To plot a circle in MATLAB, you can use the `rectangle` function with the 'Curvature' property set to [1, 1] to create a circle shape.
rectangle('Position',[x_center - radius, y_center - radius, 2*radius, 2*radius],'Curvature',[1, 1],'EdgeColor','b');
Replace `x_center`, `y_center`, and `radius` with your desired coordinates and radius values.
Understanding the Basics of MATLAB Plotting
What is MATLAB?
MATLAB is a high-performance language for technical computing. It integrates computation, visualization, and programming in an easy-to-use environment. One of the key functionalities of MATLAB is its powerful plotting capabilities which allow users to visualize data and mathematical functions effectively. Understanding how to plot shapes like circles is a fundamental skill that lays the groundwork for more complex visualizations.
Basic Plotting Commands in MATLAB
To get started with plotting in MATLAB, you should be familiar with some basic commands. The most important command for plotting is `plot()`, which is used to create a 2D line plot. Other commands such as `hold on`, `hold off`, and `axis` will help you manage the plots you create.
- `hold on`: This command allows you to add multiple plots to the same figure without replacing existing ones.
- `axis`: This command is crucial for setting the limits and aspect ratio of your plot.
These commands form the building blocks of any visualization process in MATLAB.
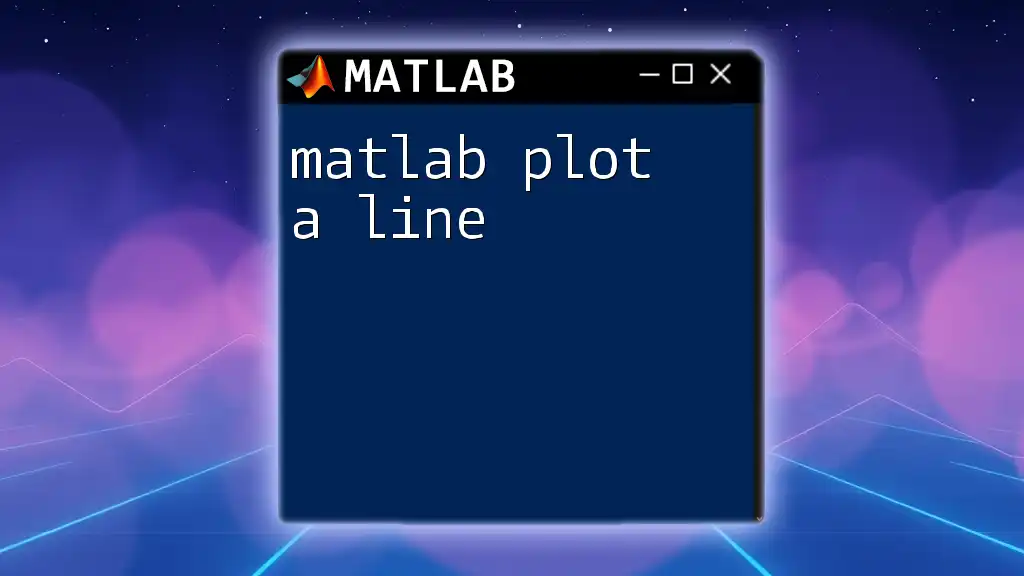
Mathematical Foundations of a Circle
The Equation of a Circle
Before we dive into the plotting process, it's essential to understand the mathematical representation of a circle. The standard equation of a circle is given by:
\[ (x - h)^2 + (y - k)^2 = r^2 \]
In this equation:
- h is the x-coordinate of the center of the circle.
- k is the y-coordinate of the center of the circle.
- r is the radius of the circle.
Understanding this equation will help you manipulate the circle's properties when plotting in MATLAB.
