The `findpeaks` function in MATLAB identifies local maxima (peaks) in a vector, which can be useful for analyzing data trends.
Here’s a simple example of how to use `findpeaks`:
% Sample data
data = [1 3 2 5 4 6 3 7 2];
% Finding peaks
[peaks, locs] = findpeaks(data);
% Displaying results
disp('Peaks:');
disp(peaks);
disp('Locations of Peaks:');
disp(locs);
Understanding Peak Finding
What is a Peak?
A peak in a dataset is defined as a local maximum point, where the value is higher than its immediate neighboring points. Peaks are crucial indicators in analyses since they can signify significant trends or changes within the data being studied. For instance, in biological signals, peaks might represent heartbeats, while in audio processing, they can define beats or notes.
Applications of Peak Finding
The application of peak finding spans a wide array of fields, including but not limited to:
- Signal processing: Detecting anomalies in audio, bio-signals, and mechanical vibrations.
- Data analysis in scientific research: Identifying key parameters in experimental data, such as the maximum concentration of a substance over time.
- Engineering: Analyzing performance metrics in systems where peak values can indicate failures or efficiency.
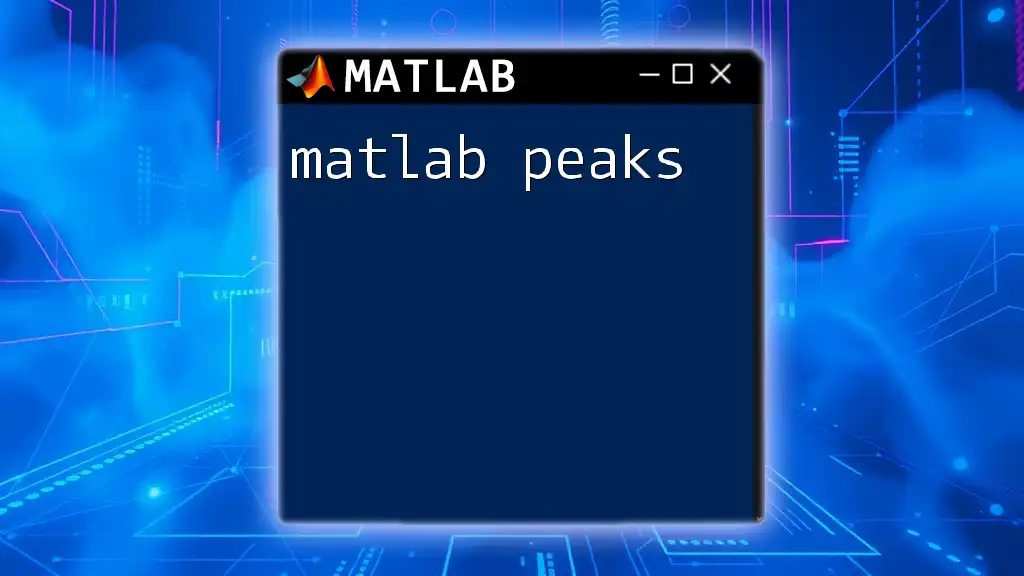
Overview of `findpeaks`
Basic Syntax
The MATLAB function `findpeaks` is employed to identify peaks within a data sequence effectively. The most basic syntax to utilize this function is:
pks = findpeaks(data)
This command will return the peak values of the input data, where `pks` holds the values of all identified peaks.
Parameters and Outputs
Input Parameters
The `findpeaks` function comes with several optional parameters that help tailor the peak-finding process to your specific needs. Some commonly used parameters include:
- `'MinPeakHeight'`: Sets a threshold for the minimum height of a peak.
- `'MinPeakDistance'`: Defines the minimum distance between neighboring peaks, preventing them from being counted as separate peaks.
- `'Threshold'`: Specifies the minimum vertical distance between a peak and its neighboring points.
- `'MinPeakProminence'`: Ensures that found peaks are sufficiently prominent compared to the surrounding data.
- `'Npeaks'`: Limits the maximum number of peaks to return.
Outputs
When using `findpeaks`, the function generates several outputs:
- Peak values: The heights of all detected peaks.
- Locations of the peaks: The corresponding indices in the original data where the peaks occur.
- Properties of the peaks: Additional characteristics, such as prominence and width, can be retrieved when using additional output arguments.
