To plot a line in MATLAB, you can use the `plot` function along with the x and y coordinate vectors to create a simple 2D line graph.
Here’s a code snippet to demonstrate:
x = 0:0.1:10; % Define x values from 0 to 10 with an increment of 0.1
y = sin(x); % Compute y values as the sine of x
plot(x, y); % Plot the line graph of y vs. x
xlabel('X-axis'); % Label for the x-axis
ylabel('Y-axis'); % Label for the y-axis
title('Line Plot of Sine Function'); % Title of the plot
grid on; % Turn on the grid for better visualization
Understanding MATLAB Plotting Functions
What is a Plot?
A plot is a graphical representation of data that allows users to visualize relationships between variables. In the context of MATLAB, plots are crucial for analyzing data trends, patterns, and distributions. MATLAB offers various types of plots, including line plots, scatter plots, bar graphs, and surface plots, each serving different analytical purposes.
Why Use Plotting Functions?
Utilizing built-in plotting functions in MATLAB streamlines the process of visualizing data, making it less error-prone and much faster than creating plots from scratch. These functions are well-optimized for performance and designed to handle a diverse range of data visualization needs. By employing MATLAB's plotting functions, you can quickly create effective visualizations that aid in understanding your data better.
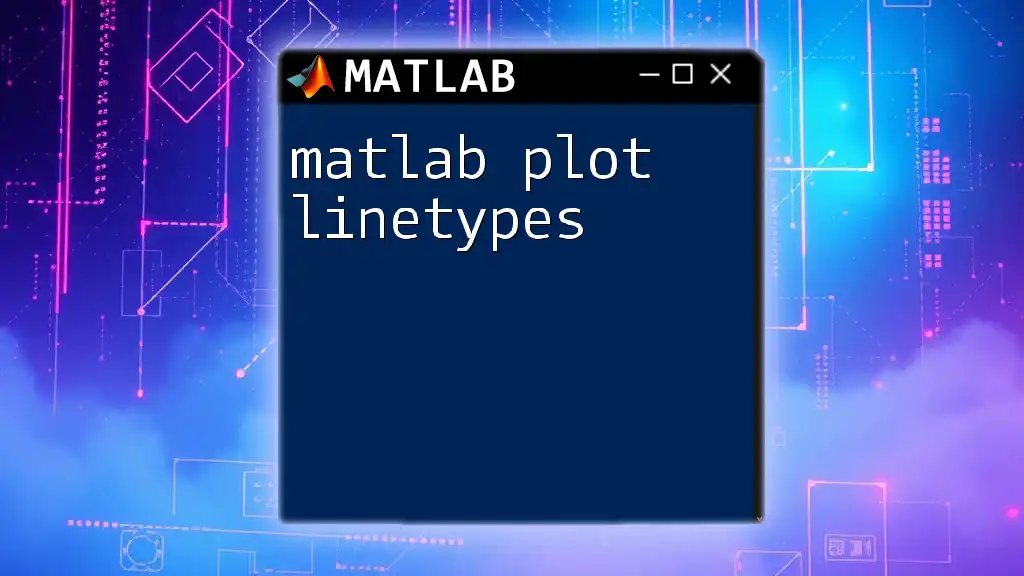
Getting Started with Line Plots in MATLAB
Basic Syntax of the `plot` Command
The fundamental command for creating line plots in MATLAB is the `plot` function. The basic syntax is:
plot(X, Y)
Where:
- X is a vector containing x-coordinates.
- Y is a vector containing corresponding y-coordinates.
This simple command draws a continuous line between given (x, y) points.
Example of Basic Line Plot
Let's visualize a simple mathematical function. Here's how to plot the sine wave:
x = 0:0.1:10; % Create a vector of x values from 0 to 10, incrementing by 0.1
y = sin(x); % Compute the sine of each x value
plot(x, y); % Plot the values
title('Sine Function'); % Add a title
xlabel('X-axis'); % Label x-axis
ylabel('Y-axis'); % Label y-axis
grid on; % Turn on the grid for better visibility
In this example, `x` is defined as a range from 0 to 10, and `y` is the sine of `x`. The resulting plot displays a smooth sine wave, which highlights the importance of using concise commands to depict complex functions.
