`parfor` is a parallel for loop in MATLAB that allows you to execute iterations of a loop concurrently, thereby improving the performance of computationally intensive operations.
Here’s a simple example of how to use `parfor` in MATLAB:
parfor i = 1:10
output(i) = i^2; % Each loop iteration calculates the square of i
end
Understanding `parfor`
What is `parfor`?
The `parfor` command in MATLAB is a powerful tool that enables parallel for-loops. Unlike traditional `for` loops, `parfor` allows iteration over a range of values concurrently, making it ideal for large datasets or computationally intensive tasks.
When to Use `parfor`
You should consider using `parfor` when:
- Iterating over large datasets where computations are independent.
- Performing operations that can be parallelized without needing to maintain the loop order.
- Working on tasks that are computationally expensive and can benefit significantly from concurrent execution.
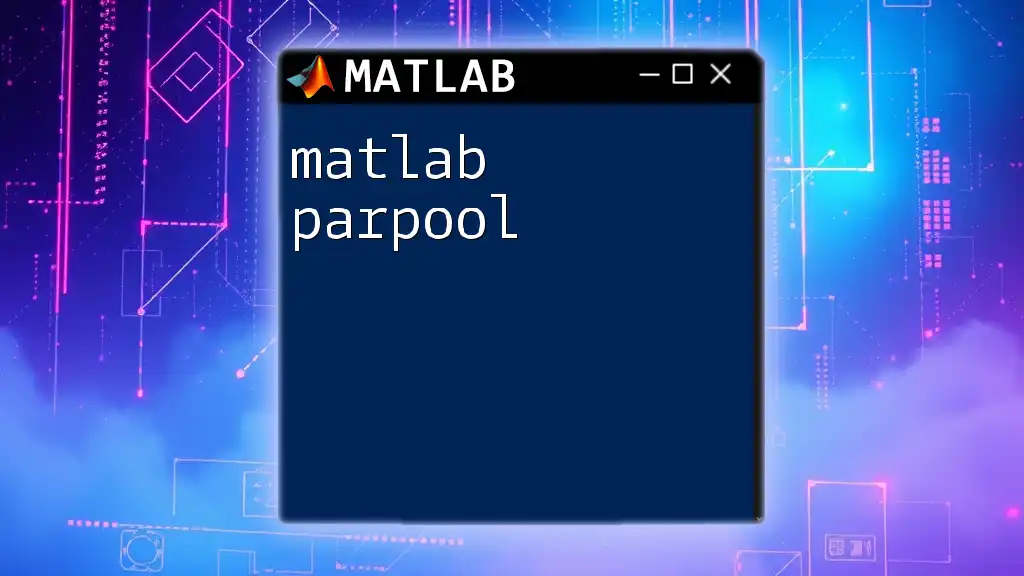
Setting Up Your Environment for Parallel Computing
Requirements for `parfor`
To utilize `parfor`, ensure you have the following:
- A compatible MATLAB version with the Parallel Computing Toolbox installed.
- Hardware that supports parallel processing, such as a multi-core CPU.
Configuring MATLAB's Parallel Computing Toolbox
Getting started with parallel computing in MATLAB requires enabling the Parallel Computing Toolbox. You can do this by following these steps:
- Open MATLAB and navigate to the Home tab.
- In the Environment section, click on “Preferences.”
- Expand the “Parallel Computing Toolbox” section and configure settings based on your hardware capabilities.