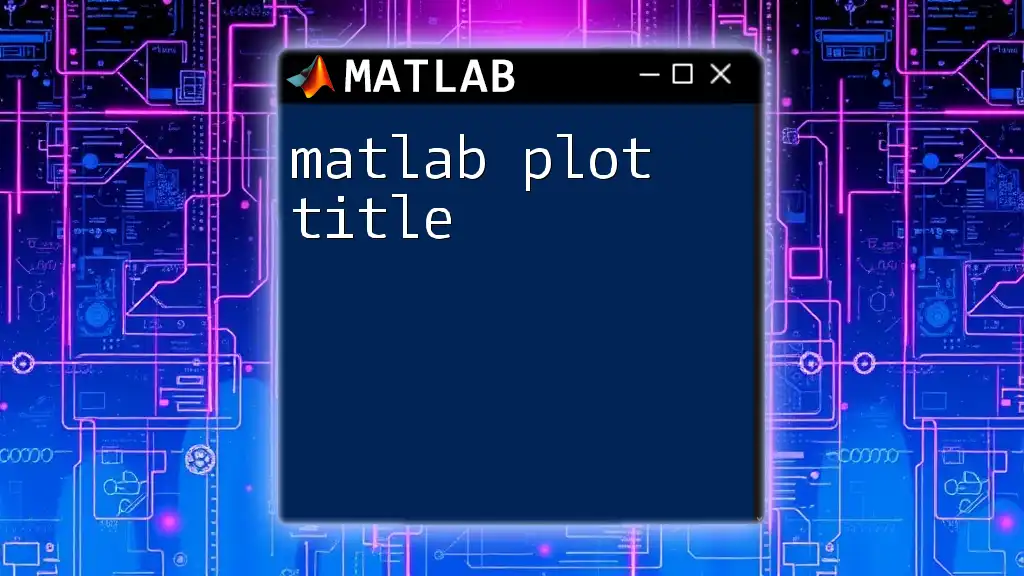
Plotting a Circle in MATLAB
Using the Parametric Equations
To plot a circle in MATLAB, we often use parametric equations, which express the circle's coordinates in terms of an angle \(\theta\). The parametric equations for a circle are defined as follows:
- \(x = h + r \cdot \cos(\theta)\)
- \(y = k + r \cdot \sin(\theta)\)
Here, \(\theta\) varies from \(0\) to \(2\pi\) to complete a full circle.
Example Code: Basic Circle Plot
To plot a circle in MATLAB, you can use the following example code:
% Define the center and radius
h = 0; % X-coordinate of the center
k = 0; % Y-coordinate of the center
r = 1; % Radius of the circle
% Create theta values
theta = linspace(0, 2*pi, 100);
% Parametric equations for x and y
x = h + r * cos(theta);
y = k + r * sin(theta);
% Plot the circle
figure; % Create a new figure
plot(x, y, 'b-', 'LineWidth', 2); % Plot circle
axis equal; % Equal scale on both axes
grid on; % Add grid
title('Circle with Radius 1'); % Title
xlabel('X-axis'); % X label
ylabel('Y-axis'); % Y label
This code breaks down as follows:
- We define \(h\), \(k\), and \(r\), specifying the center and radius.
- The `linspace` function generates \(100\) points between \(0\) and \(2\pi\) for \(\theta\).
- The parametric equations calculate the \(x\) and \(y\) coordinates based on the center and radius.
- Finally, we use the `plot()` function to visualize the circle.
Adjusting Circle Parameters
Changing the Center
To modify the center of the circle, simply adjust the values of \(h\) and \(k\). For instance, to center the circle at \((2,3)\), you can update the code as follows:
% Define new center
h = 2;
k = 3;
Making this change will translate the circle to the new coordinates.
Modifying the Radius
Changing the radius \(r\) will resize the circle. For instance, if you want a circle with a radius of \(3\), simply adjust the definition of \(r\):
% Define new radius
r = 3;
This alteration will graph a larger circle while keeping the center at its defined coordinates.