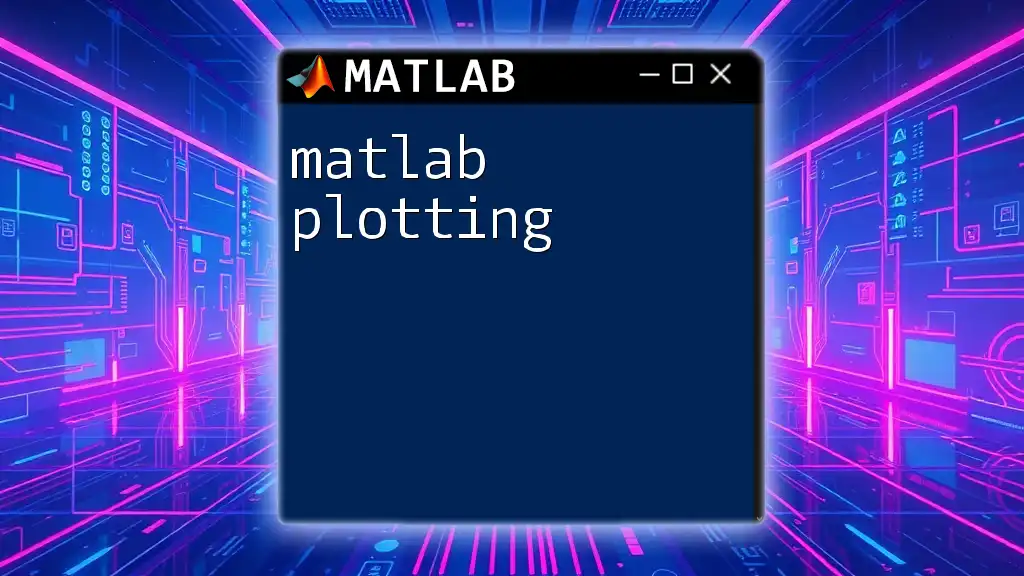
Customizing Your Line Plot
Changing Line Properties
To enhance the visual appeal and informational aspect of your plots, you can customize line properties.
Line Style
Line styles can be varied to differentiate between data series. MATLAB supports several styles, such as solid, dashed, and dotted. For example:
plot(x, y, '--r'); % Creates a red dashed line
Here, the line is rendered in red and dashed format, instantly distinguishing it from other lines.
Line Width
The line width can significantly affect readability. Thicker lines often stand out more prominently. You can specify line width as follows:
plot(x, y, 'LineWidth', 2); % Uses a line width of 2 units
Adjusting the line width can be particularly useful when presenting data, as it ensures clarity in visuals.
Marker Types
Markers highlight specific data points on the line. Common markers include circles, squares, and stars. For instance:
plot(x, y, 'o'); % This adds circle markers at each data point
This addition provides visual context and tangible data representation.
Adding Titles and Labels
Titles and axis labels are crucial for providing context. They help viewers interpret what they see clearly. For effective labeling, do the following:
title('Custom Title'); % Replace with a descriptive title
xlabel('Custom X-axis'); % Replace with specifics relevant to your data
ylabel('Custom Y-axis'); % Do the same for y-axis
When crafting titles and labels, aim for clarity and specificity, ensuring that viewers can grasp what the plot represents at a glance.
Adding a Legend
When plotting multiple lines, legends help distinguish each data series. Here's how to implement legends effectively:
plot(x, sin(x), 'r', x, cos(x), 'b'); % Plotting sine in red and cosine in blue
legend('sin(x)', 'cos(x)'); % Adding a legend to explain the colors
Placing legends appropriately enhances understanding, especially in complex plots.
