The `permute` function in MATLAB rearranges the dimensions of an array based on a specified order, allowing for flexible manipulation of multi-dimensional data.
Here’s an example of how to use `permute`:
A = rand(2, 3, 4); % Create a 2x3x4 array
B = permute(A, [2, 1, 3]); % Rearrange dimensions to 3x2x4
Understanding MATLAB Permute
What is Permutation?
In mathematics, a permutation refers to the arrangement of elements in a specific order. It plays a significant role in various fields, including statistics, probability, and data analysis. In MATLAB, the concept of permutation translates to rearranging the dimensions of data arrays, enabling users to manipulate and analyze multidimensional datasets efficiently.
Introduction to MATLAB
MATLAB (Matrix Laboratory) is a high-performance language for technical computing and data visualization. Mastery of MATLAB commands, such as matlab permute, allows users to create more efficient and readable code. This efficiency is particularly crucial when working with complex data structures that require precise operations.
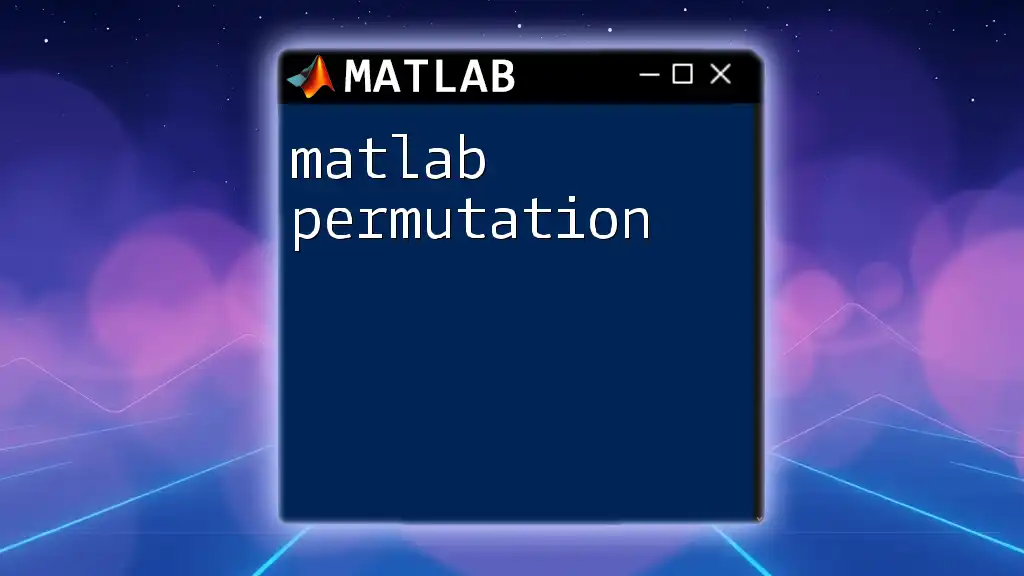
The Permute Function in MATLAB
Syntax of the Permute Function
The basic syntax of the permute function in MATLAB is structured as follows:
B = permute(A, order)
Here, `A` represents the input array, and `order` is a vector that specifies the new order of the dimensions. Understanding this structure is vital for effective use of the function.
How Permute Works
The permute function rearranges the dimensions of an array based on the specified order. This operation does not change the data itself but merely how the data is accessed. For example, if you have a 3D array, changing its order can simplify the processing of specific dimensions for calculations or visualizations.
To visualize this concept, consider a 3D array with dimensions `[x, y, z]`. Using permute, you can transform it to `[z, y, x]`, enabling you to work with data points in a different orientation.
