In MATLAB, the `polyfit` function is used to perform polynomial curve fitting by determining the coefficients of a polynomial that best fits a set of data points.
Here’s a simple code snippet demonstrating its use:
% Example data
x = [1, 2, 3, 4, 5];
y = [2.2, 2.8, 3.6, 4.5, 5.1];
% Fit a polynomial of degree 1 (linear)
p = polyfit(x, y, 1);
% Display the polynomial coefficients
disp(p);
Understanding Polynomial Fitting
Definition of Polynomial Fitting
Polynomial fitting is a mathematical technique used to model relationships within a set of data points through polynomial equations. By fitting a polynomial to the data, you can provide a clear representation of trends and patterns, making it easier to analyze or predict future values. This method is significant in statistical analysis and data modeling, primarily when you're dealing with non-linear relationships that a simple linear model cannot adequately capture.
Applications of Polynomial Fitting
Polynomial fitting finds numerous applications across various fields. For instance, in engineering, it can model the stress-strain relationship of materials; in economics, it can predict trends in stock prices; and in environmental science, it can help understand changes in pollution levels over time. The flexibility of polynomial fitting is crucial in scenarios where more complex relationships are needed to explain the phenomena being studied.
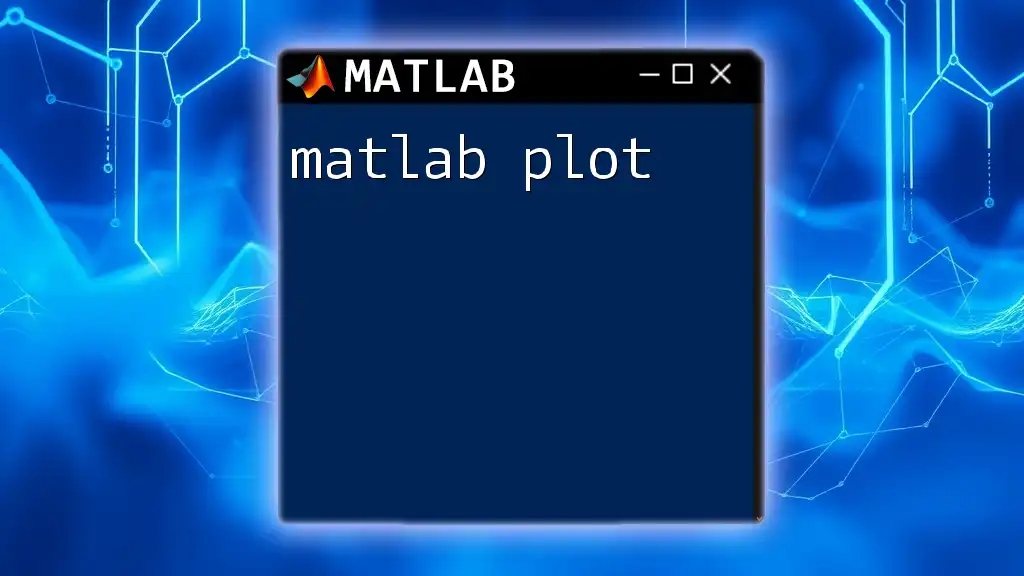
Getting Started with MATLAB
Setting up MATLAB
To begin using the `matlab polyfit` function, you need to have MATLAB installed on your computer. If you’re new to MATLAB, official documentation provides a user-friendly guide for installation. Familiarity with the MATLAB interface and basic commands is beneficial for efficiently navigating the software.
Basic Syntax of Polyfit
The essential syntax for the `polyfit` function is as follows:
p = polyfit(x, y, n)
Where:
- `x` is the vector of independent variable data.
- `y` is the vector of dependent variable data.
- `n` represents the degree of the polynomial you are fitting.
This simple line of code allows you to model your data effectively, aiding in analysis and forecasting.
Example of a Simple Polynomial Fit
Here’s a straightforward example of how to fit a polynomial to a set of sample data:
% Sample data
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
% Fitting a polynomial of degree 1 (linear fit)
p = polyfit(x, y, 1);
% Display the coefficients
disp(p);
In this case, `p` will store the coefficients of the fitted polynomial, which, for a linear fit, will provide you with the slope and intercept of the best-fitting line.
