In MATLAB, the `figure` command is used to create a new figure window for plotting, allowing you to visualize data independently of other figures. Here's a simple example:
figure; % Create a new figure window
plot(1:10, rand(1, 10)); % Plot random data
Understanding Figures in MATLAB
What is a Figure?
In MATLAB, a figure refers to a graphical window that displays visual representations of data. Each figure can host a variety of graphical outputs, allowing users to visualize data in unique and informative ways. The primary purpose of a figure window is to facilitate data visualization through plots, graphs, and images, making complex data accessible and understandable.
Types of Figures
MATLAB supports various types of figures, and the choice of figure type often depends on the kind of data being represented. Common types include 2D plots (like line and bar graphs), 3D plots (like surface and mesh plots), and image figures (for visualizing pixel data). Knowing how to choose the appropriate figure type aids significantly in effectively communicating data insights.
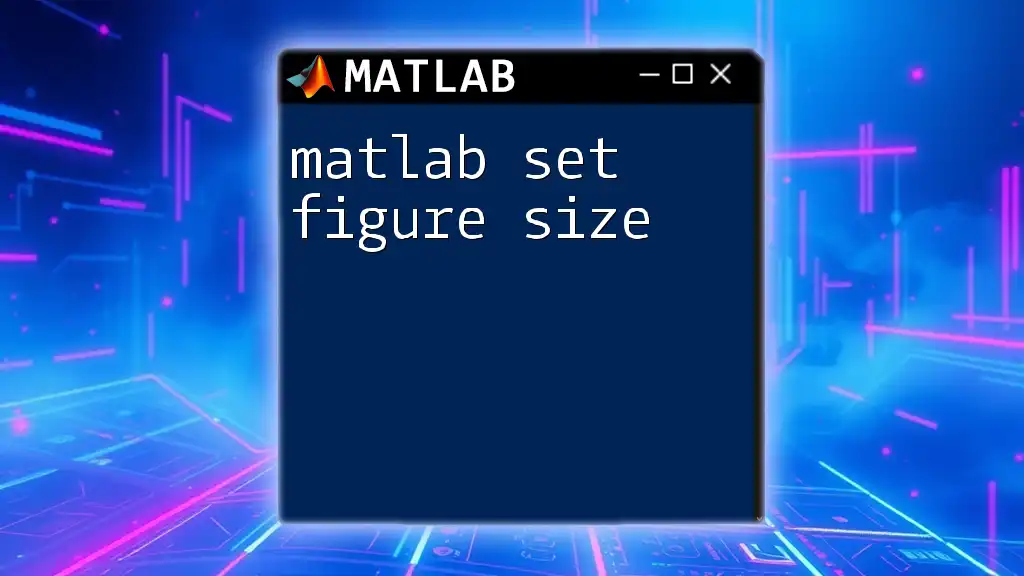
Creating a New Figure in MATLAB
Using the `figure` Command
To create a new figure in MATLAB, you can use the simple command `figure`. This command initializes a blank figure window ready for your graphical output.
Syntax:
figure
The most straightforward example is as follows:
figure;
plot(rand(1, 10));
title('Random Data Plot');
In this example, the `figure` command creates a new figure window, and the `plot` function generates a plot of random data. The title "Random Data Plot" provides context to the viewer.
Specifying Figure Properties
Customizing Figure Properties
When creating a figure, you can customize its properties to suit your requirements. By tweaking figure properties, you can control its appearance and behavior within your MATLAB workspace.
Common Properties:
- `Name`: Title of the figure window.
- `Number`: Sequential number of the figure window.
- `Color`: Background color of the figure.
- `Position`: Size and position of the figure on the screen.
Syntax and Examples
To specify properties while creating a figure, use the following syntax:
figure('PropertyName', PropertyValue)
Here’s an example highlighting customization:
figure('Name', 'My Custom Figure', 'Color', 'w', 'Position', [100, 100, 600, 400]);
plot(linspace(0, 2*pi, 100), sin(linspace(0, 2*pi, 100)));
title('Sine Wave');
In this code snippet, the figure is named "My Custom Figure" with a white background, positioned at coordinates (100, 100) with a width of 600 pixels and a height of 400 pixels. This customization can significantly enhance usability and clarity.
