To plot points in MATLAB, you can use the `plot` function to create a simple scatter plot of your specified x and y coordinates. Here's a quick example:
x = [1, 2, 3, 4, 5]; % x-coordinates
y = [2, 3, 5, 7, 11]; % y-coordinates
plot(x, y, 'o'); % Plot points as circles
title('Plot of Points');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
Understanding MATLAB Graphics Environment
What is MATLAB Graphics?
MATLAB's graphics system is a powerful tool for data visualization, allowing users to create a diverse range of plots and charts. At its core, the graphics environment consists of three main components: figures, axes, and data. The figure acts as a container for your graphs, while axes define the coordinate system in which your data points are plotted. Finally, the data consists of the actual values that you will visualize.
Types of Plots in MATLAB
Choosing the right type of plot is essential for effectively conveying your data's message. MATLAB supports various plot types, including 2D line plots, scatter plots, bar graphs, and 3D surface plots. Each type serves a unique purpose, so understanding them is crucial for effective data analysis.
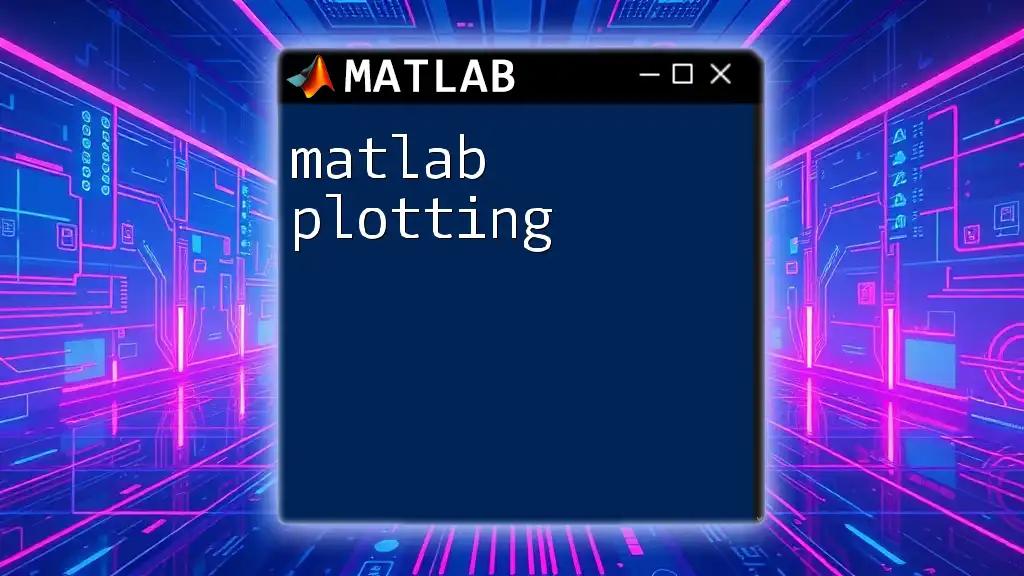
Basic Plotting of Points in MATLAB
Creating a Simple 2D Plot
To get started with plotting points in MATLAB, the `plot()` function is your best friend. This basic function allows you to create a simple 2D plot that can be customized to suit your needs.
Here is an example demonstrating how to plot points:
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
plot(x, y, 'o'); % 'o' to indicate points
title('Simple 2D Plot of Points');
xlabel('X-axis Label');
ylabel('Y-axis Label');
grid on;
In this code snippet, we define two vectors, `x` and `y`, which contain our data points. The `plot` function then visualizes these points with circles ('o') as the marker style. Adding titles and labels enhances the graph's readability and provides context for the viewer.
Customizing Point Markers
MATLAB allows for extensive customization of point markers. You can change the shape, size, and color of the markers to make your plots more informative and visually appealing.
For instance, if you want to create red star markers, you can use the following code:
plot(x, y, 'r*', 'MarkerSize', 10); % Red star markers
Here, `'r*'` specifies the use of red star markers, with the size set to 10, ensuring they stand out. Customizing your markers can help provide clarity, especially when dealing with multiple datasets.