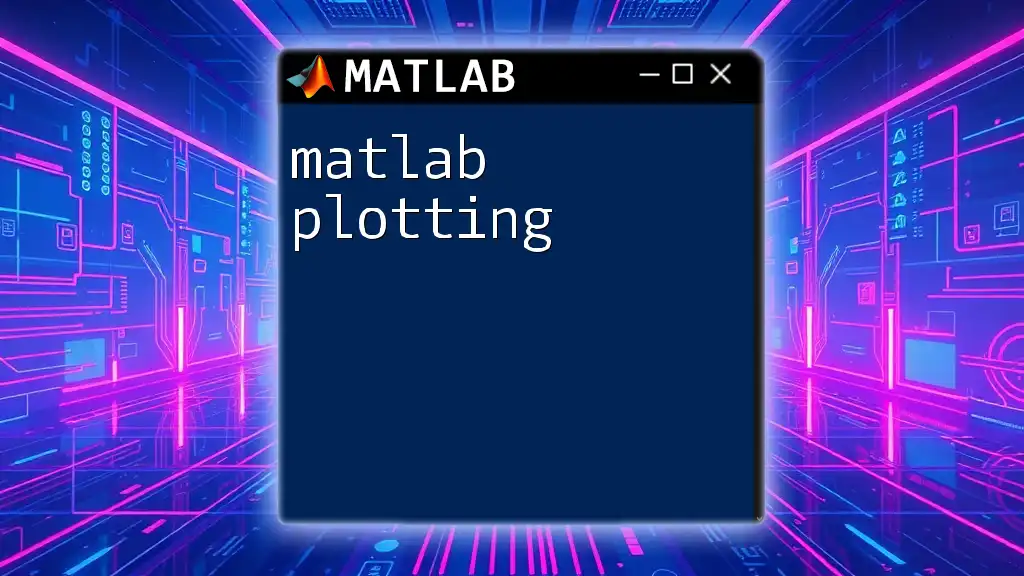
In-Depth Look at Polyfit Parameters
Overview of Input Parameters
Understanding the role of each input parameter is crucial:
- `x` (Independent variable): This array contains your input data values.
- `y` (Dependent variable): This array holds the output data values that correspond to each `x`.
- `n` (Degree of polynomial): This integer specifies the desired degree of the polynomial. Higher numbers allow for fitting more complex curves, while lower numbers result in simpler models.
Choosing the Right Degree
Selecting the appropriate degree for the polynomial is essential for obtaining a meaningful fit. A polynomial that is too low (underfitting) may not capture the underlying trend, while a polynomial that is too high (overfitting) can model noise rather than the signal in the data. Incorporating techniques such as cross-validation can help you determine the most suitable degree for your polynomial.
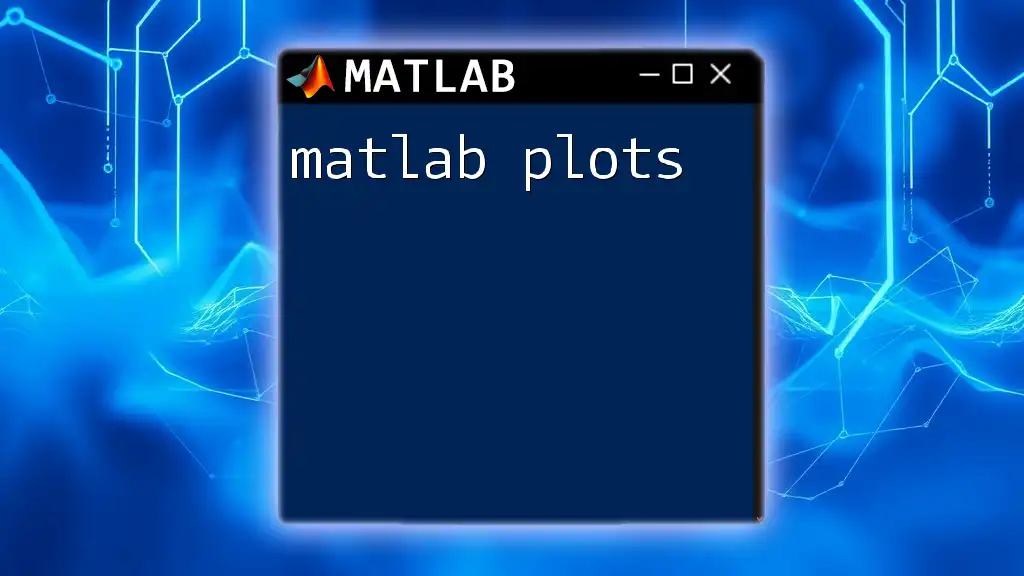
Visualizing the Fitted Polynomial
Creating a Fit Plot
Visualizing the polynomial fit is crucial for interpreting and understanding your results. An effective way to plot it involves generating points for the fitted polynomial and overlaying them on your original data:
% Generate points for plotting the fitted polynomial
xx = linspace(min(x), max(x), 100);
yy = polyval(p, xx); % Evaluate polynomial at new points
% Plot
figure;
plot(x, y, 'o', 'MarkerSize', 10); % Original data points
hold on;
plot(xx, yy, '-r', 'LineWidth', 2); % Fitted polynomial line
xlabel('X-axis label');
ylabel('Y-axis label');
title('Polynomial Fit Visualization');
legend('Data Points', 'Fitted Polynomial');
hold off;
In this code, `polyval` is used to evaluate the polynomial at new values of `x`. This visualization allows you to assess how well the polynomial fits your original data.
