In MATLAB, the "or" operator (`||` for short-circuit evaluation or `|` for element-wise operation) allows you to evaluate multiple conditions or perform logical operations on arrays.
Here's a code snippet demonstrating both types of "or" operators:
% Short-circuit OR operator (returns true if any condition is true)
a = true;
b = false;
result1 = a || b;
% Element-wise OR operator (applies to arrays)
x = [0 1 0];
y = [1 1 0];
result2 = x | y;
Understanding the OR Operator in MATLAB
The OR operator is pivotal in MATLAB, allowing programmers to evaluate multiple logical conditions efficiently. Within MATLAB, there are two primary types of OR operators: logical OR (`||`) and bitwise OR (`|`). Understanding these distinctions is crucial for writing effective and optimized code.
Definition and Syntax
-
Logical OR (`||`): This operator is often used in conditional statements and is known for short-circuit evaluation. It means that if the first condition evaluates to `true`, MATLAB does not evaluate the second condition, which can improve performance.
-
Bitwise OR (`|`): This operator evaluates two arrays element-wise. It's particularly beneficial when working with vectorized operations. Unlike logical OR, both expressions are evaluated regardless of the outcome of the first.
Why Use the OR Operator?
Using the OR operator is essential for combining conditions. In many programming scenarios, you may need to check if at least one of multiple conditions is satisfied. This operator can make your code more efficient and concise, eliminating the need for verbose nested conditional statements.
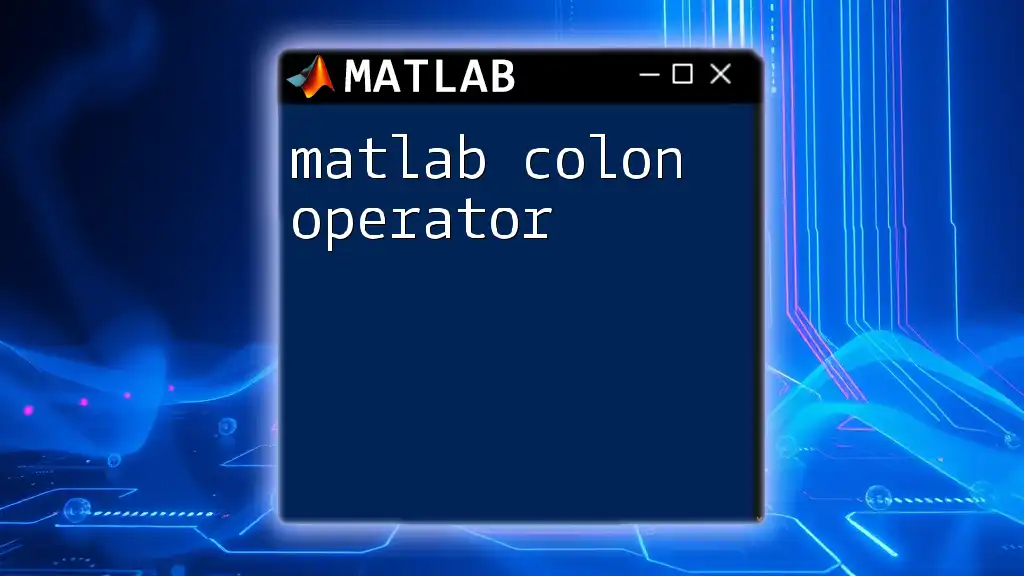
How to Use the OR Operator in MATLAB
Basic Usage
To utilize the OR operator in your MATLAB code, consider the following basic example:
a = true;
b = false;
result = a || b; % result = true
In this example, since at least one condition (`a`) is true, the overall result returns `true`.
Element-wise Operations
The bitwise OR operator functions differently when it comes to arrays. To illustrate its use, take a look at this example:
A = [1 0 1];
B = [0 1 1];
result = A | B; % result = [1 1 1]
In this case, the bitwise OR operator compares corresponding elements of the two arrays, resulting in an array where each element is the logical 'OR' of the elements from `A` and `B`.
Practical Examples
Example 1: Conditional Statements
One common application for the OR operator is within conditional statements. Here’s how you might use it in practice:
x = 15;
if x < 10 || x > 20
disp('x is outside the range of 10 to 20');
end
In this example, the condition checks whether `x` falls outside the range of 10 to 20. If `x` is either less than 10 or greater than 20, it triggers the `disp` function to notify that `x` is outside this range.
Example 2: Filtering Data
The OR operator can also be effective for filtering data. For instance, consider this example where you filter an array based on certain conditions:
data = [1, 3, 5, 7, 9];
indices = data < 3 | data > 7; % Logical array for filtering
filteredData = data(indices); % filteredData = [1, 9]
Here, the bitwise OR operator creates a logical array marking elements of `data` that either are less than 3 or greater than 7. The resulting `filteredData` only includes values that meet those conditions.
