The `VideoWriter` function in MATLAB allows you to create and save video files from image sequences or plots, enabling easy video generation for visual data representation.
Here’s a simple code snippet demonstrating how to use the `VideoWriter` function:
% Create a VideoWriter object
v = VideoWriter('myVideo.avi');
open(v);
% Create a sample plot and write frames to the video
for k = 1:10
plot(rand(10,1));
frame = getframe(gcf);
writeVideo(v, frame);
end
% Close the video file
close(v);
What is VideoWriter?
The VideoWriter function in MATLAB is a powerful tool that allows you to create video files programmatically. It serves as a bridge between your data visualizations or simulations and the ability to present them in a more engaging format. Utilizing matlab videowriter can significantly enhance your presentations and improve the communication of complex information.
Use Cases of VideoWriter
VideoWriter is versatile and finds applications in several fields, including but not limited to:
- Simulations: Capturing execution of algorithms in a real-time context.
- Animations: Creating visual representations of mathematical concepts and phenomena.
- Data Visualization: Presenting dynamic data in a way that is easier for audiences to understand.
Creating videos of your visualizations can make your findings more accessible and engaging, fostering better understanding among your audience.
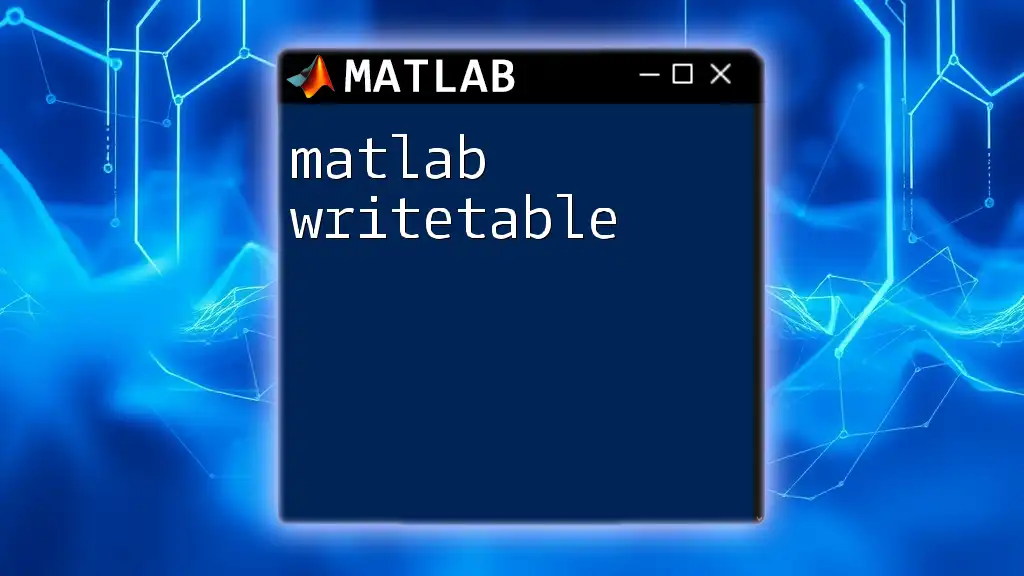
Getting Started with VideoWriter
Prerequisites
Before diving into matlab videowriter, familiarity with basic MATLAB commands is required. Understanding video formats, such as AVI and MP4, will also help in selecting the appropriate settings for your videos.
Creating a VideoWriter Object
To start using VideoWriter, you first need to create a VideoWriter object. The syntax is straightforward:
writerObj = VideoWriter('filename', 'FileFormat');
For example, to create a VideoWriter object that will save to an AVI file, the code would look like this:
writerObj = VideoWriter('myVideo', 'Uncompressed AVI');
This command initializes a video file named `myVideo.avi`, and you can specify various formats based on your needs, such as 'MPEG-4' for a more compressed option.
Setting Video Properties
The VideoWriter object allows you to customize several properties to optimize the quality and performance of your videos.
Frame Rate
The frame rate is crucial for determining how smooth your video playback will be. Setting an appropriate frame rate is essential for different applications. For example, you may want to set it as follows:
writerObj.FrameRate = 30; % Set frame rate to 30 FPS
A standard frame rate of 30 frames per second is often sufficient for most applications.