Managing Multiple Figures
Creating Multiple Figures
Often, you may need to create and manage more than one figure simultaneously. Each figure is referenced through a handle, which makes switching between figures straightforward.
Example Code:
h1 = figure('Name', 'Figure 1');
plot(rand(1, 10));
h2 = figure('Name', 'Figure 2');
plot(rand(1, 10), 'r');
The two figures are created with distinct handles: `h1` and `h2`. This allows users to interact with multiple figures within their session seamlessly.
Optional Figures & Command Window Interaction
MATLAB encourages flexibility, enabling users to create figures directly from the command window. You can activate an already-open figure or create a new one by invoking the `figure` command again. This interactive feature fosters an efficient working environment, especially during exploratory data analysis.
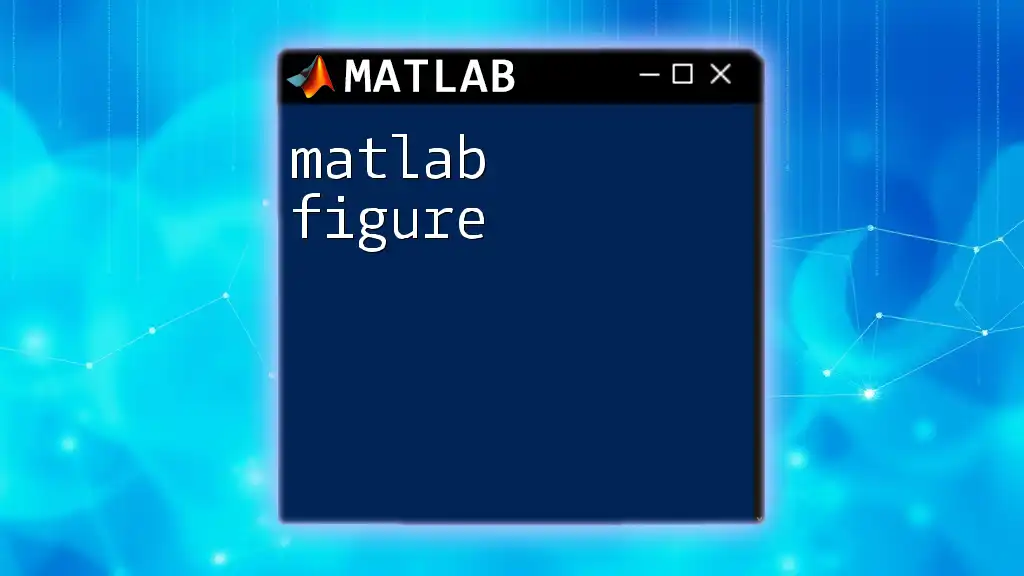
Customizing Figures
Adding Titles and Labels
Titles and axis labels are crucial for enhancing the readability of your figures. A well-labeled figure distinguishes itself and conveys its message effectively.
Example Code:
figure;
plot(cos(linspace(0, 2*pi, 100)));
title('Cosine Function');
xlabel('X-axis (0 to 2\pi)');
ylabel('Y-axis');
In the example above, a title and labels on both axes facilitate a better understanding of the data being represented—allowing viewers to grasp context at a glance.
Adding Legends
When plotting multiple datasets on the same figure, legends become invaluable. They clarify which dataset corresponds to which plot, enhancing comprehension.
Example Code:
figure;
plot(sin(linspace(0, 2*pi, 100)), 'b');
hold on;
plot(cos(linspace(0, 2*pi, 100)), 'r');
legend('Sine Function', 'Cosine Function');
hold off;
In this example, two plots exist within one figure, and the legend distinctly identifies each function—allowing viewers to understand the graphical representation definitively.