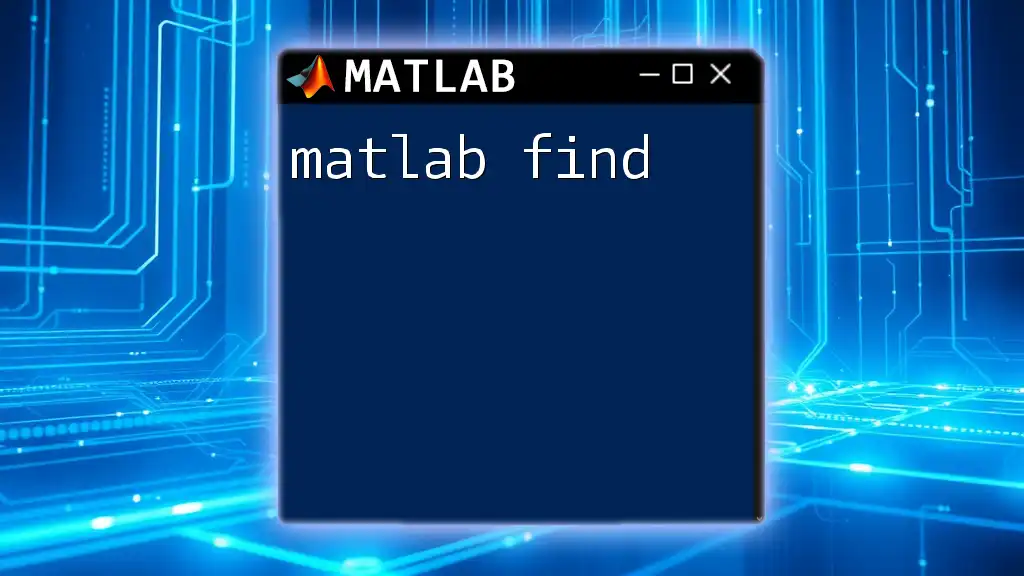
Step-by-Step Guide on Using `findpeaks`
Preparing Your Data
Before utilizing `findpeaks`, it's essential to route your data correctly. The data should be in a one-dimensional array (vector) format for accurate peak identification. You can synthesize sample data in MATLAB using the following code snippet:
x = 0:0.1:10;
data = sin(x) + 0.1*randn(size(x)); % Sample noisy signal
In this example, we create a sine wave and add random noise to simulate real-world data.
Basic Peak Finding
Now that we have our data laid out, we can directly apply `findpeaks` to identify peaks:
[pks, locs] = findpeaks(data);
plot(x, data, x(locs), pks, 'ro');
title('Find Peaks Example');
xlabel('X-axis');
ylabel('Data Values');
Upon executing this code, a plot will be generated displaying the data where the detected peaks are highlighted in red. This visual representation clearly illustrates the locations and magnitudes of the peaks identified by the function.
Customizing Peak Finding
Using Parameters
You can enhance the precision of peak detection by adjusting the parameters, starting with filtering for minimum height. For example:
[pks, locs] = findpeaks(data, 'MinPeakHeight', 0.5);
Applying this line means that only peaks that are at least `0.5` units high will be identified. This parameter helps eliminate noise from your data, focusing only on significant peaks.
Advanced Parameters
You may also cater to the distance between peaks to avoid counting close peaks as separate entities. Applying the `MinPeakDistance` parameter:
[pks, locs] = findpeaks(data, 'MinPeakDistance', 10);
Adjusting this parameter will restrict the function to only return peaks that are separated by at least `10` data points, often resulting in cleaner and more relevant peak detections.
Visualizing Results
Visual analysis is vital for understanding how well `findpeaks` has performed. Besides illustrating the identified peaks, consider generating separate plots for better clarity. Here’s how you can segment your plots:
figure;
subplot(2,1,1); % Original Data
plot(x, data);
title('Original Data');
subplot(2,1,2); % Data with identified peaks
plot(x, data, x(locs), pks, 'ro');
title('Data with Peaks Highlighted');
This arrangement allows viewers to compare the original data against the processed results easily.
