In MATLAB, you can customize the appearance of line plots using different line types by specifying a line style character in your plot command.
Here's a code snippet demonstrating various line types:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
plot(x, y1, '--r', x, y2, ':b', 'LineWidth', 2);
xlabel('X-axis');
ylabel('Y-axis');
title('MATLAB Line Types Example');
legend('Sine', 'Cosine');
grid on;
Understanding Linetypes in MATLAB
Definition of Linetypes
In MATLAB, linetypes refer to the style of lines used to represent data in plots. Each linetype can greatly enhance the clarity of your visual offerings, allowing for immediate differentiation between various data sets. Utilizing different linetypes is especially crucial when presenting multiple datasets, ensuring that viewers can easily distinguish between them without confusion.
Default Linetypes in MATLAB
Before diving into the various linetypes available, it’s essential to recognize the default linetypes MATLAB provides. By default, MATLAB assigns a series of specific colors and styles when you plot multiple datasets. Understanding these presets can guide you in choosing appropriate styles that align with your visual preferences.
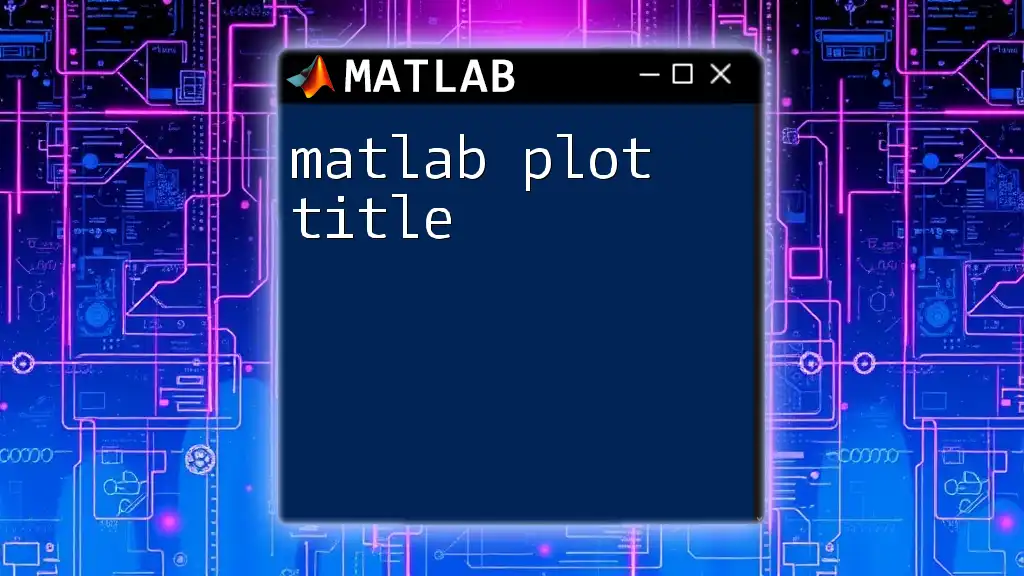
Types of Linetypes in MATLAB
Solid Linetype
The solid linetype is perhaps the most familiar and frequently used style in MATLAB. It's represented by the `'-'` symbol. This linetype is ideal for clear data representation.
Example:
x = 0:0.1:10;
y = sin(x);
plot(x, y, 'k-'); % 'k-' indicates a solid black line
title('Solid Line Example');
xlabel('X-axis');
ylabel('Y-axis');
In this example, we generate a sine wave with a solid black line. Solid lines are often favored for presenting single datasets clearly and distinctly.
Dashed Linetype
To represent trends that are interrupted or to compare datasets with less emphasis, you might opt for a dashed linetype. This is indicated with the `'--'` symbol. Using dashed lines can signify variability and infer less certainty in your data.