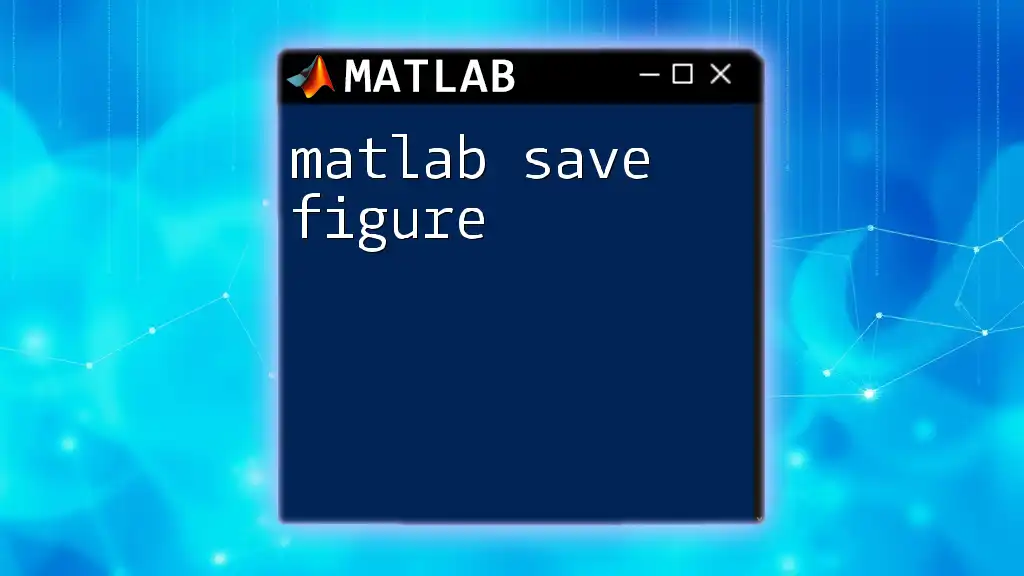
Practical Applications of New Figures
Case Study: Data Visualization
Creating figures plays a fundamental role in data visualization, significantly impacting data analysis and interpretation. For instance, a study involving sensor data can be understood more effectively through visual representation, where trends, anomalies, and patterns become immediately clear through graphs.
Tips for Effective Visualization
To ensure that your figures are both informative and visually appealing, consider the following best practices:
- Maintain consistency in color schemes and styles across figures to improve comprehension.
- Use clear and informative axis labels and titles to guide the viewer.
- Explore various plot types to represent data appropriately, enhancing insights.
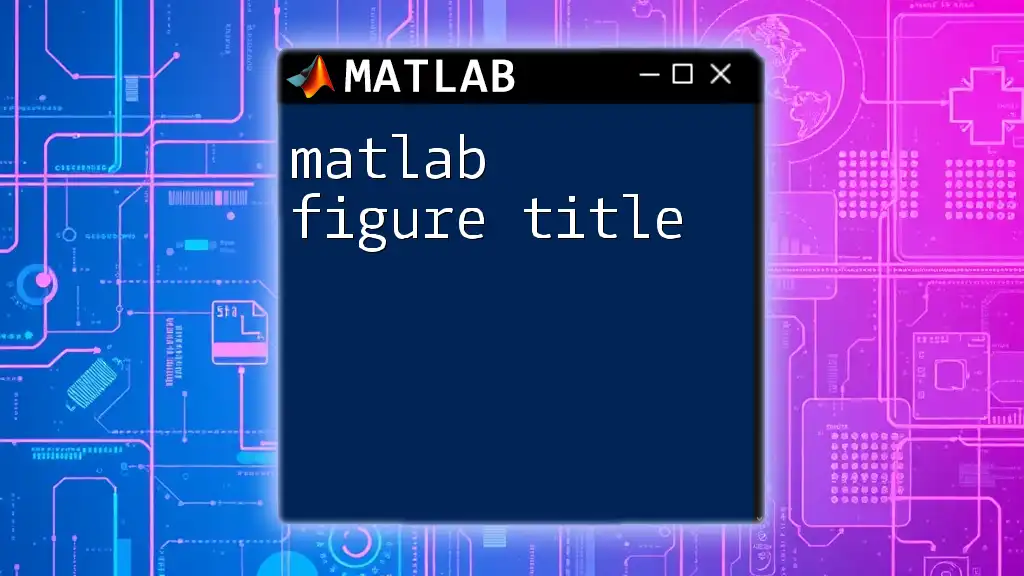
Final Thoughts
Creating and customizing figures in MATLAB is an essential skill for anyone working with data visualization. The flexibility of the `figure` command allows for a personalized and tailored representation of data, ensuring that your insights are impactful and clearly communicated. Engage creatively with the various properties and functionalities that MATLAB offers to explore the full potential of your data visualization efforts.
