The MATLAB modulus operator `%` returns the remainder after the division of two numbers.
Here's a code snippet demonstrating its usage:
result = mod(10, 3); % This will return 1, since 10 divided by 3 leaves a remainder of 1
Understanding the Matlab Modulus Operator
What is the Modulus Operator?
The matlab modulus operator is a mathematical function used to determine the remainder of a division between two numbers. In essence, it helps to find out how much is left over after dividing one number by another.
Importance of the Modulus Operator
The matlab modulus operator has significant applications in various fields. For instance, it can be used in:
- Mathematics to solve problems involving divisibility.
- Computer Science to create algorithms that require cyclic calculations.
- Engineering for tasks like signal processing where periodic functions are analyzed.
Understanding how to use the matlab modulus operator is essential for efficient programming in any of these fields.
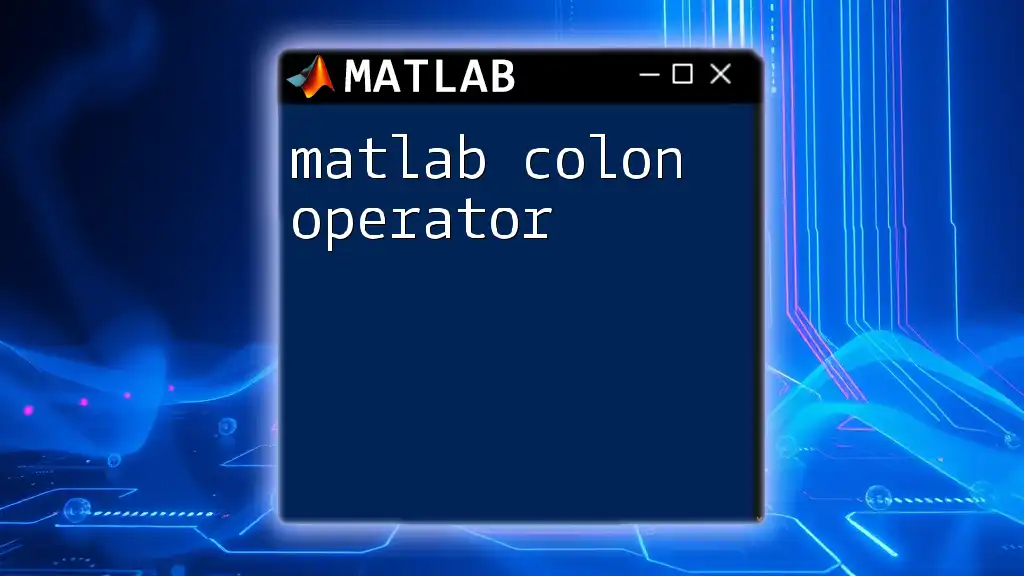
The Modulus Operator in Matlab
Syntax and Basic Usage
In Matlab, the modulus operator is expressed using the `mod` function. The typical syntax looks like this:
result = mod(a, b);
Here, `a` is the dividend, and `b` is the divisor. The `mod` function calculates the remainder of `a` when divided by `b`.
Example of Modulus Operator
To illustrate the basic functionality of the matlab modulus operator, consider the following example:
a = 10;
b = 3;
modResult = mod(a, b);
disp(modResult); % Output: 1
In this scenario, 10 divided by 3 leaves a remainder of 1, which is the output from the `mod` function. This simple example encapsulates the operator's core functionality.
Alternative Methods for Modulus in Matlab
While the `mod` function is widely used, Matlab also provides the `rem` function for calculating remainders. The difference between `mod` and `rem` surfaces in how negative numbers are treated. For example:
a = -10;
b = 3;
remResult = rem(a, b);
disp(remResult); % Output: -1
Here, `rem` gives a different output (-1) than `mod`, which would return 2 in this case. The choice between these functions depends on the specific requirements of your calculation.