Plotting Points in 3D
Introduction to 3D Plotting
3D plotting is particularly useful when analyzing datasets with three variables. MATLAB makes it easy to create 3D plots using the `plot3()` function, allowing viewers to understand complex relationships between three variables.
Example of 3D Point Plotting
To visualize points in a 3D space, you can use the following example:
z = [1, 4, 9, 16, 25];
plot3(x, y, z, 'b^'); % Blue triangle markers
title('3D Plot of Points');
xlabel('X-axis Label');
ylabel('Y-axis Label');
zlabel('Z-axis Label');
grid on;
In this example, we define a third vector, `z`, representing the third dimension. We utilize the `plot3()` function to create a 3D plot with triangle markers in blue ('b^'). Incorporating axis labels is critical in a 3D plot to ensure that viewers can interpret the data accurately.
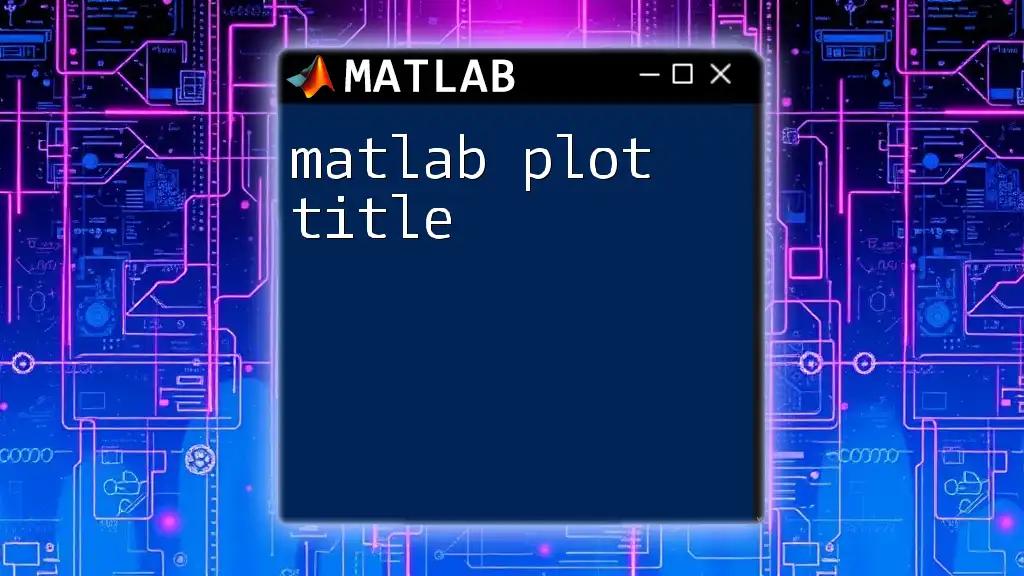
Advanced Plotting Techniques
Using Scatter Plots for Enhanced Visualization
Scatter plots offer a powerful alternative to standard line plots, particularly when dealing with large datasets. The `scatter()` function provides a more flexible way to display data, allowing for variable point sizes and colors.
Here’s how you can create a scatter plot:
scatter(x, y, 100, 'filled', 'MarkerFaceColor', 'g'); % Green filled circles
title('Scatter Plot of Points');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
In this code, the `scatter()` function is utilized to create a plot where each point is represented as a filled green circle with a size of 100. Scatter plots allow for visual representation of individual data points, making distinctions between them clearer.
Adding Annotations and Customizing Visuals
Annotations can significantly enhance the interpretability of your plots. Adding text to specific points provides context and important information.
You can annotate points using the following code snippet:
for i = 1:length(x)
text(x(i), y(i), ['(' num2str(x(i)) ', ' num2str(y(i)) ')']);
end
This code iterates through each point, placing a text annotation next to it that displays its coordinates. This feature is particularly useful in presentations where precise values are critical.