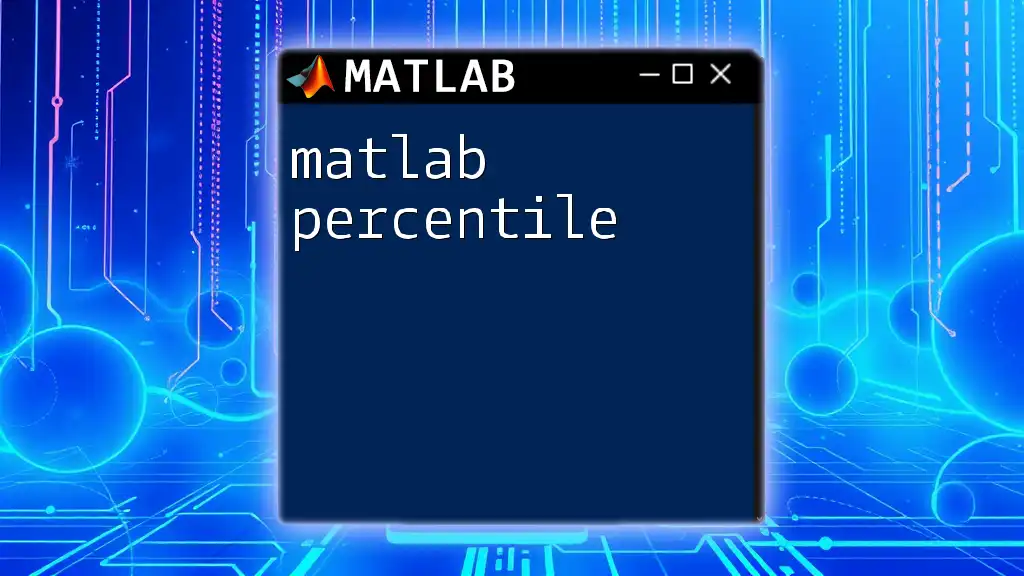
Uses of Permute in MATLAB
Reshaping Multidimensional Arrays
The ability to reshape arrays is essential in data processing. With the permute function, you can rearrange the dimensions of an input array. This is particularly useful when you want to align data with specific requirements for further analysis.
For instance, consider the following example:
A = rand(2, 3, 4); % Creates a 2x3x4 Array
B = permute(A, [2, 1, 3]); % Reshapes it to 3x2x4
Here, the original structure of the array has been altered, allowing for optimized processing and analysis.
Improving Efficiency in Calculations
Permuting your arrays can enhance computational efficiency, especially when dealing with large data sets. Certain calculations may perform better when dimensions are aligned correctly. For example, if you're applying mathematical operations that depend on specific dimensions, permute can save time by minimizing the need for additional reshaping.
Imagine a situation where you analyze large datasets. By rearranging the dimensions to suit your calculations, you can significantly reduce the time and resources required.
Data Analysis and Visualization
MATLAB permute is incredibly useful in preparing data for visualization. Certain plotting functions expect data in specific formats, and permute can rearrange your data accordingly.
Consider the following code snippet that illustrates how to rearrange a 3D array for 3D plotting:
C = rand(10, 10, 5);
D = permute(C, [2, 1, 3]);
This rearrangement allows the `D` array to fit the requirements of a particular visualization function, ensuring that the structure aligns correctly for plotting.
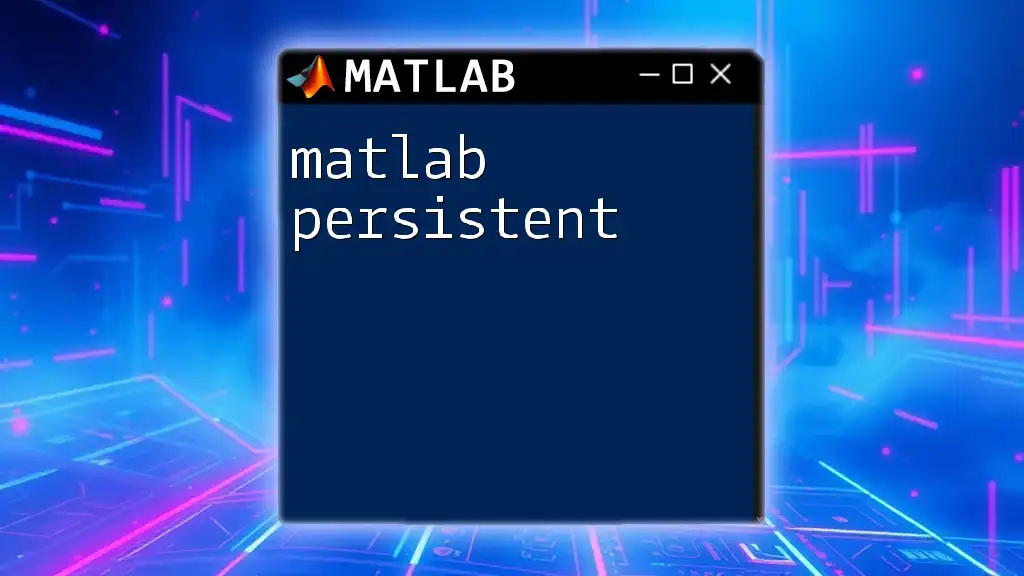
Practical Examples of Permute
Example 1: Simple Matrix Permutation
Let’s explore a simple example involving a 2D matrix:
M = [1, 2; 3, 4];
N = permute(M, [2, 1]); % Swapping rows and columns
In this case, the dimensions of the original matrix have been swapped, providing a new orientation. This rearrangement can be beneficial in data representation and how it aligns with other datasets.