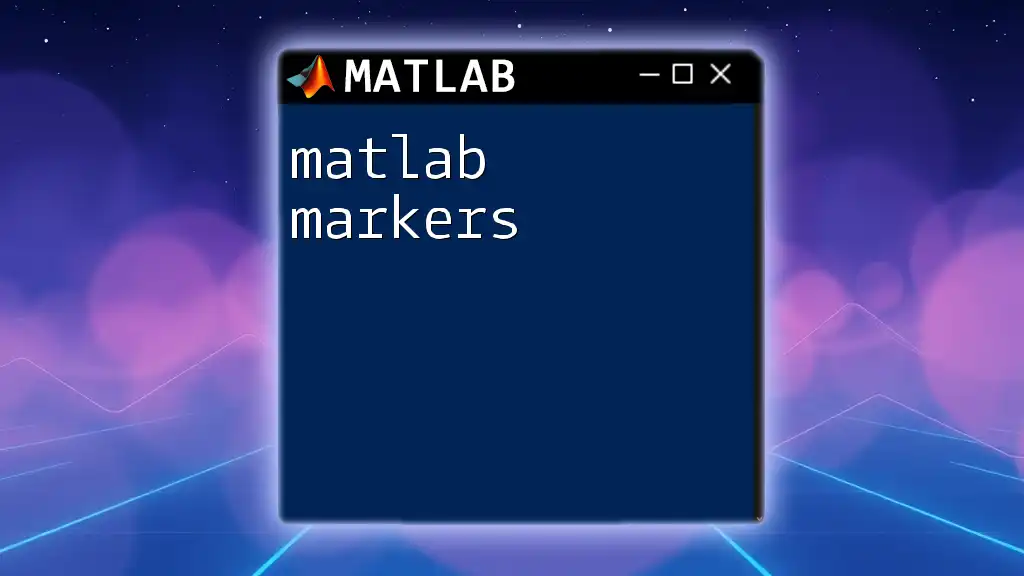
Working with `parfor`: Syntax and Structure
Basic Syntax of `parfor`
The basic syntax of a `parfor` loop is quite similar to a `for` loop. Here’s an example:
parfor index = 1:N
% Your code here
end
Within this loop, MATLAB will handle the distribution of iterations across available workers in the parallel pool.
Variables in `parfor`
It's crucial to understand how to manage variables in `parfor`. There are three main types of variables you’ll encounter:
-
Sliced variables: These are variables that change for each iteration of the loop, such as an array where each index is computed independently.
A = zeros(1, N); parfor i = 1:N A(i) = someFunction(i); end
-
Broadcast variables: These are constants shared across all iterations. For example, if you have a predefined matrix shared by all iterations, it can be defined outside the loop.
-
Reduction variables: These are variables used to accumulate results, such as sums or averages, but they must be properly structured since each worker must compute its contribution independently.
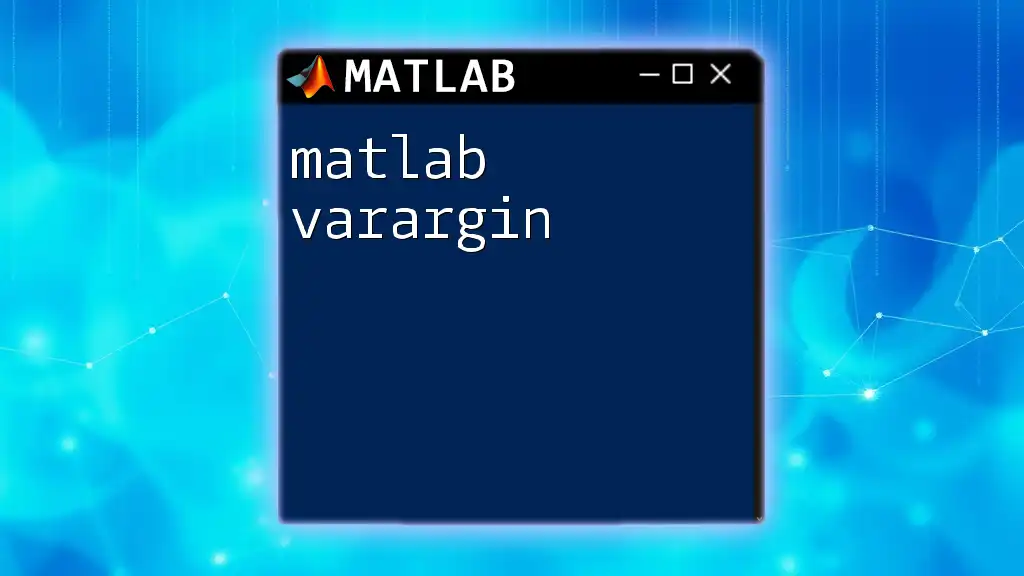
Detailed Examples of `parfor` in Action
Example 1: Simple Parallel Loop
Here's how you can use `parfor` to perform a basic mathematical operation in parallel:
parfor i = 1:100
results(i) = i^2;
end
In this example, MATLAB computes the square of numbers from 1 to 100 in parallel, significantly speeding up execution.