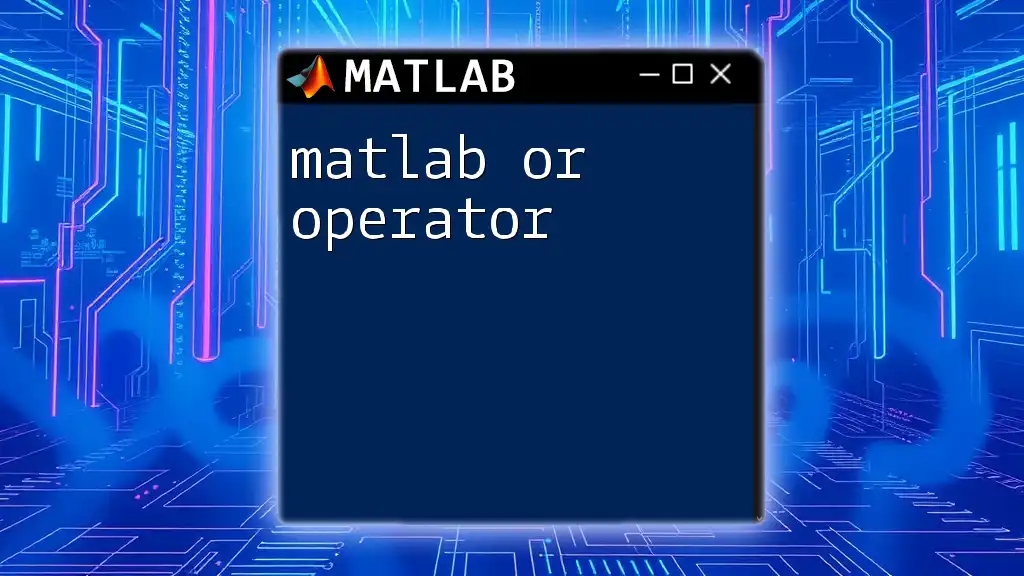
Advanced Usage of the Modulus Operator
Using Modulus with Vectors and Matrices
The matlab modulus operator can also be applied to vectors and matrices, allowing for element-wise calculations. For example:
A = [10, 15, 20];
B = 6;
result = mod(A, B);
disp(result); % Output: [4, 3, 2]
In this example, Matlab applies the modulus operation to every element in vector `A` with respect to `B`, producing a new array containing the remainders.
Complex Numerical Operations
The modulus operator becomes even more powerful when integrated into complex calculations. Consider the following expression:
a = 5;
b = 2;
c = 3;
complexOperation = mod(a + b * c, 4);
disp(complexOperation); % Output: 2
Here, the matlab modulus operator plays a crucial role in determining the outcome of more complex expressions. The operator ensures that the final result is cyclical, which can be particularly useful in many programming contexts.
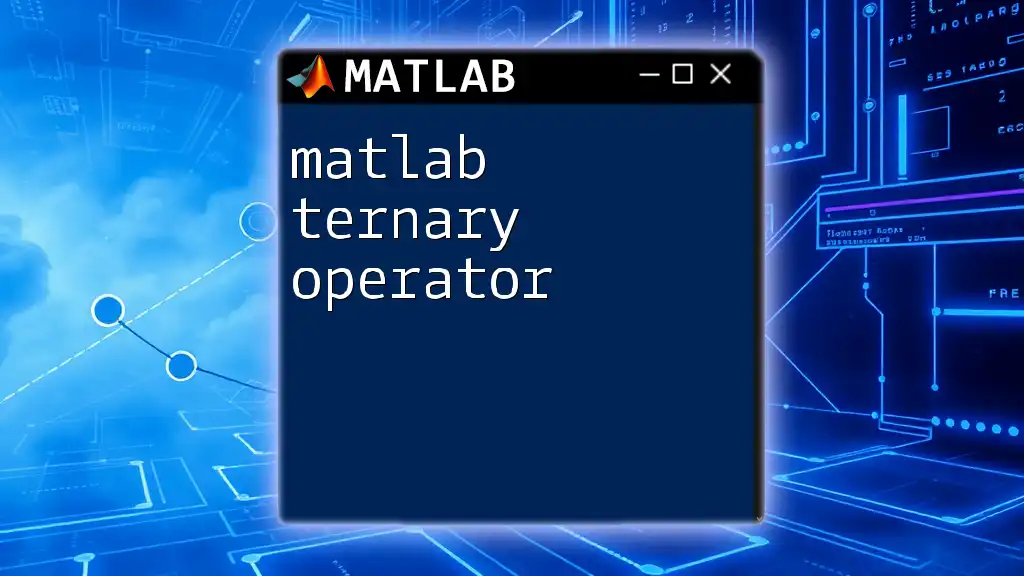
Common Mistakes and Troubleshooting
Identifying Errors in Modulus Calculations
One common mistake when using the matlab modulus operator is attempting to apply it to non-integer values. For instance, if division involves floating-point numbers, the results may yield unexpected outcomes.