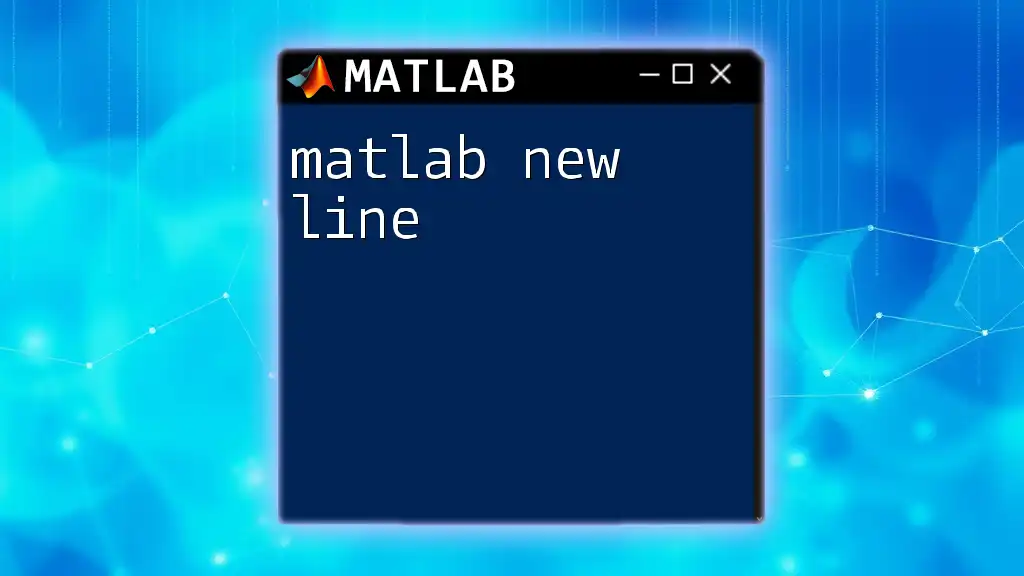
Additional Resources
For those looking to dive deeper into the world of MATLAB, consider exploring the official [MATLAB documentation](https://www.mathworks.com/help/matlab/ref/figure.html) on figure properties and commands. Additionally, online tutorials and courses designed specifically for MATLAB will aid in mastering data visualization techniques.
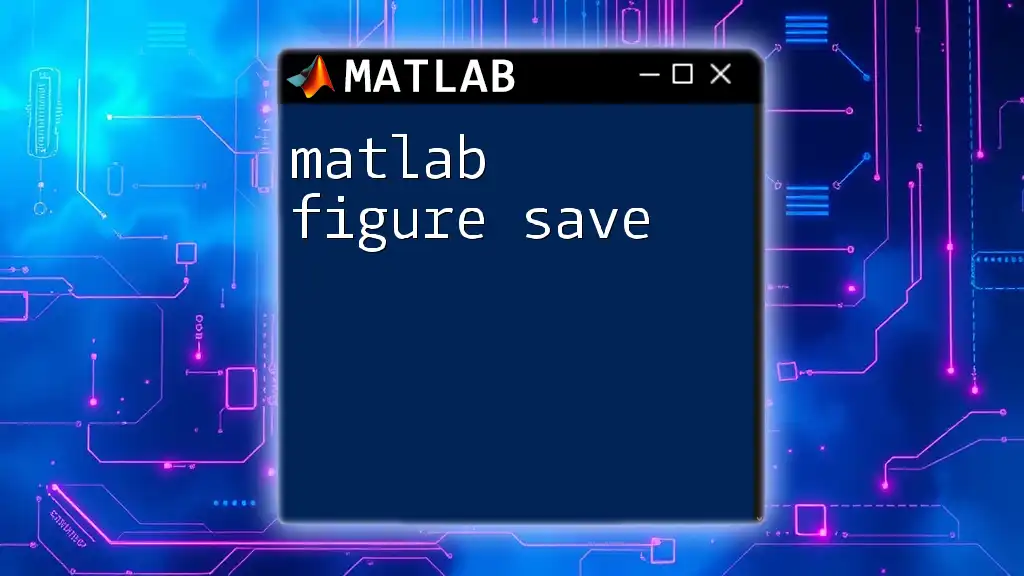
Conclusion
Understanding how to effectively use the matlab new figure command paves the way for improved data visualization methodologies. The tools and techniques discussed here will empower you to create figures that not only convey information efficiently but also enhance audience engagement and comprehension.