Example 2: Using Sliced Variables
Here’s another example demonstrating how to work with sliced variables using `parfor`:
parfor i = 1:N
A(i) = expensiveComputation(i);
end
This parallelizes the computation across N iterations, ensuring each element of A is calculated independently.
Example 3: Handling Nested Loops
One must tread carefully with nested loops in `parfor`. Here's an illustration of how not to structure them:
% Avoid this:
parfor j = 1:M
for i = 1:N
results(j, i) = complexOperation(j, i);
end
end
The reason this is problematic is that `parfor` doesn’t support parallelizing across multiple dimensions without careful structuring, and nested operations can break the independence requirement.
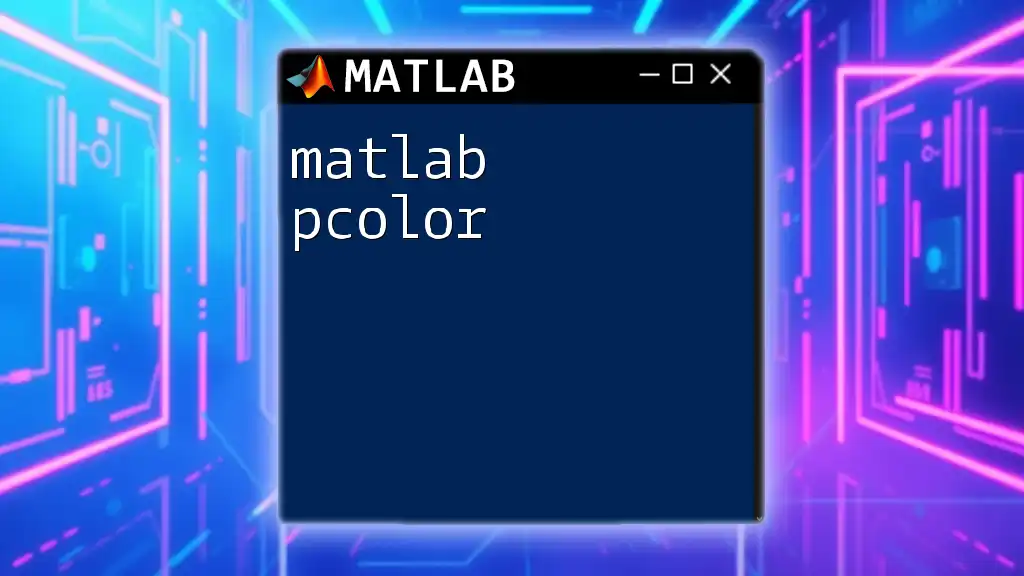
Common Pitfalls and Best Practices
Understanding Loop Dependencies
When using `parfor`, it is crucial to maintain loop independence. If one iteration relies on the results of another, you will encounter issues. Carefully analyze your code for dependencies before utilizing `parfor`.
Performance Considerations
While `parfor` can provide substantial performance improvements, it introduces overhead due to the setup of parallel workers. You should weigh the time saved in computations against the overhead time. Small loops may not benefit from `parfor`, so test performance improvements in realistic scenarios.
