise, consider exploring the official MATLAB documentation, which provides extensive examples and detailed explanations. Additionally, several books and online courses are available to broaden your knowledge in using MATLAB effectively for various applications.