In MATLAB, the `size` function returns the dimensions of an array or matrix, allowing users to determine the number of rows and columns it contains.
Here’s a code snippet demonstrating its usage:
A = [1, 2, 3; 4, 5, 6]; % Create a 2x3 matrix
dimensions = size(A); % Get the size of the matrix A
disp(dimensions); % Display the dimensions
Understanding `sizeof` in MATLAB
Introduction to Memory Management
Memory management is a crucial aspect of programming that directly impacts the performance and efficiency of your applications. Understanding how much memory your variables use allows you to optimize your code and avoid potential bottlenecks, especially when working with large datasets or complex calculations in MATLAB.
Differentiating Between `sizeof` in MATLAB vs. Other Languages
In many programming languages such as C, C++, and Python, the `sizeof` operator is commonly used to determine the amount of memory (in bytes) that a data type or variable occupies. However, MATLAB does not feature a direct `sizeof` command. Instead, it provides a variety of other tools, like the `whos` command and functions such as `numel`, to help you manage memory effectively.
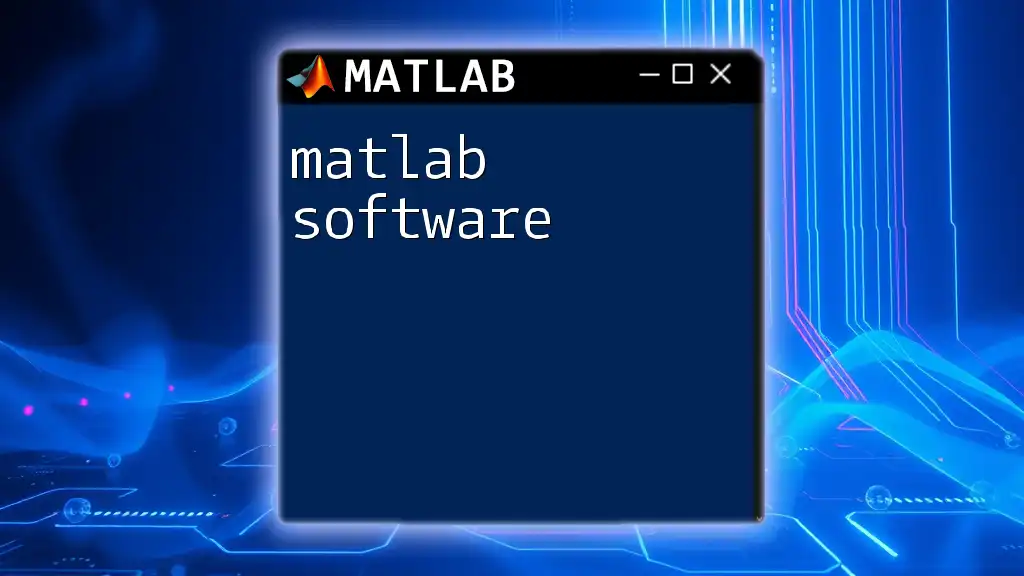
MATLAB Data Types and Memory Consumption
Common Data Types in MATLAB
MATLAB supports several fundamental data types, each with their memory sizing characteristics:
- Numeric Types: The most commonly used are `double` (default, 8 bytes), `single` (4 bytes), and various integer types like `int8`, `int16`, etc.
- Character Arrays and Strings: Character arrays consume 2 bytes per character in MATLAB.
- Cell Arrays and Structures: Memory usage can vary based on the complexity and types of contents.
Memory Size of Different Data Types
Determining how much memory each of these data types uses is essential for optimizing performance. To calculate memory size, you can use the `whos` command, which provides detailed information about the variables in your workspace.
Here's an example of how you might use this in practice:
num = 100; % double
charArr = 'Hello'; % character array
structVar = struct('field', 1); % structure
fprintf('Size of num: %d bytes\n', whos('num').bytes);
fprintf('Size of charArr: %d bytes\n', whos('charArr').bytes);
fprintf('Size of structVar: %d bytes\n', whos('structVar').bytes);
This code snippet will display the memory sizes of variables `num`, `charArr`, and `structVar`, revealing fascinating insights into how each one consumes RAM.