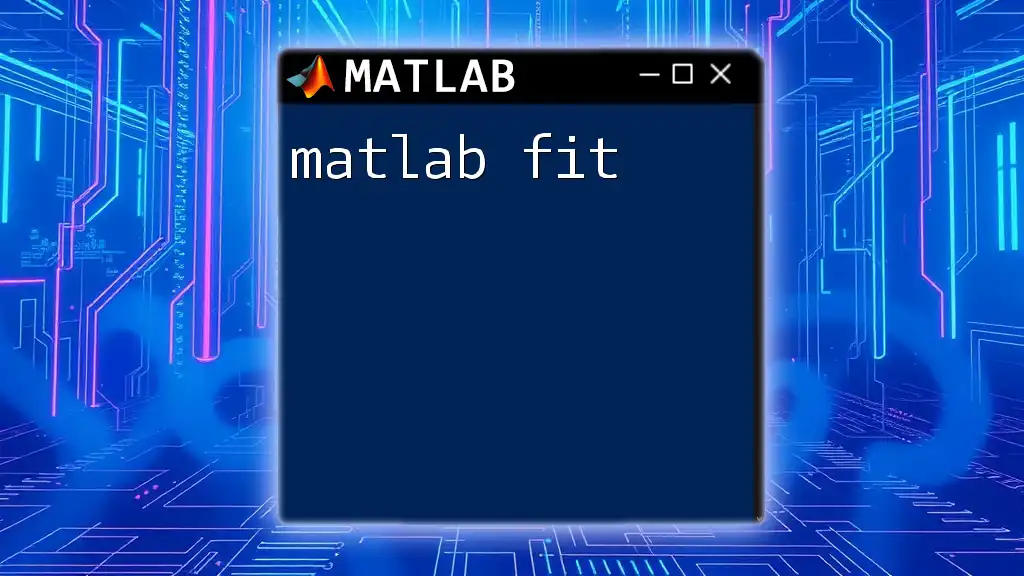
Evaluating the Fit
Residual Analysis
Evaluating your polynomial fit includes analyzing the residuals—differences between the observed and predicted values. The following code calculates and visualizes the residuals:
residuals = y - polyval(p, x);
figure;
plot(x, residuals, 'o', 'MarkerSize', 10);
xlabel('X-axis label');
ylabel('Residuals');
title('Residuals of the Fit');
Assessing residuals helps determine whether the polynomial is adequately capturing the data trends. Ideally, residuals should appear random and scattered around zero.
Goodness of Fit Metrics
To gauge how well your model fits the data, you may calculate metrics such as R-squared or the Root Mean Square Error (RMSE). The R-squared value provides insight into the proportion of variance in the dependent variable predictable from the independent variable:
% Calculate R-squared
y_fit = polyval(p, x);
SS_res = sum((y - y_fit).^2);
SS_tot = sum((y - mean(y)).^2);
R_squared = 1 - (SS_res / SS_tot);
disp(R_squared);
A high R-squared value signifies a better fit, while a low value may indicate that a polynomial of this degree is insufficient for your dataset.