Memory Management
Effective management of memory becomes increasingly important as you utilize `parfor`. Large data sets can lead to memory exhaustion if not handled properly. Aim to minimize the size of data shared among workers and avoid creating large intermediate results within the loop.
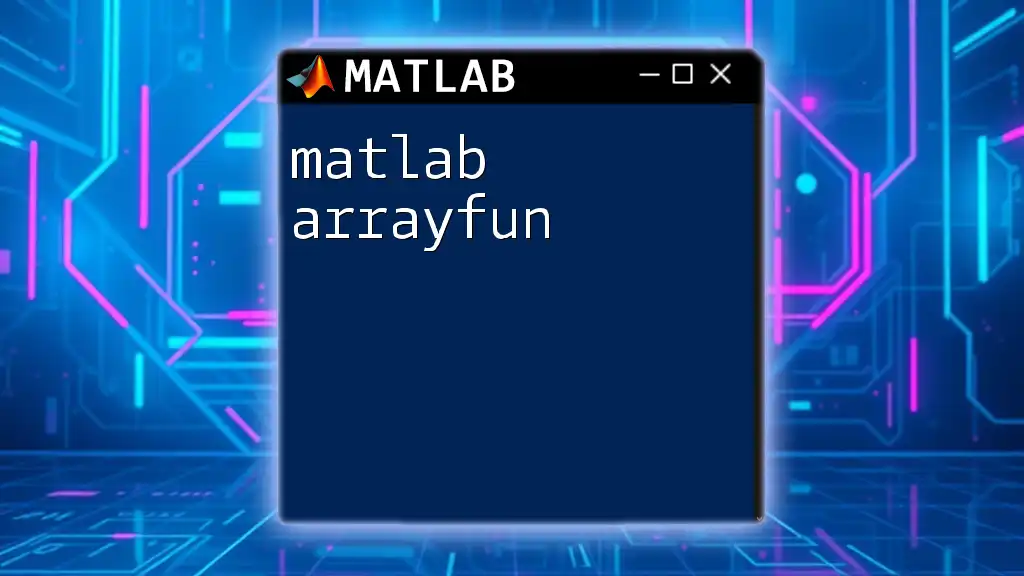
Advanced Features of `parfor`
Nested `parfor` Loops
MATLAB does not support true nested `parfor` loops due to the need for independent iterations. For advanced strategies, consider restructuring your code to flatten loops where possible or using additional MATLAB functions to handle complex data manipulations.
Customizing Parallel Pool Settings
Optimize your parallel pool settings based on your hardware capabilities for maximum efficiency. You can customize the number of workers, which can be achieved by:
parpool('local', numWorkers); % numWorkers is the number of CPU cores available
This allows you to align the amount of work with the resources available.
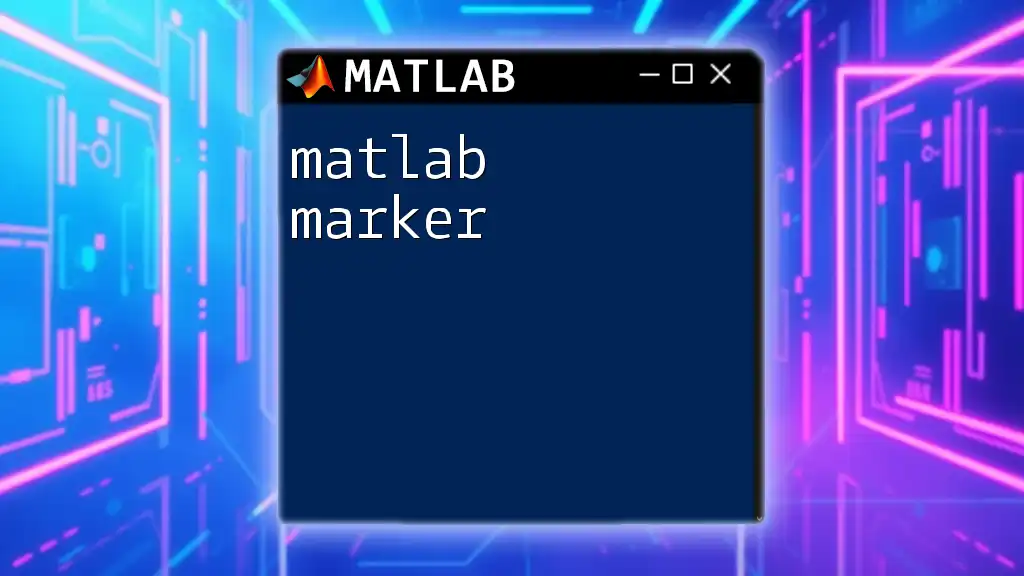
Troubleshooting `parfor`
Common Errors and Solutions
When using `parfor`, you may encounter errors like variable dependency issues. To resolve this, ensure all variables used within the loop are independent or initialized correctly outside the loop.