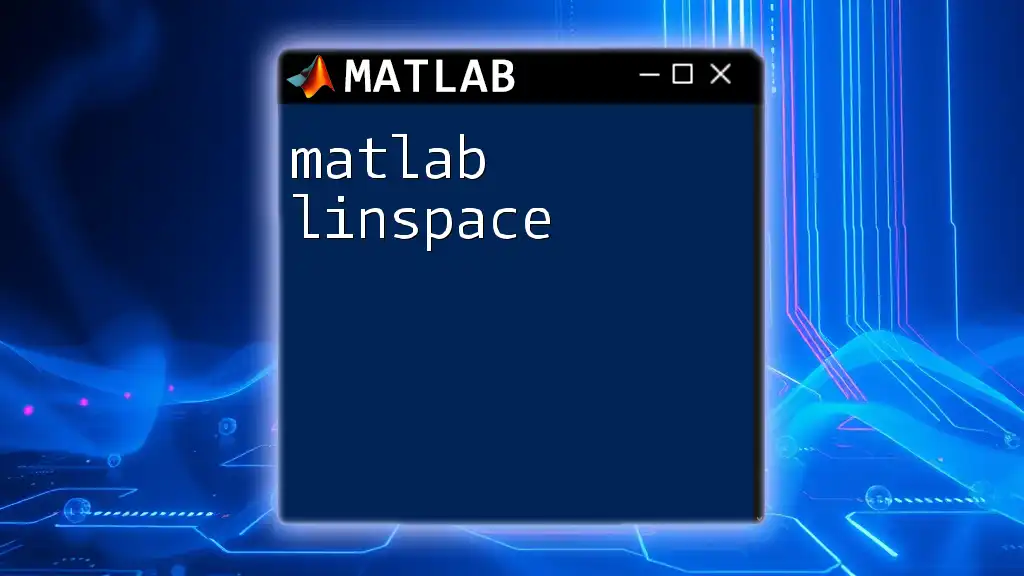
Common Issues and Troubleshooting
Identifying False Peaks
In many cases, datasets can present false peaks due to excessive noise or minor fluctuations. A false peak is typically characterized by small, inconsequential spikes within larger data trends. To reduce the occurrence of these false peaks, ensure to tweak parameters like `MinPeakHeight` and `MinPeakProminence`, focusing on thresholds that fit your data's nature.
Adjusting Thresholds
Tuning parameters often requires experimentation. Finding the right balance is vital; if thresholds are set too high, significant peaks may be missed, while too low thresholds can lead to false detections. Consider analyzing results iteratively, adjusting parameters based on previous findings.
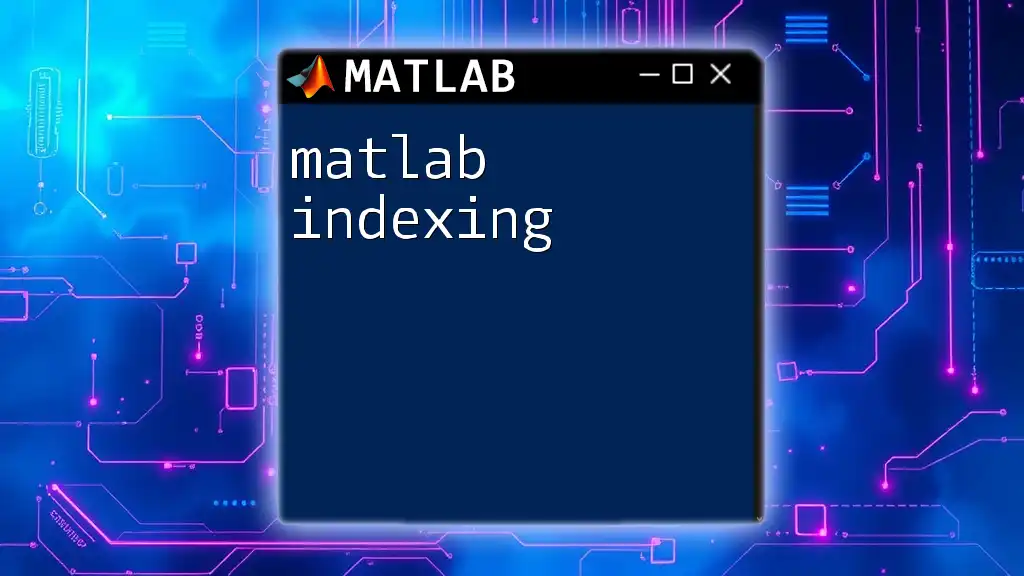
Practical Applications and Examples
Signal Processing Example
One of the prominent areas where the `findpeaks` function shines is in analyzing audio signals. For instance, let's consider peak detection in sound waves:
% Read an audio file
[y, FS] = audioread('sample.wav');
pks = findpeaks(y);
This method processes the audio signal effectively, allowing you to capture peaks that correspond with loud sounds or musical notes present in the piece.
Biological Data Analysis
In the field of biology, peak detection is crucial when interpreting heart-related data or other bio-signals. For instance, finding peaks in heart rate data can pinpoint crucial moments indicative of health changes, providing real-time insights for diagnostics.