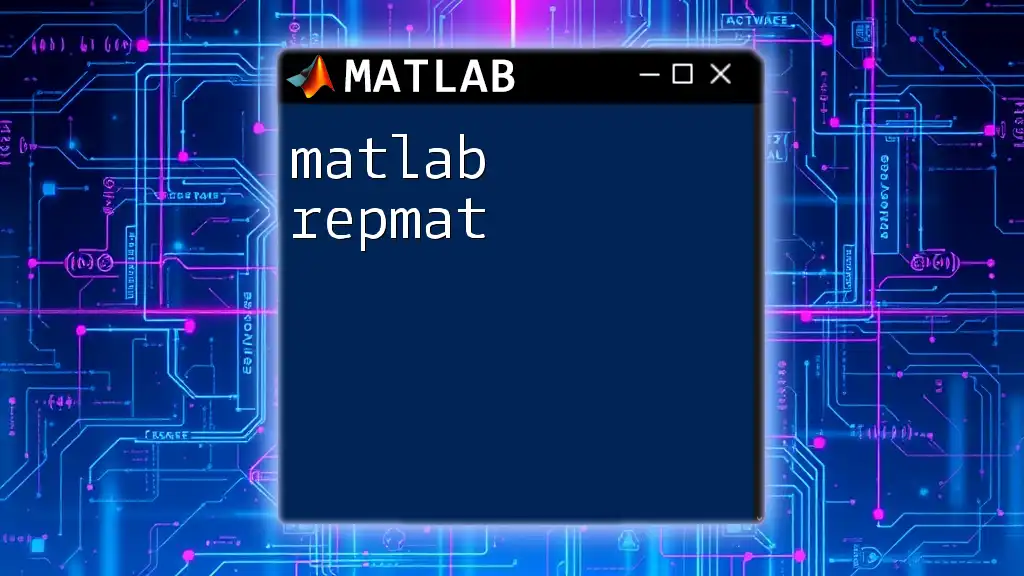
Best Practices When Using the OR Operator
Short-Circuiting with Logical OR (`||`)
Leveraging the short-circuiting behavior of the logical OR operator can be beneficial for performance. When using `||`, if the first condition is true, MATLAB will not evaluate the second condition. This not only saves processing time but also prevents potential errors in complex logical expressions, where subsequent conditions might depend on the first.
Element-wise vs. Short-Circuit OR
It is paramount to choose the correct OR operator based on your needs. Use `||` when dealing with single logical expressions (for instance, in if-statements). Opt for `|` when performing operations over arrays, as this ensures that each corresponding element is evaluated appropriately.
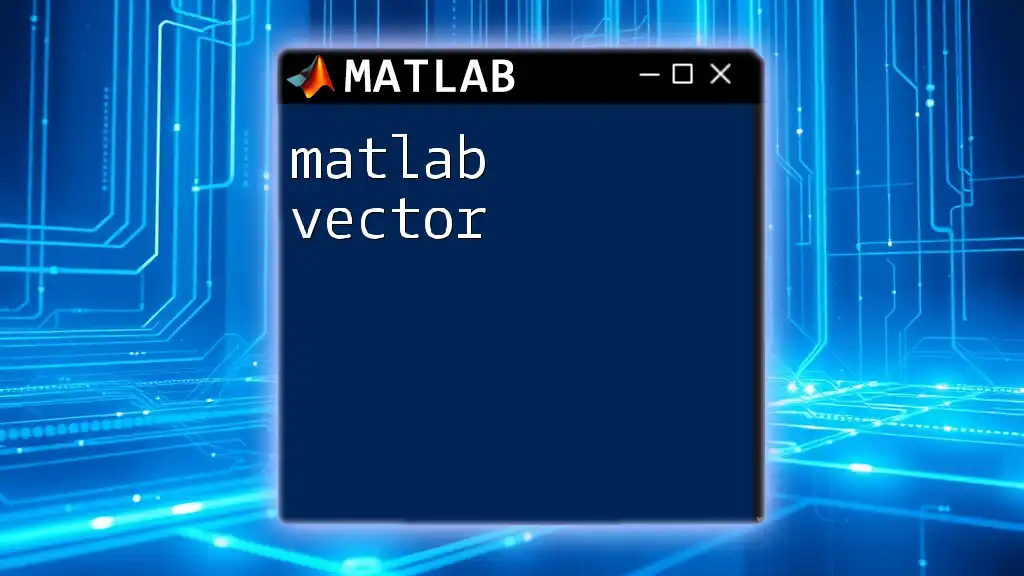
Common Mistakes to Avoid
Confusing Logical and Bitwise Operations
A common pitfall for many beginners is confusing the logical and bitwise operators. It’s crucial to recognize that `||` is not interchangeable with `|`. Using them incorrectly can lead to logic errors in your code.