Using MATLAB’s Diagnostic Tools
MATLAB provides several tools to help diagnose performance issues. You can analyze your parallel code using the Parallel Computing Toolbox Profiler to understand where bottlenecks may occur.
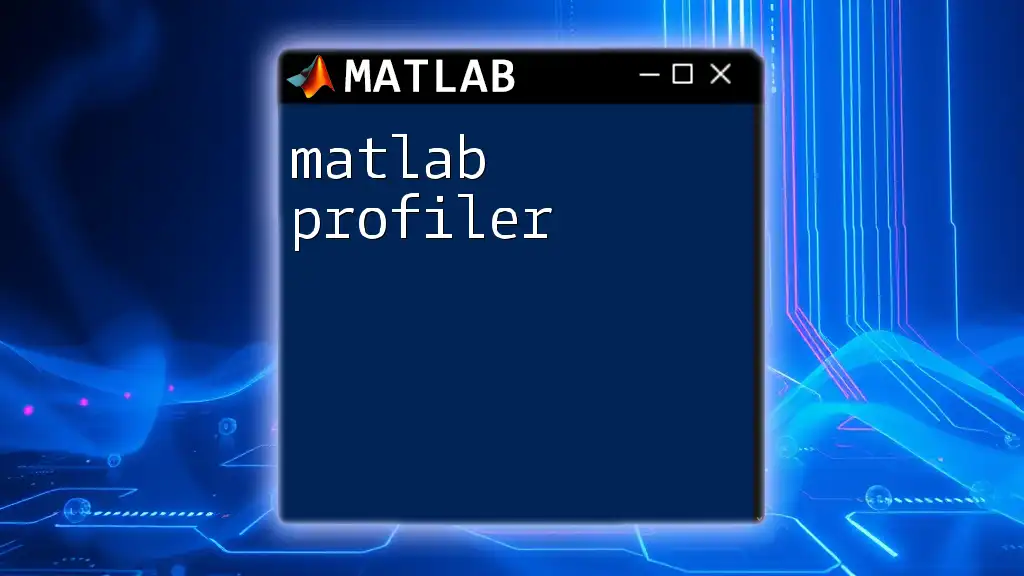
Conclusion
In summary, `parfor` is an invaluable tool for speeding up computations in MATLAB, particularly for large datasets where parallelization is possible. Understanding the structure, limitations, and best practices associated with `parfor` will enable you to optimize your MATLAB code effectively.