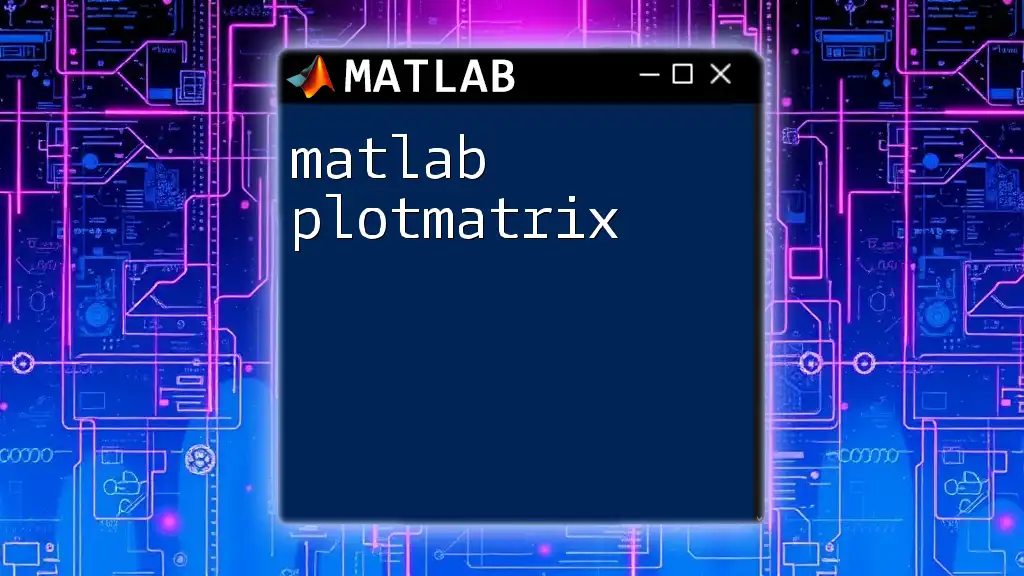
Plotting Multiple Circles
Using a Loop to Create Multiple Circles
Sometimes, you may want to visualize multiple circles in one plot. You can achieve this using a loop to iterate through different centers and radii. The following example code demonstrates how to create several circles at different positions:
% Define an array of centers and radii
centers = [0, 0; 2, 2; -2, -1];
radii = [1, 2, 1.5];
% Loop through centers and radii to plot circles
figure;
hold on; % Hold the current plot
for i = 1:size(centers, 1)
x = centers(i, 1) + radii(i) * cos(theta);
y = centers(i, 2) + radii(i) * sin(theta);
plot(x, y); % Plot each circle
end
axis equal; % Equal scale for all axes
grid on;
title('Multiple Circles');
xlabel('X-axis');
ylabel('Y-axis');
hold off; % Release hold
In this code:
- An array of center coordinates and corresponding radii is specified.
- A loop iterates through each center and radius, plotting each circle with the defined parameters.
- The `hold on` command ensures that all circles are displayed in the same figure.
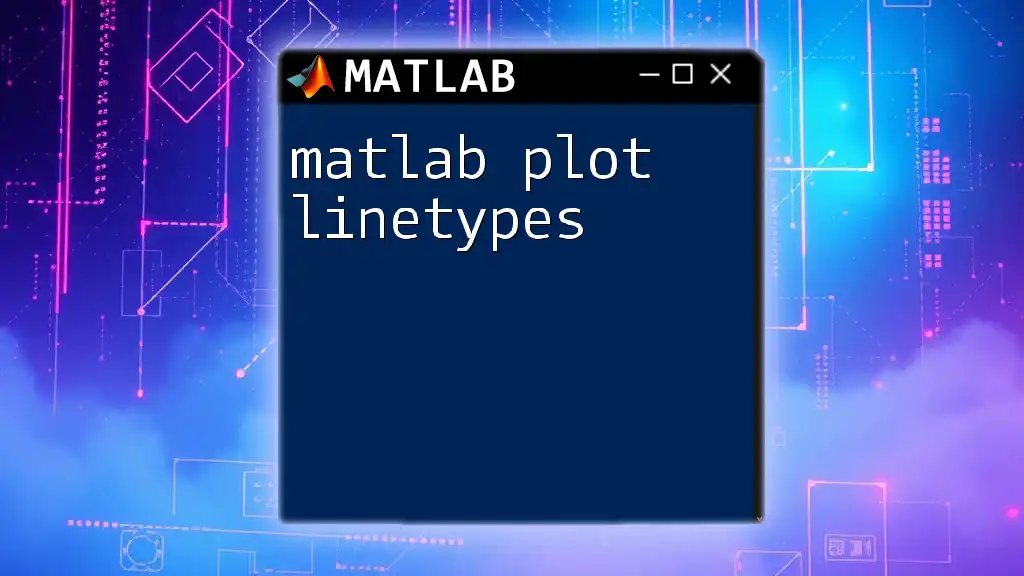
Customizing Your Circle Plot
Changing Line Styles and Colors
MATLAB allows you to customize the appearance of your circles using different styles and colors. You can specify parameters like line width and color in the `plot()` function. For instance, you could modify a circle's appearance like this:
plot(x, y, 'r--', 'LineWidth', 2); % Dashed red line
This would change the circle to a dashed red line while keeping the line width for better visibility.