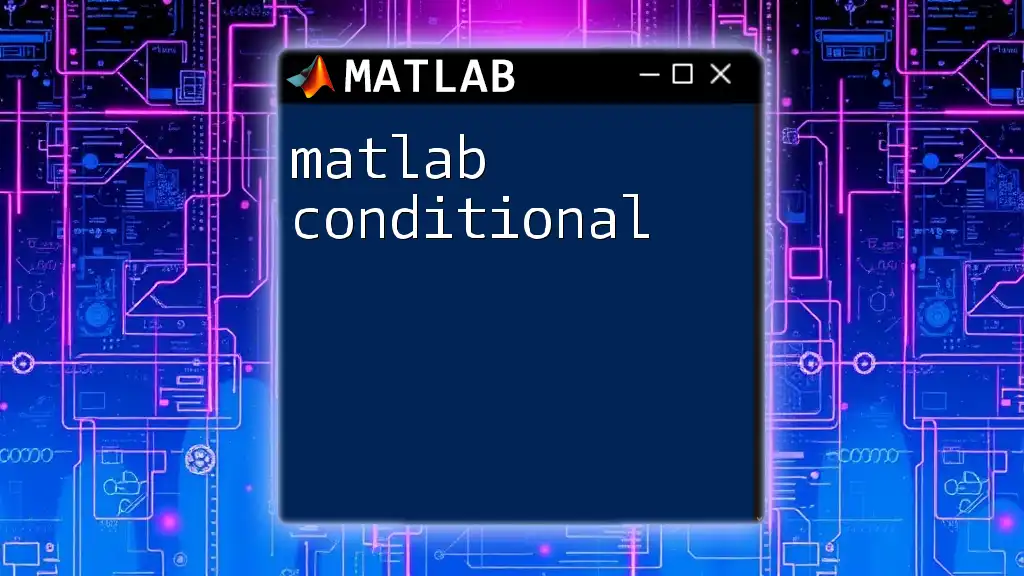
Advanced Usage of Polyfit
Handling Multiple Variables
While polyfit primarily accommodates single-variable polynomial fitting, with clever manipulation, you can extend it to multivariable fits. This typically requires using matrix methods and considering interaction terms to capture relationships accurately in more complex data.
Customizing Polynomial Coefficients
In certain scenarios, you may wish to manually manipulate polynomial coefficients for specific applications or exploratory analyses. Familiarity with MATLAB's parameter handling can allow for creative adjustments tailored to unique datasets.
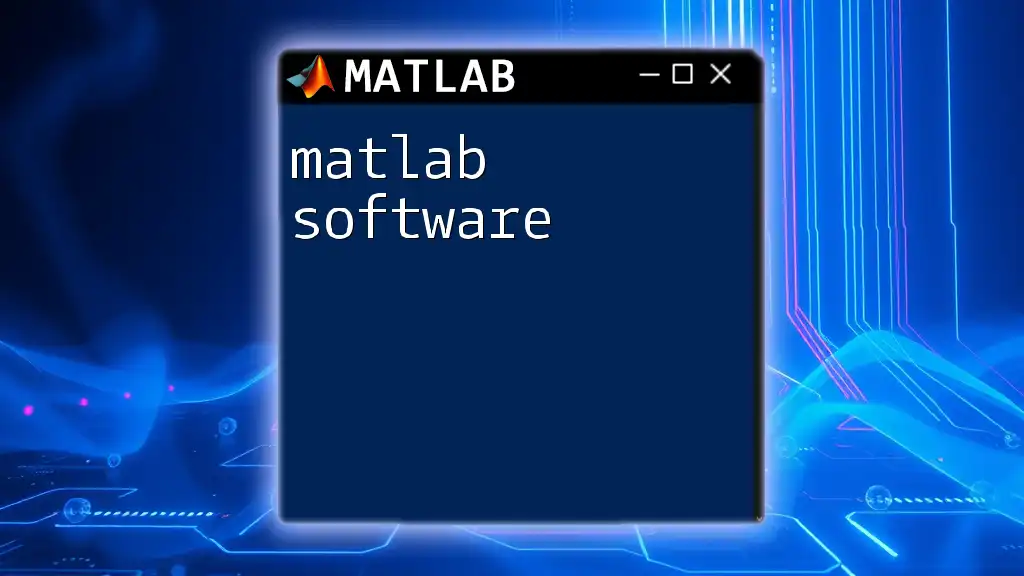
Common Pitfalls and Troubleshooting
Common Errors and How to Resolve Them
As you work with `matlab polyfit`, you might encounter errors related to mismatched dimensions between `x` and `y` or inappropriate polynomial degrees. Ensuring your data is correctly formatted and thoroughly checking values before running `polyfit` can help mitigate these issues.
Best Practices for Using Polyfit
To maximize the efficacy of polynomial fitting, consider normalizing your data before applying polyfit. This practice can help reduce numerical errors and improve fitting accuracy, particularly when dealing with large datasets or extreme values.
