In MATLAB, a figure legend is used to identify the data series in a plot, providing clarity by labeling each dataset, typically created with the `legend` function.
Here’s a simple code snippet to add a legend to your plot:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
plot(x, y1, 'r', x, y2, 'b');
legend('Sine Wave', 'Cosine Wave');
What is a Figure Legend?
A figure legend is a descriptive text that explains the symbols, colors, and patterns used in a plotted graph or figure. It serves as a critical guide for interpreting the data presented, helping to clarify the meaning of each dataset within the context of the visualization. Having a clear and informative legend is essential in conveying the results of an analysis effectively.
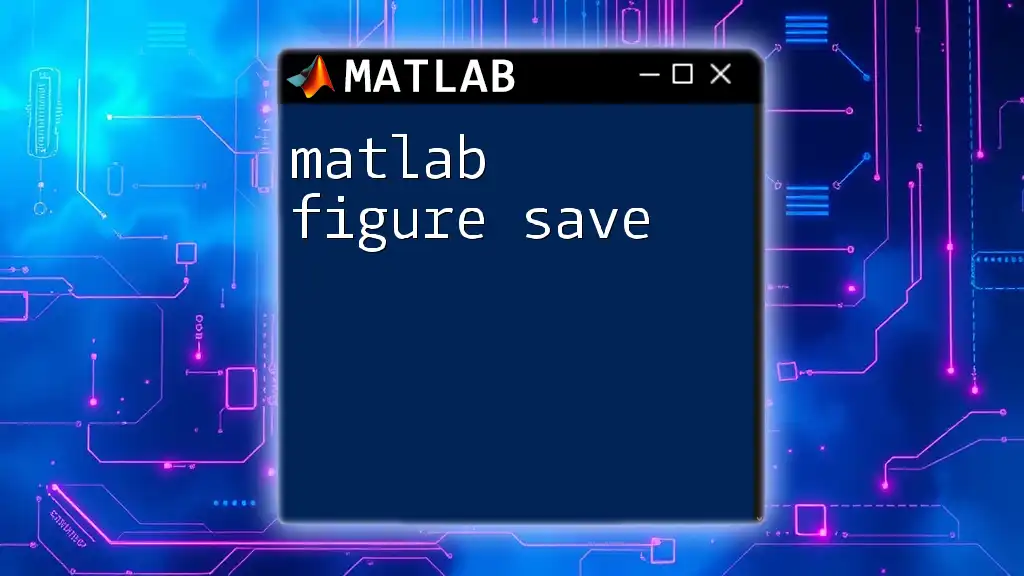
Creating a Basic Figure Legend in MATLAB
To create a basic figure legend in MATLAB, you can use the `legend` function. The syntax is straightforward:
legend(location)
or
legend(string array)
By using the `legend` function, MATLAB identifies the plots you've created and will automatically generate the corresponding entries for them in the legend. Here's a simple example:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
plot(x, y1, x, y2);
legend('Sine Wave', 'Cosine Wave'); % Simple legend
In this example, we plot both a sine and cosine wave and provide a clear label for each in the legend.
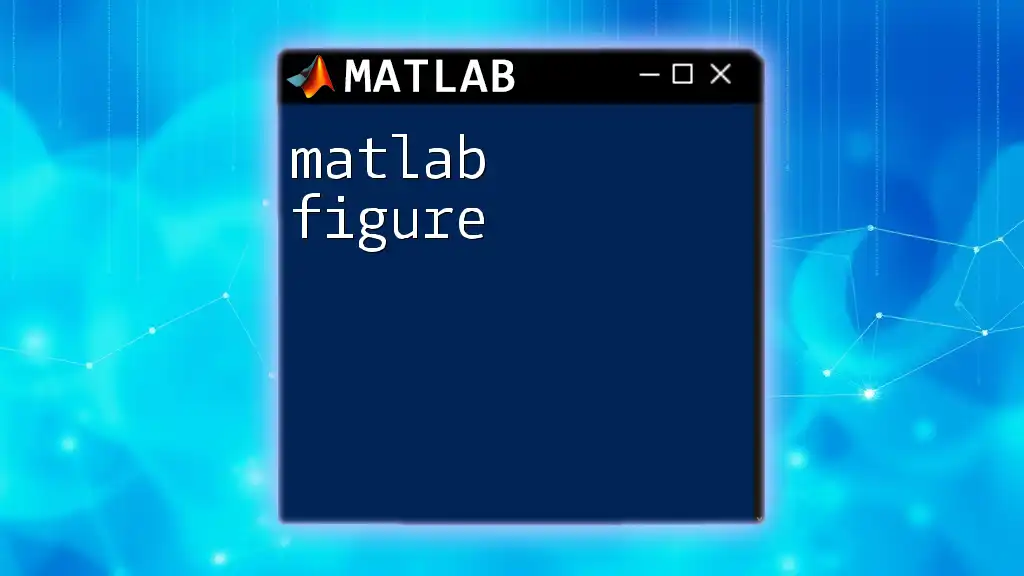
Customizing Figure Legends
Changing Legend Location
MATLAB offers predefined locations for legends, which provide several options for placing the legend within the figure. Common examples include 'northwest', 'southeast', 'northeast', and 'southwest'. Here’s how you can set the location:
legend('Sine Wave', 'Cosine Wave', 'Location', 'northeast');
This function call places the legend in the northeast corner of the plot, ensuring it doesn't obscure the data being visualized.