Example:
plot(x, y, 'r--'); % 'r--' indicates a dashed red line
title('Dashed Line Example');
xlabel('X-axis');
ylabel('Y-axis');
Here, the sine function is plotted with a dashed red line, ideal for showing a secondary dataset that you want to contrast against a primary plot.
Dotted Linetype
The dotted linetype is another valuable option in your MATLAB toolkit. Represented by the `':'` symbol, dotted lines work best in situations where subtlety is required or when representing guidelines or references.
Example:
plot(x, y, 'g:.'); % 'g:.' indicates a dotted green line
title('Dotted Line Example');
xlabel('X-axis');
ylabel('Y-axis');
In this example, the sine wave is displayed with a dotted green line, useful for stylistic charts or when indicating auxiliary data.
Dash-Dot Linetype
The combination of dashes and dots results in the dash-dot linetype, represented by `'-.'`. This linetype is useful when distinguishing between datasets that share similarities but need to remain identifiable at a glance.
Example:
plot(x, y, 'b-.'); % 'b-.' indicates a blue dash-dot line
title('Dash-Dot Line Example');
xlabel('X-axis');
ylabel('Y-axis');
As showcased, we can clearly present two datasets, making their comparative analysis straightforward.
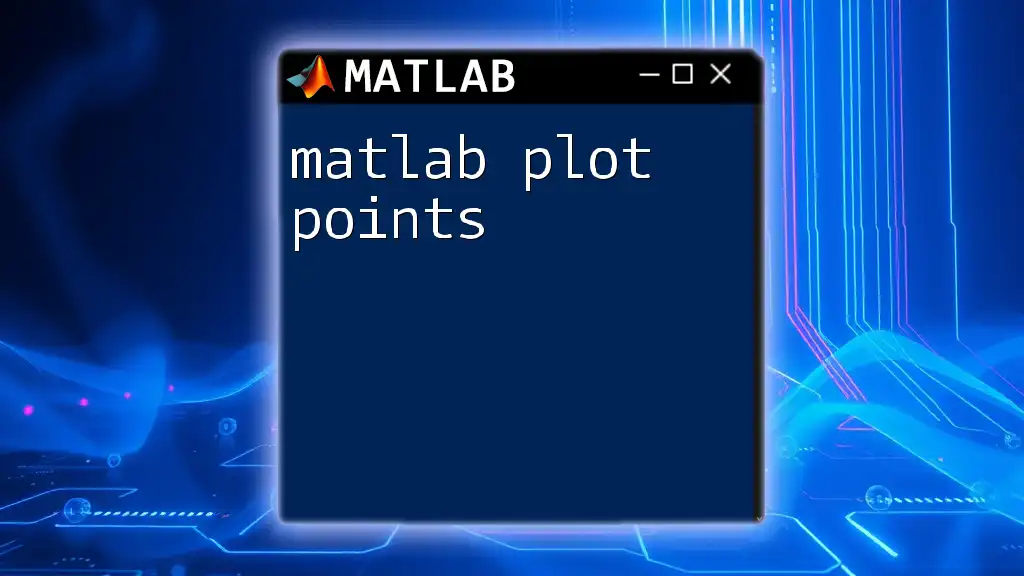
Customizing Linetypes
Combining Linetypes
MATLAB allows you to enhance your plots by incorporating multiple linetypes in a single figure. This can facilitate comparative assessment across various datasets.
Example:
y2 = cos(x);
plot(x, y, 'k-', x, y2, 'r--'); % Both a solid and a dashed line in one plot
title('Combining Linetypes');
xlabel('X-axis');
ylabel('Y-axis');
legend('sin(x)', 'cos(x)');
In this example, both sine and cosine functions are displayed together—one with a solid black line and the other with a dashed red line. This visual distinction aids the viewer in grasping the differences between datasets rapidly.
Changing Linetype Properties
MATLAB offers the flexibility to modify linetypes programmatically. This can streamline adjustments and ensure consistency across visualizations.