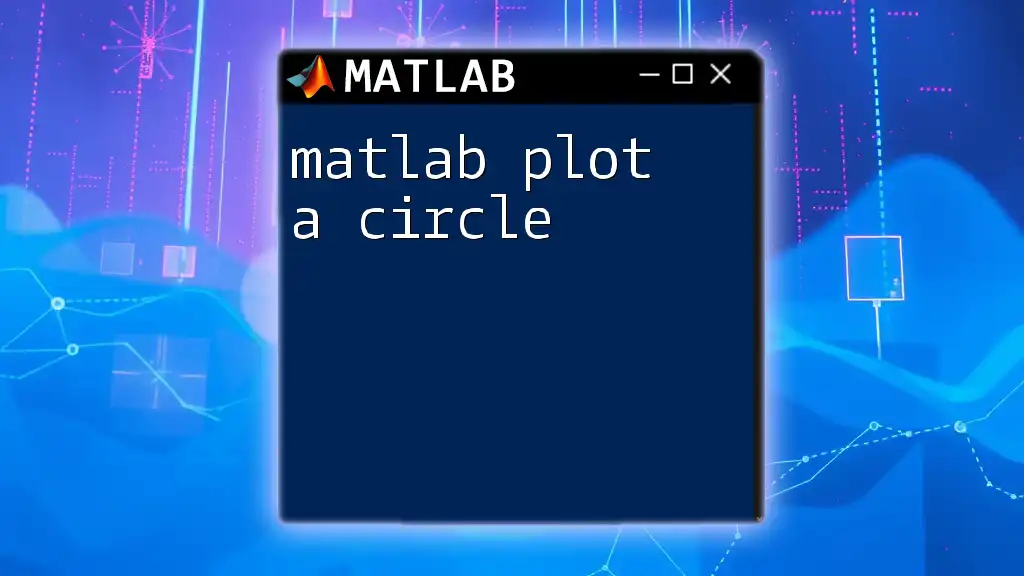
Enhancing Your Plot with Additional Features
Grid and Axes Control
Grids enhance readability by providing reference lines. Enable grids with:
grid on; % This turns on the major grid lines
grid minor; % This adds minor grid lines for better accuracy
Utilizing grid lines can significantly improve the readability of plots, especially when dealing with precise data points.
Setting Axis Limits
To provide a clearer view of your data, it can be beneficial to set axis limits. This allows you to zoom into key areas of interest:
xlim([0, 10]); % Set x-axis limit from 0 to 10
ylim([-1.5, 1.5]); % Set y-axis limit to focus on specific ranges
Adjusting axis limits will help in viewing data distributions more clearly, ensuring viewers focus on the relevant parts of the plot.
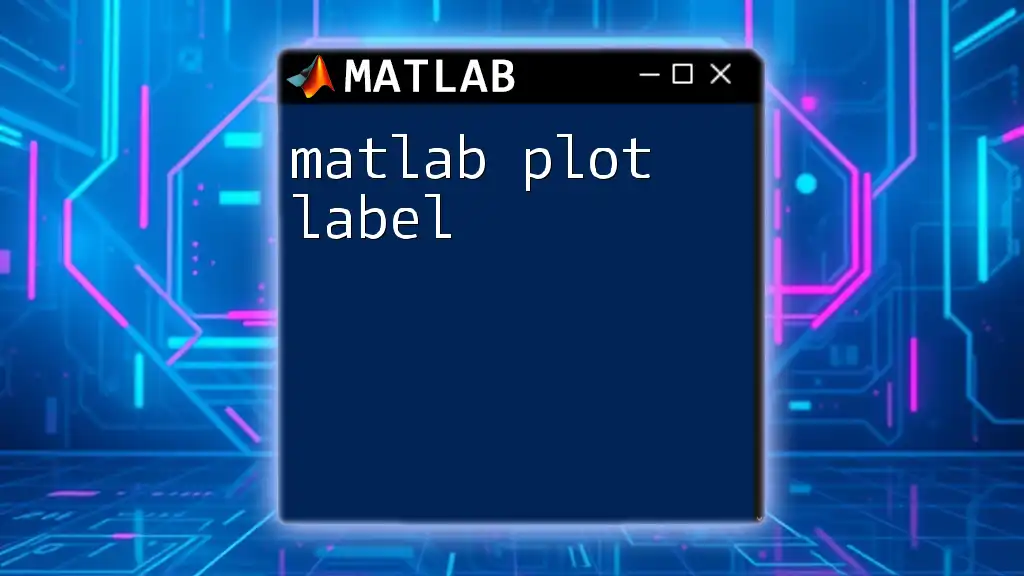
Advanced Plotting Techniques
Subplots: Multiple Plots in One Figure
Subplots allow the simultaneous representation of multiple datasets in one figure, which is particularly useful for comparison. For example:
subplot(2, 1, 1); % Create a 2-row, 1-column grid and plot in the first row
plot(x, sin(x));
title('Sine Function');
subplot(2, 1, 2); % Plot in the second row
plot(x, cos(x));
title('Cosine Function');
This approach provides a compact way to view different datasets together, facilitating easier comparison.