Adjusting Legend Appearance
Customizing the appearance of your legend can significantly enhance its clarity and visual appeal. You can modify text properties of the legend, such as font size and color. Here’s an example of how to change these properties:
lgd = legend('Sine Wave', 'Cosine Wave');
lgd.FontSize = 12; % Change font size
lgd.TextColor = 'blue'; % Change text color
In this snippet, we set the font size to 12 and change the text color to blue, making the legend more legible and visually striking.
Adding a Box Around the Legend
By default, a box may not surround the legend. You can visually delineate the legend area by using the `Box` property. Here’s how to turn the box on:
lgd.Box = 'on'; % Turn on box around the legend
This slight adjustment adds a clear boundary around the legend, enhancing the overall structure of your figure.
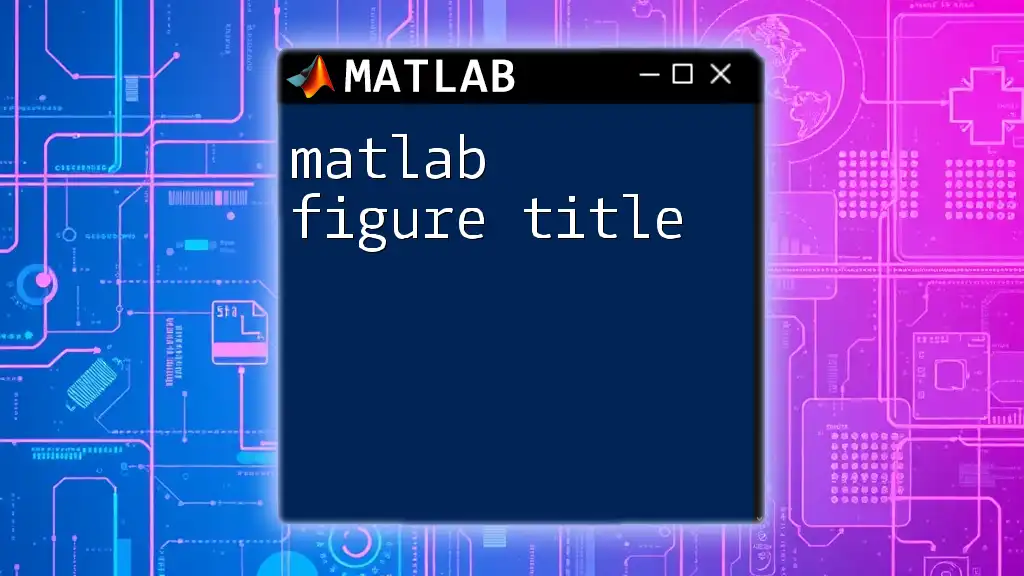
Multiple Legends in One Figure
When dealing with multiple plots or subplots, you might find the need to create multiple legends within the same figure. One effective approach is to utilize multiple axes. Here’s an illustration of how to achieve this:
subplot(2,1,1);
plot(x, y1); legend('Sine');
subplot(2,1,2);
plot(x, y2); legend('Cosine');
In this example, we first create a subplot for the sine wave and assign it a legend. Then, we create a second subplot for the cosine wave, giving both their respective legends. This method allows clearer distinctions between different data sets.