Example:
h = plot(x, y);
set(h, 'LineStyle', '--', 'Color', 'm'); % Change to magenta dashed line
title('Changing Linetype Properties');
xlabel('X-axis');
ylabel('Y-axis');
In this scenario, we change the previously drawn sine wave to a magenta dashed line programmatically. Adjusting line properties through code ensures greater control over visual aspects without causing redundancy in code.
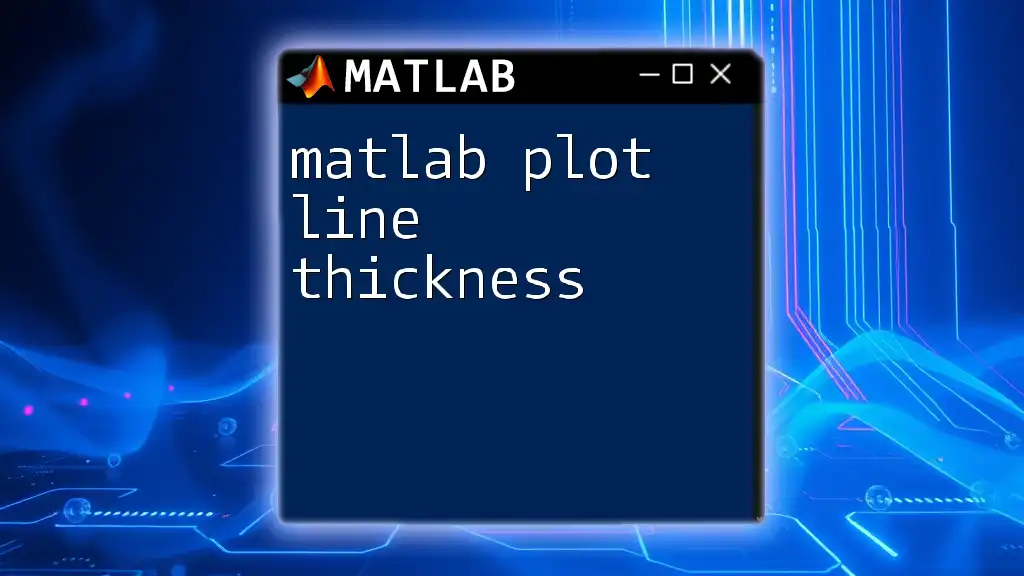
Advanced Tips for Linetype Usage
Using Linetypes in Multiple Plotting Functions
When creating multiple subplots, choosing the appropriate linetypes becomes even more vital. Keeping a consistent style among similar data can enhance overall interpretability.
Example:
subplot(1, 2, 1);
plot(x, y, 'k-')
title('Solid Line in Subplot');
subplot(1, 2, 2);
plot(x, y2, 'r--')
title('Dashed Line in Subplot');
With this layout, the first subplot displays a solid line indicating the sine function while the second showcases the dashed line for the cosine function. This format allows for direct comparison while maintaining distinct visual cues.
Interactive Customization with Plot Tools
In addition to visualizing data through code, MATLAB enables interactive customization of plot properties via its GUI tools. By using the properties editor in the figure window, you can adjust linetypes, colors, and other characteristics dynamically. This is especially beneficial for those who may prefer visual adjustments over coding, allowing for immediate feedback on changes.