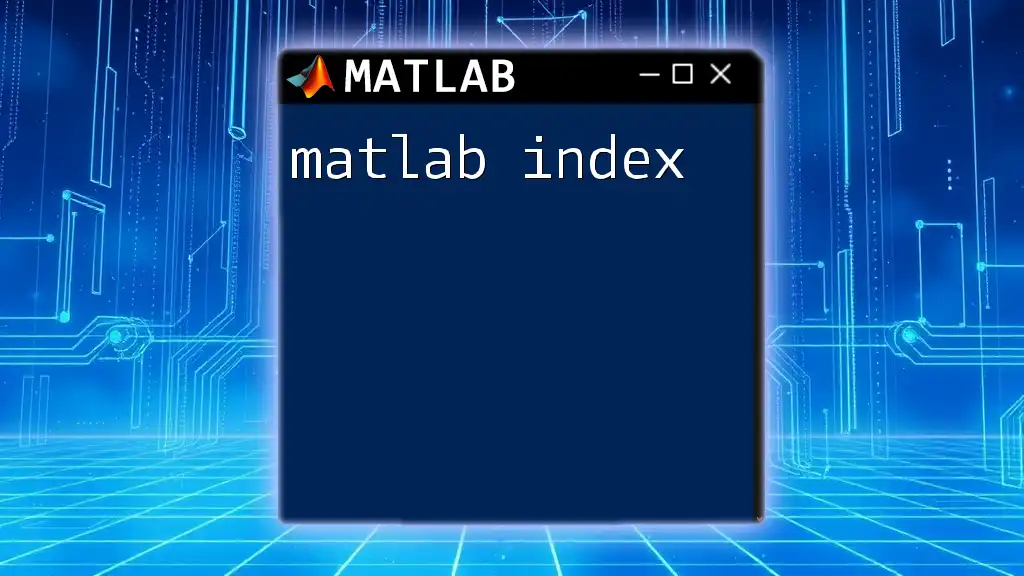
Conclusion
The MATLAB `findpeaks` function is a robust tool that enables users to identify significant trends and patterns within datasets, enhancing data analysis and interpretation. By understanding the function's parameters, practicing with various datasets, and customizing your approach, you can significantly improve your ability to detect and analyze peaks effectively. Embrace this powerful tool—experiment, learn, and apply it to your own datasets!
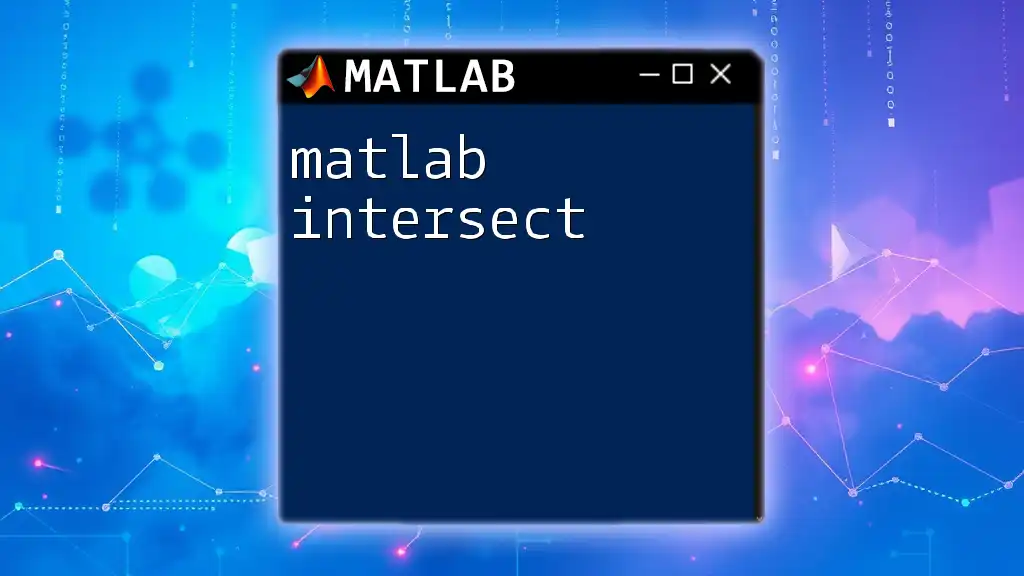
Additional Resources
For further exploration, refer to the official MATLAB documentation on `findpeaks` for in-depth technical details, and don’t hesitate to access interactive tutorials or additional reading materials to strengthen your understanding and usage of MATLAB in data analysis.