Example 2: 3D Array Manipulation
Working with 3D arrays is common in data analysis. Consider the following snippet:
A = rand(4, 4, 3);
B = permute(A, [3, 1, 2]); % Change order of dimensions
Here, we shifted the third dimension to the front, which might be essential for specific processing tasks or analyses.
Example 3: Complex Data Structures
When engaging with higher-dimensional data, permuting the structure can be crucial for efficient processing. For instance:
A = rand(2, 3, 4, 5);
B = permute(A, [4, 1, 3, 2]); % Changes the data structure significantly
This transformation reorders the dimensions, which could be particularly useful in applications such as image processing or multidimensional analysis.
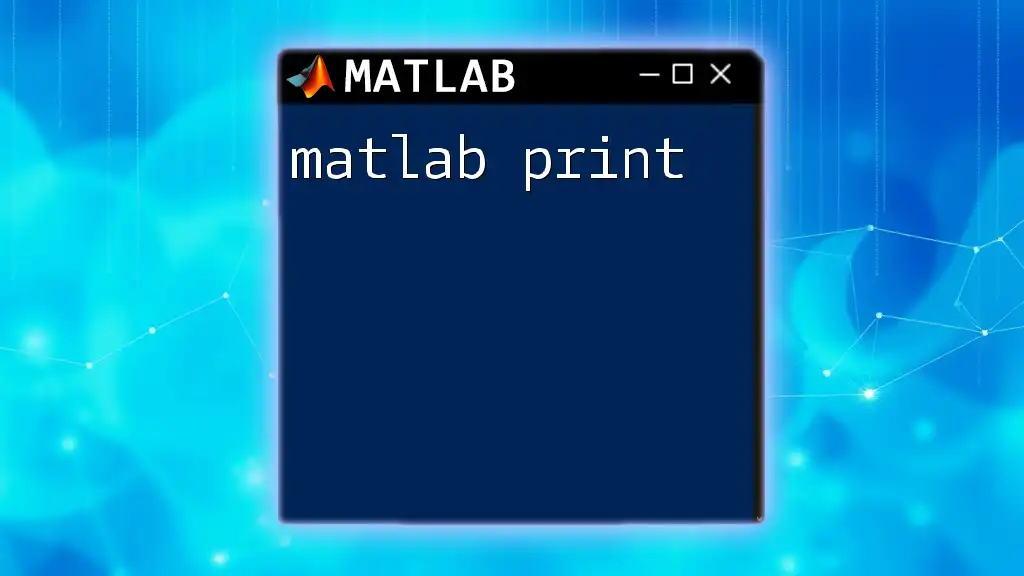
Tips and Best Practices for Using Permute
Common Mistakes to Avoid
When working with the permute function, it's crucial to avoid indexing errors. One common mistake is providing an incorrect order vector that doesn’t correspond to the number of dimensions in your array. Always ensure that the length of the order vector matches the number of dimensions in the input array.
Best Practices for Efficient Coding
To maintain clean and readable code, it’s advisable to use descriptive variable names that indicate what each dimension represents. This practice will help prevent confusion about the purpose of each permutation. Additionally, consider commenting your code generously, especially in complex manipulations, to ensure future maintainability.