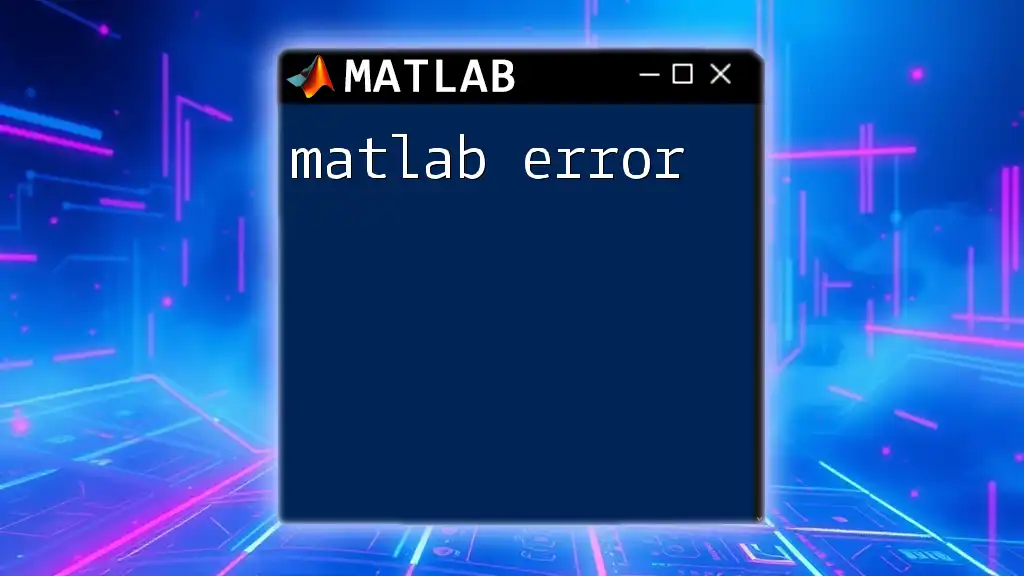
Additional Resources
For more detailed information, refer to the official MATLAB documentation, which offers comprehensive insights and further reading materials related to parallel computing. Additionally, consider enrolling in online courses that delve deeper into advanced topics in parallel computing and `parfor` usage.
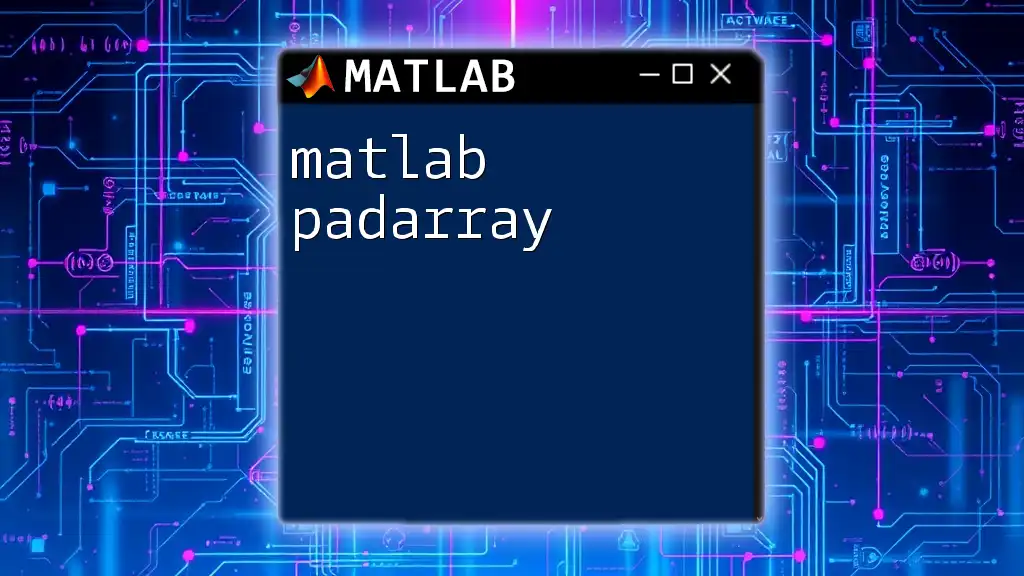
About Us
At our company, we are dedicated to simplifying the learning process for MATLAB users. Through concise, practical lessons, we strive to empower individuals with the skills needed to harness the full potential of MATLAB, including parallel computing with `parfor`. Don’t hesitate to reach out for more resources or any queries you may have!