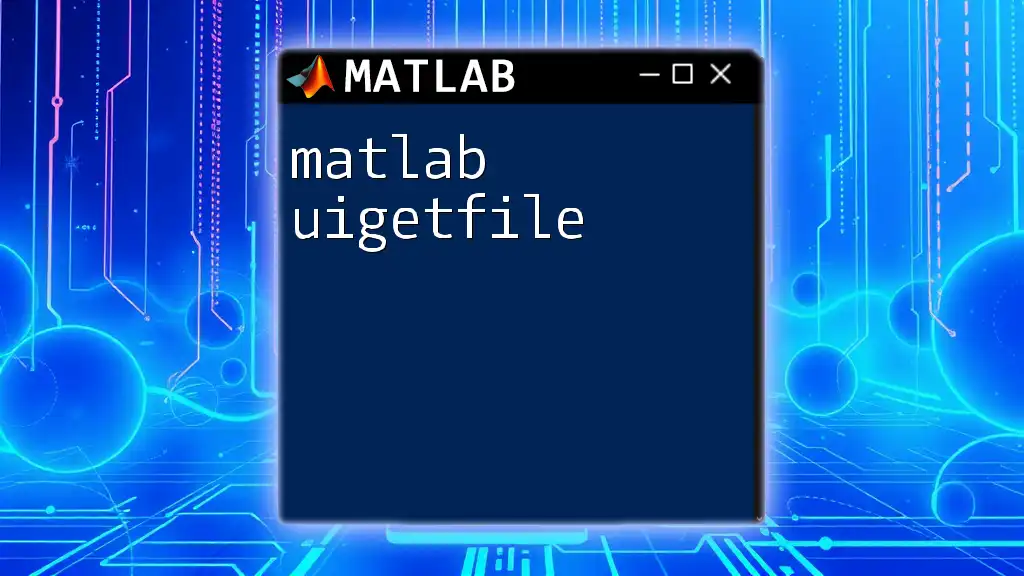
Using the `whos` Command for Memory Size
Overview of the `whos` Command
The `whos` command is a powerful tool in MATLAB for generating information about the variables currently in the workspace. It reveals their names, sizes, memory requirements, and data types, enabling you to make informed decisions when managing memory.
Practical Examples of Using `whos`
To see how `whos` works, let's consider multiple variable types in one command.
a = 5;
b = 'MATLAB';
c = [1, 2, 3; 4, 5, 6];
whos % Display all variables and their sizes
In this example, when you run the `whos` command, MATLAB provides a detailed table outlining the size, bytes, and class of each variable. This helps you see the memory footprint of your workspace at a glance.
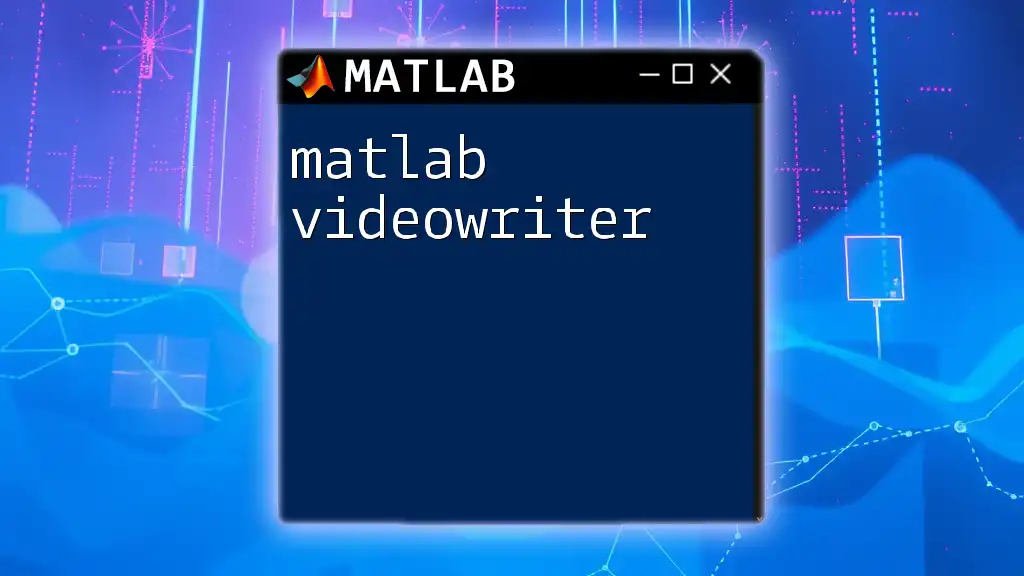
The `numel` Function and Its Usefulness
Understanding `numel`
The `numel` function is invaluable in MATLAB for determining the number of elements in an array or data structure. This is especially important when you need to assess how large a dataset is and its potential impact on memory usage.