Solutions to Common Issues
To avoid errors, ensure that the inputs to the `mod` function are appropriate. Always validate that your variables are integers if you expect integer results. Using functions like `floor` or `ceil` can help:
a = 10.5;
b = 3;
roundedResult = mod(floor(a), b);
disp(roundedResult); % Output: 1
This approach ensures that you work with integer values, leading to more predictable outcomes.
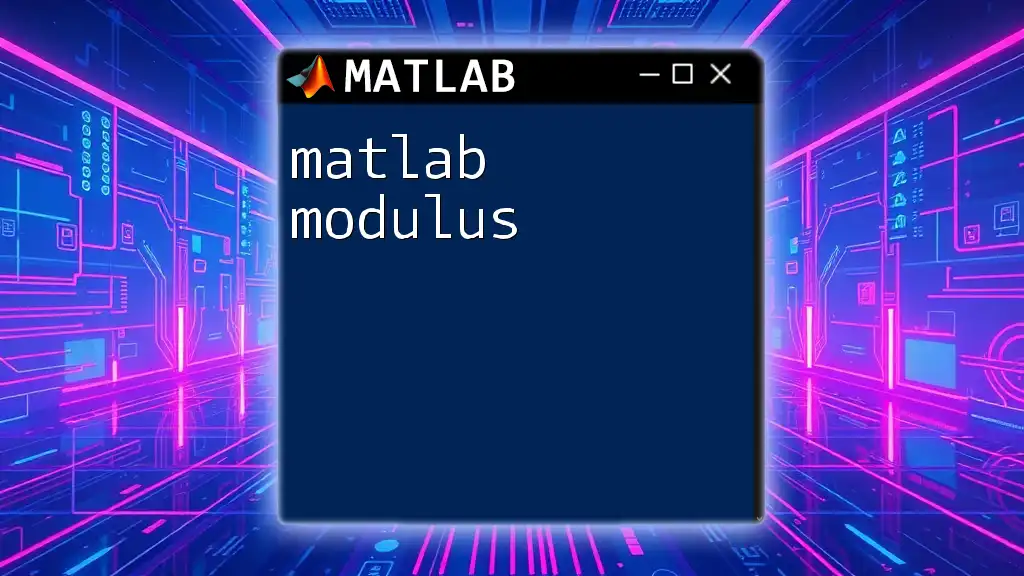
Practical Applications of the Modulus Operator
Creating Even and Odd Number Functions
The matlab modulus operator can be instrumental in creating functions to determine the parity of numbers. The following function checks whether a number is even or odd:
function isEven = checkEven(number)
isEven = mod(number, 2) == 0;
end
This simple function returns true if the number is even and false if it is odd. The use of the modulus operator here facilitates a quick assessment of number properties.
Cyclic Data Structures
The matlab modulus operator also proves useful in scenarios involving cyclic data structures. For example, when you want to cycle through an array, you can use:
array = [1, 2, 3];
index = 5;
cyclicValue = array(mod(index, length(array)) + 1);
disp(cyclicValue); % Output: 2
In this code snippet, the modulus operator helps wrap the index around when it exceeds the length of the array, providing the correct value in a cyclic manner.