Combining Multiple Plots
Combining different types of plots can create a more informative and visually engaging representation of your data. MATLAB makes it straightforward to overlay various graphs.
Here’s an example of how to overlay a scatter plot on a line plot:
hold on; % Keep the current graph
scatter(x, y, 100, 'filled'); % Scatter plot
plot(x, y, 'r--'); % Line plot
legend('Data Points', 'Connecting Line');
hold off; % Release the graph
Using `hold on` allows you to add more graphic elements to an existing plot without erasing it. The `legend` function helps distinguish between the various plotted elements, enhancing clarity for the viewer.
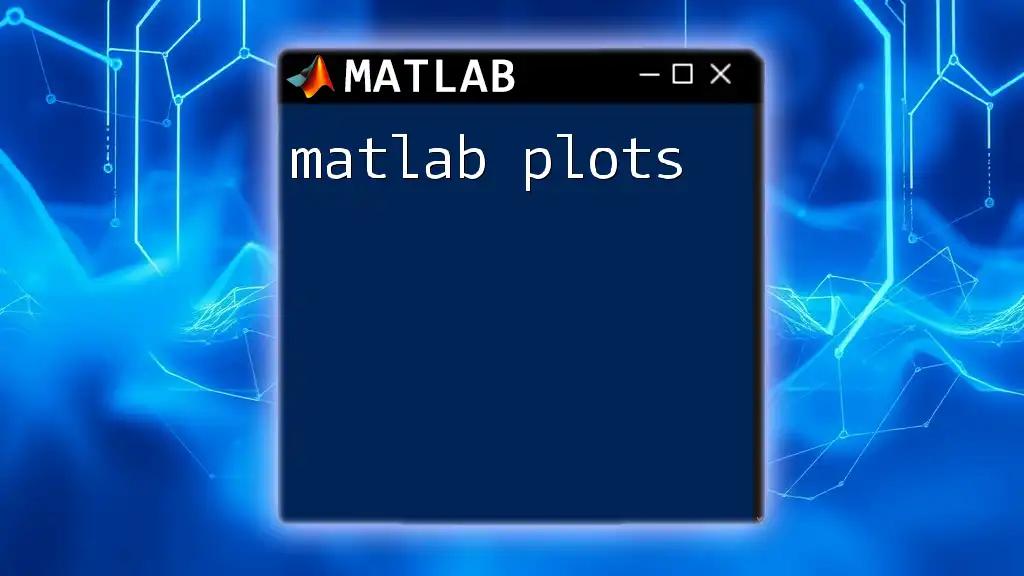
Troubleshooting Common Issues
Common Plotting Errors and Solutions
When working with MATLAB plots, errors may arise ranging from simple typos to more complex issues such as dimension mismatches. A common error is attempting to plot vectors or matrices of different lengths. Always ensure that the vectors passed to the `plot()` or `scatter()` functions have equal dimensions.
Performance Tips for Large Datasets
When plotting large datasets, performance can become an issue. Here are a few best practices to enhance performance:
- Downsample the data: If your dataset is too large, consider downsampling while maintaining the integrity of the information.
- Use built-in functions: MATLAB provides optimized functions that handle large data efficiently, so avoid looping through data points where possible.
- Limit visual elements: Reducing the number of markers or using simpler plot types can help boost performance.