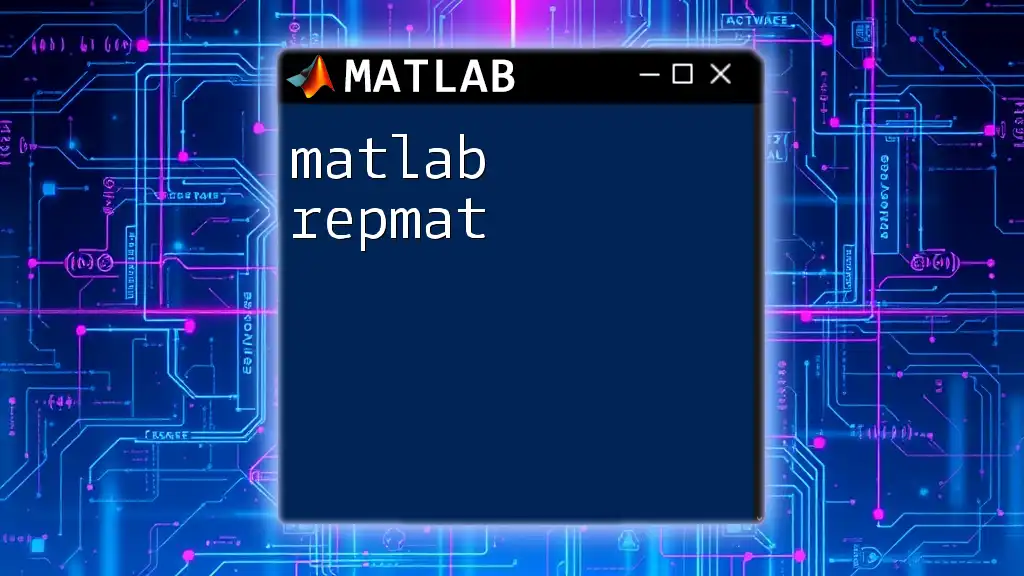
Conclusion
Summary of Key Takeaways
The matlab permute function is a powerful tool for rearranging the dimensions of arrays, enhancing both data analysis and visualization. Understanding its syntax and applying it effectively can streamline your data processing tasks significantly.
Further Resources for Learning
For those looking to deepen their understanding, numerous resources are available, including MATLAB's official documentation, online courses, and community forums. Engaging with these resources can further assist you in mastering MATLAB commands and expanding your coding expertise.
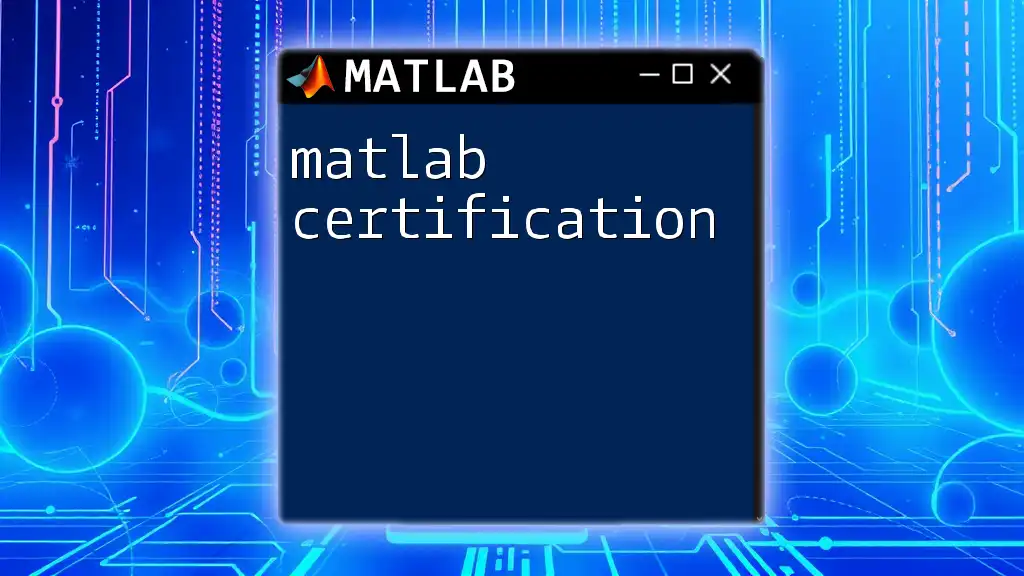
Call to Action
Start Your MATLAB Journey Today!
Experimenting with the permute function is an excellent way to enhance your skills in MATLAB. Consider signing up for courses or tutorials to continue your learning and explore the exciting capabilities that MATLAB has to offer!