Adding Labels and Legends
To make your plots more informative, you can add labels and a legend to identify different circles when plotting multiple shapes. For example, you might use:
legend('Circle 1', 'Circle 2', 'Circle 3'); % Adding legends
This simple command enhances the plot's usability and clarity by indicating what each circle represents.
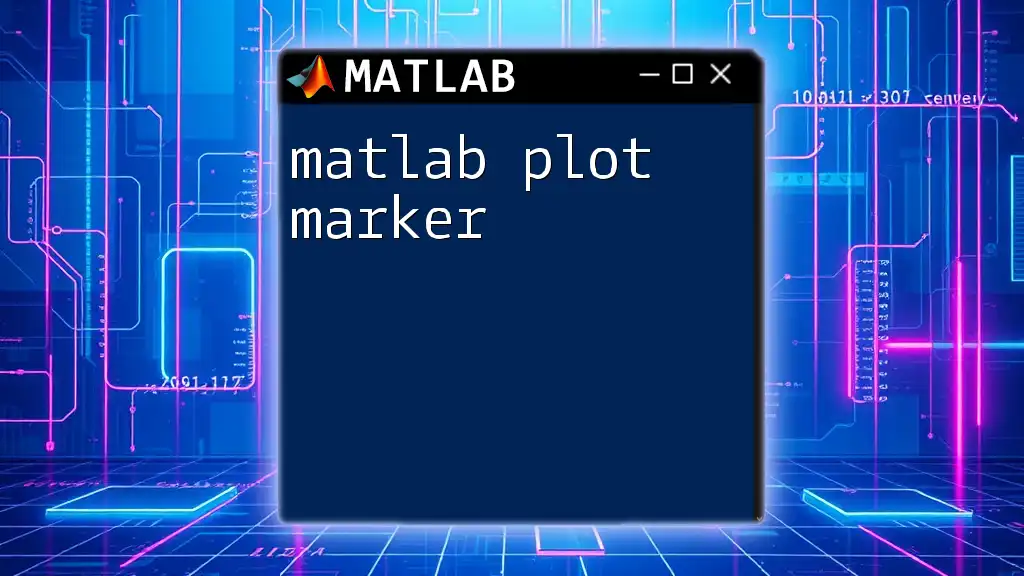
Conclusion
In this comprehensive guide, we explored the process of how to MATLAB plot a circle. From basic equations to plotting multiple circles, we covered essential techniques and coding practices to build your plotting skills. The ability to visualize mathematical functions like circles is foundational for creating more complex plots in MATLAB.
Feel free to experiment with the parameters of your circles, utilize customization options, and challenge yourself with more complicated plotting tasks. Being part of a community that shares knowledge and tips can also enhance your learning experience, so consider joining our MATLAB community for ongoing support and inspiration.