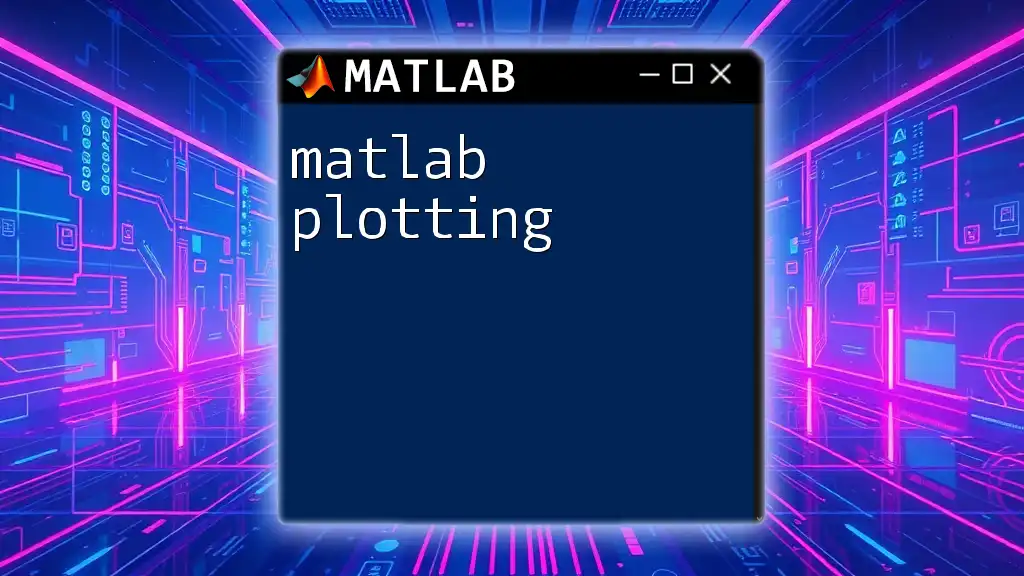
Conclusion
In this comprehensive guide on MATLAB plot linetypes, we explored a variety of styles, their coding commands, and how each contributes to effective data representation. Whether you are comparing trends, highlighting variations, or aligning multiple datasets side by side, understanding and utilizing linetypes will significantly boost the clarity of your visual output. I encourage you to experiment with these options and infuse creativity into your data presentations for maximum impact.
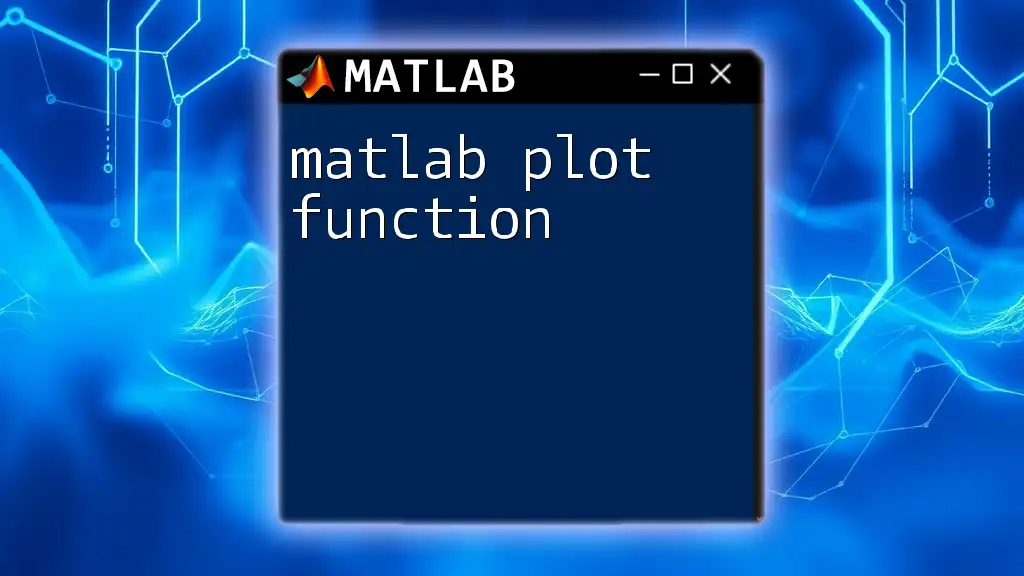
References
For additional information on linetypes and advanced plotting techniques, refer to the [MATLAB documentation](https://www.mathworks.com/help/matlab/ref/plot.html) and further resources available online.