Ignoring Data Types
Different data types significantly influence the outcome of OR operations. For example, using a string or a non-boolean value with a logical operator can result in unexpected behavior. Always ensure that data types align with your operations to prevent errors.
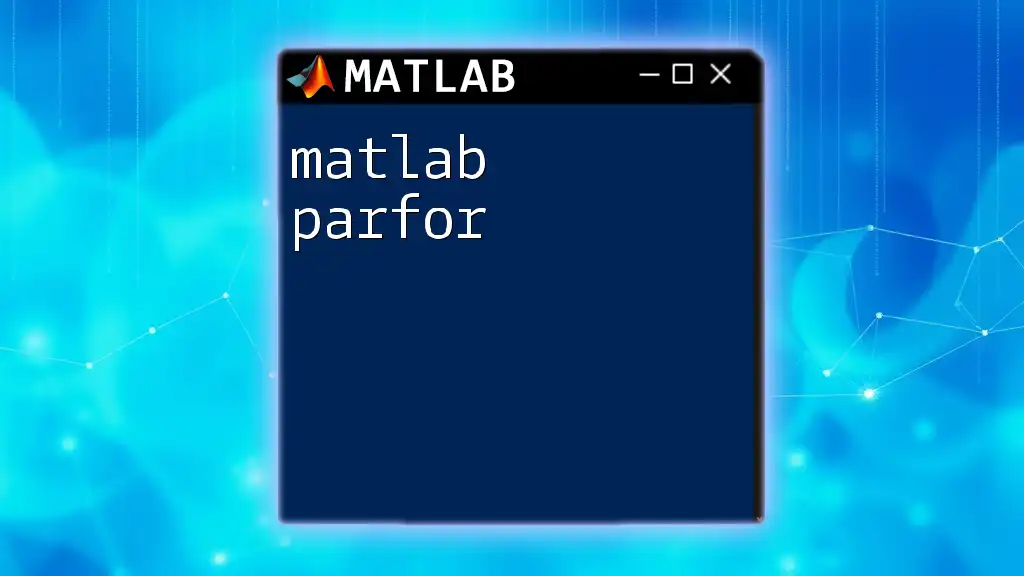
Conclusion
The MATLAB OR operator is a powerful tool that allows programmers to efficiently evaluate multiple conditions. By understanding its syntax and applications, you can streamline your code and enhance its readability. Remember to practice using both the logical and bitwise OR operators to solidify your understanding.
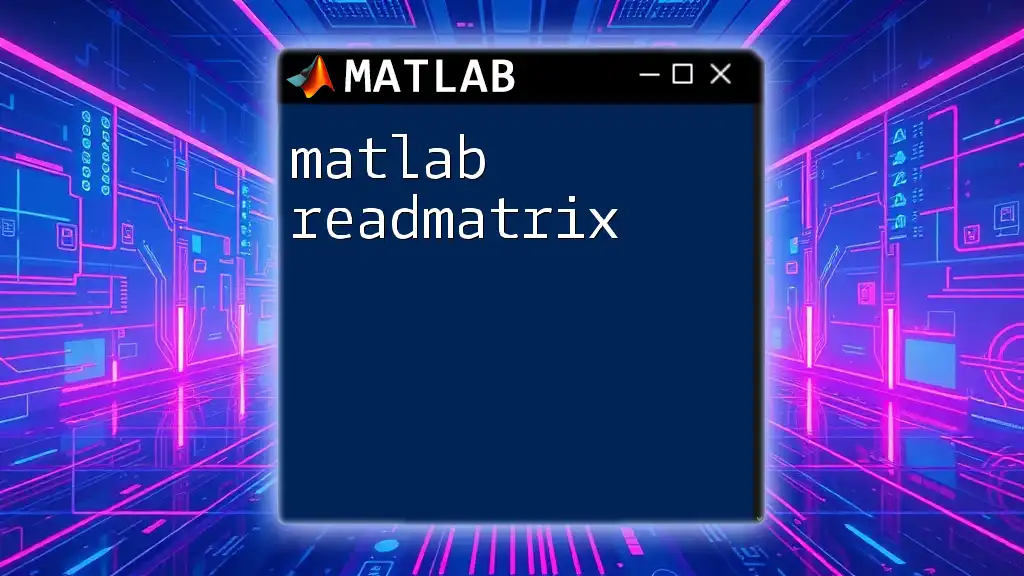
Additional Resources
For further reading, refer to the official MATLAB documentation to deepen your knowledge of the OR operator and explore other logical operations. Additionally, try out the suggested exercises to gain hands-on experience with the concepts presented in this article.