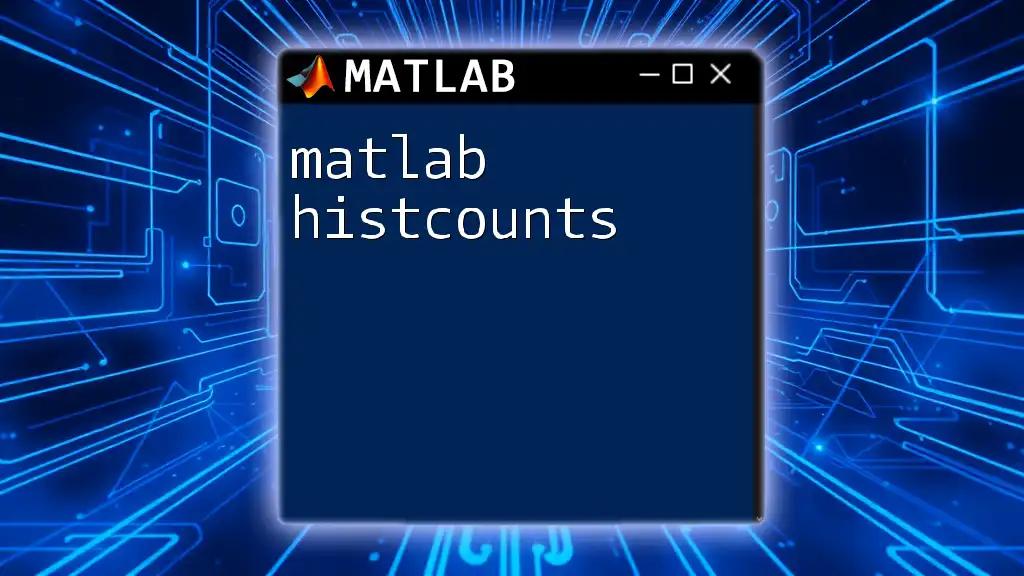
Conclusion
In this article, we explored the fundamental aspects of how to effectively matlab plot points. We covered basic 2D and 3D plotting, customization of markers, advanced plotting techniques, and troubleshooting practices. Mastering these techniques unlocks the full potential of MATLAB's visualization capabilities, enabling you to present your data in clear, informative ways. Consider experimenting with more datasets to hone your skills further and explore additional customization features in MATLAB.
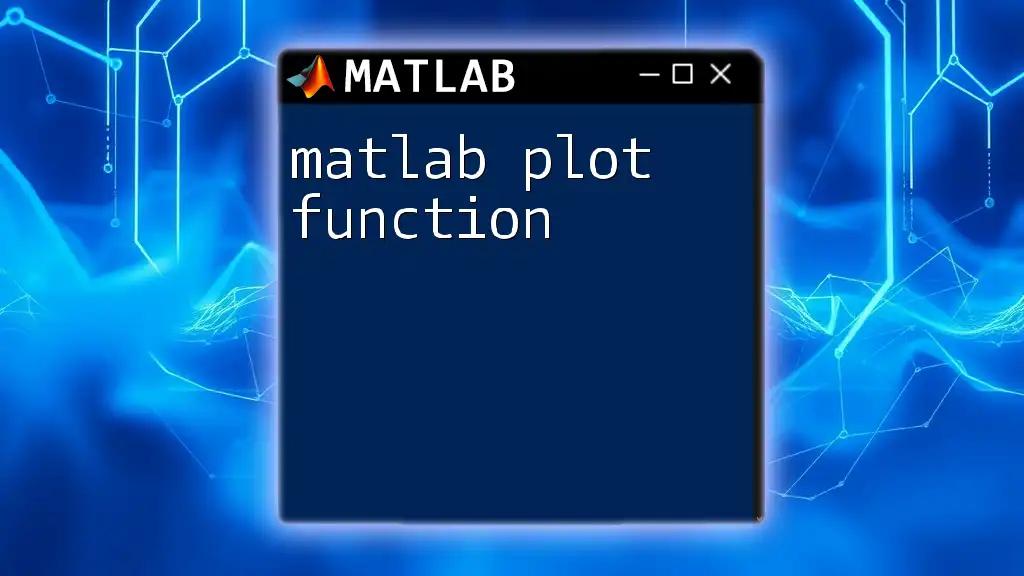
Additional Resources
For further exploration, refer to the [MATLAB documentation](https://www.mathworks.com/help/matlab/visualize.html) and seek out tutorials or courses specifically designed for enhancing your MATLAB proficiency. Happy plotting!