Exporting Your Plots
After creating your visualizations, saving them for future use is essential. You can export figures easily with:
saveas(gcf, 'myPlot.png'); % Saves the current figure as a PNG file
MATLAB supports various formats, including PNG, JPG, and PDF. Exporting your work preserves your visualizations for reports, presentations, or further analysis.
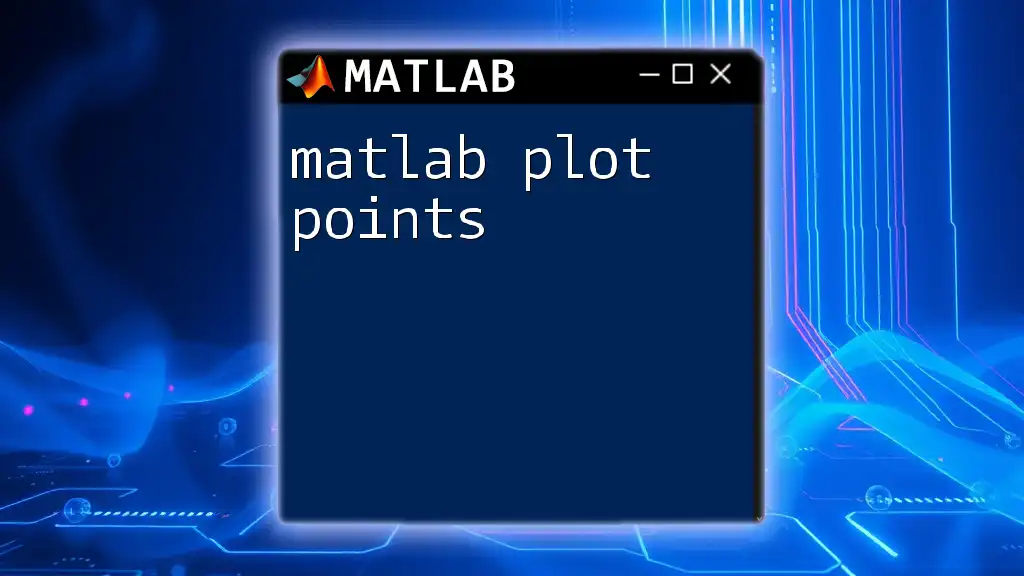
Conclusion
This comprehensive guide has walked you through the essentials of how to matlab plot a line effectively. From basic commands to advanced customization and exporting techniques, you now have the tools to create compelling visualizations in MATLAB. Experiment with these features and enhance your plotting skills to better convey data insights.