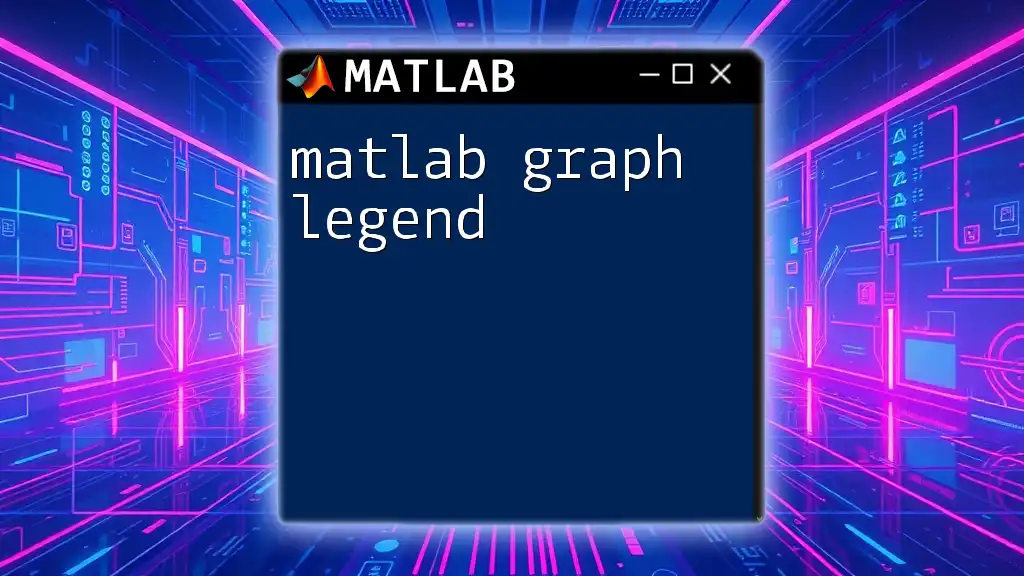
Advanced Customization Techniques
Customizing Legend Items
Sometimes, you may need to hide specific items from the legend for clarity. By using the 'off' keyword, you can prevent certain plot elements from appearing in the legend. Here’s how you can accomplish that:
h = plot(x, y1); hold on;
h2 = plot(x, y2);
lgd = legend([h h2], {'Sin', 'Cos'});
h2.Visible = 'off'; % Hiding the cosine line
In this scenario, the sine wave will be displayed in the legend, while the cosine line is hidden from it, allowing for a cleaner presentation of the data.
Adding Images and Markers to Legends
You can also incorporate different markers in your legend for enhanced representation. For instance, if you want to display a circle marker for the sine wave and a star marker for the cosine wave, you can do this:
plot(x, y1, 'o'); hold on; % Circle marker for sine
plot(x, y2, '*'); % Star marker for cosine
lgd = legend('Sine','Cosine');
This way, the legend visually communicates not just the type of data represented, but also the specific markers, adding another layer of detail.
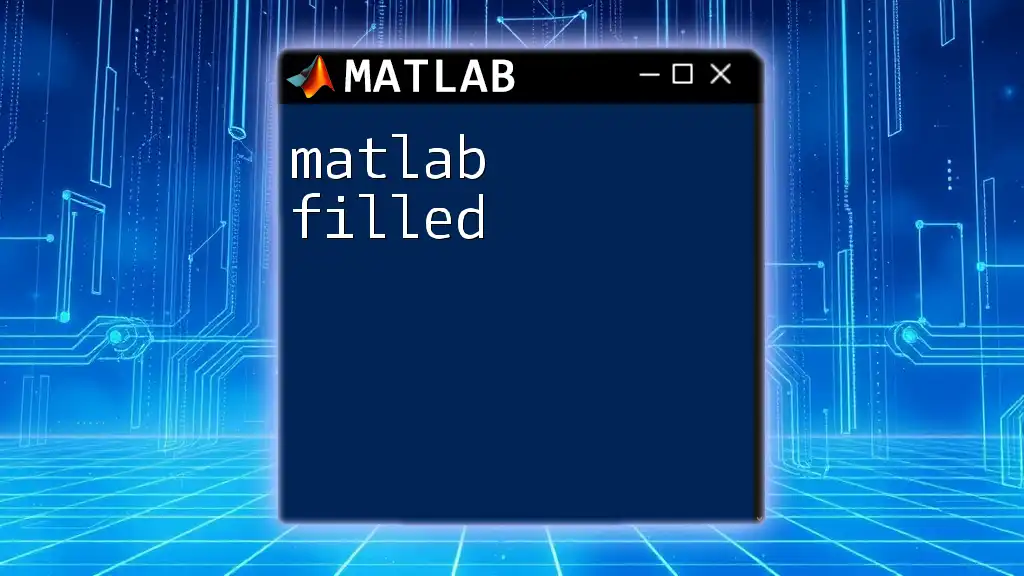
Best Practices for Effective Legends
Keep It Simple
When creating legends, clarity and brevity are crucial. Avoid overly verbose descriptions, and instead focus on concise labels that clearly describe what each line or marker represents. This helps viewers quickly grasp the insights the visualization offers.
Consistency Across Figures
It’s essential to maintain consistency in your legends across multiple figures. Keeping a similar format, size, and color scheme ensures that your data presentation remains coherent and professional, facilitating easier comparisons between different visualizations.