Quality and Compression
Another important property is the quality of the video. You can control the quality using the `Quality` property. Here’s how to set it:
writerObj.Quality = 75; % Set quality level between 0 to 100
Choosing the right quality can impact the file size and visual clarity, so it is beneficial to fine-tune this setting.
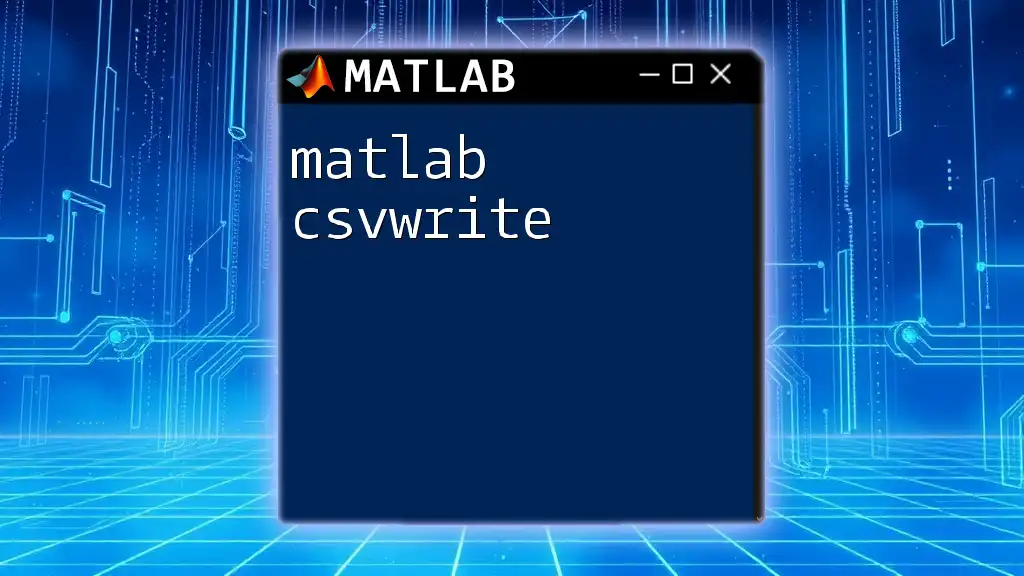
Writing Video Frames
Opening the Video Writer
Before you can write frames to your video, you need to open the video writer object:
open(writerObj);
This command prepares the object for writing frames and ensures that the final output is correctly saved.
Adding Frames to Your Video
Using `writeVideo` Function
The `writeVideo` function is the core method for adding frames to your video. Each frame must be structured correctly (i.e., a 3D matrix with dimensions [height, width, color channels]). A simple example of how to add a single frame looks like this:
writeVideo(writerObj, frame);
Generating Frames in a Loop
In scenarios where you are generating multiple frames, often inside a loop, you might do the following:
for k = 1:numFrames
frame = rand(480,640,3); % Random frame generation for demonstration
writeVideo(writerObj, frame);
end
This example generates `numFrames` of random RGB images, illustrating how you can dynamically create the content of your video.
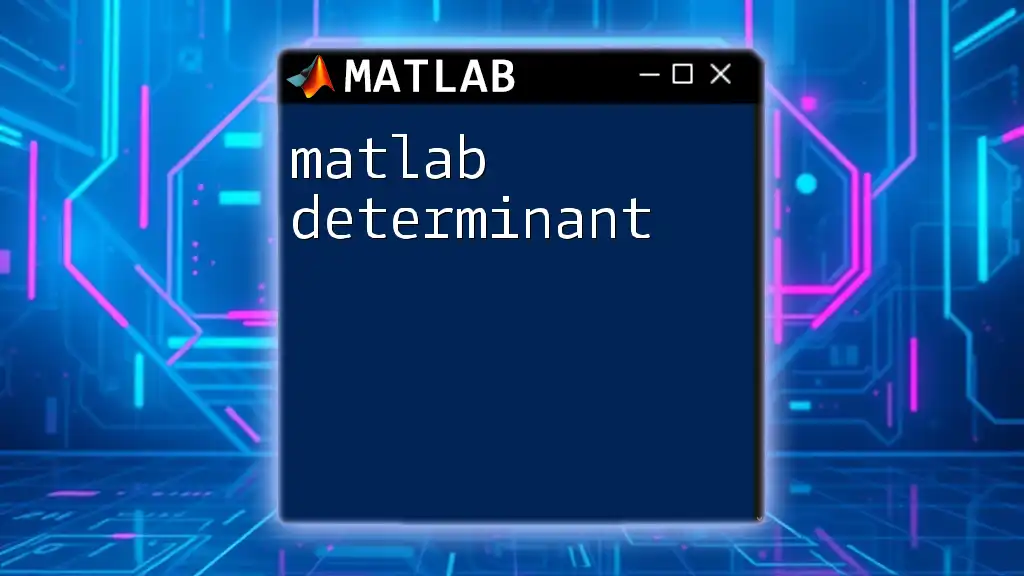
Closing the Video Writer
Finalizing the Video
After you are done adding your frames, it’s essential to close the video writer to ensure that everything is saved properly:
close(writerObj);
Failing to close the object may lead to incomplete or corrupted video files.