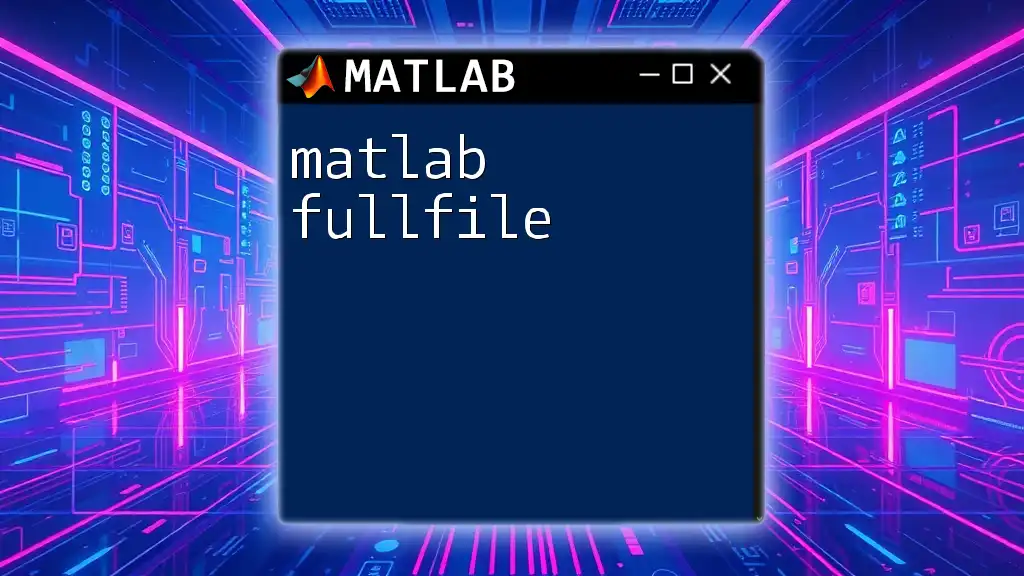
Conclusion
In this comprehensive guide, you've learned how to efficiently use the `matlab polyfit` function for polynomial fitting. By understanding essential concepts, mastering the syntax, visualizing results, and evaluating fit quality, you can effectively model complex relationships in your data. Exploring these techniques will not only enhance your analytical capabilities but also empower you to tackle real-world problems with confidence.
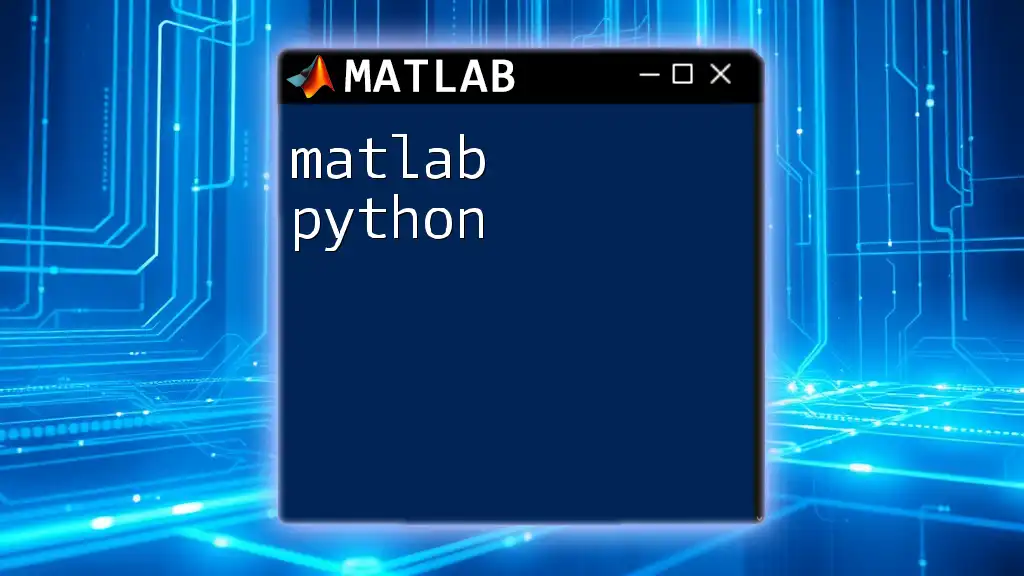
Additional Resources
For further exploration, consider visiting the official MATLAB documentation and tutorials, or look into recommended books and courses to deepen your understanding of MATLAB and polynomial fitting techniques.