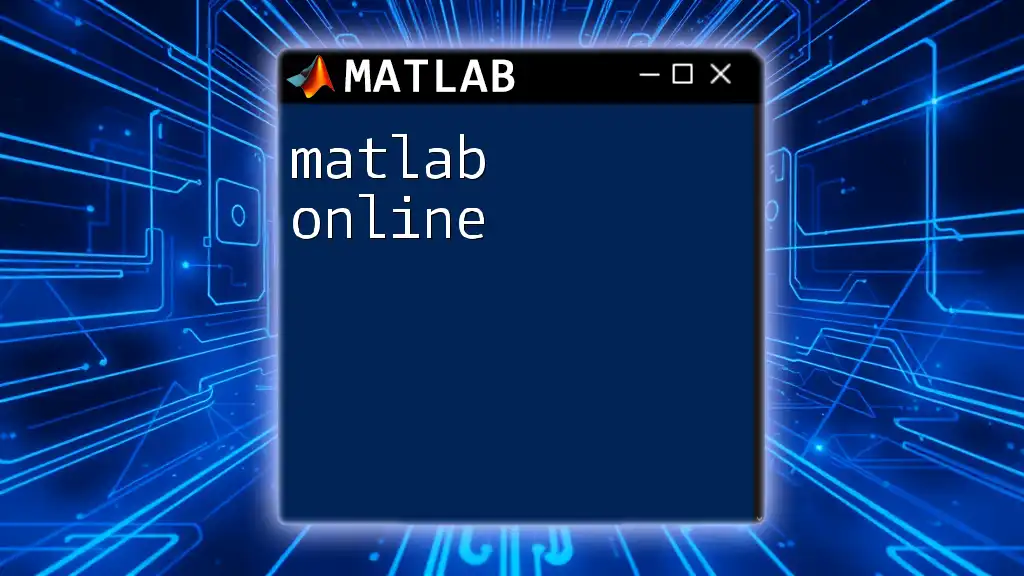
Additional Resources
For further exploration, consider checking MATLAB's official documentation on plotting functions and engaging with online tutorials or courses that delve deeper into data visualization with MATLAB.
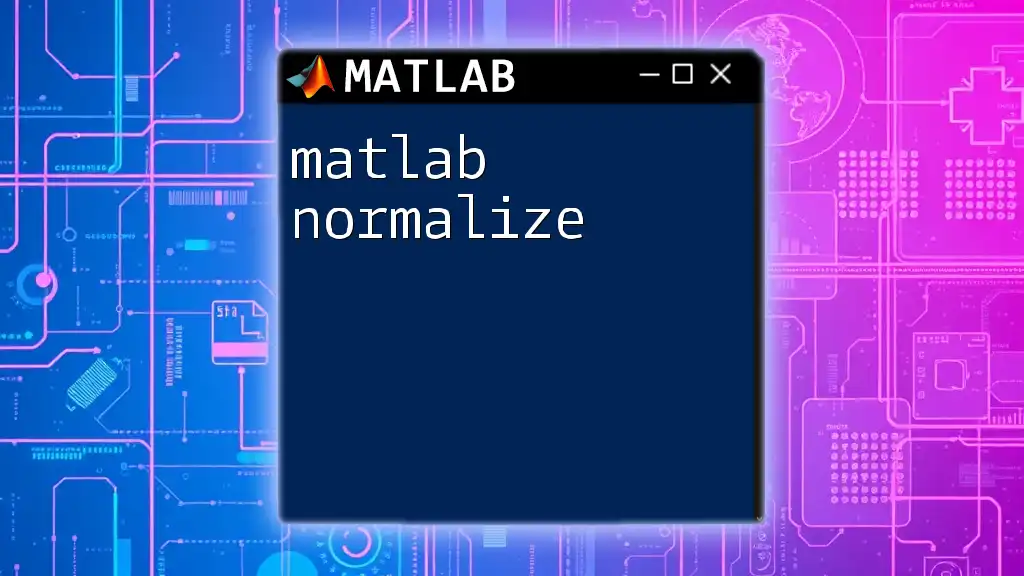
Call to Action
We'd love to hear about your experiences with MATLAB plotting! Please share your thoughts in the comments and consider signing up for our newsletters to stay updated on more exciting MATLAB tutorials and tips.