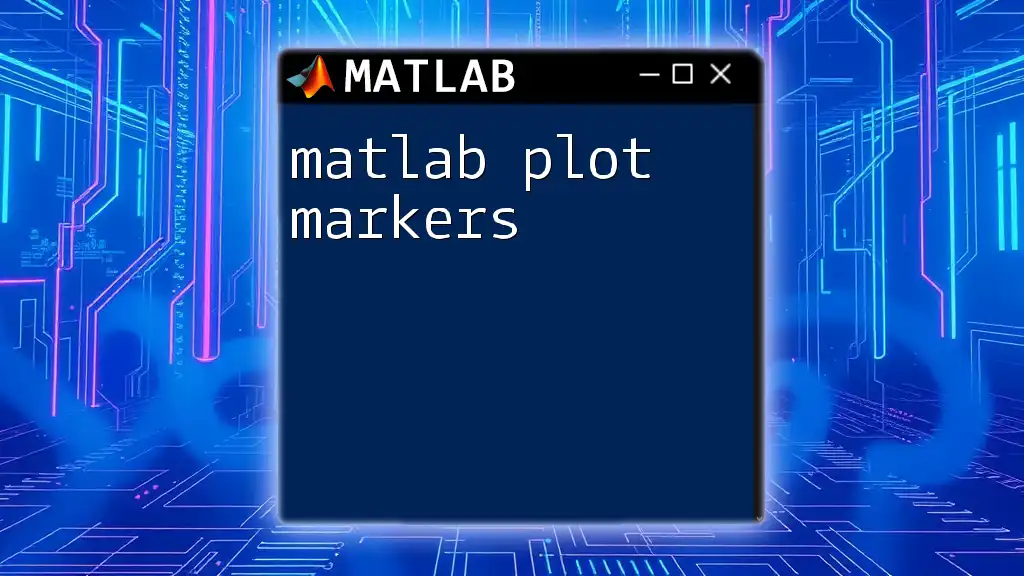
Additional Resources
MATLAB Documentation
For a deeper dive into MATLAB plotting functions, refer to the [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/plot.html).
Recommended Tutorials
Explore online tutorials and courses that focus on MATLAB plotting for further learning.
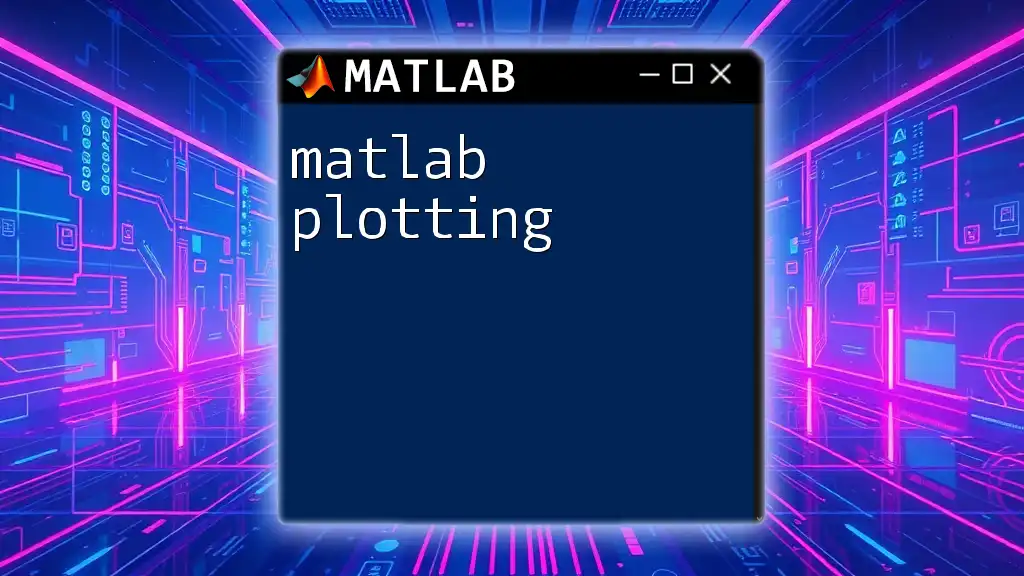
FAQs
Common Questions About Plotting Circles in MATLAB
One common question is: "Why is my circle not appearing as expected?" This issue could arise from mismatched axes limits or improper parameter definitions. Always ensure you're using the `axis equal` command to maintain the aspect ratio.