Confirming Video Creation
Once you have closed the writer, you can navigate to the location where you saved your video file and play it to confirm that it has been created correctly.
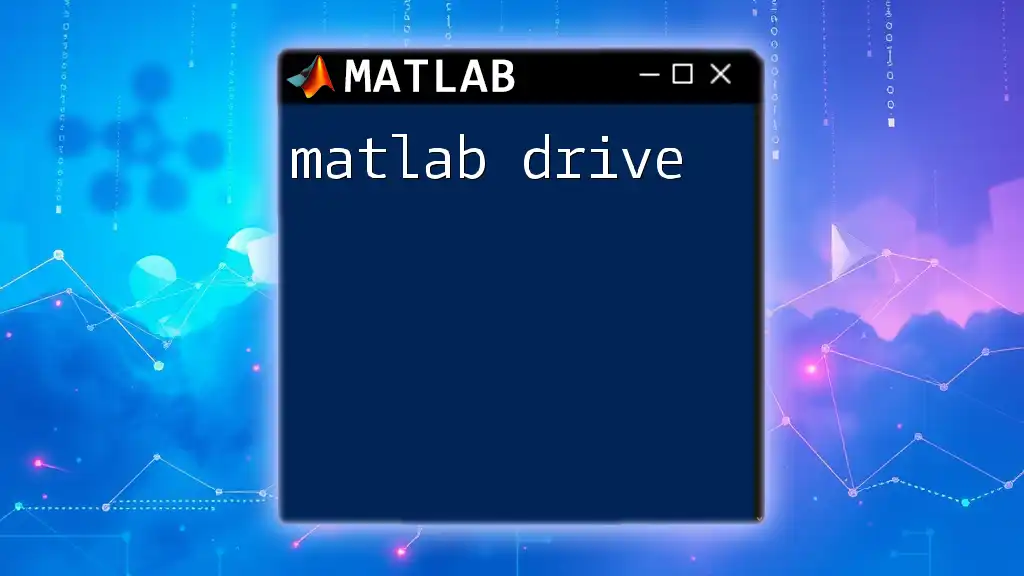
Advanced Features of VideoWriter
Adding Annotations and Overlays
To enhance the viewer's understanding, you might want to add text or overlays to your video frames. Here’s how you can annotate a frame:
position = [10, 10]; % Position of the text
frame = insertText(frame, position, 'Hello World', 'FontSize', 18);
writeVideo(writerObj, frame);
These annotations can help you to label important points in your visualizations as you present them.
Creating a Video from an Existing Object
If you have sets of images or data visualizations saved as files, you can also generate a video from those assets. For example:
for k = 1:length(images)
img = imread(images(k)); % Read each image
writeVideo(writerObj, img);
end
This loop reads a sequence of images and writes each as a frame in the video, allowing you to create dynamic presentations from existing media.