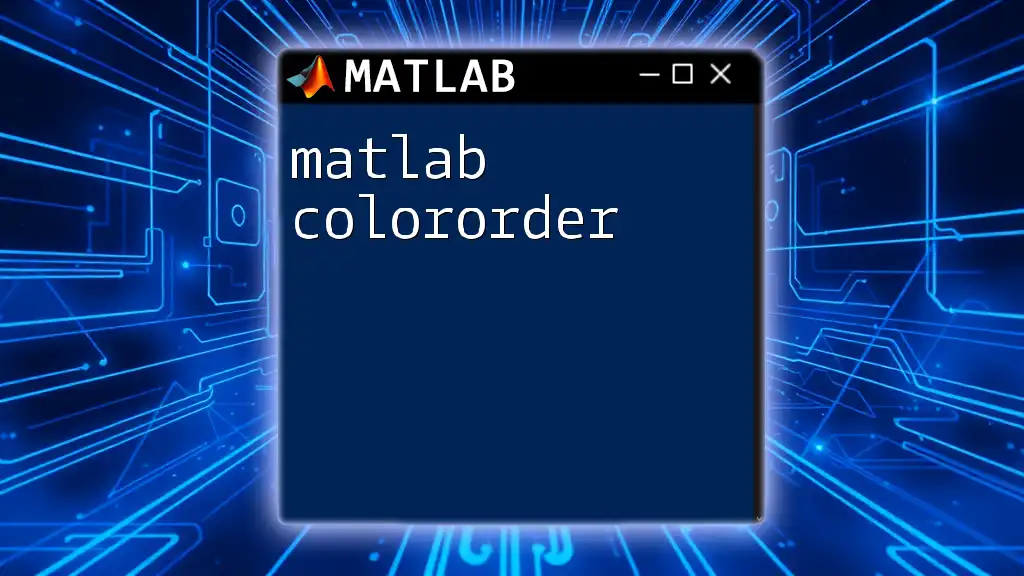
Conclusion
Summary of Key Points
In summary, the matlab modulus operator is a versatile tool essential for performing division-related calculations, determining remainders and enabling efficient programming techniques. Understanding its syntax and applications allows one to enhance coding skills in Matlab significantly.
Final Thoughts
Experimenting with the matlab modulus operator opens doors to numerous opportunities in both academic and professional environments. Whether you're working on mathematical problems, developing algorithms, or analyzing data, mastering this operator will undoubtedly prove beneficial.
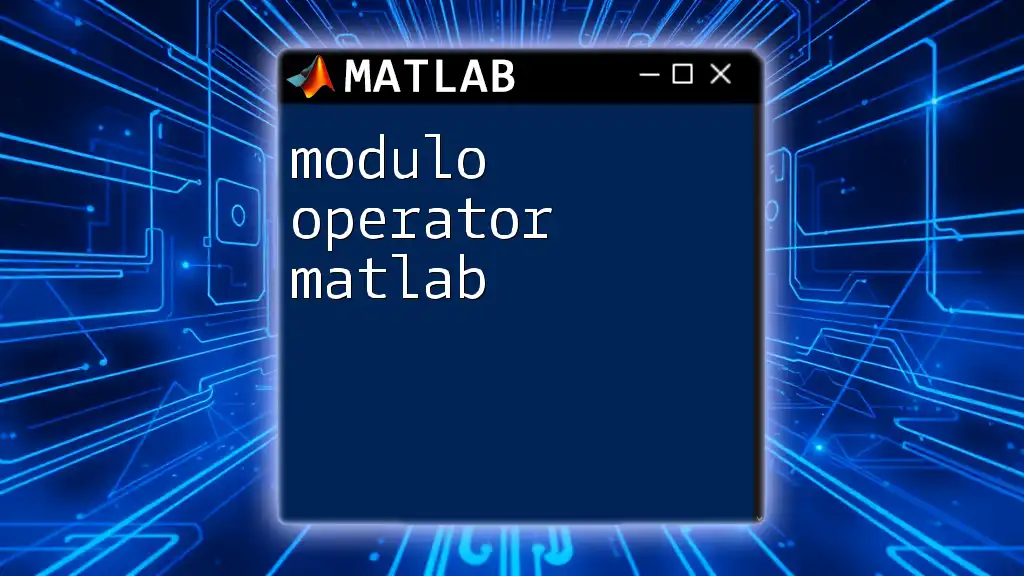
Additional Resources
For those interested in delving deeper, consider referring to the official Matlab documentation or seeking out tutorials that provide further examples and practical exercises to solidify your understanding of the matlab modulus operator.