Code Examples for `numel`
You can easily find out the number of elements in various data structures by utilizing the `numel` function. Here’s how it can look in practice:
vector = [1, 2, 3, 4, 5];
matrix = [1, 2; 3, 4];
cellArray = {1, 2, 3, 'text'};
fprintf('Number of elements in vector: %d\n', numel(vector));
fprintf('Number of elements in matrix: %d\n', numel(matrix));
fprintf('Number of elements in cellArray: %d\n', numel(cellArray));
This code will print the number of elements in each data structure, helping you visualize the contents and complexity of your variables.
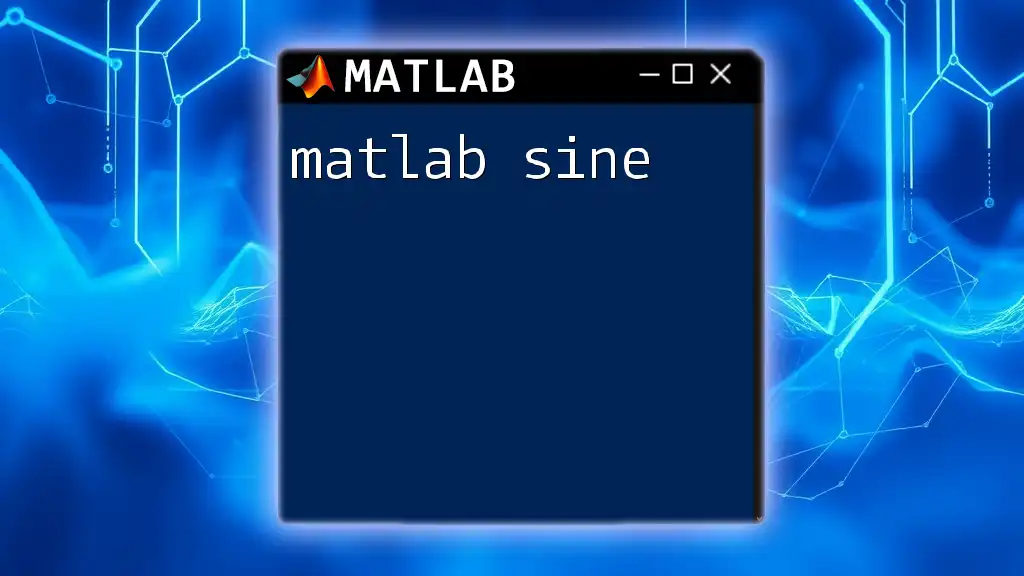
Calculating Overall Memory Usage of Your Environment
Using the `memory` Command in MATLAB
To gain an understanding of memory consumption and allocation in your MATLAB environment, the `memory` command is your go-to option. This command gives you an overview of memory limits imposed on your MATLAB session, as well as the memory in use.
Example Code for Memory Overview
You can simply type the following command in the MATLAB command window:
memory % Display information about memory limits in the MATLAB environment
This command will output crucial information regarding your available memory, enabling you to identify if you’re approaching any memory limits—a particularly important task when dealing with large datasets.