Consider Color Blindness
When designing your figure legends, consider accessibility for colorblind viewers. Choosing colorblind-friendly palettes can make your visualizations more inclusive. There are various tools and resources available to help you select appropriate combinations that are easily distinguishable for all viewers.
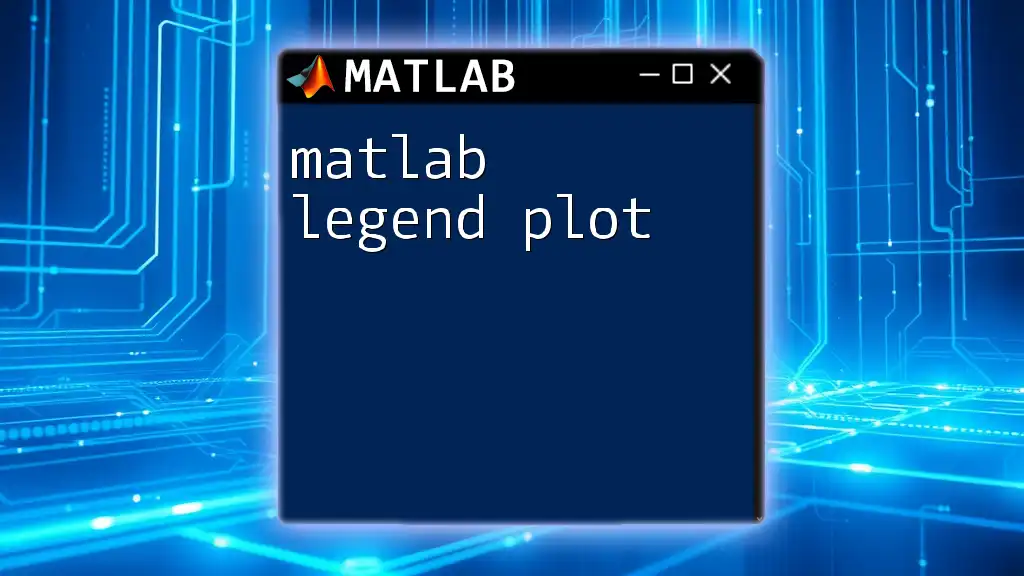
Conclusion
In summary, the MATLAB figure legend is a crucial aspect of data visualization that helps in conveying the information effectively to the audience. By creating clear and concise legends, customizing their appearances, and applying best practices, you can enhance the interpretability of your plots, ensuring that your visual data storytelling is as effective as possible.
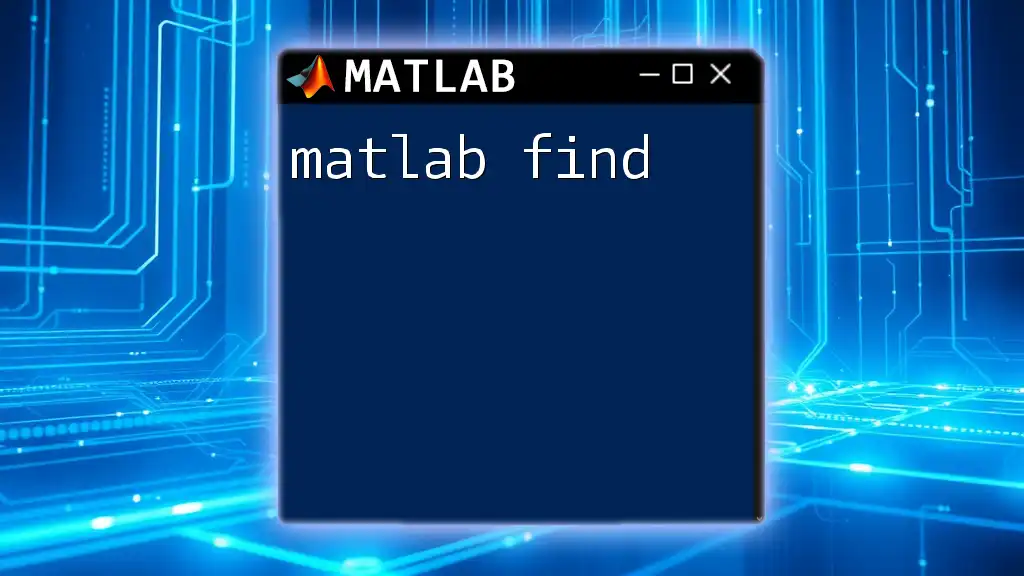
Additional Resources
For more in-depth information, consult the official MATLAB documentation on the `legend` function, and explore additional literature focused on data visualization practices to further enrich your understanding.