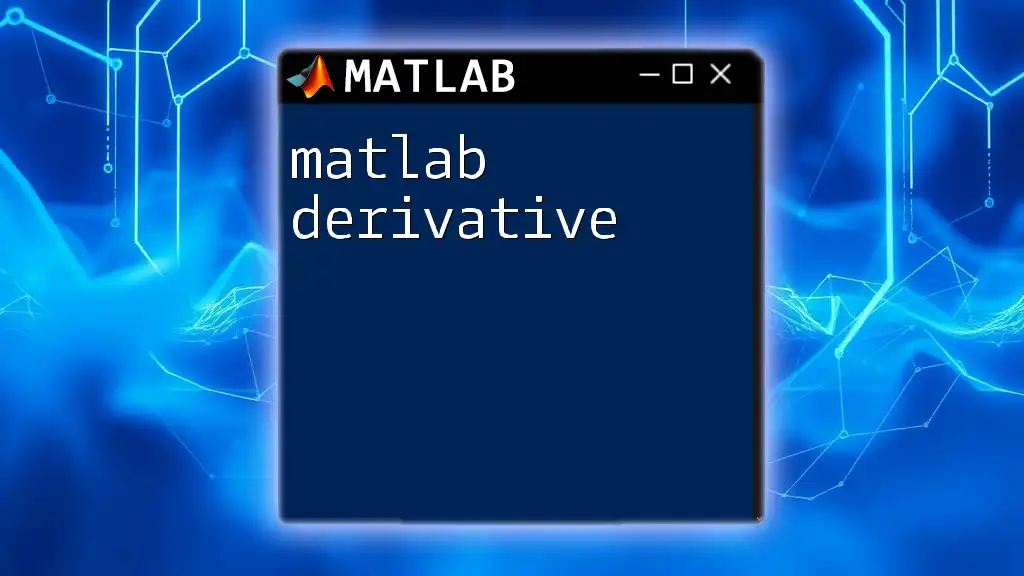
Best Practices for Using VideoWriter
Choosing the Right Format and Resolution
Selecting matlab videowriter formats and resolutions should align with your goals. If your primary audience will view your videos online, consider using formats like MP4, as they offer a good balance between quality and file size.
Frame Rate Considerations
While determining a good frame rate, keep in mind that higher rates are generally better for smoother video but may increase your file size and rendering time. For presentations involving simple static images, a lower frame rate could suffice.
Optimizing Video for Web Use
When preparing videos for online sharing, setting compression options to reduce file size without sacrificing quality is beneficial. Use the `Quality` and `FrameRate` settings wisely to balance between performance and clarity.
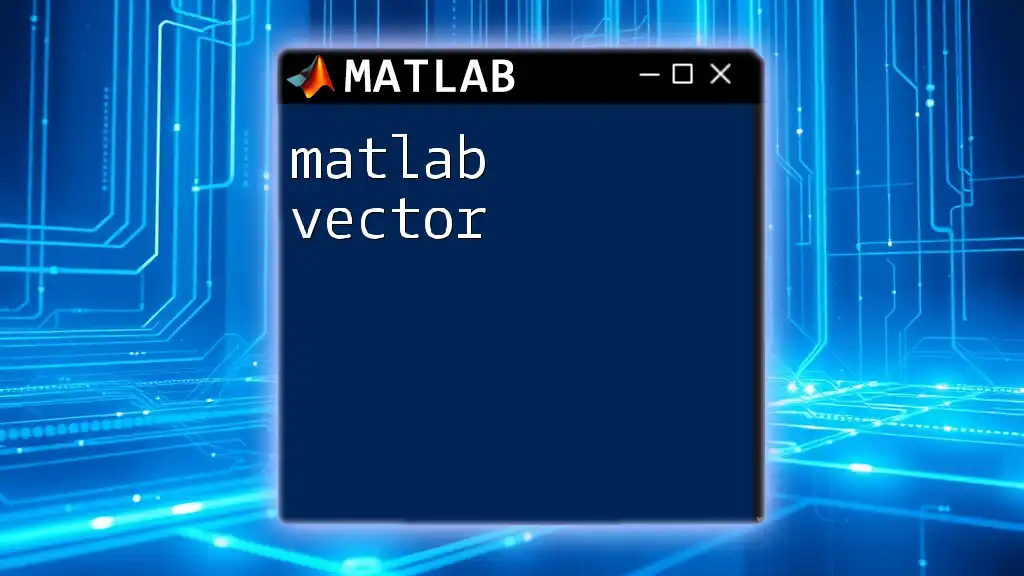
Troubleshooting Common Issues
Common Errors When Using VideoWriter
Some common issues include forgetting to call `open` or `close` and adding frames that do not conform to the expected dimensions. Always ensure that your frames are correctly formatted to avoid runtime errors.
Performance Issues and Solutions
If you notice performance lags, consider reducing the frame dimensions or lowering the frame rate. Profiling your MATLAB script may also reveal bottlenecks that you can address for smoother execution.