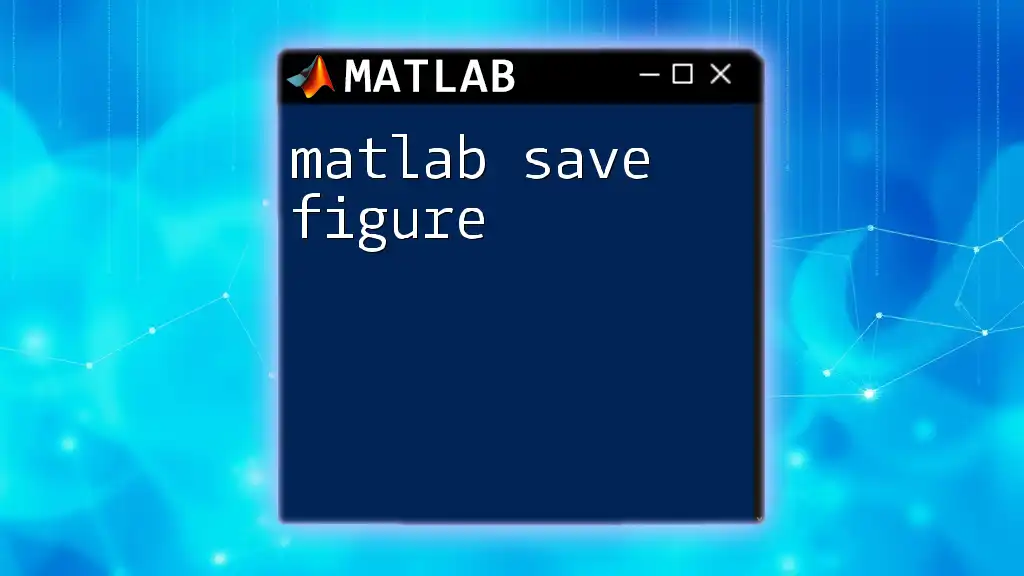
Summary of Key Points
Recap of Memory Management Strategies
Understanding the memory usage in MATLAB is vital for writing efficient code. Take advantage of commands like `whos`, `numel`, and `memory` to monitor and manage your variable sizes. Knowing how much memory your data types consume allows you to optimize your resources wisely, ensuring your MATLAB applications run smoothly and efficiently.
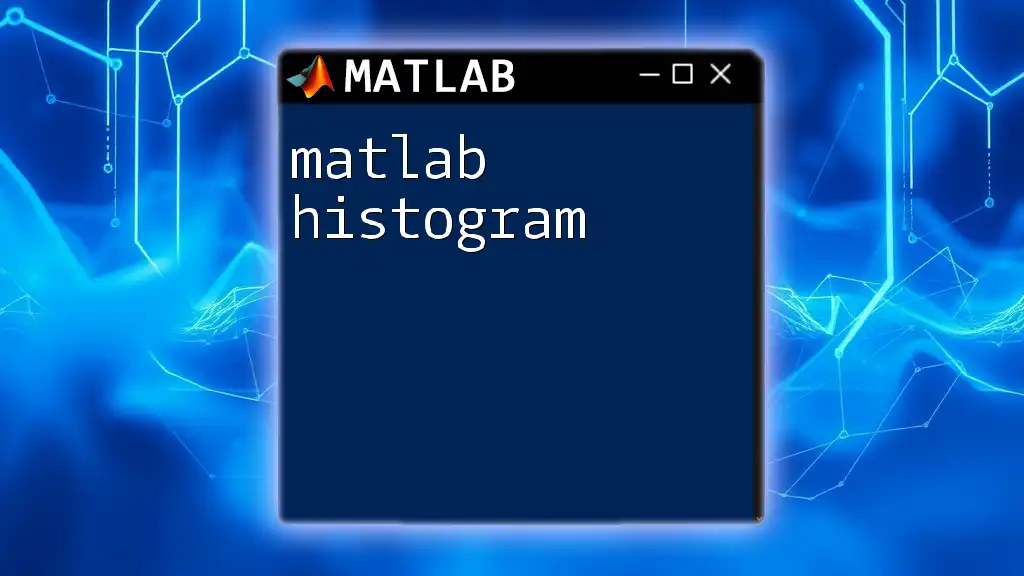
Further Resources
Recommended MATLAB Documentation
For those interested in diving deeper into the world of memory management in MATLAB, consult the official MathWorks documentation, which contains in-depth resources on optimizing performance and memory allocations.
Join Our MATLAB Community
If you're eager to learn more, consider joining forums and communities focused on MATLAB. Also, connect with our company for succinct, comprehensive tutorials designed to help you master MATLAB commands in no time. Let us guide you on your journey to becoming proficient in MATLAB!