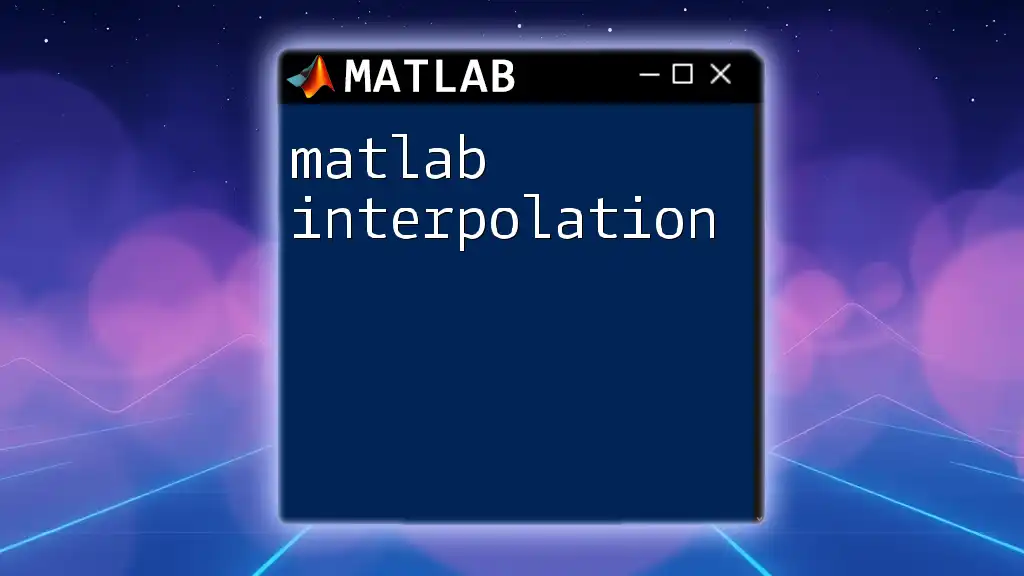
Conclusion
In summary, the matlab videowriter is a versatile and invaluable tool for anyone looking to convert their visualizations or simulations into engaging video formats. By mastering the ability to create, manipulate, and annotate videos, you can effectively showcase your data and insights. Don't hesitate to explore its many facets and experiment with your projects; real learning often comes from the hands-on implementation of these commands.
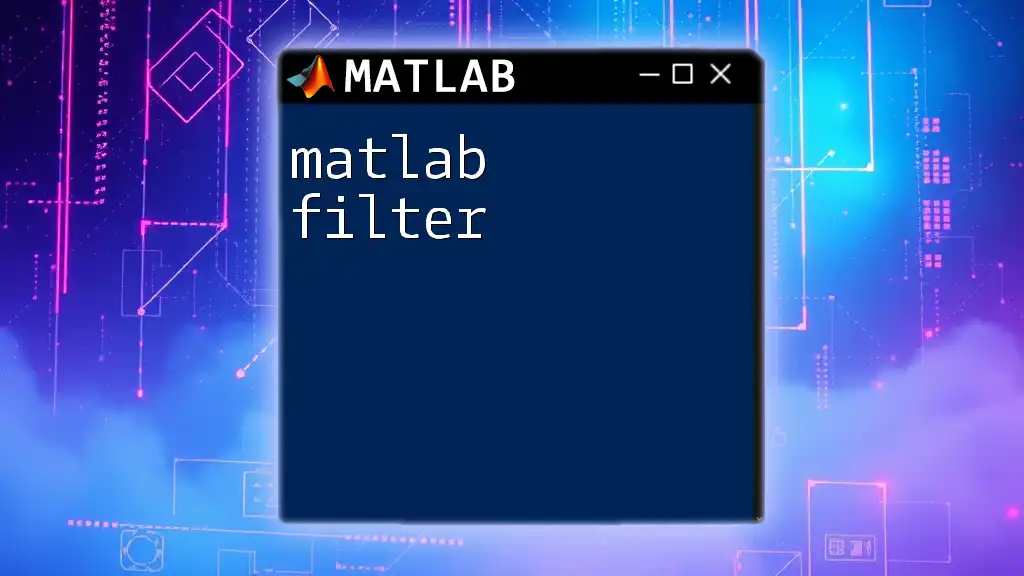
Additional Resources
For further exploration, you can check out MATLAB’s official documentation on VideoWriter for detailed insights and examples. Additionally, consider looking into related commands like `imshow` and `getframe` to enhance your video creation toolkit.